How to Get Weekly Page Views with the Google Analytics API in PHP
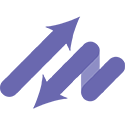
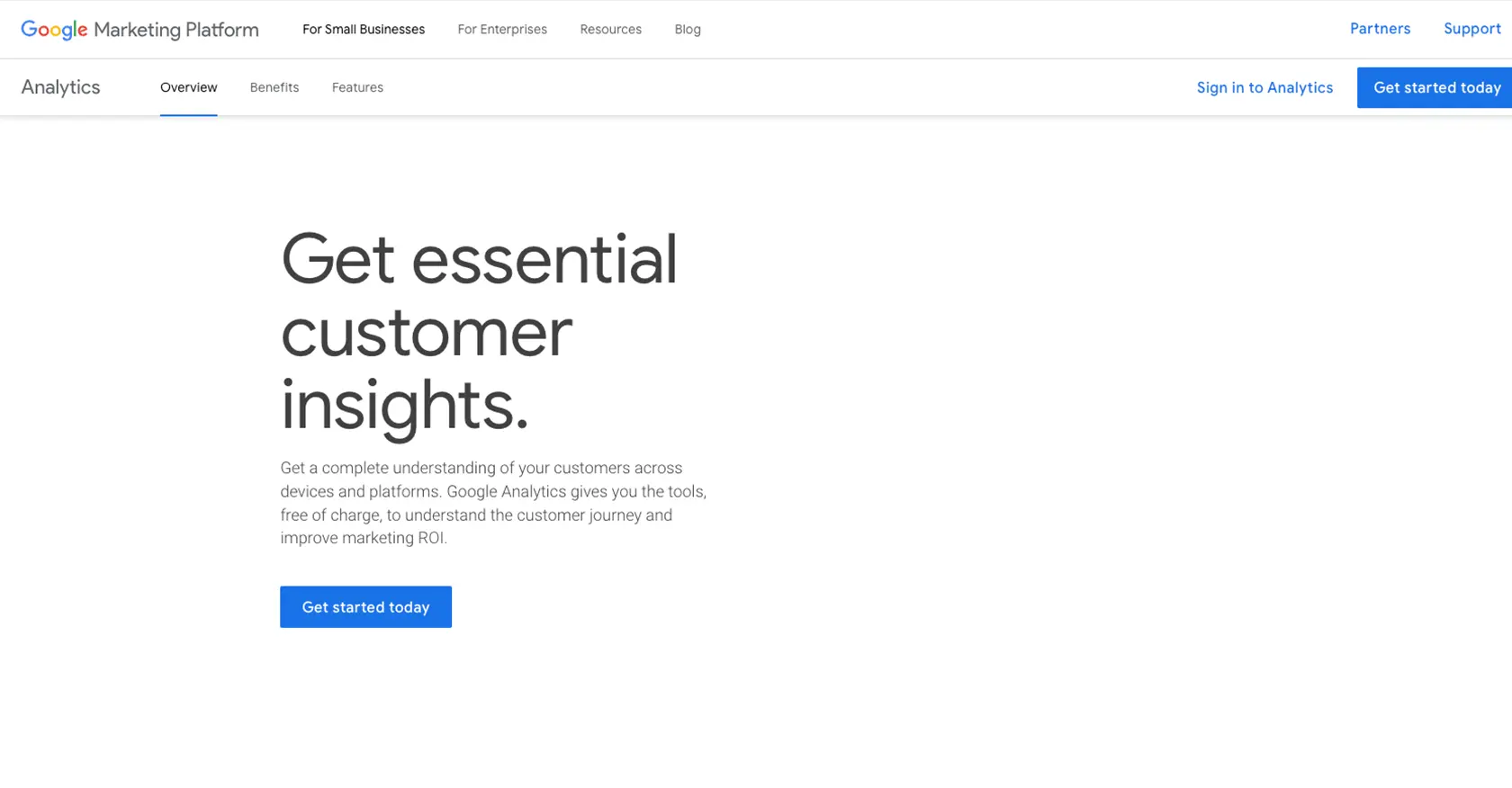
Introduction to Google Analytics API
Google Analytics is a powerful tool that provides insights into website traffic and user behavior. It offers a comprehensive suite of features that help businesses track and analyze their digital presence, making data-driven decisions to enhance user engagement and optimize marketing strategies.
For developers, integrating with the Google Analytics API allows for automated data retrieval and custom reporting. This can be particularly useful for generating weekly page view reports, enabling businesses to monitor website performance over time. For example, a developer might use the Google Analytics API to fetch weekly page views and display them on a custom dashboard, providing stakeholders with real-time insights into website traffic trends.
