Using the Sage Accounting API to Create or Update Customers (with Javascript examples)
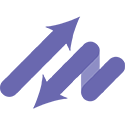
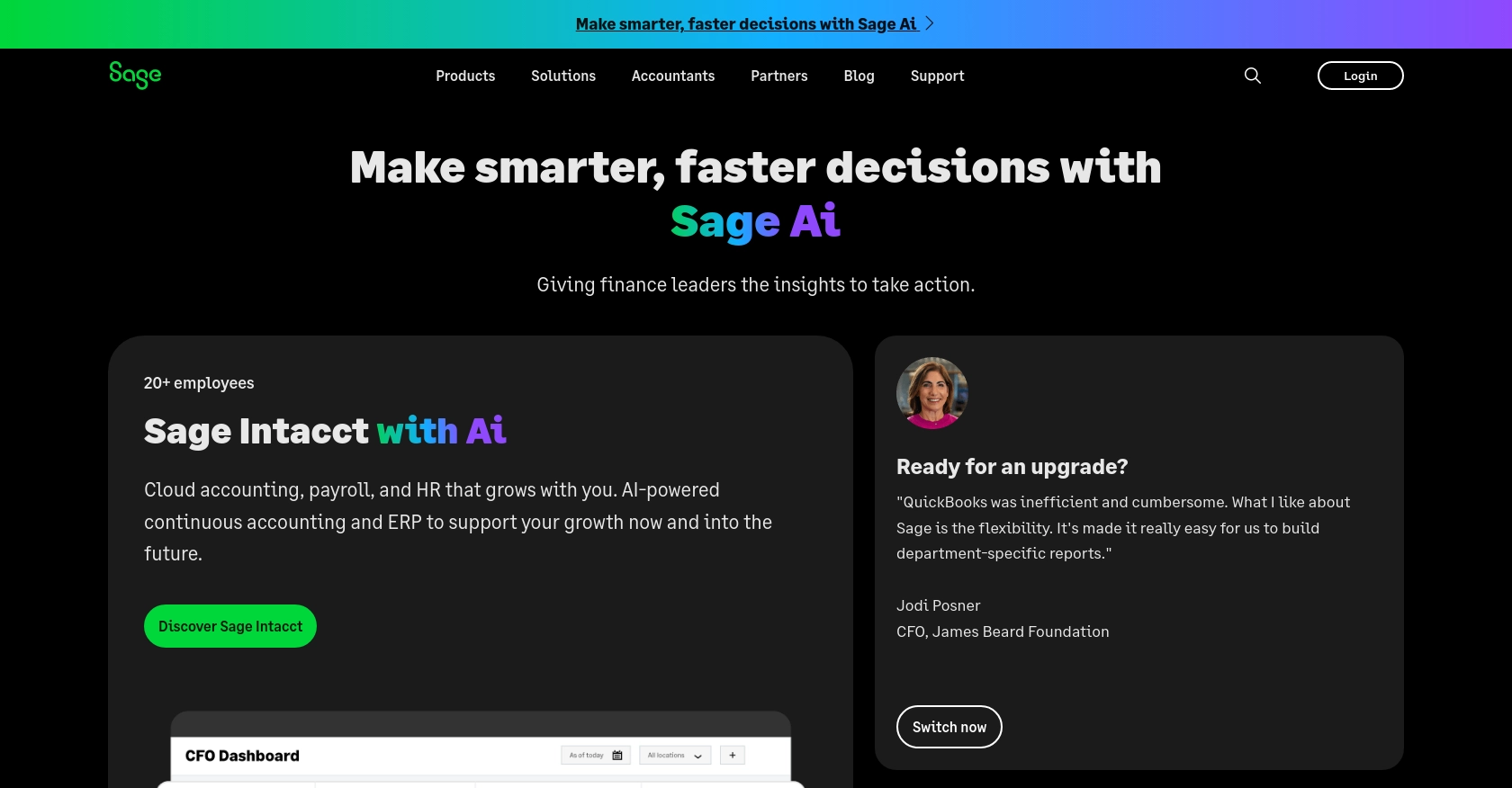
Introduction to Sage Accounting API
Sage Accounting is a robust cloud-based accounting solution tailored for small to medium-sized businesses. It offers a comprehensive suite of tools to manage financial transactions, invoicing, and customer relationships, all within a user-friendly interface.
Integrating with the Sage Accounting API allows developers to automate and streamline accounting processes, such as creating or updating customer records. For example, a developer might use the Sage Accounting API to automatically update customer information from an e-commerce platform, ensuring that financial records are always up-to-date and accurate.
