Using the Sage Accounting API to Create or Update Item (with PHP examples)
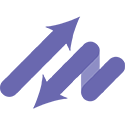
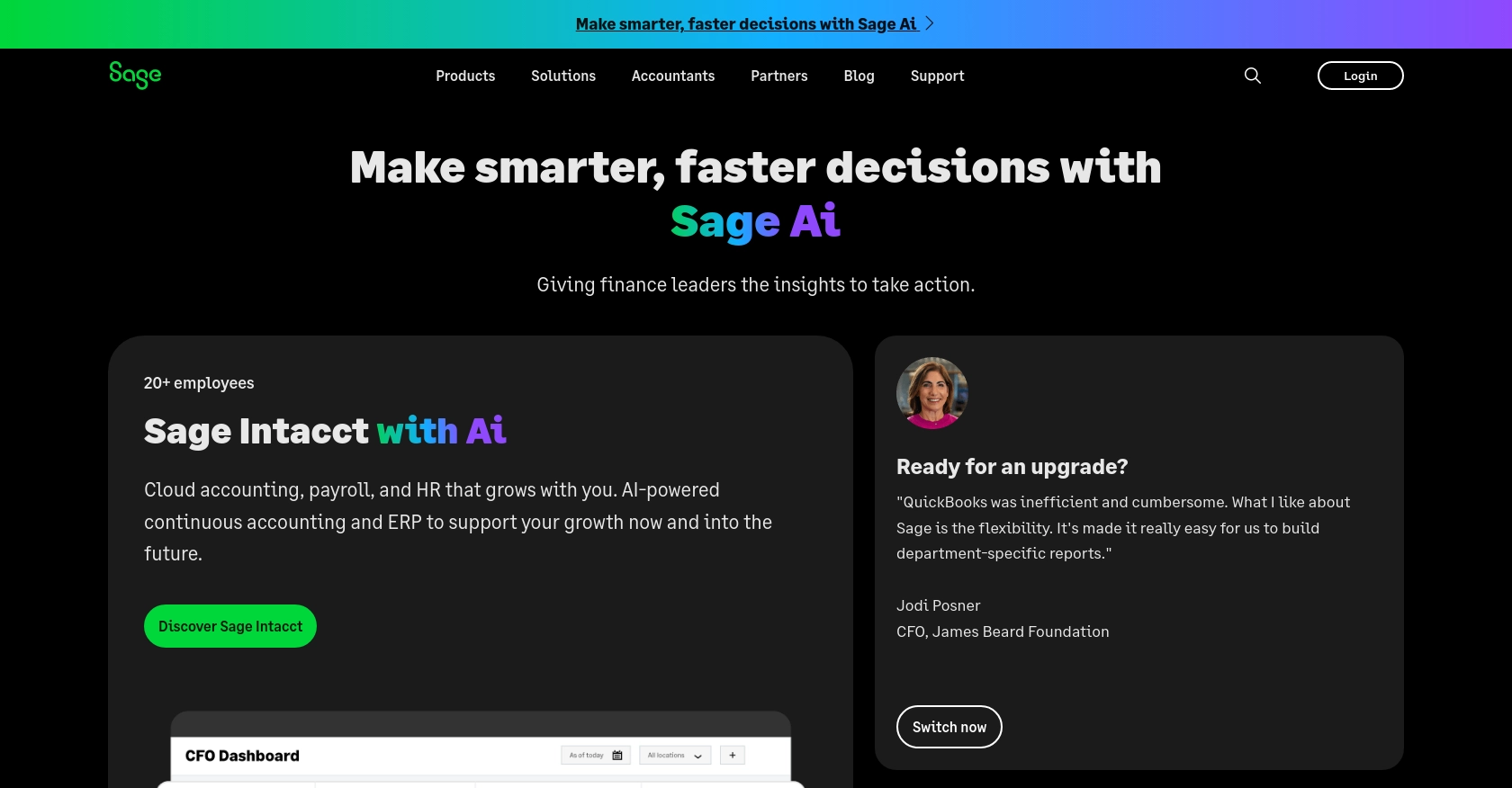
Introduction to Sage Accounting API
Sage Accounting is a comprehensive cloud-based accounting solution tailored for small to medium-sized businesses. It offers a suite of tools to manage finances, track expenses, and streamline accounting processes. With its robust features, Sage Accounting is a popular choice for businesses looking to enhance their financial management capabilities.
