How to Get People with the Salesloft API in Python
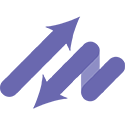
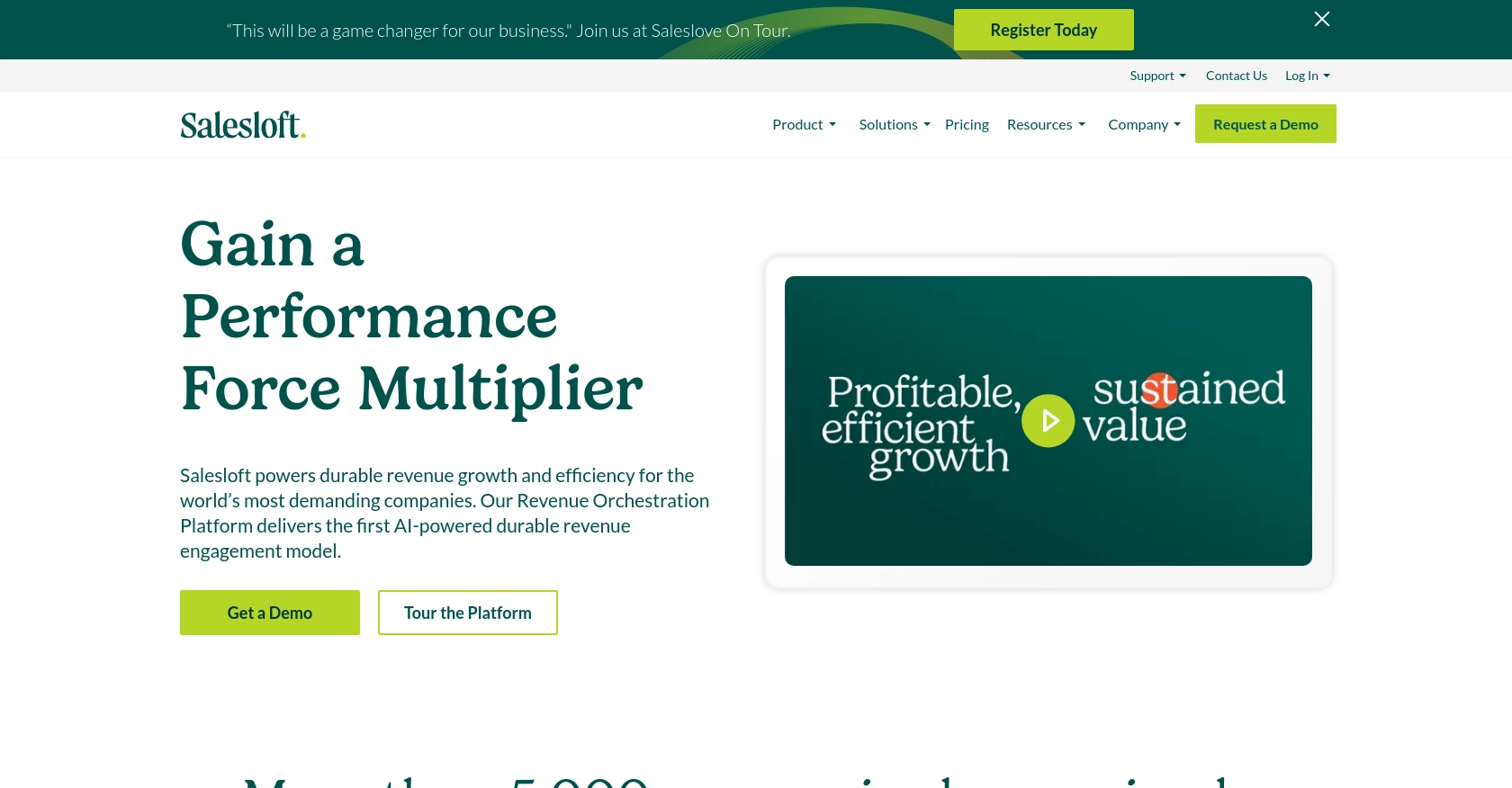
Introduction to Salesloft API Integration
Salesloft is a powerful sales engagement platform designed to enhance the efficiency and effectiveness of sales teams. It offers a suite of tools that streamline communication, automate workflows, and provide valuable insights into sales processes.
Integrating with the Salesloft API allows developers to access and manage sales data programmatically, enabling seamless integration with other systems. For example, you might want to retrieve a list of people from Salesloft to analyze engagement metrics or synchronize contact information with a CRM system.
