How to Get Deals with the Zoho CRM API in Python
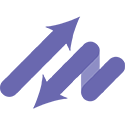
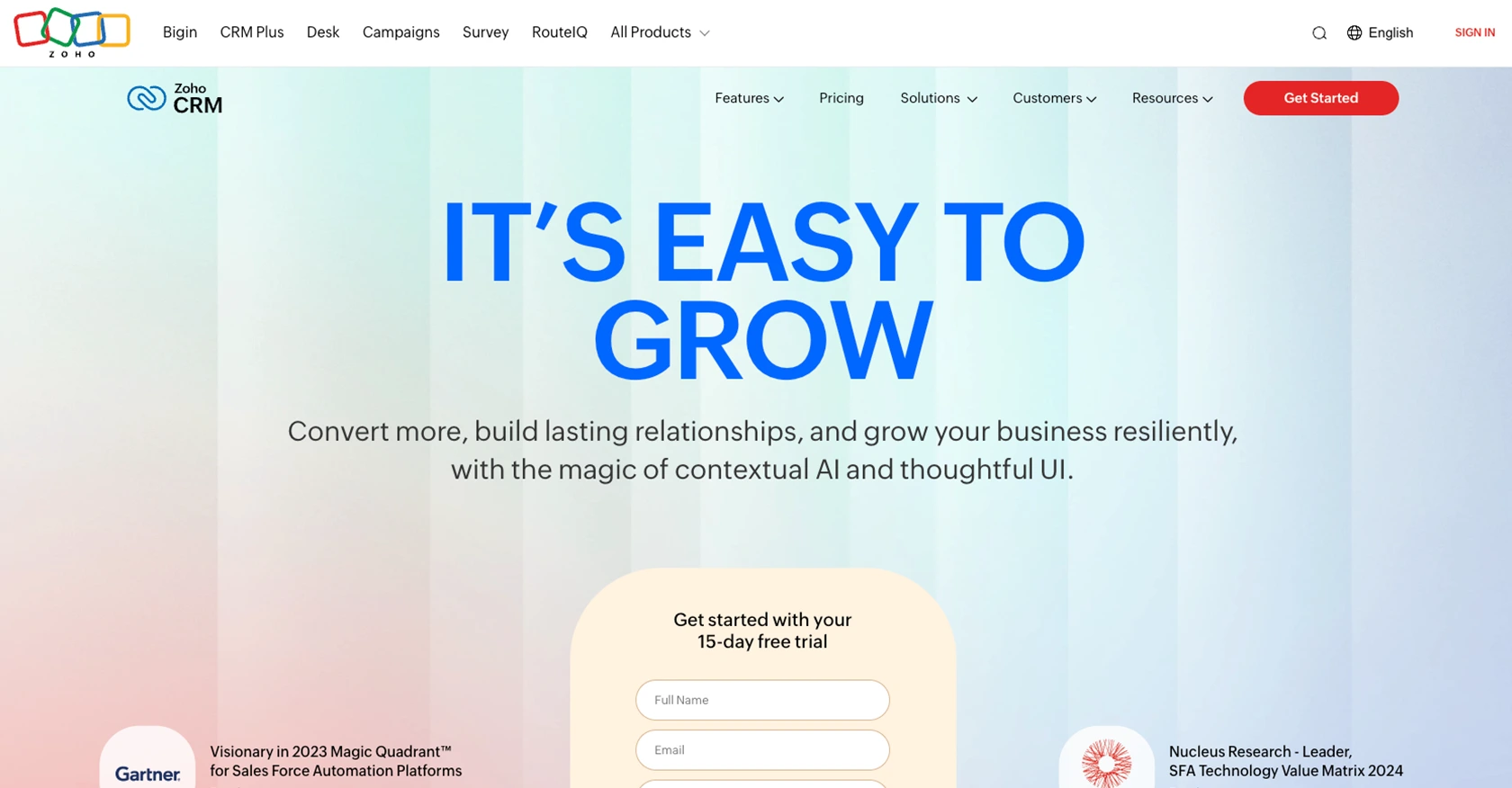
Introduction to Zoho CRM
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and support in a unified system. Known for its flexibility and robust features, Zoho CRM is a popular choice for organizations seeking to enhance their customer engagement and streamline operations.
