How to Create Or Update Contacts with the Pipeliner CRM API in Javascript
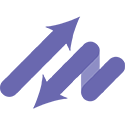
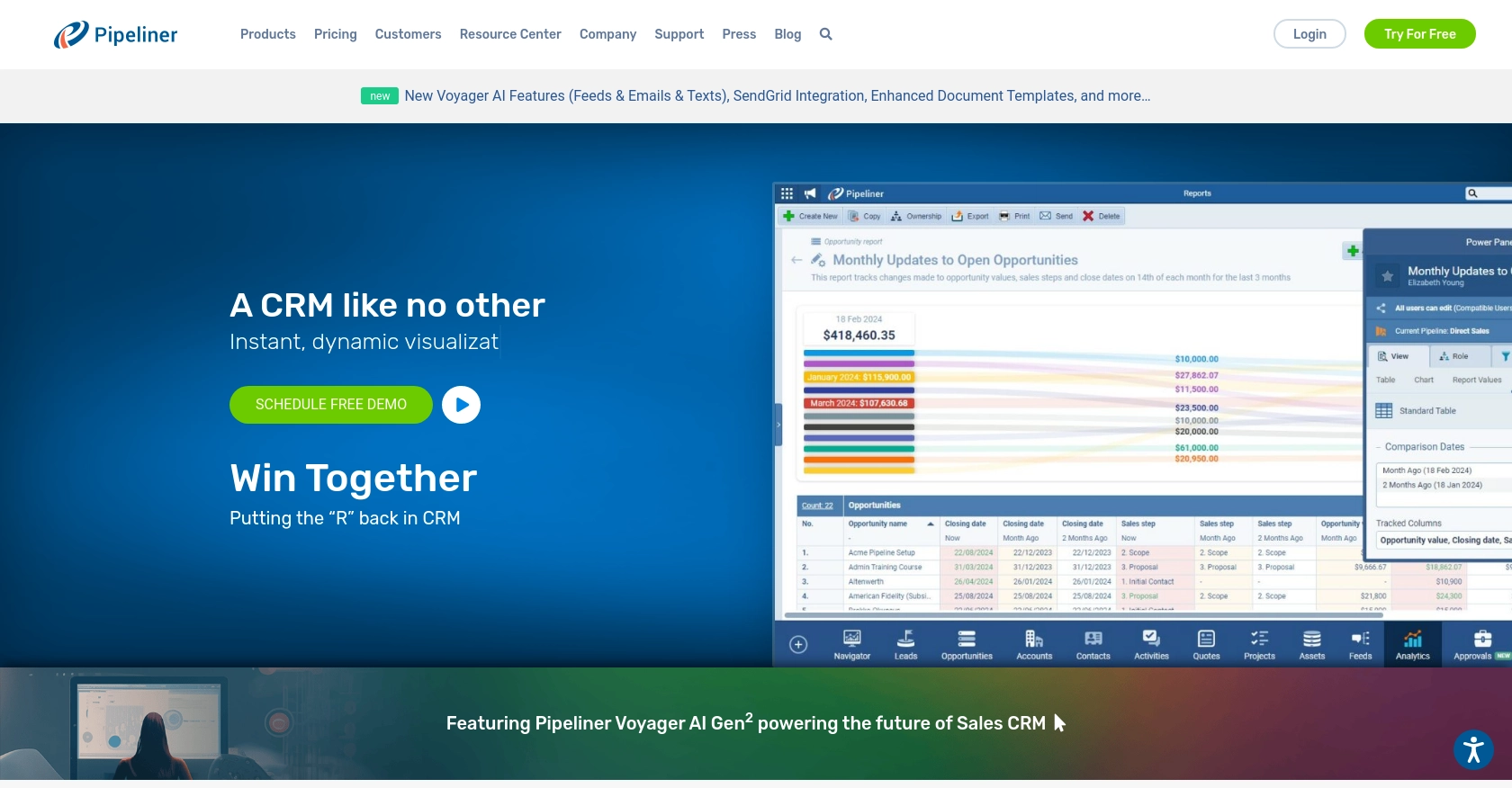
Introduction to Pipeliner CRM
Pipeliner CRM is a robust customer relationship management platform designed to enhance sales processes and improve business efficiency. With its intuitive interface and powerful features, Pipeliner CRM helps businesses manage contacts, track opportunities, and streamline workflows.
