Using the Sugar Market API to Get Tasks (with Javascript examples)
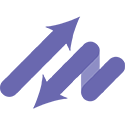
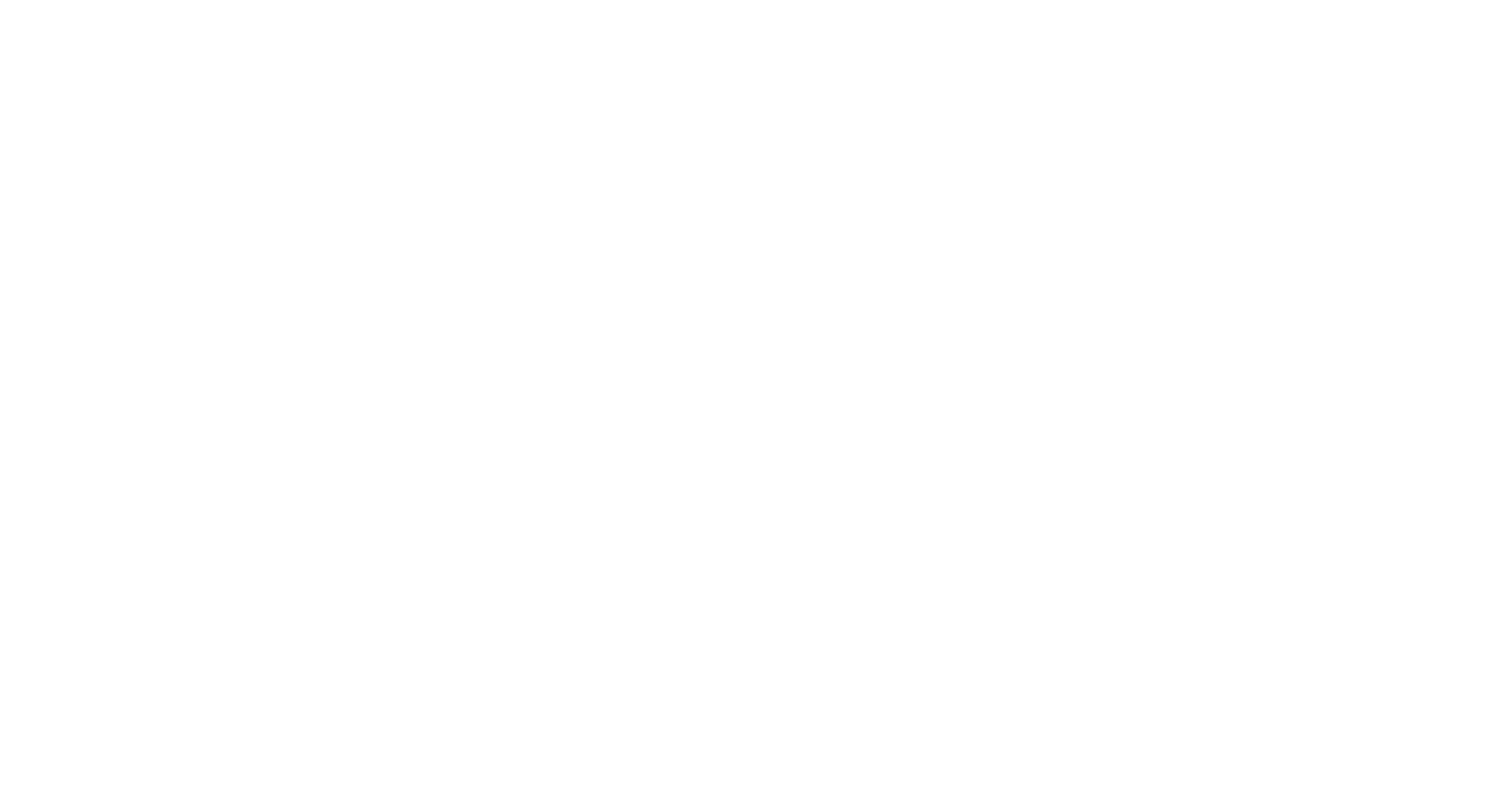
Introduction to Sugar Market API
Sugar Market is a robust marketing automation platform designed to enhance the marketing efforts of businesses by providing tools for campaign management, lead nurturing, and analytics. It offers a comprehensive suite of features that enable marketers to create, manage, and analyze marketing campaigns effectively.
Developers may want to integrate with the Sugar Market API to streamline marketing processes and access valuable data. For example, by retrieving tasks using the Sugar Market API, developers can automate task management, ensuring that marketing teams stay organized and efficient.
