Using the Copper API to Get Users (with PHP examples)
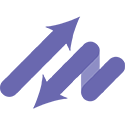
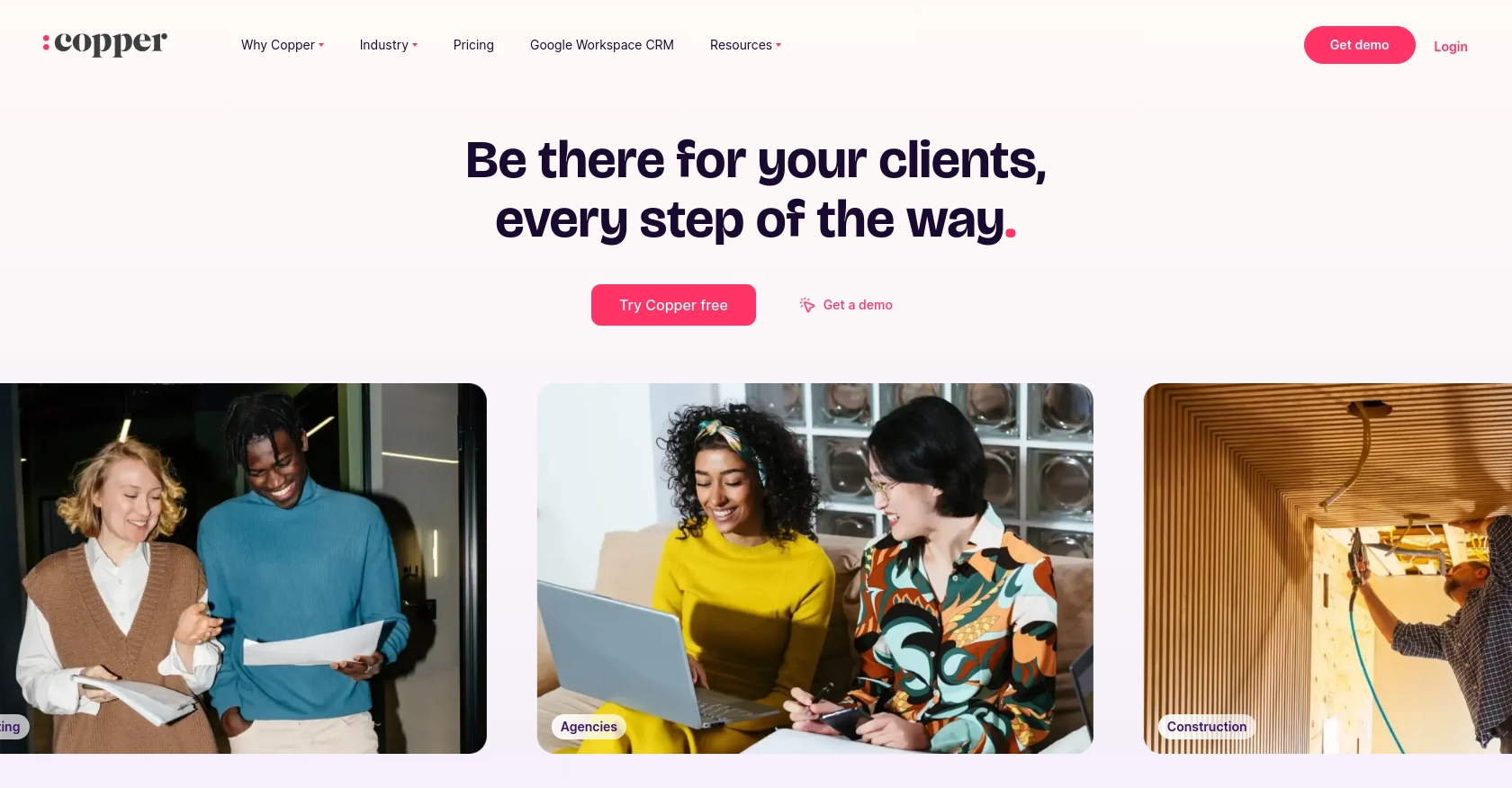
Introduction to Copper API
Copper is a robust platform designed to streamline cryptocurrency management and trading for businesses. It offers a comprehensive suite of tools that enable secure and efficient handling of digital assets, making it a preferred choice for organizations looking to optimize their crypto operations.
Integrating with Copper's API allows developers to automate various processes, such as retrieving user data, managing portfolios, and executing trades. For example, a developer might use the Copper API to fetch user information and integrate it into a custom dashboard, providing real-time insights and enhancing user experience.