Developers often seek to integrate with the Sage Accounting API to automate and optimize various accounting tasks. For example, using the API to create or update items in the inventory can significantly reduce manual data entry and improve accuracy. This integration can be particularly beneficial for businesses that need to manage a large volume of products or services efficiently.
In this article, we will explore how to interact with the Sage Accounting API using PHP to create or update items. This guide will provide step-by-step instructions and practical examples to help developers seamlessly integrate Sage Accounting into their applications.
Setting Up Your Sage Accounting Test/Sandbox Account
Before diving into the integration process, it's essential to set up a Sage Accounting test or sandbox account. This environment allows developers to experiment and test API interactions without affecting live data. Follow these steps to get started:
Create a Sage Developer Account
To begin, you'll need a Sage Developer account. This account enables you to register and manage applications, obtain client credentials, and configure essential details like callback URLs.
- Visit the Sage Developer Portal.
- Sign up using your GitHub account or an email address.
- Follow the guide on creating an app to complete your registration.
Set Up a Trial Business for Development
Next, create a trial business account to test your integration:
- Navigate to the Sage Accounting Quick Start Guide.
- Select the appropriate region and subscription tier for your trial business.
- Use email services that support aliasing to manage multiple environments efficiently.
Create a Sage Accounting App for OAuth Authentication
Since the Sage Accounting API uses OAuth for authentication, you'll need to create an app to obtain the necessary credentials:
- Log in to your Sage Developer account.
- Click on "Create App" and provide a name and callback URL for your app.
- Save the app to generate a Client ID and Client Secret, which you'll use for authentication.
Upgrade to a Developer Account
To fully utilize the Sage Accounting API, upgrade your trial account to a developer account:
- Submit a request through the Upgrade Your Account page.
- Provide details such as your name, email, app name, and Client ID.
- Wait for confirmation, which typically takes 3-5 working days.
With your Sage Accounting sandbox account set up, you're ready to start integrating and testing API calls in a safe environment.
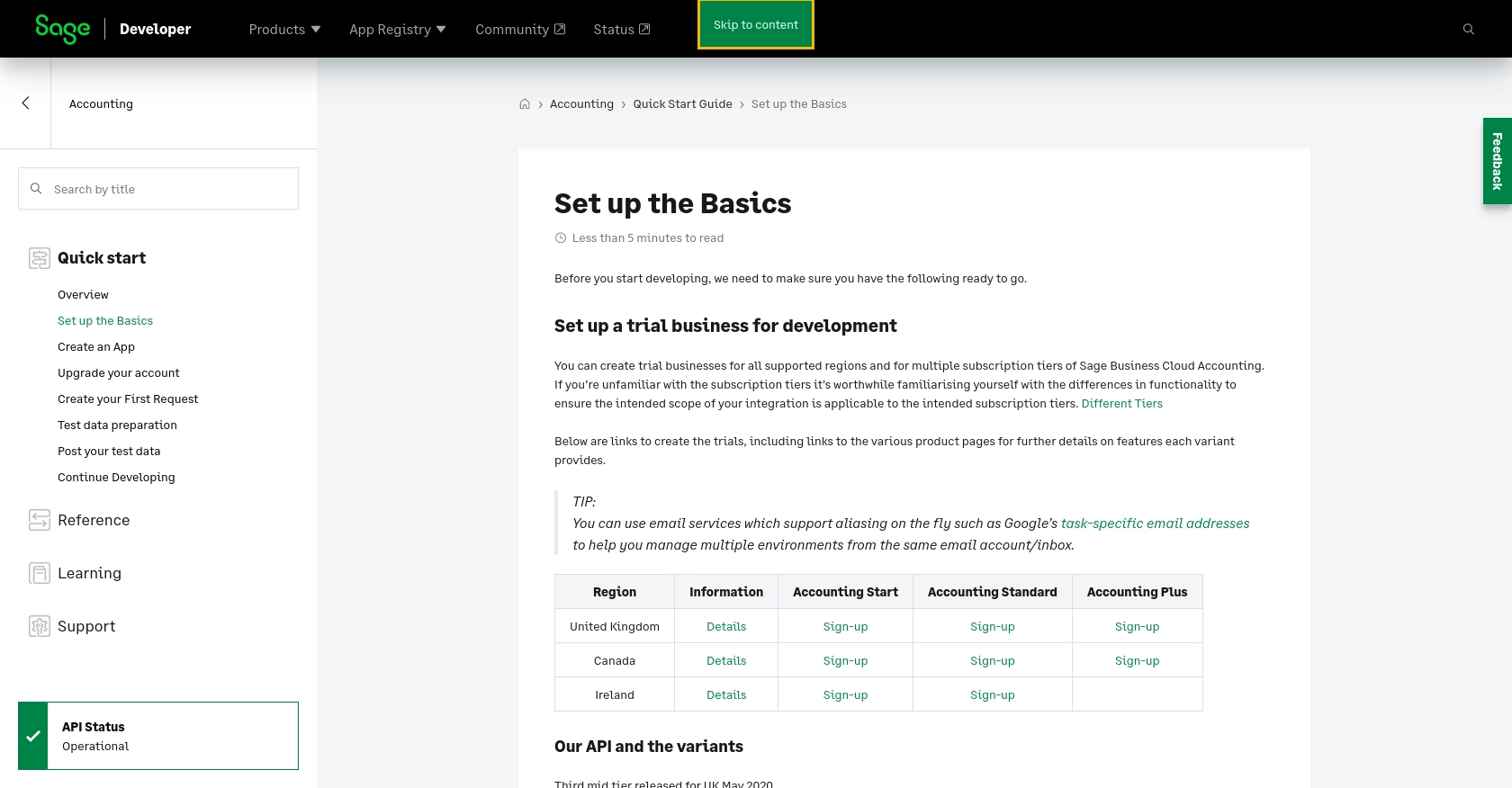
sbb-itb-96038d7
Making API Calls to Create or Update Items in Sage Accounting Using PHP
