Using the Salesforce Sandbox API to Create or Update Records in PHP
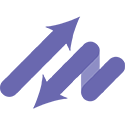
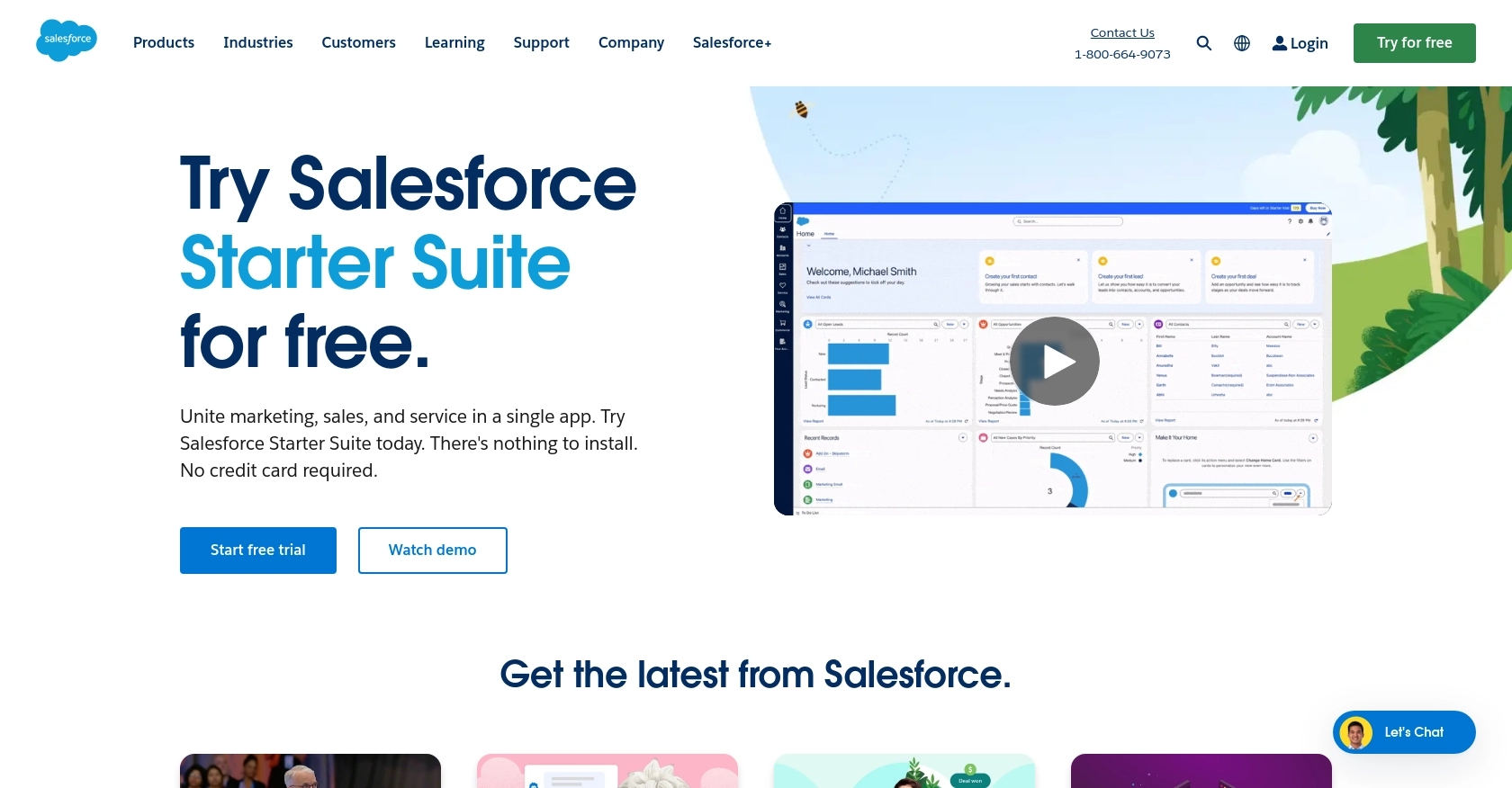
Introduction to Salesforce Sandbox API
Salesforce Sandbox is a powerful tool that allows developers to create and test applications in a safe environment that mirrors their production Salesforce instance. This enables developers to experiment with new features, integrations, and customizations without affecting live data or operations.
Integrating with the Salesforce Sandbox API is essential for developers looking to streamline their development process. By using the API, developers can automate tasks such as creating or updating records, which can significantly enhance productivity and ensure consistency across applications. For example, a developer might use the Salesforce Sandbox API to automatically update customer records with new information from an external CRM system, ensuring that all data remains synchronized and up-to-date.