Setting Up Your Google Analytics API Test Account
Before you can start using the Google Analytics API to retrieve weekly page views, you'll need to set up a Google Cloud project and configure OAuth 2.0 authentication. This process involves creating a test account, enabling the necessary APIs, and obtaining the credentials required for API access.
Create a Google Cloud Project for Google Analytics API
- Go to the Google Cloud Console.
- Click on the Menu icon, navigate to IAM & Admin, and select Create a Project.
- Enter a descriptive name for your project and click Create.
Enable Google Analytics API
- In the Google Cloud Console, navigate to APIs & Services and select Library.
- Search for Google Analytics API and click on it.
- Click Enable to activate the API for your project.
Configure OAuth Consent Screen
- In the Google Cloud Console, go to APIs & Services and select OAuth consent screen.
- Select the user type for your app and click Create.
- Fill out the required fields, such as application name and support email, then click Save and Continue.
Create OAuth 2.0 Credentials
- Navigate to APIs & Services and select Credentials.
- Click Create Credentials and choose OAuth client ID.
- Select Web application as the application type.
- Enter a name for your OAuth client and configure the authorized redirect URIs.
- Click Create to generate your client ID and client secret. Save these credentials securely.
With your Google Cloud project set up and the necessary credentials in hand, you're ready to start making API calls to Google Analytics. Ensure you keep your client ID and secret secure, as they are essential for authenticating your requests.
For more detailed steps, refer to the official Google documentation: Create a Google Cloud Project, Enable APIs, Configure OAuth Consent, and Create Credentials.
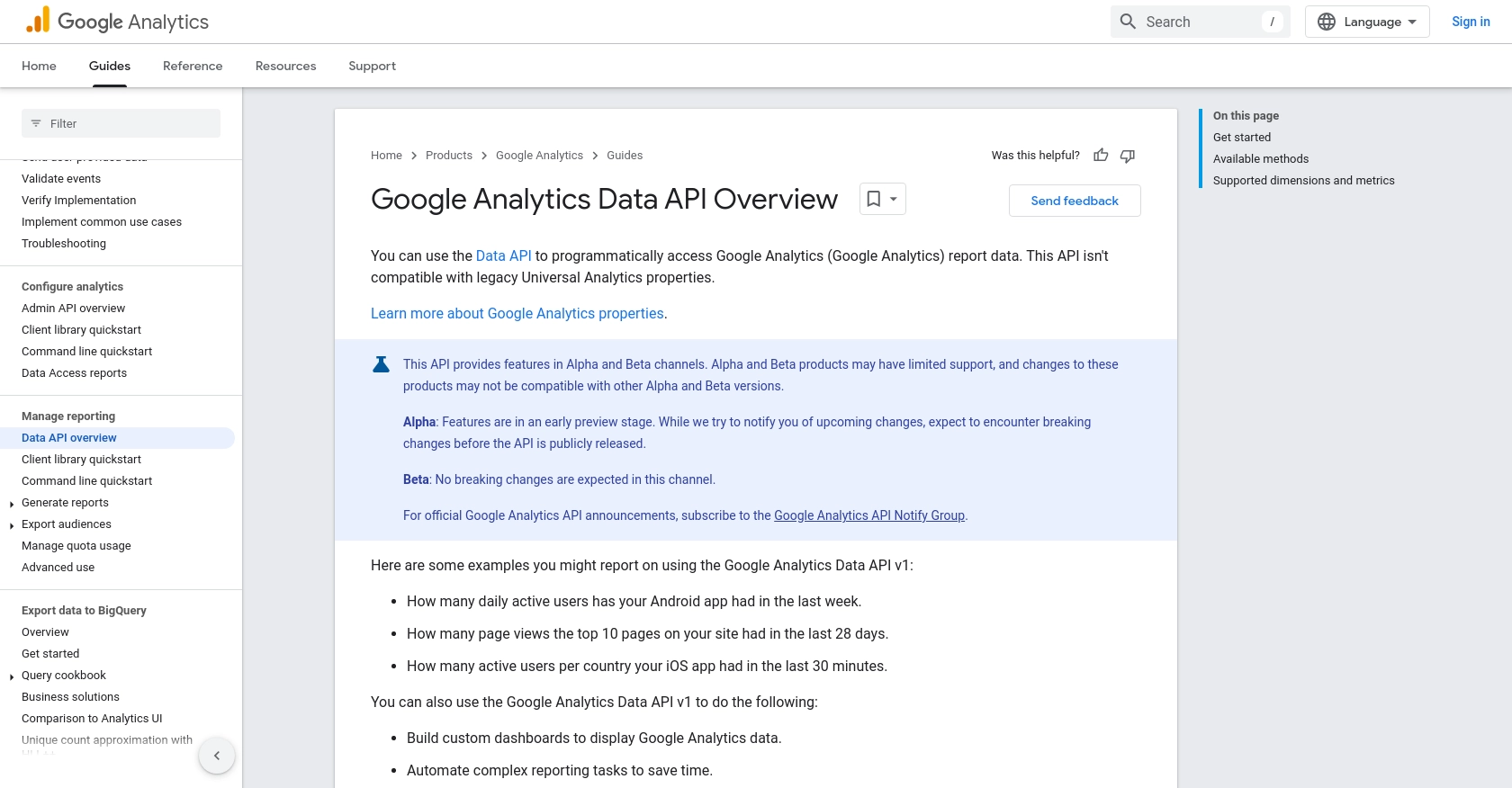
sbb-itb-96038d7
Making API Calls to Retrieve Weekly Page Views with Google Analytics API in PHP
Now that you have set up your Google Cloud project and obtained the necessary credentials, it's time to make API calls to the Google Analytics API using PHP. This section will guide you through the process of retrieving weekly page views, ensuring you have the right tools and code to get started.
Prerequisites for Using Google Analytics API with PHP
Before diving into the code, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer, the PHP package manager