Developers may want to integrate with Pipeliner CRM's API to automate contact management tasks, such as creating or updating contact information. For example, a developer could use the Pipeliner CRM API to automatically update contact details from an external data source, ensuring that the CRM always has the most current information.
Setting Up Your Pipeliner CRM Test Account
Before you can start integrating with the Pipeliner CRM API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Pipeliner CRM Account
If you don't already have a Pipeliner CRM account, follow these steps to create one:
- Visit the Pipeliner CRM website and sign up for an account.
- Follow the on-screen instructions to complete the registration process.
- Once your account is created, log in to access your Pipeliner CRM space.
Generating Pipeliner CRM API Keys for Authentication
Pipeliner CRM uses Basic Authentication, which requires a username and password generated specifically for API access. Follow these steps to obtain your API keys:
- Log in to your Pipeliner CRM space.
- Navigate to the Administration section.
- Under the Unit, Users & Roles tab, select Applications.
- Create a new application to generate API access credentials.
- Click on Show API Access to view your API username and password. These credentials are generated only once, so store them securely.
These credentials will be used to authenticate your API requests.
Understanding Pipeliner CRM API Request Structure
Each API request to Pipeliner CRM requires a Space ID and Service URL. These are part of every URL request and are essential for accessing the correct data within your CRM space.
With your test account and API keys set up, you're ready to start making API calls to create or update contacts in Pipeliner CRM using JavaScript.
For more detailed information, refer to the Pipeliner CRM Authentication Documentation.
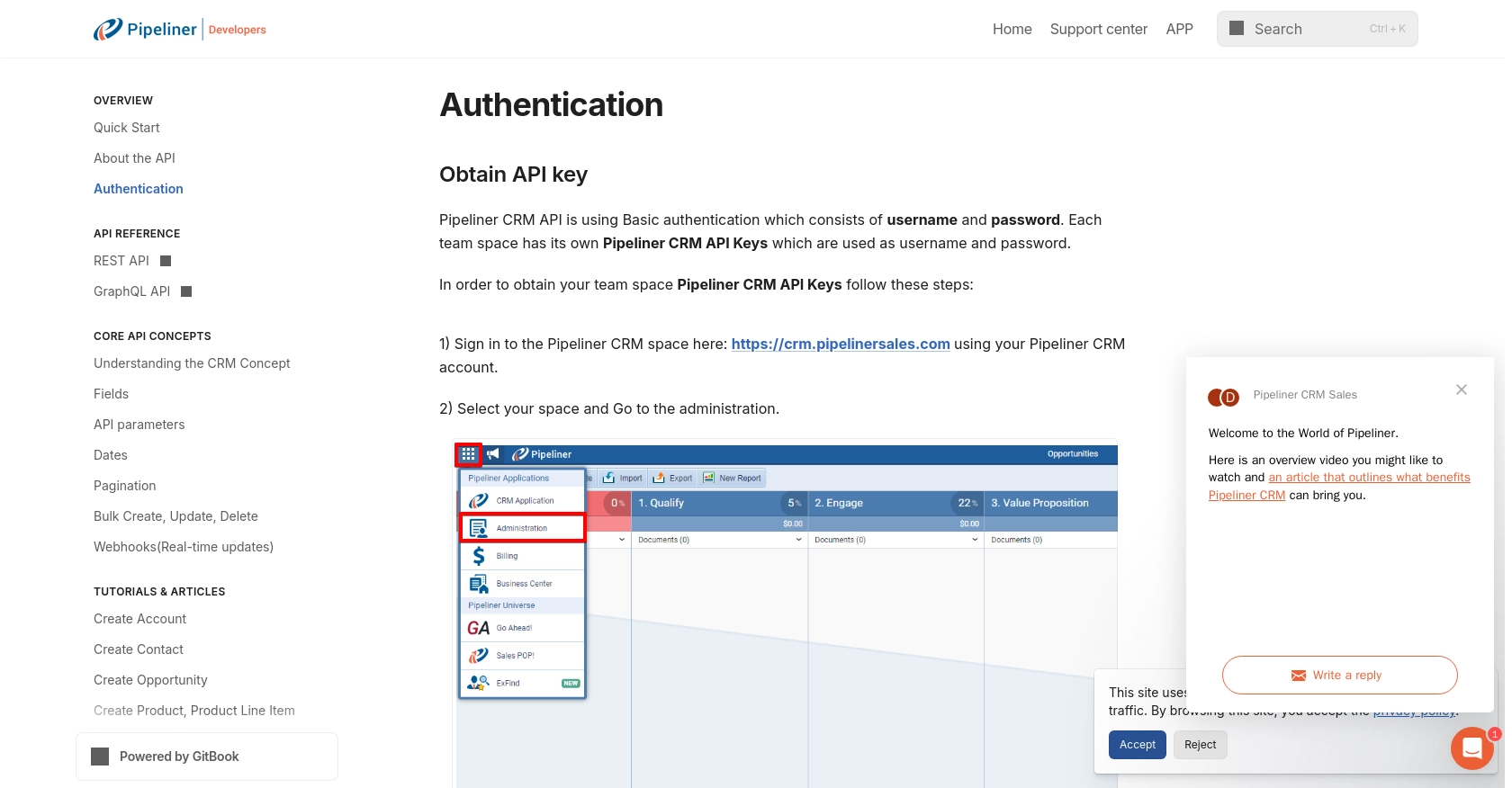
sbb-itb-96038d7
Making API Calls to Create or Update Contacts with Pipeliner CRM in JavaScript
To interact with the Pipeliner CRM API using JavaScript, you'll need to set up your environment and write code to make HTTP requests. This section will guide you through the process of creating or updating contacts using the Pipeliner CRM API.