Setting Up Your Salesforce Sandbox Account
Before you can start using the Salesforce Sandbox API, you'll need to set up a Salesforce Developer Edition account. This will provide you with a sandbox environment to test and develop your applications without impacting your live data.
Step-by-Step Guide to Creating a Salesforce Developer Edition Account
- Visit the Salesforce Developer Edition sign-up page.
- Fill out the registration form with your details, including your name, email, company, and country.
- Agree to the terms of service and click the "Sign Me Up" button.
- Check your email for a confirmation message from Salesforce. Click the link in the email to verify your account.
- Log in to your new Salesforce Developer Edition account.
Creating a Connected App for OAuth Authentication
To interact with the Salesforce Sandbox API, you'll need to create a connected app to handle OAuth authentication. This will allow you to securely access Salesforce data.
- In your Salesforce Developer Edition account, navigate to Setup.
- In the Quick Find box, type App Manager and select it.
- Click the New Connected App button.
- Fill in the required fields, such as Connected App Name, API Name, and Contact Email.
- Under API (Enable OAuth Settings), check the Enable OAuth Settings box.
- Enter a Callback URL. This can be a placeholder URL for testing purposes.
- Select the appropriate OAuth Scopes that your application will need access to, such as Full Access or Access and manage your data (api).
- Click Save to create the connected app.
Obtaining Your Client ID and Client Secret
After creating the connected app, you'll receive a Consumer Key (Client ID) and Consumer Secret (Client Secret). These credentials are essential for authenticating your API requests.
- In the App Manager, find your newly created connected app and click the dropdown arrow next to it.
- Select View to see the app details.
- Copy the Consumer Key and Consumer Secret and store them securely. You'll need these for OAuth authentication in your PHP application.
With your Salesforce Developer Edition account and connected app set up, you're ready to start interacting with the Salesforce Sandbox API using PHP.
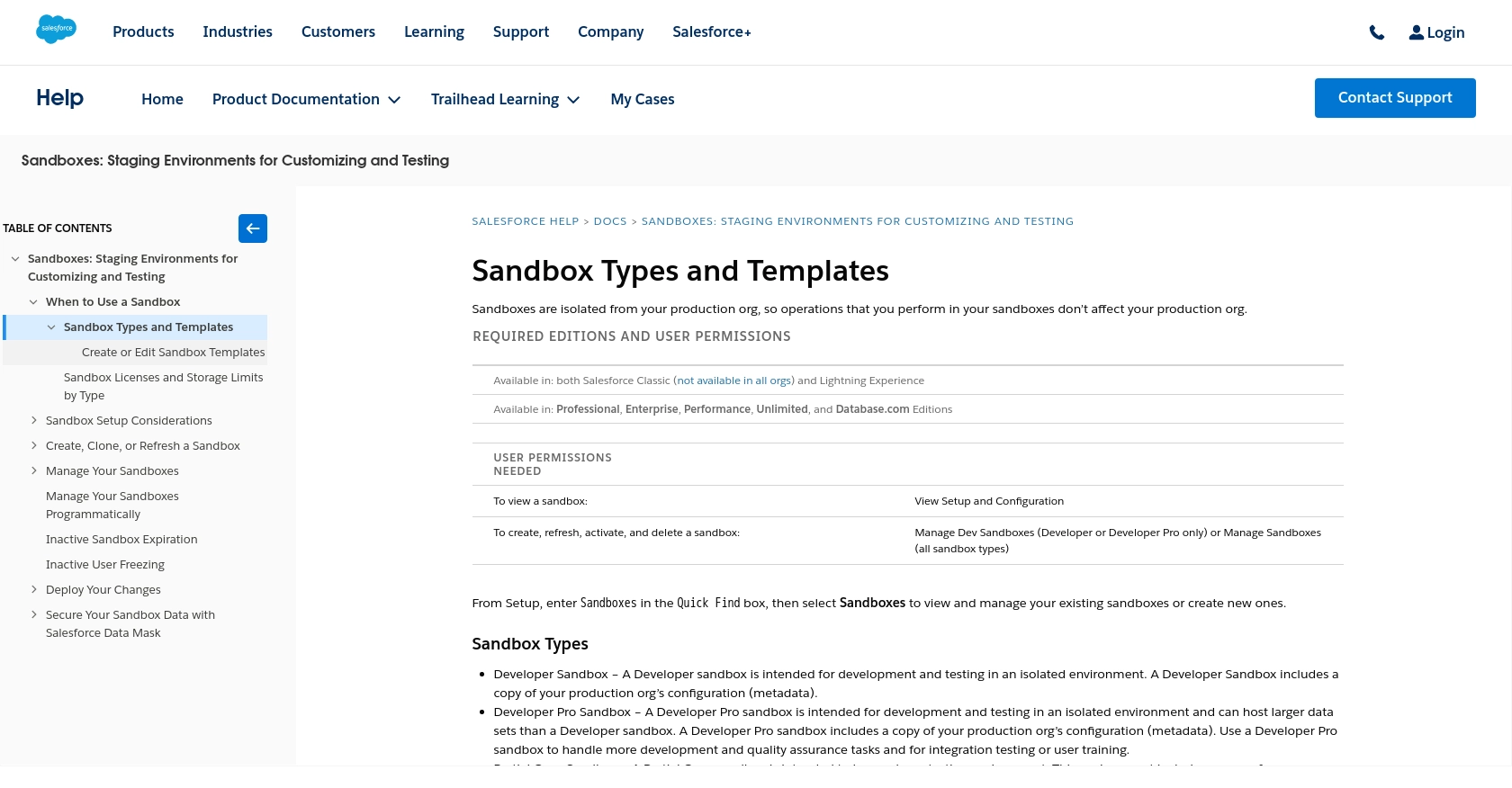
sbb-itb-96038d7
Making API Calls to Salesforce Sandbox Using PHP
With your Salesforce Developer Edition account and connected app ready, you can now proceed to make API calls to the Salesforce Sandbox using PHP. This section will guide you through the process of setting up your PHP environment, installing necessary dependencies, and executing API requests to create or update records.