Setting Up Your Sage Accounting Test/Sandbox Account
Before you begin integrating with the Sage Accounting API, it's essential to set up a test or sandbox account. This environment allows you to safely develop and test your application without affecting live data.
Create a Sage Developer Account
To access the Sage Accounting API, you first need to create a Sage Developer account. This account will enable you to register and manage your applications, obtain client credentials, and specify key details such as callback URLs.
- Visit the Sage Developer portal.
- Sign up using your GitHub account or an email address.
- Follow the guide on creating an app to obtain your Client ID and Client Secret.
Set Up a Trial Business for Development
Sage allows you to create trial businesses for development purposes. This is crucial for testing your integration without impacting real data.
- Navigate to the trial business setup page.
- Select your region and the appropriate subscription tier (e.g., Accounting Start, Accounting Standard).
- Sign up for a trial account using an email service that supports aliasing to manage multiple environments efficiently.
Upgrade to a Developer Account
Once your trial account is set up, you can request an upgrade to a developer account. This upgrade provides 12 months of free access for testing your integration.
- Fill out the upgrade form with your trial account details, including your name, email address, app name, and Client ID.
- Submit the form and wait for confirmation, which typically takes 3-5 working days.
- For more details, refer to the upgrade guide.
OAuth Authentication Setup
The Sage Accounting API uses OAuth for authentication. Follow these steps to set up OAuth:
- Log in to your Sage Developer account and navigate to the app you created.
- Ensure you have your Client ID and Client Secret ready.
- Set up your callback URL as specified in the authentication guide.
With your test account and OAuth setup, you're ready to start integrating with the Sage Accounting API.
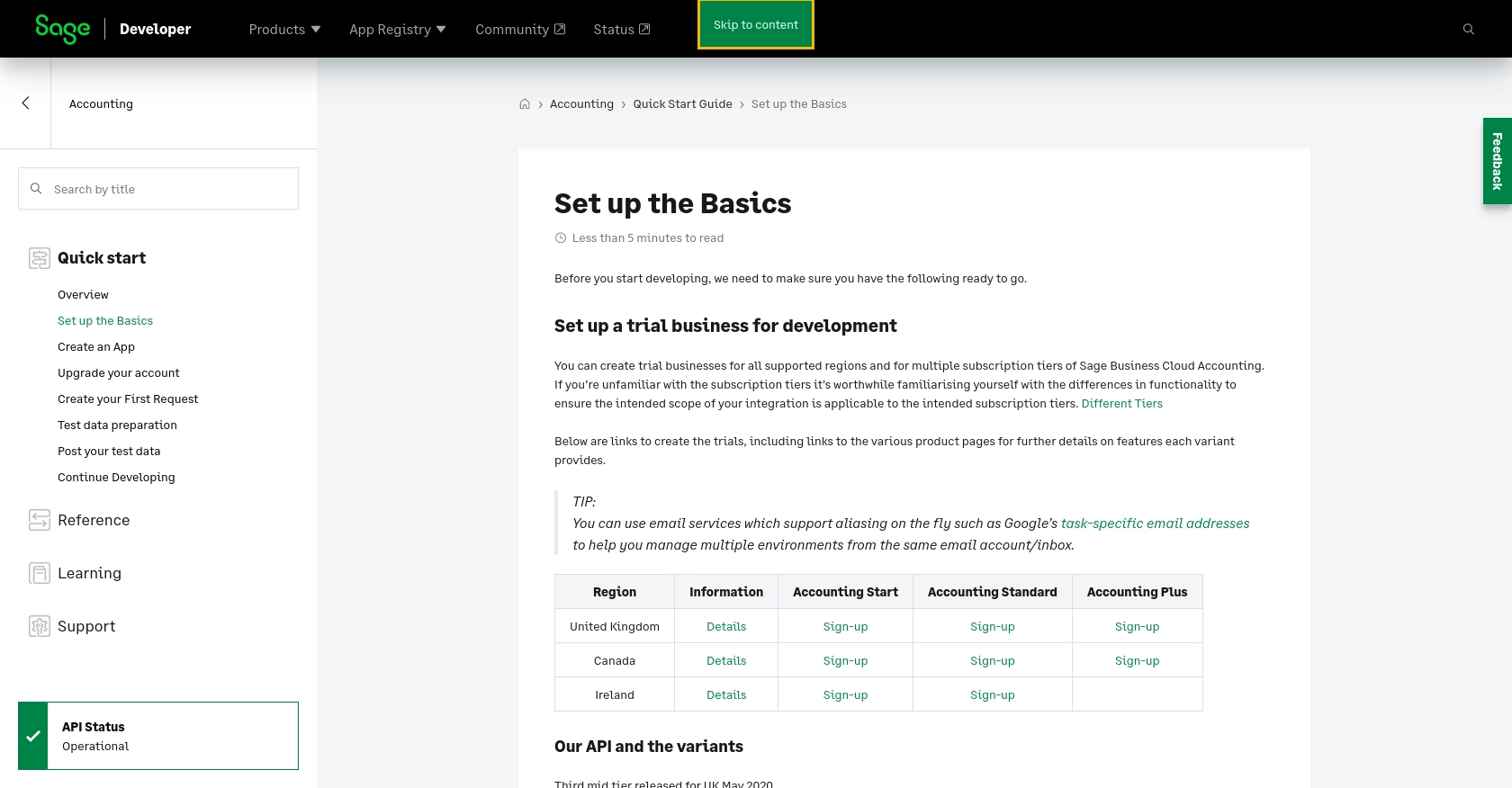
sbb-itb-96038d7
Making API Calls to Create or Update Customers in Sage Accounting Using JavaScript
To interact with the Sage Accounting API for creating or updating customer records, you'll need to use JavaScript to make HTTP requests. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses and errors.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have Node.js installed, as it provides the runtime for executing JavaScript outside the browser. Additionally, you'll need the axios
