Using the Sap Business One API to Create or Update Items in Python
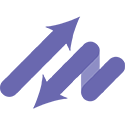
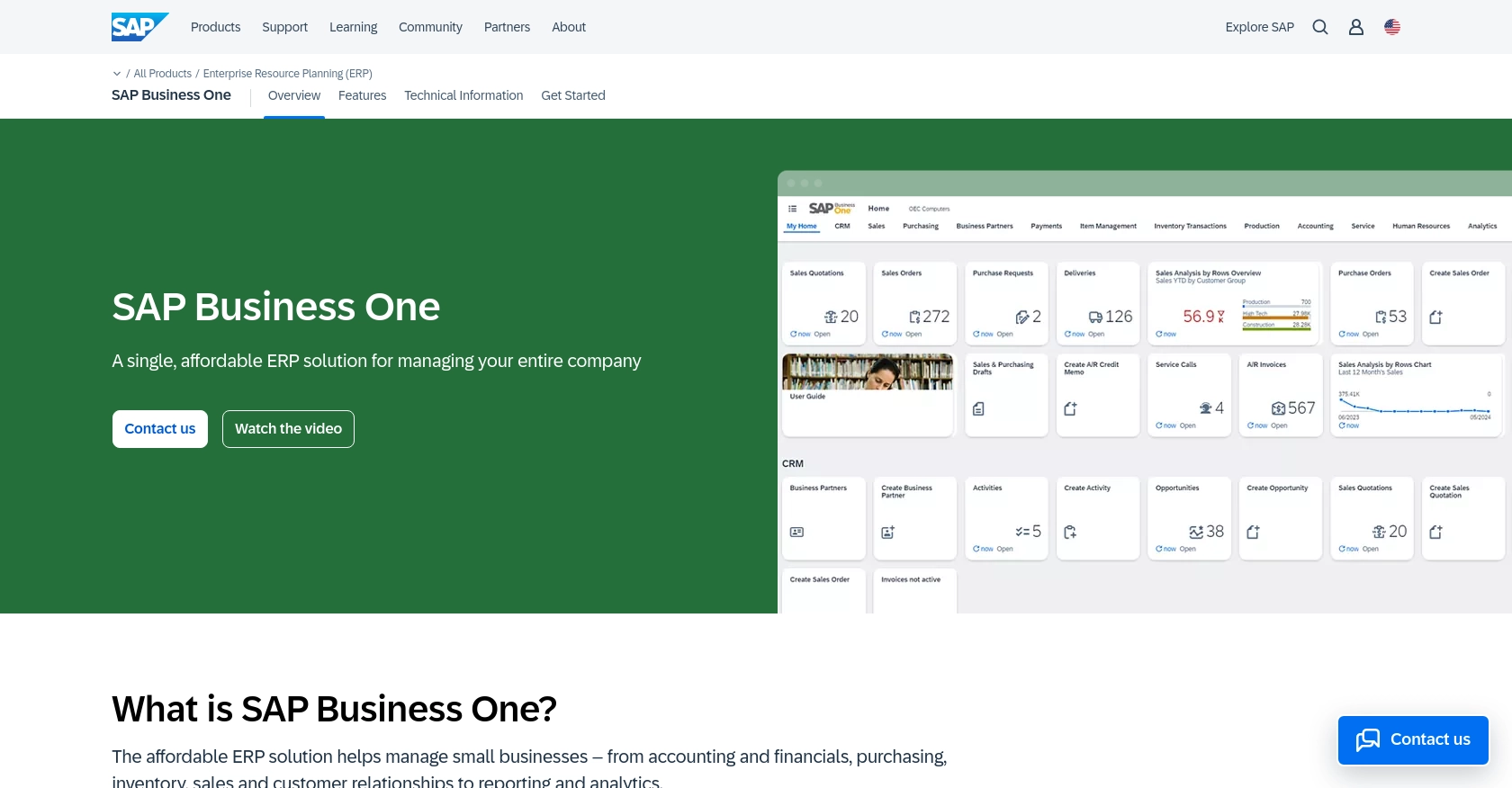
Introduction to SAP Business One API
SAP Business One is a comprehensive enterprise resource planning (ERP) solution designed for small to medium-sized businesses. It offers a wide range of functionalities, including financial management, sales, customer relationship management, and inventory control, all within a single platform.