Setting Up Your PHP Environment
Before making API calls, ensure that you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer, the PHP package manager
Once you have these installed, open your terminal and create a new project directory:
mkdir salesforce-sandbox-api
cd salesforce-sandbox-api
Installing Required PHP Libraries
To interact with the Salesforce API, you'll need the Guzzle HTTP client. Install it using Composer:
composer require guzzlehttp/guzzle
Authenticating with Salesforce Sandbox API
To authenticate with the Salesforce Sandbox API, you'll need to obtain an access token using your Client ID and Client Secret. Here's how you can do it in PHP:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$response = $client->post('https://login.salesforce.com/services/oauth2/token', [
'form_params' => [
'grant_type' => 'password',
'client_id' => 'Your_Client_ID',
'client_secret' => 'Your_Client_Secret',
'username' => 'Your_Salesforce_Username',
'password' => 'Your_Salesforce_Password'
]
]);
$tokenData = json_decode($response->getBody(), true);
$accessToken = $tokenData['access_token'];
Replace Your_Client_ID
, Your_Client_Secret
, Your_Salesforce_Username
, and Your_Salesforce_Password
with your actual credentials.
Creating or Updating Records in Salesforce Sandbox
Once authenticated, you can create or update records in Salesforce. Here's an example of how to create a new account record:
$response = $client->post('https://your_instance.salesforce.com/services/data/vXX.X/sobjects/Account/', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json'
],
'json' => [
'Name' => 'New Account Name'
]
]);
if ($response->getStatusCode() == 201) {
echo "Account created successfully!";
} else {
echo "Failed to create account.";
}
Replace your_instance
with your Salesforce instance URL and vXX.X
with the API version you are using.
Handling API Responses and Errors
It's crucial to handle API responses and errors effectively. Salesforce API responses include status codes that indicate the success or failure of a request. Here are some common status codes:
- 200: OK - The request was successful.
- 201: Created - The resource was successfully created.
- 400: Bad Request - The request was malformed.
- 401: Unauthorized - Authentication failed.
- 403: Forbidden - The request is not allowed.
- 404: Not Found - The resource could not be found.
Always check the response status code and handle errors appropriately in your application.
By following these steps, you can efficiently interact with the Salesforce Sandbox API using PHP, enabling you to automate and streamline your development processes.
Best Practices for Using Salesforce Sandbox API in PHP
When working with the Salesforce Sandbox API, it's essential to follow best practices to ensure security, efficiency, and maintainability. Here are some key recommendations:
- Securely Store Credentials: Always store your Client ID, Client Secret, and access tokens securely. Use environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limiting: Salesforce imposes rate limits on API requests. Monitor your API usage and implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that data fields are consistent and standardized across your application to avoid discrepancies and data integrity issues.
- Implement Error Handling: Robust error handling is crucial. Log errors and provide meaningful messages to help diagnose issues quickly.
Streamline Your Integrations with Endgrate
Building and maintaining integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Salesforce Sandbox. By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an easy, intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website today.
Read More
- https://endgrate.com/provider/salesforce-sandbox
- https://help.salesforce.com/s/articleView?id=sf.create_test_instance.htm&type=5
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/intro_oauth_and_connected_apps.htm
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/quickstart_dev_org.htm
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/resources_list.htm
Ready to get started?