Using the Hubspot API to Get Companies (with PHP examples)
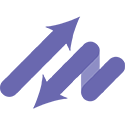
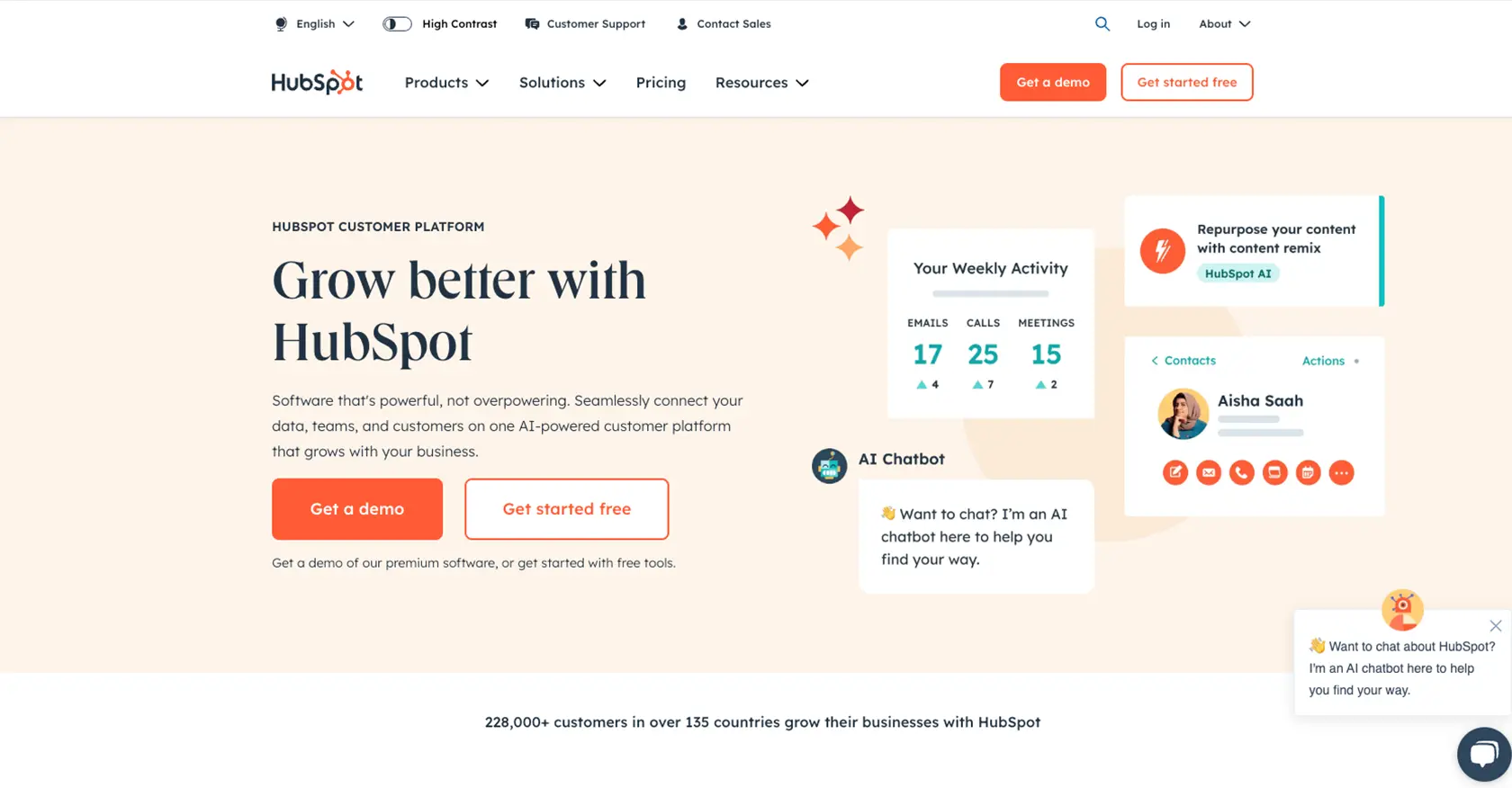
Introduction to HubSpot API for Company Data Integration
HubSpot is a comprehensive CRM platform that offers a suite of tools to help businesses manage their marketing, sales, and customer service efforts. Its robust API allows developers to integrate seamlessly with HubSpot's CRM functionalities, enabling the automation and customization of business processes.