To install the Google Analytics Data API client library, run the following command in your terminal:
composer require google/analytics-data
Setting Up the PHP Script to Fetch Weekly Page Views
Create a new PHP file named get_weekly_page_views.php
and add the following code to it:
use Google\Analytics\Data\V1beta\Client\BetaAnalyticsDataClient;
use Google\Analytics\Data\V1beta\DateRange;
use Google\Analytics\Data\V1beta\Dimension;
use Google\Analytics\Data\V1beta\Metric;
use Google\Analytics\Data\V1beta\RunReportRequest;
use Google\Analytics\Data\V1beta\RunReportResponse;
function getWeeklyPageViews(string $propertyId) {
$client = new BetaAnalyticsDataClient();
$request = (new RunReportRequest())
->setProperty('properties/' . $propertyId)
->setDateRanges([
new DateRange([
'start_date' => '7daysAgo',
'end_date' => 'yesterday',
]),
])
->setDimensions([
new Dimension(['name' => 'date']),
])
->setMetrics([
new Metric(['name' => 'pageviews']),
]);
$response = $client->runReport($request);
printReportResponse($response);
}
function printReportResponse(RunReportResponse $response) {
printf('%s rows received%s', $response->getRowCount(), PHP_EOL);
foreach ($response->getDimensionHeaders() as $dimensionHeader) {
printf('Dimension header name: %s%s', $dimensionHeader->getName(), PHP_EOL);
}
foreach ($response->getMetricHeaders() as $metricHeader) {
printf('Metric header name: %s (%s)%s', $metricHeader->getName(), $metricHeader->getType(), PHP_EOL);
}
print 'Report result: ' . PHP_EOL;
foreach ($response->getRows() as $row) {
printf('%s %s%s', $row->getDimensionValues()[0]->getValue(), $row->getMetricValues()[0]->getValue(), PHP_EOL);
}
}
// Replace 'YOUR-GA4-PROPERTY-ID' with your actual Google Analytics 4 property ID
getWeeklyPageViews('YOUR-GA4-PROPERTY-ID');
Running the PHP Script and Verifying the Output
To execute the script, run the following command in your terminal:
php get_weekly_page_views.php
Upon successful execution, the script will output the weekly page views for your specified Google Analytics property. Verify the results by checking the data against your Google Analytics dashboard.
Handling Errors and Troubleshooting
If you encounter any errors, ensure that your credentials are correctly configured and that your Google Analytics property ID is valid. Common error codes and their solutions can be found in the Google Analytics API documentation.