In this section, we'll guide you through making API calls to the Sage Accounting API using PHP. This will involve creating or updating items in your Sage Accounting inventory. We'll cover the necessary PHP setup, dependencies, and provide example code to help you get started.
Setting Up PHP Environment for Sage Accounting API Integration
Before making API calls, ensure your PHP environment is correctly set up. You'll need:
- PHP 7.4 or higher
- Composer for dependency management
Install the required dependencies using Composer:
composer require guzzlehttp/guzzle
The Guzzle HTTP client will help you make HTTP requests to the Sage Accounting API.
Creating a New Item in Sage Accounting with PHP
To create a new item in Sage Accounting, you'll need to make a POST request to the API endpoint. Here's a sample PHP script to create an item:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token';
$response = $client->post('https://api.accounting.sage.com/v3.1/products', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json'
],
'json' => [
'product' => [
'description' => 'New Product',
'item_code' => 'NP001',
'sales_ledger_account_id' => '12345',
'purchase_ledger_account_id' => '67890',
'active' => true
]
]
]);
$data = json_decode($response->getBody(), true);
echo 'Product Created: ' . $data['id'];
Replace Your_Access_Token
with the token obtained during OAuth authentication. This script sends a POST request to create a new product, and upon success, it prints the product ID.
Updating an Existing Item in Sage Accounting with PHP
To update an existing item, you'll need to make a PUT request. Here's how you can update an item using PHP:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token';
$productKey = 'Existing_Product_Key';
$response = $client->put("https://api.accounting.sage.com/v3.1/products/{$productKey}", [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json'
],
'json' => [
'product' => [
'description' => 'Updated Product Description',
'active' => false
]
]
]);
$data = json_decode($response->getBody(), true);
echo 'Product Updated: ' . $data['id'];
Replace Existing_Product_Key
with the key of the product you wish to update. This script updates the product's description and active status.
Handling API Responses and Errors
After making API calls, it's crucial to handle responses and potential errors. Check the response status code to verify success:
201
- Created successfully200
- Updated successfully400
- Bad request, check your input data401
- Unauthorized, check your access token
Use try-catch blocks in PHP to handle exceptions and log errors for troubleshooting.