Developers may want to connect with HubSpot's API to access and manage company data, which can be crucial for maintaining up-to-date business records and enhancing customer relationship management. For example, a developer might use the HubSpot API to retrieve company information and integrate it with an internal analytics system to gain insights into customer interactions and business performance.
This article will guide you through using PHP to interact with the HubSpot API to retrieve company data. You'll learn how to set up your environment, authenticate your requests, and execute API calls to efficiently manage company records within HubSpot.
Setting Up Your HubSpot Test/Sandbox Account for API Integration
Before you can start interacting with the HubSpot API to retrieve company data using PHP, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Creating a HubSpot Developer Account
If you don't already have a HubSpot developer account, you'll need to create one. Follow these steps:
- Visit the HubSpot Developer Portal.
- Click on "Create a developer account" and fill in the required information.
- Once your account is created, log in to access the developer dashboard.
Setting Up a HubSpot Sandbox Environment
HubSpot provides a sandbox environment where you can test your integrations. To set it up:
- Navigate to the "Integrations" section in your HubSpot account.
- Select "Private Apps" and click on "Create a private app."
- Fill in the necessary details for your app, such as name and description.
Configuring OAuth Authentication for HubSpot API
Since HubSpot uses OAuth for authentication, you'll need to configure it for your app:
- In the app settings, navigate to the "Auth" tab.
- Select "OAuth" as the authentication method.
- Provide the necessary redirect URL and scopes. For accessing company data, ensure you include the
crm.objects.companies.read
scope. - Save your changes to generate the client ID and client secret.
Generating OAuth Tokens
To authenticate your API requests, you'll need to generate OAuth tokens:
- Use the client ID and client secret to request an access token from HubSpot's OAuth server.
- Refer to the HubSpot OAuth documentation for detailed steps on generating tokens.
With your test account and OAuth setup complete, you're ready to start making API calls to retrieve company data from HubSpot using PHP.
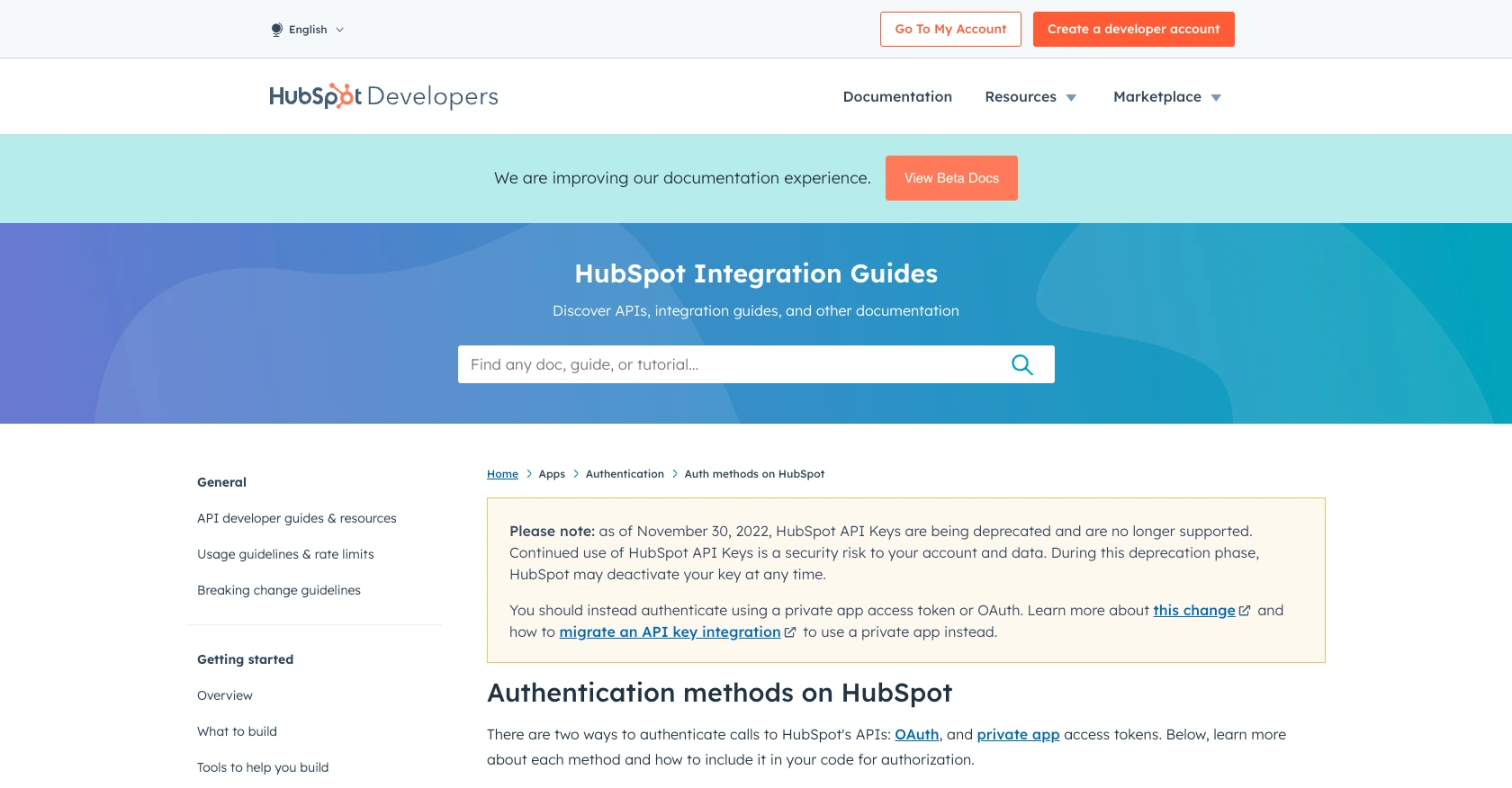
sbb-itb-96038d7
Executing HubSpot API Calls to Retrieve Company Data Using PHP