This article will guide you through using JavaScript to interact with the Sugar Market API, specifically focusing on retrieving tasks. By the end of this tutorial, you'll be equipped with the knowledge to efficiently access and manage tasks within the Sugar Market platform using JavaScript.
Setting Up a Sugar Market Test Account for API Access
Before you can start interacting with the Sugar Market API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Follow these steps to create a test account and obtain the necessary credentials for API access.
Creating a Sugar Market Test Account
If you don't already have a Sugar Market account, you can sign up for a free trial or demo account on the Sugar Market website. This will give you access to the platform's features and allow you to test API interactions.
- Visit the Sugar Market website and navigate to the sign-up page.
- Follow the instructions to create your account. You'll need to provide some basic information such as your name, email, and company details.
- Once your account is created, you'll receive an email with login details. Use these to access your Sugar Market dashboard.
Generating API Credentials for Sugar Market
To authenticate your API requests, you'll need to generate API credentials. Sugar Market uses a custom authentication method, so follow these steps to obtain your credentials:
- Log in to your Sugar Market account and navigate to the API settings section.
- Look for an option to create a new API key or token. This may be under a section labeled "API Access" or "Developer Tools."
- Generate a new API key or token. Make sure to copy and securely store this information, as you'll need it for authenticating your API requests.
Configuring OAuth for Sugar Market API
If the Sugar Market API requires OAuth-based authentication, you'll need to set up an application within your test account:
- Navigate to the OAuth settings in your Sugar Market dashboard.
- Create a new application by providing necessary details such as the application name and redirect URI.
- Once the application is created, you'll receive a client ID and client secret. Keep these credentials secure, as they are essential for OAuth authentication.
With your test account and API credentials set up, you're ready to start making API calls to Sugar Market. In the next section, we'll explore how to use JavaScript to retrieve tasks from the Sugar Market API.
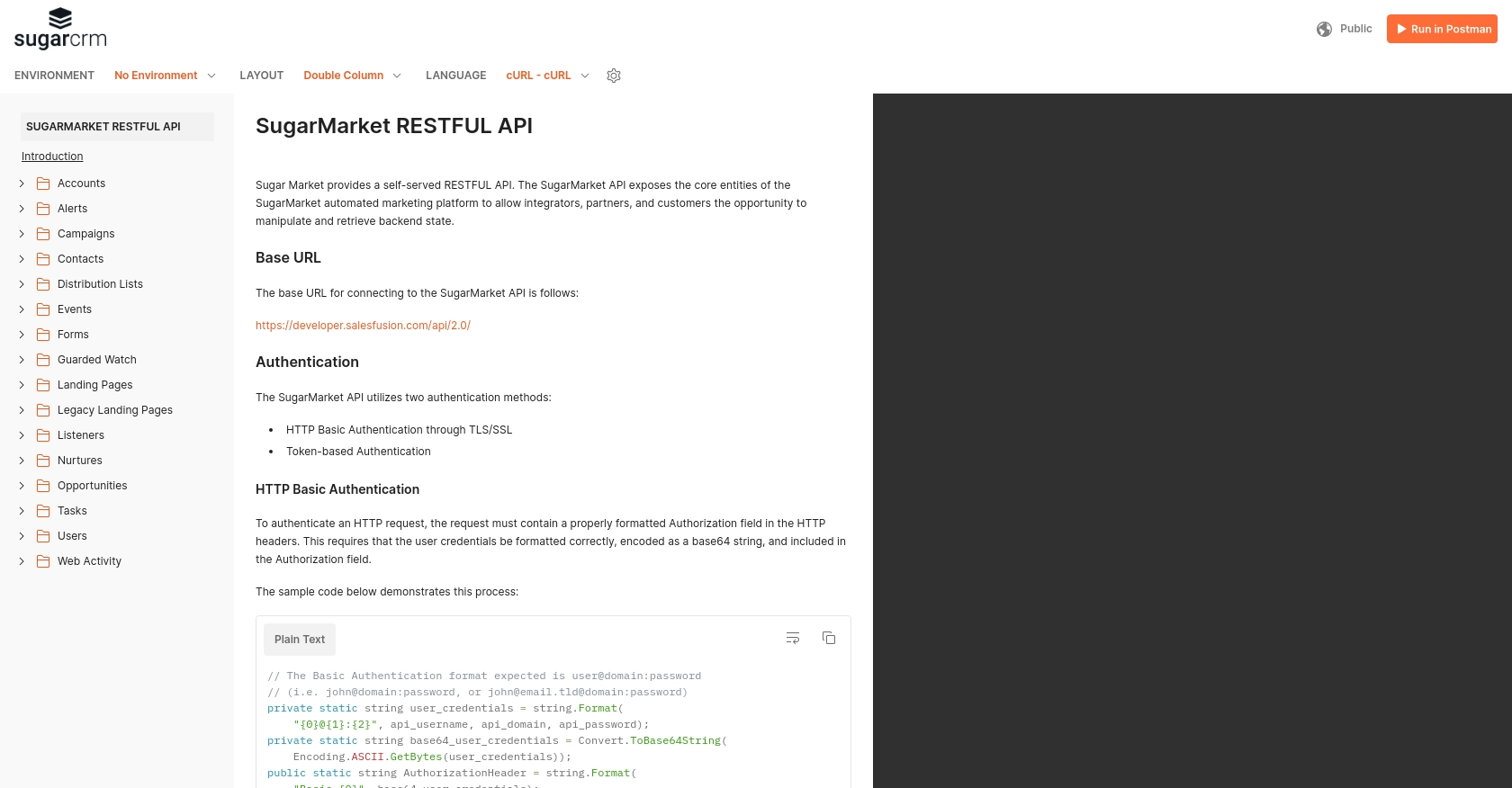
sbb-itb-96038d7
Making API Calls to Retrieve Tasks from Sugar Market Using JavaScript
To interact with the Sugar Market API and retrieve tasks, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the necessary code, and handling responses effectively.
Setting Up Your JavaScript Environment for Sugar Market API Integration
Before making API calls, ensure you have a suitable JavaScript environment. You can use Node.js or a browser-based environment for this purpose. Make sure you have the following:
- Node.js installed on your machine (if using a server-side environment).
- A code editor like Visual Studio Code for writing JavaScript code.
- The
axios
library for making HTTP requests. You can install it using npm:
npm install axios
Writing JavaScript Code to Retrieve Tasks from Sugar Market API