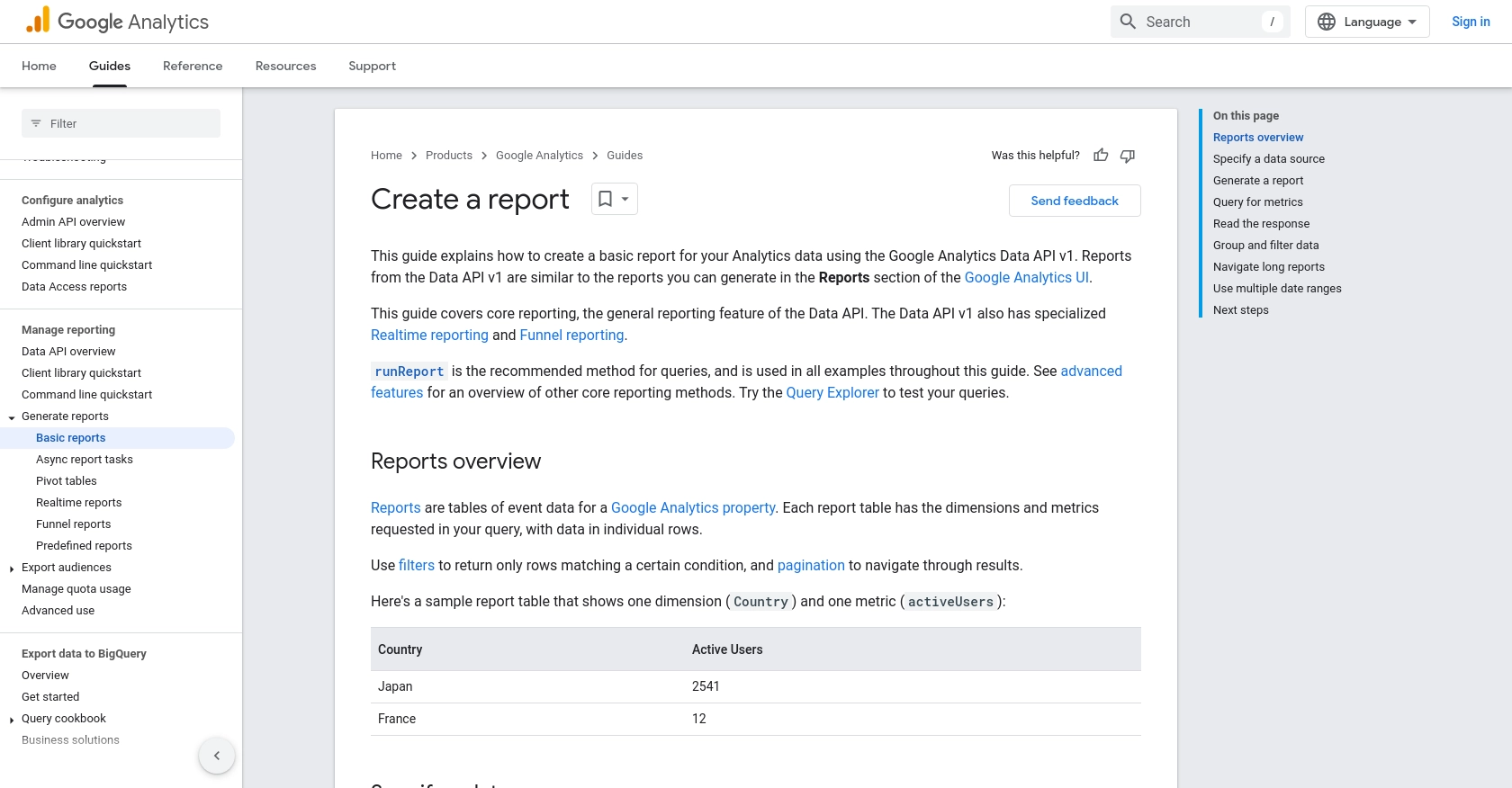
Conclusion and Best Practices for Using Google Analytics API in PHP
Integrating with the Google Analytics API using PHP allows developers to automate data retrieval and create custom reports, providing valuable insights into website performance. By following the steps outlined in this guide, you can efficiently fetch weekly page views and present them in a meaningful way to stakeholders.
Best Practices for Secure and Efficient API Integration
- Secure Credentials: Always store your OAuth client ID and secret securely. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be aware of the API's rate limits and implement retry logic or backoff strategies to handle rate limit errors gracefully. For more details, refer to the Google Analytics API documentation.
- Data Transformation: Standardize and transform data fields as needed to ensure consistency across different reports and dashboards.
- Error Handling: Implement robust error handling to manage API errors and exceptions, ensuring your application can recover gracefully from unexpected issues.
Leverage Endgrate for Seamless Integration
While integrating with Google Analytics API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API endpoint that simplifies integration across various platforms, including Google Analytics. By using Endgrate, you can streamline your integration process, focus on your core product, and provide an intuitive experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/googleanalytics
- https://developers.google.com/analytics/devguides/reporting/data/v1
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/analytics/devguides/reporting/data/v1/basics
Ready to get started?