Now that you have your HubSpot sandbox environment and OAuth authentication set up, it's time to dive into making actual API calls to retrieve company data using PHP. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code to interact with the HubSpot API, and handling potential errors.
Setting Up Your PHP Environment for HubSpot API Integration
Before making API calls, ensure your PHP environment is correctly configured:
- Ensure you have PHP 7.4 or later installed on your system.
- Install the HubSpot PHP client library using Composer. Run the following command in your terminal:
composer require hubspot/api-client
Writing PHP Code to Retrieve Companies from HubSpot API
With your environment ready, you can now write the PHP code to interact with the HubSpot API. Below is an example script to retrieve company data:
<?php
require 'vendor/autoload.php';
use HubSpot\Client\Crm\Companies\ApiException;
use HubSpot\Factory;
// Initialize the HubSpot client
$client = Factory::createWithAccessToken('YOUR_ACCESS_TOKEN');
try {
// Retrieve companies
$response = $client->crm()->companies()->basicApi()->getPage();
foreach ($response->getResults() as $company) {
echo "Company ID: " . $company->getId() . "\n";
echo "Company Name: " . $company->getProperties()['name'] . "\n";
}
} catch (ApiException $e) {
echo "Exception when calling HubSpot API: ", $e->getMessage(), PHP_EOL;
}
?>
Replace YOUR_ACCESS_TOKEN
with the OAuth access token you generated earlier. This script initializes the HubSpot client, retrieves a list of companies, and prints out their IDs and names.
Verifying Successful API Requests in HubSpot Sandbox
After running the script, verify the request's success by checking the output in your terminal. The company data should match the records in your HubSpot sandbox environment. If you encounter any issues, ensure your access token is valid and that you have the correct scopes set.
Handling Errors and Understanding HubSpot API Error Codes
When making API calls, it's crucial to handle potential errors gracefully. The HubSpot API may return various error codes, such as:
- 401 Unauthorized: Indicates an issue with authentication. Check your access token.
- 429 Too Many Requests: You've hit the rate limit. Implement rate limiting strategies.
- 500 Internal Server Error: A server-side error occurred. Try again later.
Refer to the HubSpot API documentation for more details on error handling and codes.