This article will guide you through the process of using Python to interact with the Salesloft API, specifically focusing on retrieving people data. By following these steps, you can efficiently access and utilize Salesloft's robust features within your applications.
Setting Up Your Salesloft Test Account
Before you can begin interacting with the Salesloft API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Create a Salesloft Account
If you don't already have a Salesloft account, you can sign up for a free trial or demo account on the Salesloft website. This will give you access to the platform's features and allow you to create an OAuth application for API access.
Generate OAuth Credentials for Salesloft API
Salesloft uses OAuth 2.0 for authentication, which requires you to create an OAuth application to obtain the necessary credentials. Follow these steps:
- Log in to your Salesloft account and navigate to Your Applications under the account settings.
- Select OAuth Applications and click on Create New.
- Fill in the required fields, such as the application name and redirect URI, then click Save.
- Once saved, you will receive your Client ID and Client Secret. Keep these credentials secure as they will be used to authenticate your API requests.
Obtain Authorization Code and Access Tokens
To interact with the Salesloft API, you'll need to obtain an access token. Here's how:
- Generate a request to the authorization endpoint by replacing
YOUR_CLIENT_ID
andYOUR_REDIRECT_URI
in the following URL: - Authorize the application when prompted. Upon approval, you'll receive an authorization code.
- Exchange the authorization code for an access token by making a POST request to the token endpoint:
- The response will include an access_token and a refresh_token. Use the access token for API requests and the refresh token to obtain a new access token when needed.
https://accounts.salesloft.com/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&response_type=code
POST https://accounts.salesloft.com/oauth/token
{
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET",
"code": "AUTHORIZATION_CODE",
"grant_type": "authorization_code",
"redirect_uri": "YOUR_REDIRECT_URI"
}
With your test account and OAuth credentials set up, you're ready to start making API calls to retrieve people data from Salesloft.