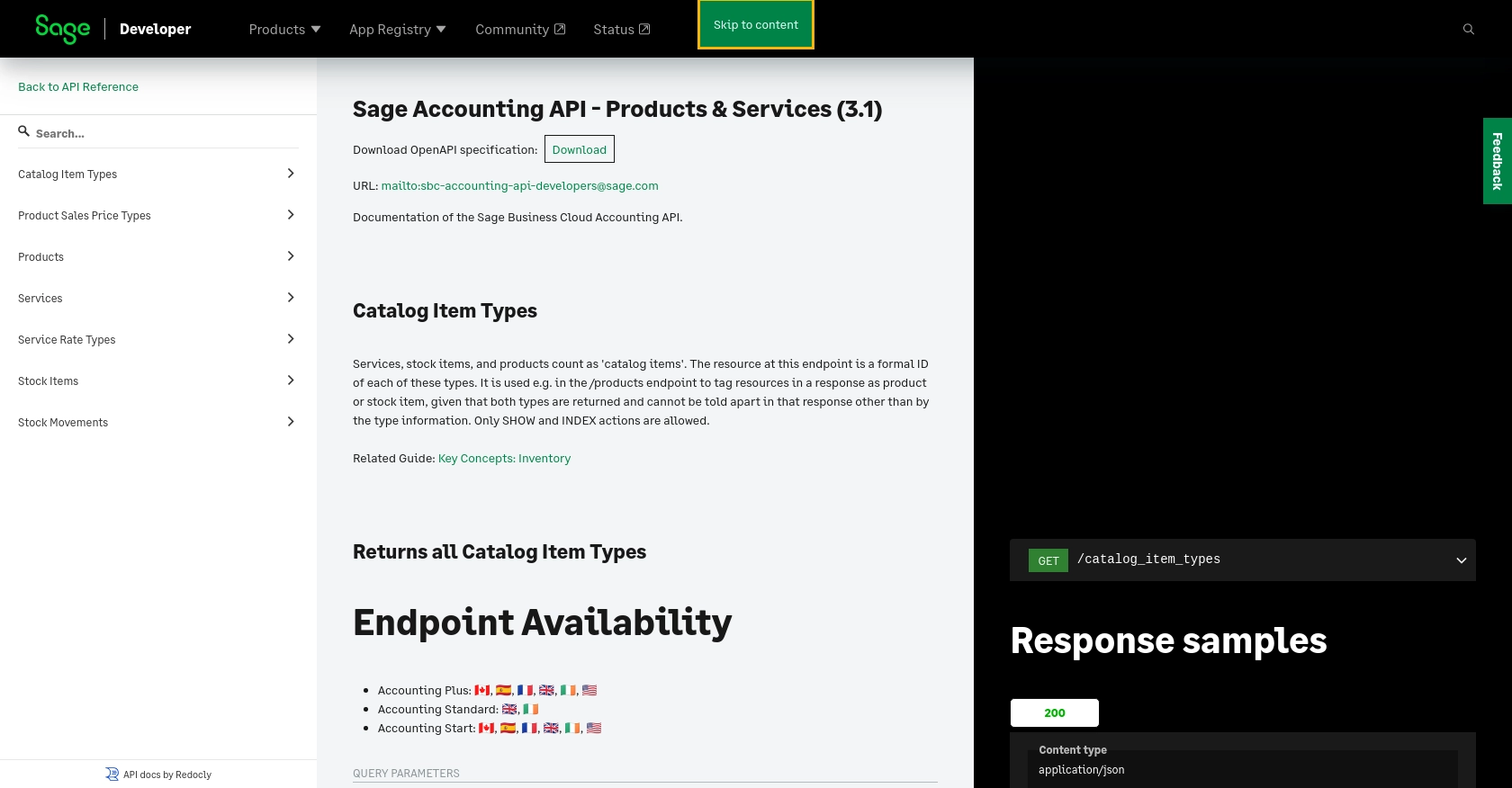
Conclusion and Best Practices for Integrating with Sage Accounting API
Integrating with the Sage Accounting API using PHP can significantly enhance your business's financial management capabilities by automating the creation and updating of inventory items. This integration not only streamlines processes but also reduces the risk of human error, ensuring more accurate and efficient accounting operations.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth credentials, such as Client ID and Client Secret, securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be aware of API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that data fields are standardized across your application to maintain consistency and avoid data discrepancies.
- Log and Monitor API Calls: Implement logging and monitoring for your API interactions to quickly identify and resolve issues. This will help in maintaining a robust integration.
Leverage Endgrate for Seamless Integration
While integrating with the Sage Accounting API can be highly beneficial, it can also be complex and time-consuming. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to multiple platforms, including Sage Accounting. By using Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Provide an easy, intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration needs by visiting Endgrate and discover a more efficient way to manage your business integrations.
Read More
- https://endgrate.com/provider/sage
- https://developer.sage.com/accounting/quick-start/set-up-the-basics/
- https://developer.sage.com/accounting/quick-start/create-an-app/
- https://developer.sage.com/accounting/quick-start/upgrade-your-account/
- https://developer.sage.com/accounting/reference/products-services/
Ready to get started?