Setting Up Your JavaScript Environment for Pipeliner CRM API Integration
Before making API calls, ensure you have Node.js installed on your machine. You can download it from the official Node.js website. Additionally, you'll need the axios
library to handle HTTP requests. Install it using the following command:
npm install axios
Creating a Contact with Pipeliner CRM API
To create a contact, you'll need to make a POST request to the Pipeliner CRM API. Here's a sample code snippet to create a contact:
const axios = require('axios');
const createContact = async () => {
const url = 'https://your-space-id.pipelinersales.com/api/v1/contacts';
const auth = {
username: 'your_api_username',
password: 'your_api_password'
};
const contactData = {
firstName: 'John',
lastName: 'Doe',
email: 'john.doe@example.com'
};
try {
const response = await axios.post(url, contactData, { auth });
console.log('Contact Created:', response.data);
} catch (error) {
console.error('Error Creating Contact:', error.response.data);
}
};
createContact();
Replace your-space-id
, your_api_username
, and your_api_password
with your actual Pipeliner CRM credentials. This code sends a POST request to create a new contact with the specified details.
Updating a Contact with Pipeliner CRM API
To update an existing contact, you'll need to make a PUT request. Here's how you can update a contact:
const updateContact = async (contactId) => {
const url = `https://your-space-id.pipelinersales.com/api/v1/contacts/${contactId}`;
const auth = {
username: 'your_api_username',
password: 'your_api_password'
};
const updatedData = {
email: 'new.email@example.com'
};
try {
const response = await axios.put(url, updatedData, { auth });
console.log('Contact Updated:', response.data);
} catch (error) {
console.error('Error Updating Contact:', error.response.data);
}
};
updateContact('contact-id');
Replace contact-id
with the ID of the contact you wish to update. This code sends a PUT request to update the contact's email address.
Handling API Responses and Errors
After making an API call, it's crucial to handle the response and any potential errors. The axios
library provides a straightforward way to manage these:
- Check the response status to verify if the request was successful.
- Log any errors to understand what went wrong and how to address it.
For more detailed information on error codes and handling, refer to the Pipeliner CRM API Documentation.