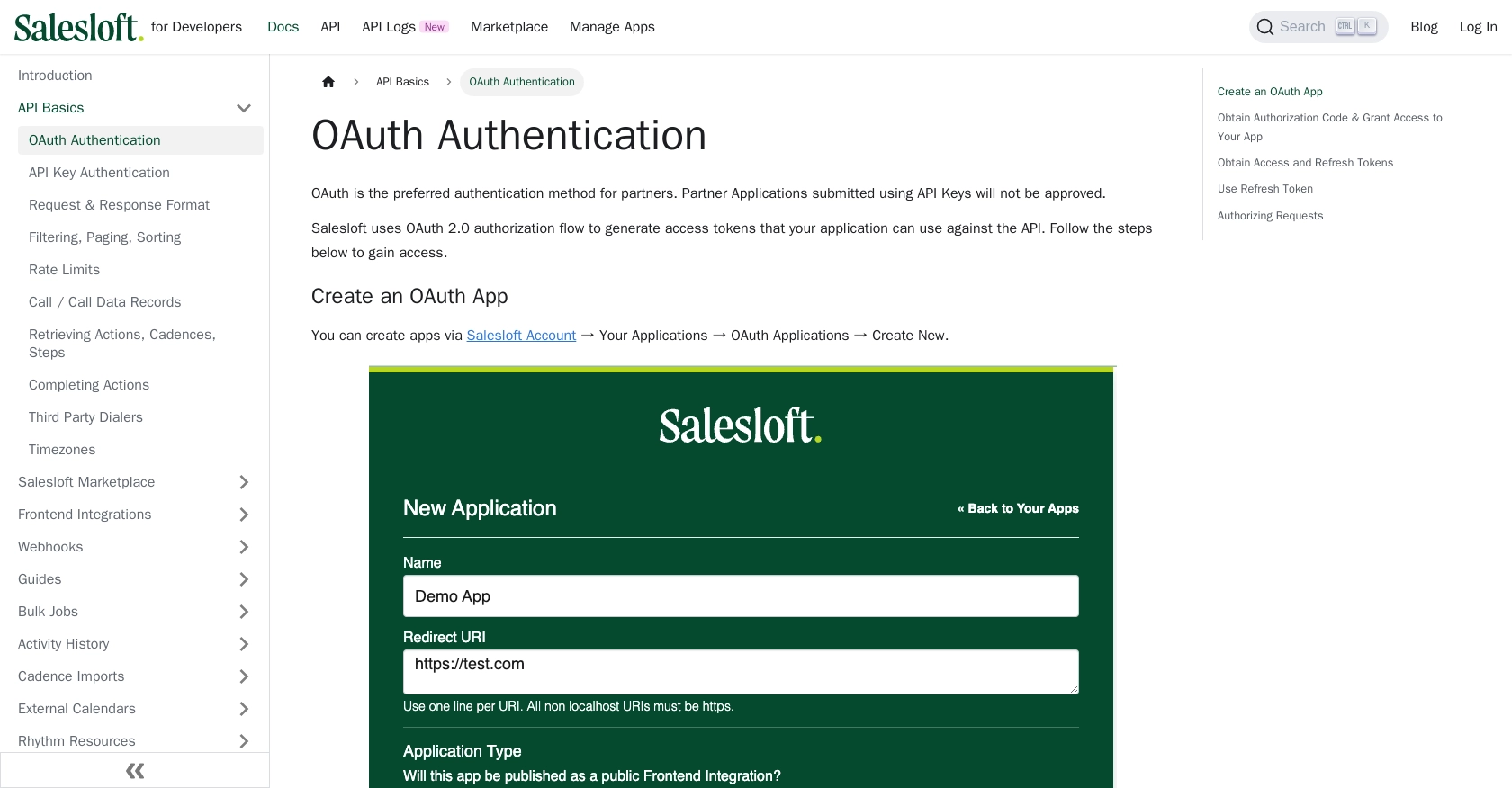
sbb-itb-96038d7
Making API Calls to Retrieve People Data from Salesloft Using Python
Now that you have your Salesloft test account and OAuth credentials set up, you can start making API calls to retrieve people data. This section will guide you through the process of using Python to interact with the Salesloft API.
Setting Up Your Python Environment for Salesloft API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using pip:
pip install requests
Writing Python Code to Fetch People Data from Salesloft
Create a new Python file named get_salesloft_people.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://api.salesloft.com/v2/people"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Accept": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
# Loop through the people and print their information
for person in data["data"]:
print(f"Name: {person['first_name']} {person['last_name']}, Email: {person['email_address']}")
else:
print(f"Failed to retrieve data: {response.status_code} - {response.text}")
Replace YOUR_ACCESS_TOKEN
with the access token you obtained earlier. This script sends a GET request to the Salesloft API to fetch people data and prints out their names and email addresses.
Running the Python Script and Verifying the Output
Execute the script from your terminal or command line:
python get_salesloft_people.py
If successful, you should see a list of people with their names and email addresses printed in the console. If there are any errors, the script will output the error code and message.
Handling Errors and Understanding Salesloft API Responses
Salesloft API responses include status codes and error messages to help you troubleshoot issues. Common error codes include:
- 403 Forbidden: You don't have permission to access the resource.
- 404 Not Found: The requested resource doesn't exist.
- 422 Unprocessable Entity: The request was well-formed but couldn't be processed due to semantic errors.
For more details on error handling, refer to the Salesloft API documentation.
Optimizing API Calls with Salesloft Rate Limits
Salesloft enforces a rate limit of 600 requests per minute per team. Be mindful of this limit to avoid throttling. For more information, see the Salesloft rate limits documentation.