Developers often integrate with Zoho CRM to access and manipulate customer data, such as deals, to optimize sales processes and improve decision-making. For example, a developer might use the Zoho CRM API to retrieve deal information and analyze sales trends, enabling more targeted marketing strategies and efficient resource allocation.
Setting Up Your Zoho CRM Test/Sandbox Account
Before you can start integrating with the Zoho CRM API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Zoho CRM Account
If you don't already have a Zoho CRM account, you can sign up for a free trial on the Zoho CRM website. Follow the instructions to create your account. Once your account is set up, you can log in to access the CRM dashboard.
Registering a Client Application for OAuth Authentication
Zoho CRM uses OAuth 2.0 for authentication, which requires you to register your application to obtain the necessary credentials.
- Go to the Zoho Developer Console.
- Select the appropriate client type for your application (e.g., Web Based, Mobile).
- Enter the required details:
- Client Name: The name of your application.
- Homepage URL: The URL of your application's homepage.
- Authorized Redirect URIs: The URL where Zoho will redirect after authentication.
- Click Create to register your application.
Upon successful registration, you will receive a Client ID and Client Secret. Keep these credentials secure as they are essential for making authenticated API requests.
Generating Access and Refresh Tokens
With your client credentials, you can now generate access and refresh tokens to authenticate API requests.
- Make an authorization request to Zoho's OAuth server using your client credentials and redirect URI.
- Once authorized, you'll receive an authorization code at your redirect URI.
- Exchange this authorization code for access and refresh tokens by making a POST request to Zoho's token endpoint.
Refer to the Zoho CRM OAuth documentation for detailed steps on generating tokens.
Configuring API Scopes
Ensure that your application has the necessary scopes to access the Zoho CRM modules you intend to use. For retrieving deals, you will need scopes such as ZohoCRM.modules.deals.READ
.