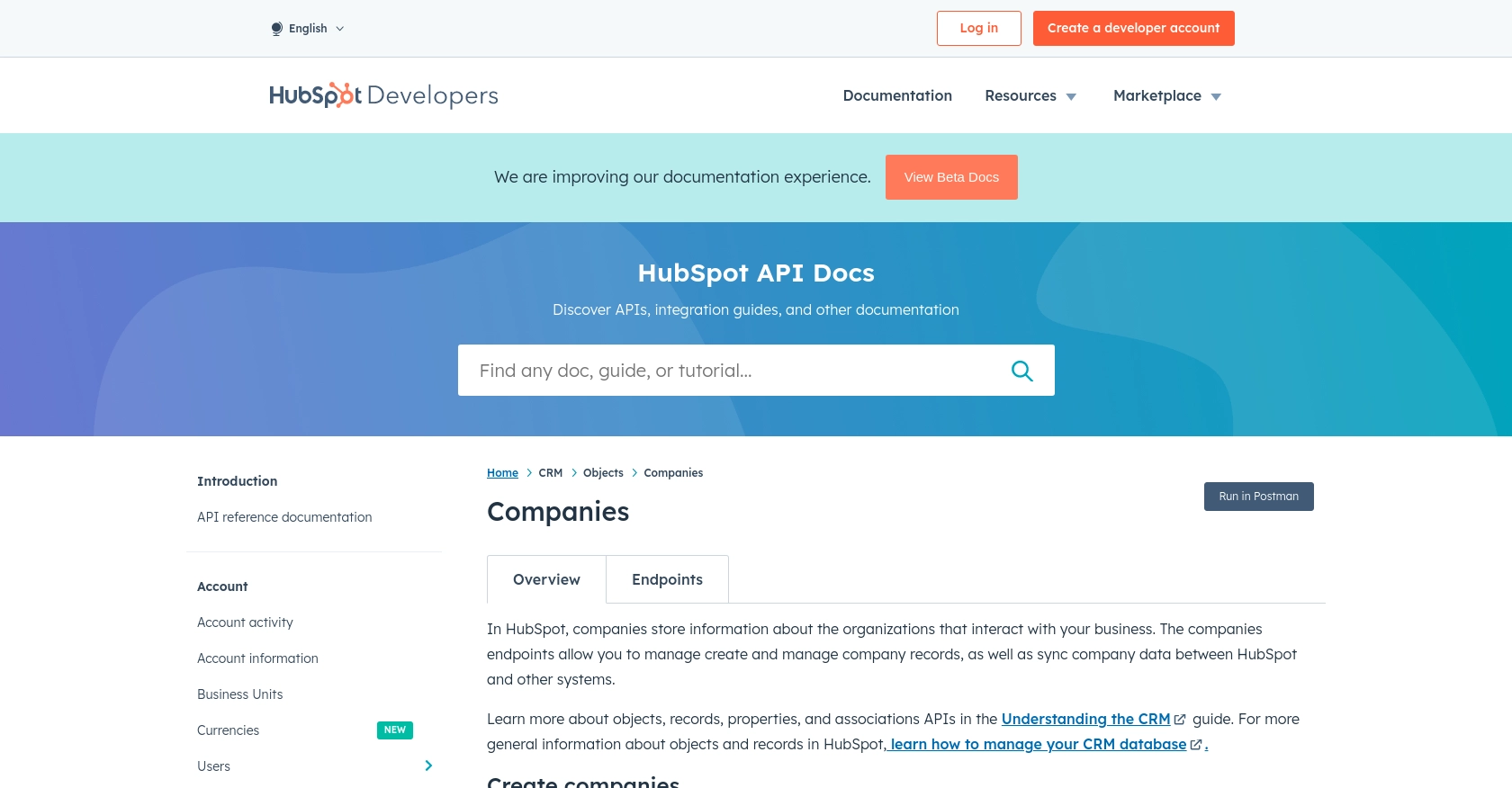
Best Practices for Using HubSpot API with PHP
When integrating with the HubSpot API using PHP, it's essential to follow best practices to ensure a secure and efficient implementation. Here are some key recommendations:
- Securely Store Credentials: Always store your OAuth tokens securely. Avoid hardcoding them in your scripts. Use environment variables or secure vaults to manage sensitive information.
- Implement Rate Limiting: HubSpot's API has rate limits, such as 100 requests every 10 seconds for OAuth apps. Implement logic to handle 429 errors and retry requests after a delay. For more details, refer to the HubSpot API usage guidelines.
- Data Transformation and Standardization: Ensure that the data retrieved from HubSpot is transformed and standardized to fit your application's requirements. This might include mapping HubSpot fields to your internal data structures.
Leverage Endgrate for Streamlined HubSpot Integrations
If you're looking to simplify your integration process with HubSpot and other platforms, consider using Endgrate. Endgrate provides a unified API endpoint that connects to multiple services, allowing you to build integrations once and deploy them across various platforms. This approach saves time and resources, enabling you to focus on your core product development.
With Endgrate, you can offer an intuitive integration experience for your customers, enhancing their interaction with your product. Visit Endgrate to learn more about how it can help streamline your integration efforts.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/companies
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?