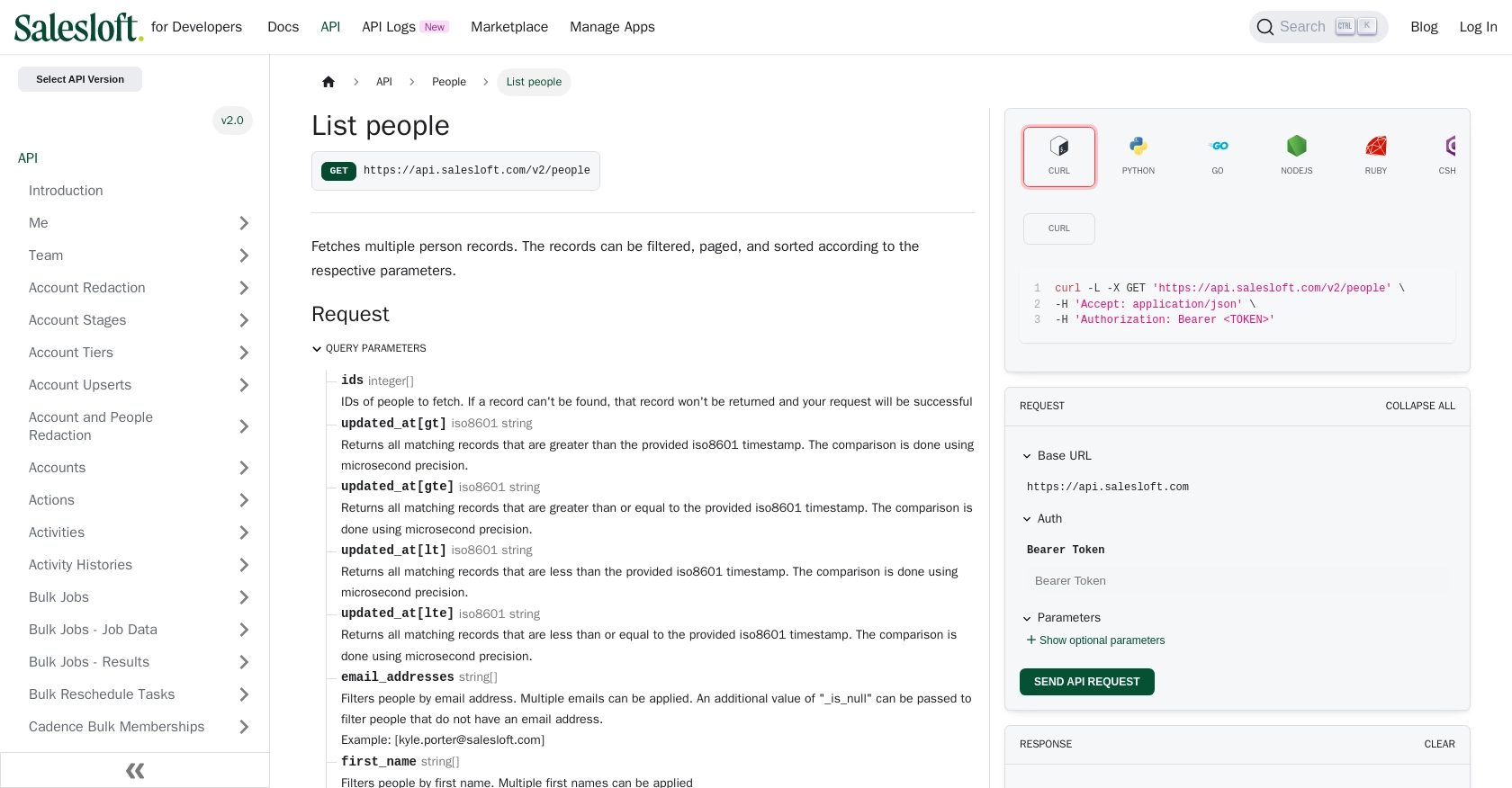
Conclusion and Best Practices for Integrating with Salesloft API Using Python
Integrating with the Salesloft API using Python provides a powerful way to access and manage sales data, enhancing your ability to streamline workflows and improve sales efficiency. By following the steps outlined in this guide, you can effectively retrieve and utilize people data from Salesloft within your applications.
Best Practices for Secure and Efficient Salesloft API Integration
- Securely Store Credentials: Always store your OAuth credentials, such as the client ID, client secret, and access tokens, securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limits: Be aware of Salesloft's rate limit of 600 requests per minute per team. Implement logic to handle rate limit responses gracefully and consider using exponential backoff strategies to retry requests.
- Refresh Tokens Regularly: Access tokens have a limited lifespan. Use the refresh token to obtain new access tokens without user intervention, ensuring uninterrupted access to the API.
- Optimize Data Handling: When retrieving large datasets, consider using pagination to manage data efficiently. Salesloft provides parameters for paging, filtering, and sorting to help you tailor your requests.
Enhancing Your Integration Experience with Endgrate
While integrating with the Salesloft API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development.
With Endgrate, you can:
- Save time and resources by outsourcing integration management.
- Build once for each use case instead of multiple times for different integrations.
- Provide an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/salesloft
- https://developers.salesloft.com/docs/platform/api-basics/oauth-authentication/
- https://developers.salesloft.com/docs/platform/api-basics/request-response-format/
- https://developers.salesloft.com/docs/platform/api-basics/filtering-paging-sorting/
- https://developers.salesloft.com/docs/platform/api-basics/rate-limits/
- https://developers.salesloft.com/docs/api/people-index/
Ready to get started?