library to simplify HTTP requests.
- Install Node.js from the official website.
- Open your terminal and run the following command to install
axios
:
npm install axios
Creating a New Customer with Sage Accounting API
To create a new customer, you'll need to make a POST request to the Sage Accounting API's contacts endpoint. Below is a sample code snippet to achieve this:
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://api.sage.com/accounting/contacts';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Define the customer data
const customerData = {
contact: {
name: 'John Doe',
email: 'john.doe@example.com',
phone: '123-456-7890'
}
};
// Make the POST request
axios.post(endpoint, customerData, { headers })
.then(response => {
console.log('Customer created successfully:', response.data);
})
.catch(error => {
console.error('Error creating customer:', error.response.data);
});
Replace YOUR_ACCESS_TOKEN
with the actual token obtained during the OAuth setup. The above code sends a POST request with customer details, and upon success, logs the response data.
Updating an Existing Customer with Sage Accounting API
To update an existing customer, you'll need to make a PUT request to the same endpoint, specifying the customer's ID. Here's how you can do it:
const customerId = 'CUSTOMER_ID'; // Replace with actual customer ID
const updateData = {
contact: {
name: 'Jane Doe',
email: 'jane.doe@example.com'
}
};
// Make the PUT request
axios.put(`${endpoint}/${customerId}`, updateData, { headers })
.then(response => {
console.log('Customer updated successfully:', response.data);
})
.catch(error => {
console.error('Error updating customer:', error.response.data);
});
Ensure to replace CUSTOMER_ID
with the actual ID of the customer you wish to update. This code updates the customer's information and logs the result.
Handling API Responses and Errors
When making API calls, it's crucial to handle responses and errors effectively. The Sage Accounting API provides status codes that indicate the success or failure of your requests. Common status codes include:
- 200 OK: The request was successful.
- 201 Created: A new resource was successfully created.
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 404 Not Found: The requested resource could not be found.
Always check the response status and handle errors gracefully to ensure a robust integration.
For more detailed information on the API endpoints and request structures, refer to the Sage Accounting API documentation.