Setting Up Your Copper API Test or Sandbox Account
Before you can start integrating with the Copper API, you'll need to set up a test or sandbox account. This will allow you to experiment with API calls without affecting live data. Follow these steps to get started:
Step 1: Sign Up for a Copper Account
If you don't already have a Copper account, visit the Copper website and sign up for a free trial or demo account. This will give you access to the platform's features and allow you to explore its capabilities.
Step 2: Access the Developer Portal
Once your account is set up, navigate to the Copper Developer Portal. Here, you can find documentation, API keys, and other resources necessary for integration.
Step 3: Generate API Keys
To authenticate your API requests, you'll need to generate API keys. Follow these steps:
- Log in to your Copper account.
- Go to the API section in the settings.
- Click on "Generate API Key" and save the key and secret securely.
These keys will be used to authenticate your API requests.
Step 4: Configure API Authentication
Copper uses a custom authentication method. You will need to include the following headers in your API requests:
$apiKey = 'your_api_key';
$secret = 'your_api_secret';
$timestamp = round(microtime(true) * 1000);
$method = 'GET'; // or POST, PATCH, etc.
$urlPath = '/platform/users';
$body = ''; // JSON body if applicable
$signature = hash_hmac('sha256', $timestamp . $method . $urlPath . $body, $secret);
$headers = [
'Authorization: ApiKey ' . $apiKey,
'X-Signature: ' . $signature,
'X-Timestamp: ' . $timestamp,
'Content-Type: application/json'
];
Ensure that you replace your_api_key
and your_api_secret
with the actual values from your Copper account.
Step 5: Test API Calls
With your API keys and authentication configured, you can start making test API calls. Use tools like Postman or cURL to verify that your setup is working correctly.
For example, to retrieve user data, you can use the following PHP code:
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, 'https://api.copper.co/platform/users');
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
This code snippet demonstrates how to make a GET request to the Copper API to retrieve user information.
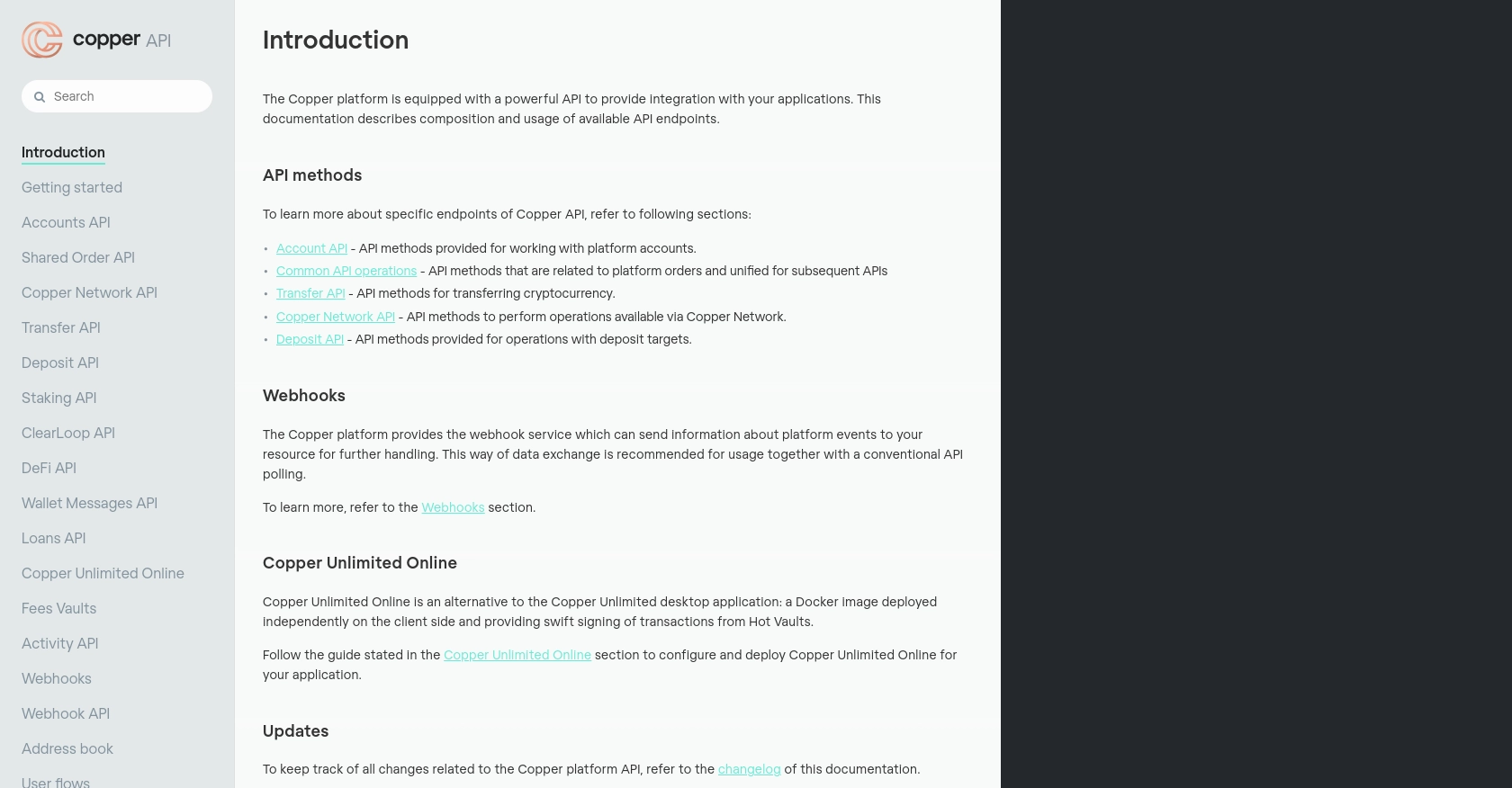
sbb-itb-96038d7
Making API Calls to Retrieve Copper Users with PHP
To effectively interact with the Copper API and retrieve user information, you'll need to ensure your PHP environment is properly set up. This section will guide you through the necessary steps, including setting up your PHP environment, installing dependencies, and executing API calls.
Setting Up Your PHP Environment for Copper API Integration
Before making API calls, ensure you have PHP installed on your system. It's recommended to use PHP version 7.4 or later for compatibility and security reasons. Additionally, you'll need the cURL extension enabled to handle HTTP requests.
- Verify your PHP installation by running
php -v
in your terminal. - Ensure cURL is enabled by checking your
php.ini
file or runningphp -m