For more information on scopes, visit the Zoho CRM Scopes documentation.
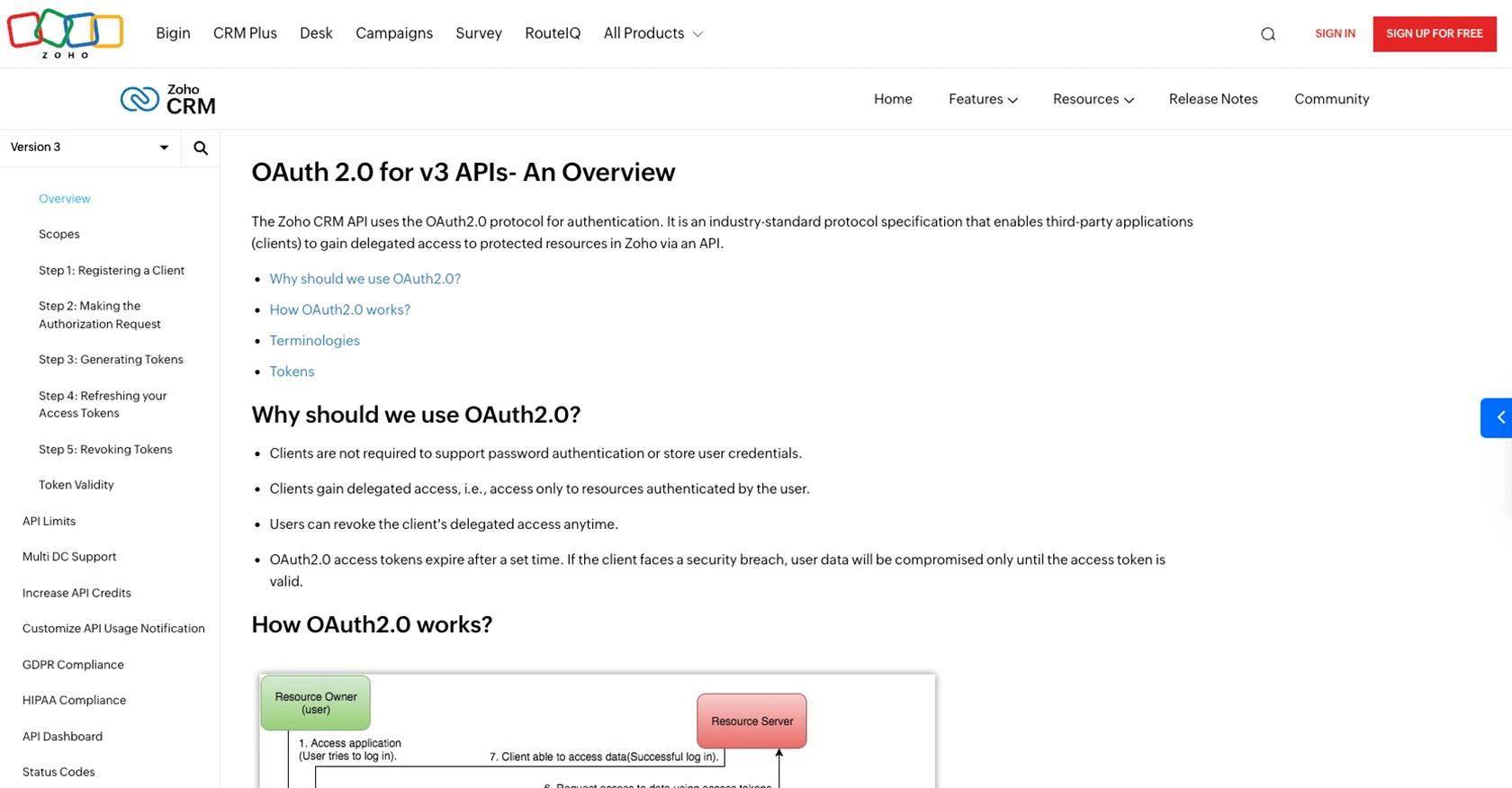
sbb-itb-96038d7
Making API Calls to Retrieve Deals from Zoho CRM Using Python
To interact with the Zoho CRM API and retrieve deals, you'll need to use Python. This section will guide you through setting up your environment, writing the code, and handling responses effectively.
Setting Up Your Python Environment for Zoho CRM API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
pip install requests
Writing Python Code to Retrieve Deals from Zoho CRM
Create a new Python file named get_zoho_deals.py
and add the following code:
import requests
# Define the API endpoint and headers
endpoint = "https://www.zohoapis.com/crm/v3/Deals"
headers = {
"Authorization": "Zoho-oauthtoken Your_Access_Token"
}
# Make a GET request to the Zoho CRM API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
deals = response.json().get('data', [])
for deal in deals:
print(f"Deal Name: {deal.get('Deal_Name')}, Amount: {deal.get('Amount')}")
else:
print(f"Failed to retrieve deals: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the access token you obtained during the OAuth setup.
Understanding the Zoho CRM API Response
When the API call is successful, the response will contain a JSON object with deal data. The code above extracts the deal name and amount for each deal and prints them to the console.
If the request fails, the code will print an error message with the status code and response text, helping you diagnose the issue.
Verifying API Call Success in Zoho CRM Sandbox
After running your script, log in to your Zoho CRM sandbox account to verify the retrieved deals. Ensure the data matches the expected results from your API call.
Handling Errors and Rate Limits in Zoho CRM API
It's crucial to handle potential errors and respect Zoho CRM's rate limits. Common error codes include:
- INVALID_MODULE: Ensure the module name is correct.
- TOKEN_BOUND_DATA_MISMATCH: Verify your page token and parameters.
- NO_PERMISSION: Check user permissions for accessing records.
Zoho CRM enforces rate limits based on API credits. Refer to the API Limits documentation for more details.