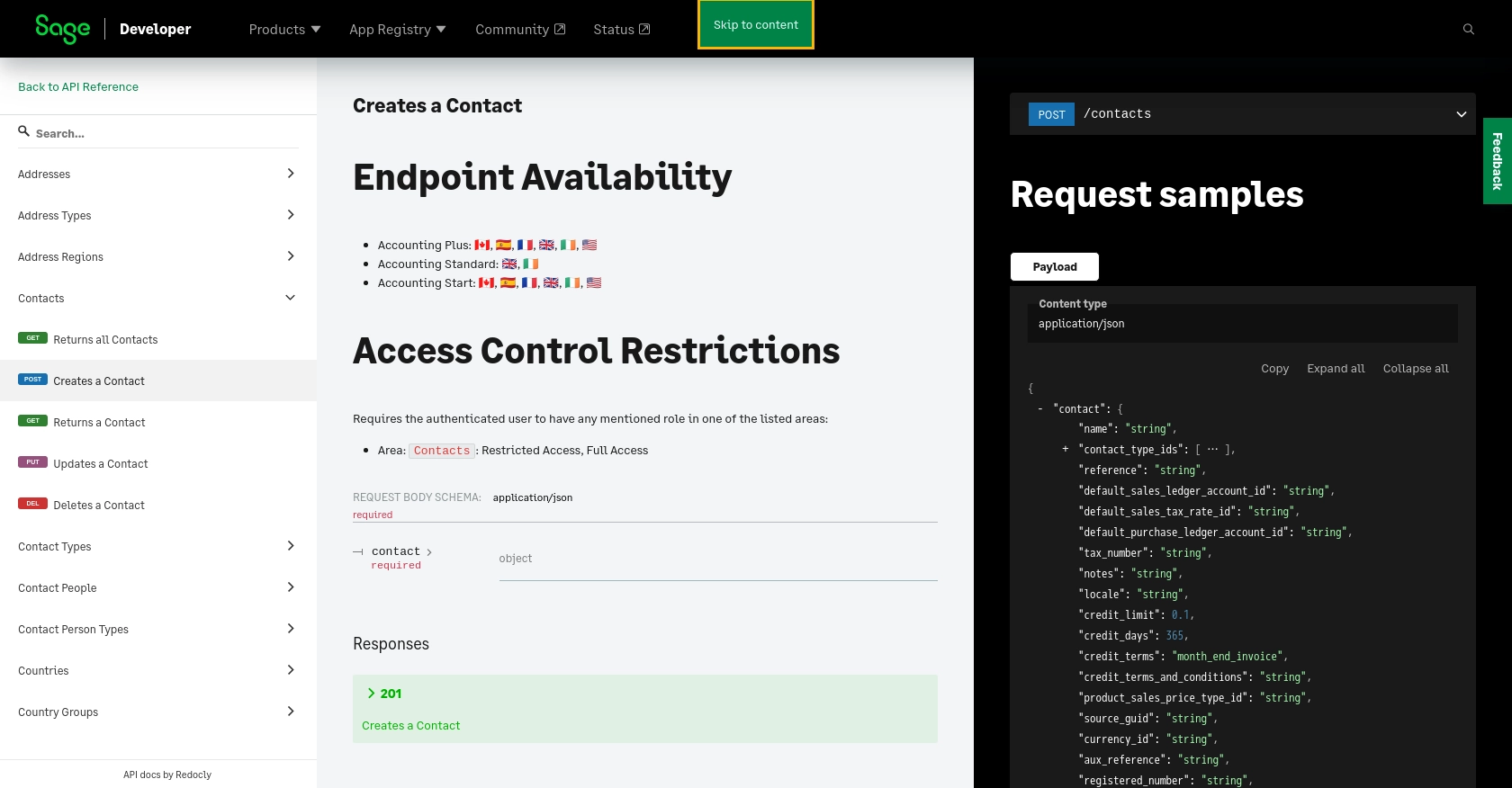
Conclusion and Best Practices for Integrating with Sage Accounting API
Integrating with the Sage Accounting API using JavaScript can significantly enhance your ability to manage customer data efficiently. By automating the creation and updating of customer records, you can ensure that your financial data remains accurate and up-to-date, reducing manual errors and saving valuable time.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your OAuth credentials securely. Consider using environment variables or secure vaults to keep your Client ID and Client Secret safe from unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Sage Accounting API. Implement retry logic with exponential backoff to handle rate limit errors gracefully and avoid overwhelming the API.
- Data Standardization: Ensure that customer data is standardized before sending it to the API. This includes consistent formatting for names, emails, and phone numbers to maintain data integrity across systems.
- Error Handling: Implement robust error handling to manage different response codes effectively. Log errors for troubleshooting and provide meaningful feedback to users when issues arise.
Streamline Your Integration Process with Endgrate
While integrating with individual APIs like Sage Accounting can be beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Sage Accounting.
By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations, ensuring consistency and efficiency.
- Provide an easy, intuitive integration experience for your customers, enhancing user satisfaction and retention.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined, unified API approach.
Read More
- https://endgrate.com/provider/sage
- https://developer.sage.com/accounting/quick-start/set-up-the-basics/
- https://developer.sage.com/accounting/quick-start/create-an-app/
- https://developer.sage.com/accounting/quick-start/upgrade-your-account/
- https://developer.sage.com/accounting/reference/contacts/#tag/Contacts/operation/postContacts
Ready to get started?