to list installed modules.
Installing Necessary PHP Dependencies for Copper API
For seamless API interaction, you might need to install additional PHP libraries. Use Composer, a dependency manager for PHP, to manage these installations:
composer require guzzlehttp/guzzle
This command installs Guzzle, a popular HTTP client for PHP, which simplifies making API requests.
Executing Copper API Calls to Retrieve User Data
With your environment set up, you can now make API calls to Copper. Below is a PHP example demonstrating how to retrieve user data using the Copper API:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiKey = 'your_api_key';
$secret = 'your_api_secret';
$timestamp = round(microtime(true) * 1000);
$method = 'GET';
$urlPath = '/platform/users';
$body = '';
$signature = hash_hmac('sha256', $timestamp . $method . $urlPath . $body, $secret);
$response = $client->request('GET', 'https://api.copper.co' . $urlPath, [
'headers' => [
'Authorization' => 'ApiKey ' . $apiKey,
'X-Signature' => $signature,
'X-Timestamp' => $timestamp,
'Content-Type' => 'application/json'
]
]);
echo $response->getBody();
This script uses Guzzle to send a GET request to the Copper API, retrieving user data. Ensure you replace your_api_key
and your_api_secret
with your actual Copper API credentials.
Verifying API Call Success and Handling Errors
After executing the API call, it's crucial to verify the response to ensure the request was successful. Check the HTTP status code and handle any errors appropriately:
if ($response->getStatusCode() === 200) {
echo "User data retrieved successfully.";
} else {
echo "Failed to retrieve user data. Error: " . $response->getReasonPhrase();
}
This code snippet checks the response status and prints a success or error message accordingly.
Checking API Call Results in Copper Sandbox
To confirm the API call's success, log into your Copper sandbox account and verify the retrieved user data. This ensures that the API interaction is functioning as expected.
Conclusion and Best Practices for Copper API Integration
Integrating with the Copper API provides a powerful way to manage cryptocurrency operations efficiently. By following the steps outlined in this guide, developers can seamlessly retrieve user data and enhance their applications with real-time insights.
Best Practices for Secure Copper API Integration
- Secure API Keys: Always store your API keys and secrets securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Copper API has rate limits to prevent abuse. Implement logic to handle HTTP 429 errors and retry requests after a delay.
- Data Standardization: Ensure that data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage different HTTP status codes and provide meaningful feedback to users.
Enhance Your Integration with Endgrate
Consider leveraging Endgrate to streamline your integration processes. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers.
For more information, visit Endgrate and explore how it can enhance your integration capabilities.
Read More
Ready to get started?