Integrating with the SAP Business One API allows developers to automate and streamline business processes by interacting directly with the system's data. For example, a developer might use the API to create or update inventory items, ensuring that product information is always current and accurate. This capability is crucial for businesses looking to maintain efficient operations and accurate reporting.
Setting Up Your SAP Business One Test or Sandbox Account
Before you can start integrating with the SAP Business One API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your live data. SAP Business One provides a comprehensive sandbox environment for developers to test their integrations.
Creating a SAP Business One Sandbox Account
To begin, you'll need to sign up for a SAP Business One sandbox account. Visit the SAP Business One Service Layer documentation for detailed instructions on how to create a sandbox account. This document provides step-by-step guidance on setting up your environment.
Configuring OAuth Authentication for SAP Business One
SAP Business One uses a custom authentication method to secure API access. To authenticate, you'll need to create an application within your sandbox account. Follow these steps to configure OAuth authentication:
- Log in to your SAP Business One sandbox account.
- Navigate to the 'Applications' section and click on 'Create New Application'.
- Fill in the required details, including the application name and description.
- Once the application is created, you will receive a client ID and client secret. Keep these credentials secure as they are essential for API authentication.
Generating API Keys for SAP Business One
In addition to OAuth credentials, you may need to generate API keys for certain operations. Here's how to obtain your API key:
- Within your sandbox account, go to the 'API Keys' section.
- Click on 'Generate New Key' and provide a name for your key.
- Copy the generated API key and store it securely. This key will be used in your API requests.
With your sandbox account set up and authentication configured, you're ready to start making API calls to SAP Business One. This setup ensures you can test your integration thoroughly before deploying it to a live environment.
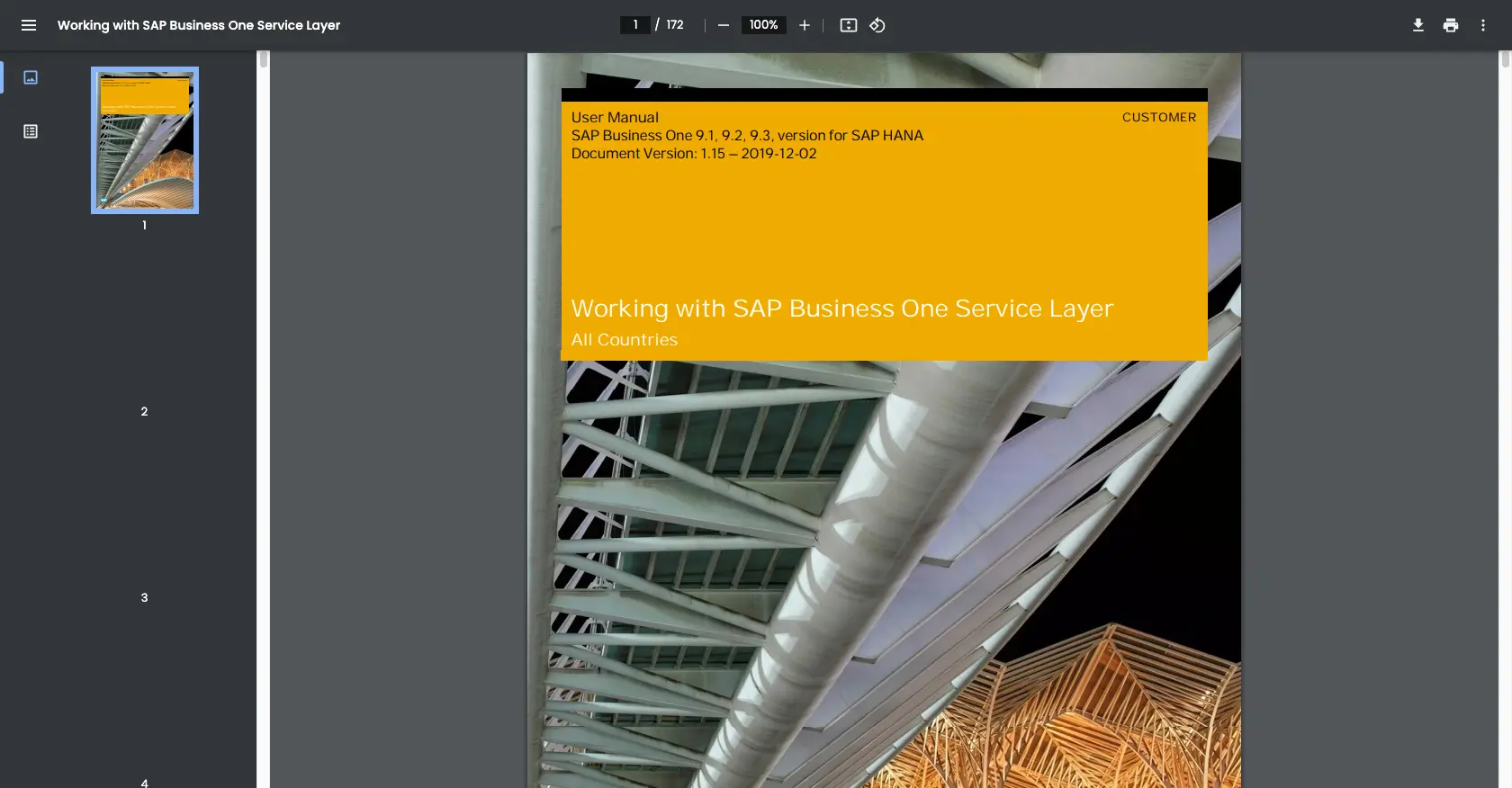
sbb-itb-96038d7
Making API Calls to SAP Business One Using Python
To interact with the SAP Business One API, you'll need to use Python to make HTTP requests. This section will guide you through the process of setting up your Python environment, writing the code to create or update items, and handling potential errors.
Setting Up Your Python Environment for SAP Business One API Integration
Before you begin, ensure you have Python 3.x installed on your machine. You'll also need to install the requests
library, which allows you to send HTTP requests easily.
pip install requests
Creating or Updating Items in SAP Business One Using Python
Once your environment is ready, you can proceed to write the code to interact with the SAP Business One API. Below is a sample script to create or update items:
import requests
import json
# Define the API endpoint and headers
url = "https://your-sap-business-one-instance.com/b1s/v1/Items"
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Token"
}
# Define the item data
item_data = {
"ItemCode": "A0001",
"ItemName": "Sample Item",
"ItemType": "itInventory",
"InventoryItem": "tYES"
}
# Make a POST request to create or update the item
response = requests.post(url, headers=headers, data=json.dumps(item_data))
# Check the response status
if response.status_code == 201:
print("Item created successfully.")
elif response.status_code == 204:
print("Item updated successfully.")
else:
print(f"Failed to create or update item. Status code: {response.status_code}")
Replace Your_Token
with the token you obtained during the authentication setup. This script sends a POST request to the SAP Business One API to create or update an item. The response status code will indicate whether the operation was successful.
Verifying API Call Success in SAP Business One Sandbox
After running the script, verify the success of the API call by checking the SAP Business One sandbox environment. Navigate to the items section to confirm that the item has been created or updated as expected.
Handling Errors and Error Codes in SAP Business One API
It's important to handle errors gracefully when making API calls. The SAP Business One API may return various error codes, such as 400 for bad requests or 401 for unauthorized access. Ensure your code checks for these errors and handles them appropriately, possibly by logging the error details or retrying the request.
Conclusion and Best Practices for SAP Business One API Integration
Integrating with the SAP Business One API using Python can significantly enhance your business processes by automating the creation and updating of inventory items. By following the steps outlined in this guide, you can ensure a seamless integration experience.
Best Practices for Secure and Efficient SAP Business One API Usage
- Secure Storage of Credentials: Always store your API credentials, such as tokens and API keys, securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handling Rate Limits: Be mindful of the API rate limits to avoid throttling. Implement exponential backoff strategies to manage retries effectively.
- Data Transformation and Standardization: Ensure that data fields are transformed and standardized according to your business requirements before sending them to the API.
- Error Handling: Implement robust error handling to manage API errors gracefully. Log errors for debugging and consider retry mechanisms for transient issues.
Streamlining Integrations with Endgrate
While integrating with SAP Business One can be highly beneficial, managing multiple integrations can become complex. Endgrate simplifies this process by providing a unified API endpoint for various platforms, including SAP Business One. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the advantages of a streamlined integration experience.
Read More
Ready to get started?