With your environment set up, you can now write the JavaScript code to interact with the Sugar Market API. The following example demonstrates how to retrieve tasks:
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://api.sugarmarket.com/v1/tasks';
const headers = {
'Authorization': 'Bearer YOUR_API_KEY',
'Content-Type': 'application/json'
};
// Function to get tasks from Sugar Market
async function getTasks() {
try {
const response = await axios.get(endpoint, { headers });
const tasks = response.data;
console.log('Retrieved Tasks:', tasks);
} catch (error) {
console.error('Error retrieving tasks:', error.response ? error.response.data : error.message);
}
}
// Call the function to retrieve tasks
getTasks();
Replace YOUR_API_KEY
with the API key you generated earlier. This code uses the axios
library to send a GET request to the Sugar Market API endpoint for tasks. It handles the response by logging the retrieved tasks to the console.
Verifying API Request Success and Handling Errors
After running the code, you should verify the success of your API request. Check the console output to see if the tasks were retrieved successfully. If there are any errors, the code will log them to the console, providing details about the issue.
Common error codes and their meanings can be found in the Sugar Market API documentation. Ensure you handle these errors gracefully in your application to improve user experience.
By following these steps, you can effectively retrieve tasks from the Sugar Market API using JavaScript. This integration allows you to automate task management and streamline marketing processes within your application.
Conclusion and Best Practices for Using Sugar Market API with JavaScript
Integrating with the Sugar Market API using JavaScript provides a powerful way to automate and enhance your marketing processes. By retrieving tasks programmatically, you can ensure that your marketing team remains organized and efficient, allowing them to focus on strategic initiatives rather than manual task management.
Best Practices for Secure and Efficient API Integration
- Securely Store API Credentials: Always store your API keys and OAuth credentials securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully. Check the Sugar Market API documentation for specific rate limit information.
- Error Handling: Implement robust error handling to manage API errors effectively. Log errors for debugging and provide user-friendly messages to improve the user experience.
- Data Transformation: Standardize and transform data fields as needed to ensure compatibility with your application's data model. This will help maintain data integrity and consistency.
Streamlining Integrations with Endgrate
If managing multiple integrations is becoming a challenge, consider leveraging Endgrate to simplify the process. Endgrate offers a unified API endpoint that connects to various platforms, including Sugar Market, allowing you to build once and deploy across multiple services. This approach saves time and resources, enabling you to focus on your core product development.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover how it can streamline your integration efforts.
Read More
Ready to get started?