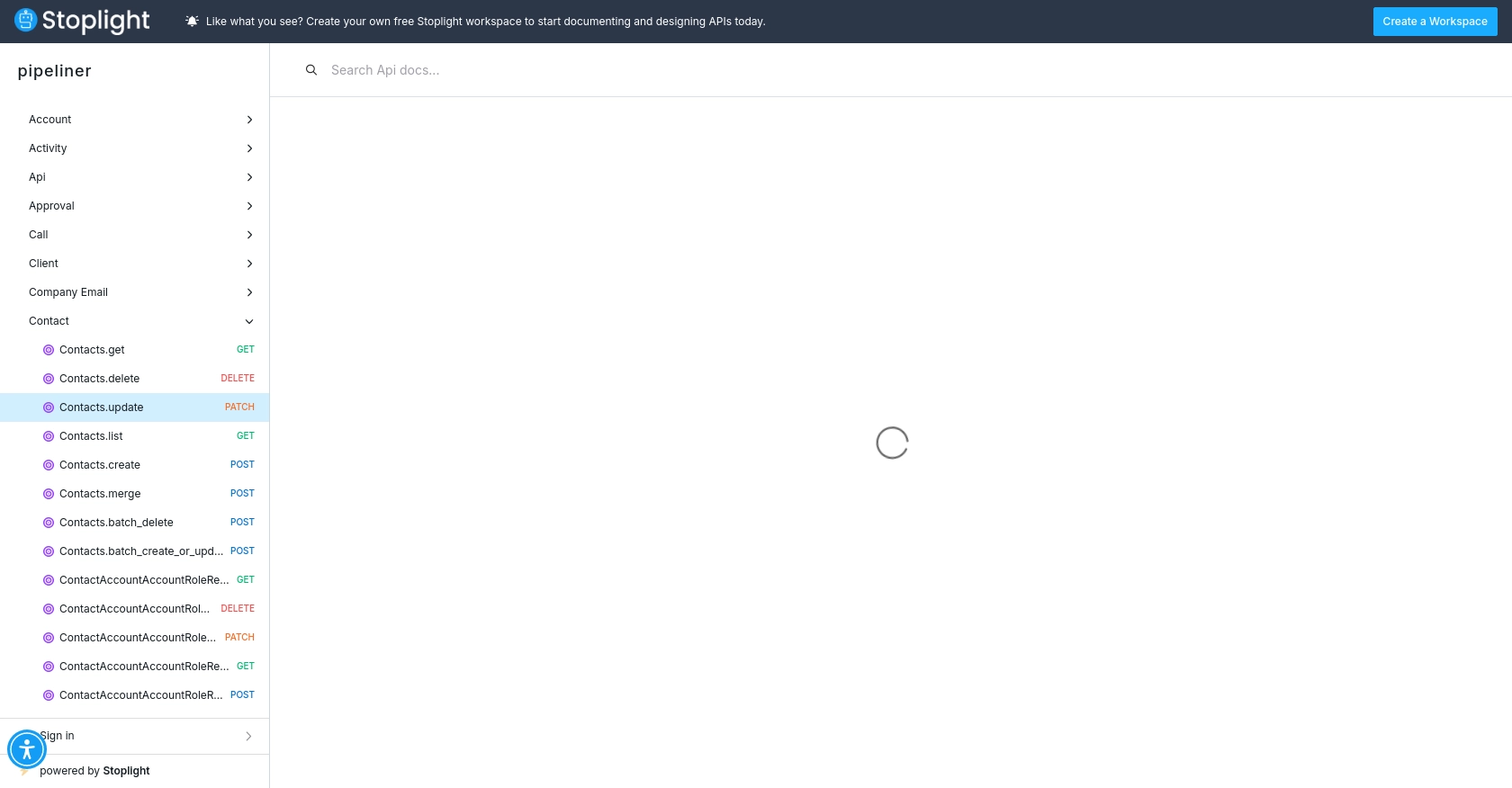
Best Practices for Using Pipeliner CRM API in JavaScript
When integrating with the Pipeliner CRM API, it's essential to follow best practices to ensure efficient and secure interactions. Here are some recommendations:
- Securely Store Credentials: Always store your API credentials securely. Avoid hardcoding them in your source code. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully. Refer to the Pipeliner CRM API Documentation for specific rate limit details.
- Implement Error Handling: Ensure robust error handling in your code to manage API response errors effectively. Log errors for debugging and provide meaningful messages to users when issues arise.
- Utilize Pagination: When retrieving large datasets, use pagination to manage data efficiently. Pipeliner CRM supports cursor-based pagination, allowing you to navigate through records seamlessly. Refer to the Pipeliner CRM Pagination Documentation for more information.
- Standardize Data Formats: Ensure that data formats are consistent when creating or updating contacts. This helps maintain data integrity and simplifies data processing.
Streamlining Integrations with Endgrate
Building and maintaining integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Pipeliner CRM. With Endgrate, you can:
- Save Time and Resources: Focus on your core product development while Endgrate handles the integration complexities.
- Build Once, Use Multiple Times: Develop a single integration for each use case and apply it across various platforms effortlessly.
- Enhance User Experience: Offer your customers a seamless and intuitive integration experience with minimal effort.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/pipelinercrm
- https://developers.pipelinersales.com/api-docs/overview/authentication
- https://developers.pipelinersales.com/api-docs/core-api-concepts/pagination
- https://pipeliner.stoplight.io/docs/api-docs/ah9798x4qjh7r-contacts-update
- https://pipeliner.stoplight.io/docs/api-docs/hds5thtgx28ti-contacts-create
Ready to get started?