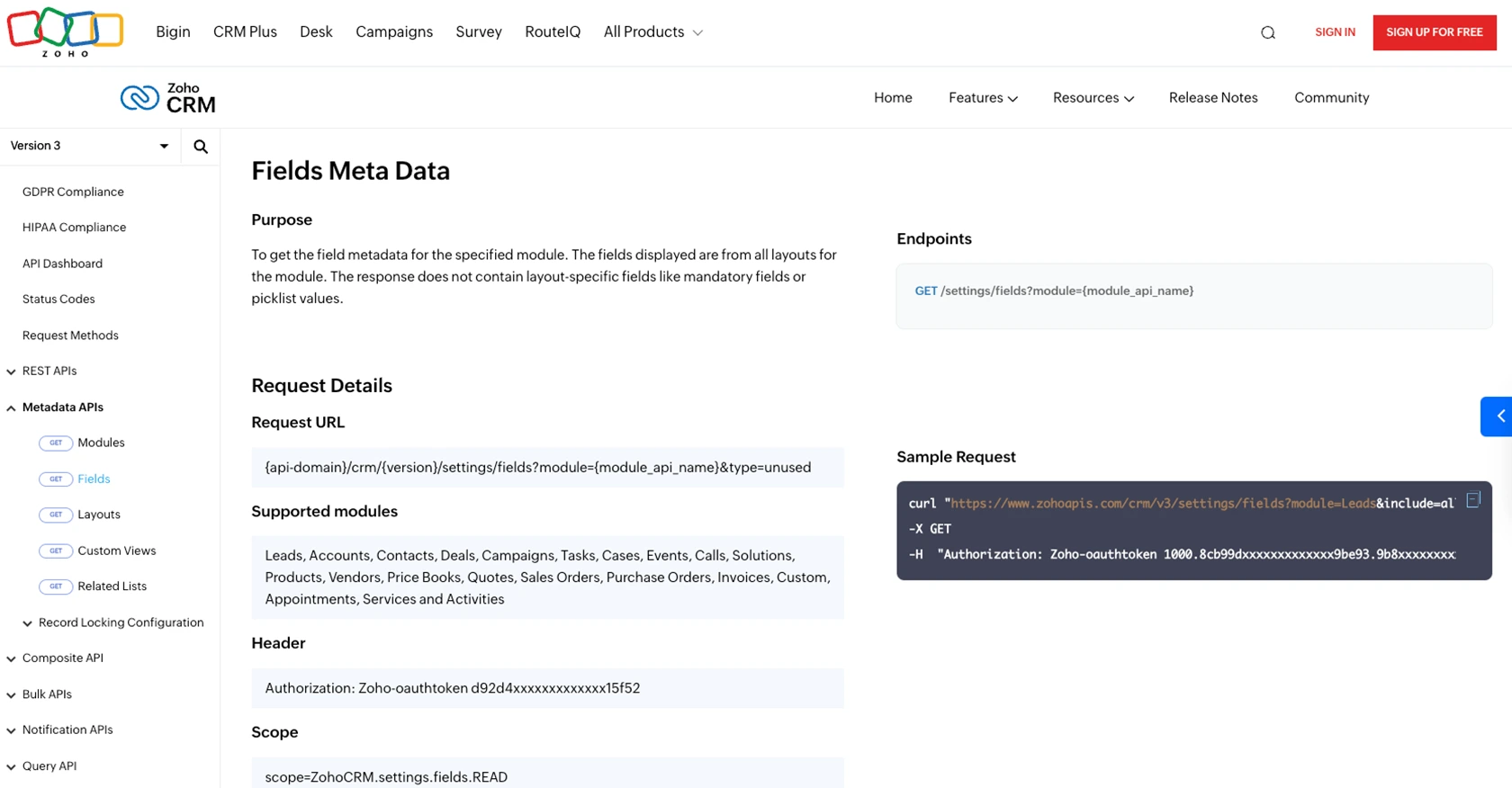
Conclusion and Best Practices for Zoho CRM API Integration
Integrating with the Zoho CRM API using Python provides a powerful way to manage and analyze your sales data efficiently. By following the steps outlined in this guide, you can successfully retrieve deal information and leverage it to enhance your business strategies.
Best Practices for Secure and Efficient Zoho CRM API Usage
- Secure Storage of Credentials: Always store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code. Consider using environment variables or a secure vault.
- Handling Rate Limits: Be mindful of Zoho CRM's API rate limits. Implement retry logic with exponential backoff to handle rate limit errors gracefully. Refer to the API Limits documentation for more details.
- Data Standardization: Ensure that the data retrieved from Zoho CRM is standardized and transformed as needed for your application. This will help maintain data consistency across different systems.
- Error Handling: Implement robust error handling to manage various API errors. Use the error codes provided by Zoho CRM to diagnose and resolve issues effectively.
Streamlining Integrations with Endgrate
While building integrations with Zoho CRM can be rewarding, it can also be time-consuming and complex. Endgrate offers a solution to simplify this process by providing a unified API endpoint for multiple platforms, including Zoho CRM. This allows you to focus on your core product while outsourcing integration tasks.
With Endgrate, you can build once for each use case and avoid the hassle of maintaining multiple integrations. This not only saves time and resources but also enhances the integration experience for your customers.
Explore how Endgrate can help streamline your integration processes by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/get-records.html
Ready to get started?