How to Create or Update Companies with the Keap API in Python
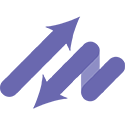
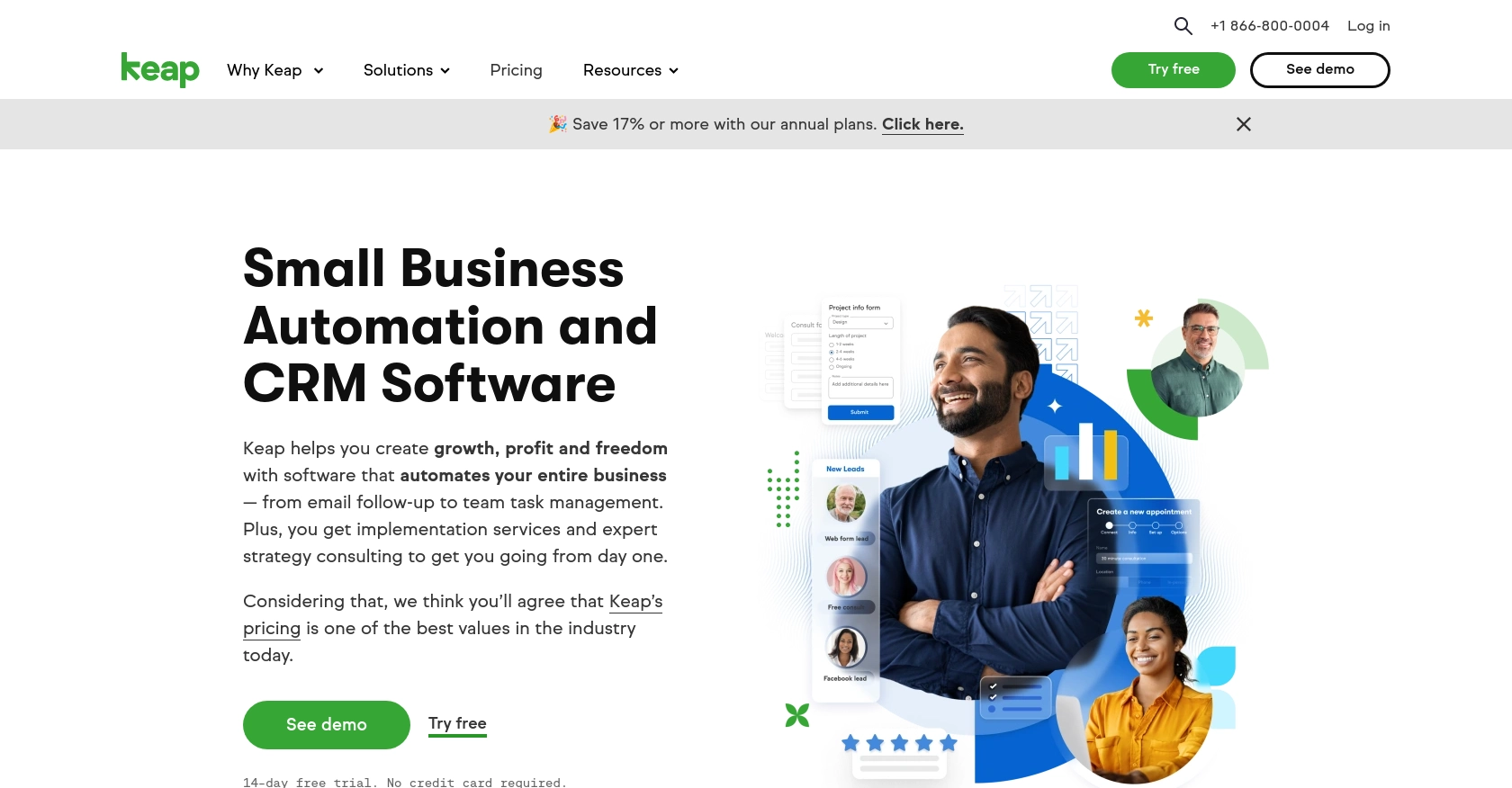
Introduction to Keap API for Company Management
Keap, formerly known as Infusionsoft, is a robust CRM and marketing automation platform designed to help small businesses streamline their sales and marketing efforts. With its comprehensive suite of tools, Keap enables businesses to manage customer relationships, automate marketing campaigns, and track sales activities effectively.
For developers, integrating with the Keap API offers the opportunity to enhance business processes by automating tasks such as creating or updating company records. For example, a developer might use the Keap API to automatically update company information in response to changes in a connected system, ensuring that all data remains consistent and up-to-date across platforms.
This article will guide you through the process of using Python to interact with the Keap API, specifically focusing on creating or updating company records. By following this tutorial, you'll learn how to efficiently manage company data within the Keap platform, leveraging Python's capabilities to streamline your integration efforts.
Setting Up Your Keap Developer Account and Sandbox Environment
Before you can start integrating with the Keap API, you'll need to set up a developer account and create a sandbox environment. This will allow you to safely test your API interactions without affecting live data.
Registering for a Keap Developer Account
To begin, you'll need to register for a Keap developer account. This account will provide you with access to the necessary tools and resources to build and test your integration.
- Visit the Keap Developer Portal.
- Click on the "Register" button to create a new developer account.
- Fill out the registration form with your details and submit it.
- Once registered, log in to your developer account to access the dashboard.
Creating a Keap Sandbox App for Testing
With your developer account set up, the next step is to create a sandbox app. This environment will allow you to test API calls without impacting your production data.
- Navigate to the "Sandbox" section in your developer dashboard.
- Click on "Create Sandbox App" and provide the necessary information.
- Once created, you'll receive a client ID and client secret, which are essential for OAuth authentication.
Configuring OAuth Authentication for Keap API
The Keap API uses OAuth 2.0 for authentication. Follow these steps to configure OAuth for your sandbox app:
- In your sandbox app settings, locate the "OAuth" section.
- Enter a redirect URI, which is the URL where users will be redirected after authentication.
- Save the settings to generate your OAuth credentials.
You'll use the client ID and client secret obtained here to authenticate API requests in your Python application.
With your Keap developer account and sandbox app set up, you're ready to start making API calls to create or update company records. In the next section, we'll dive into the specifics of using Python to interact with the Keap API.
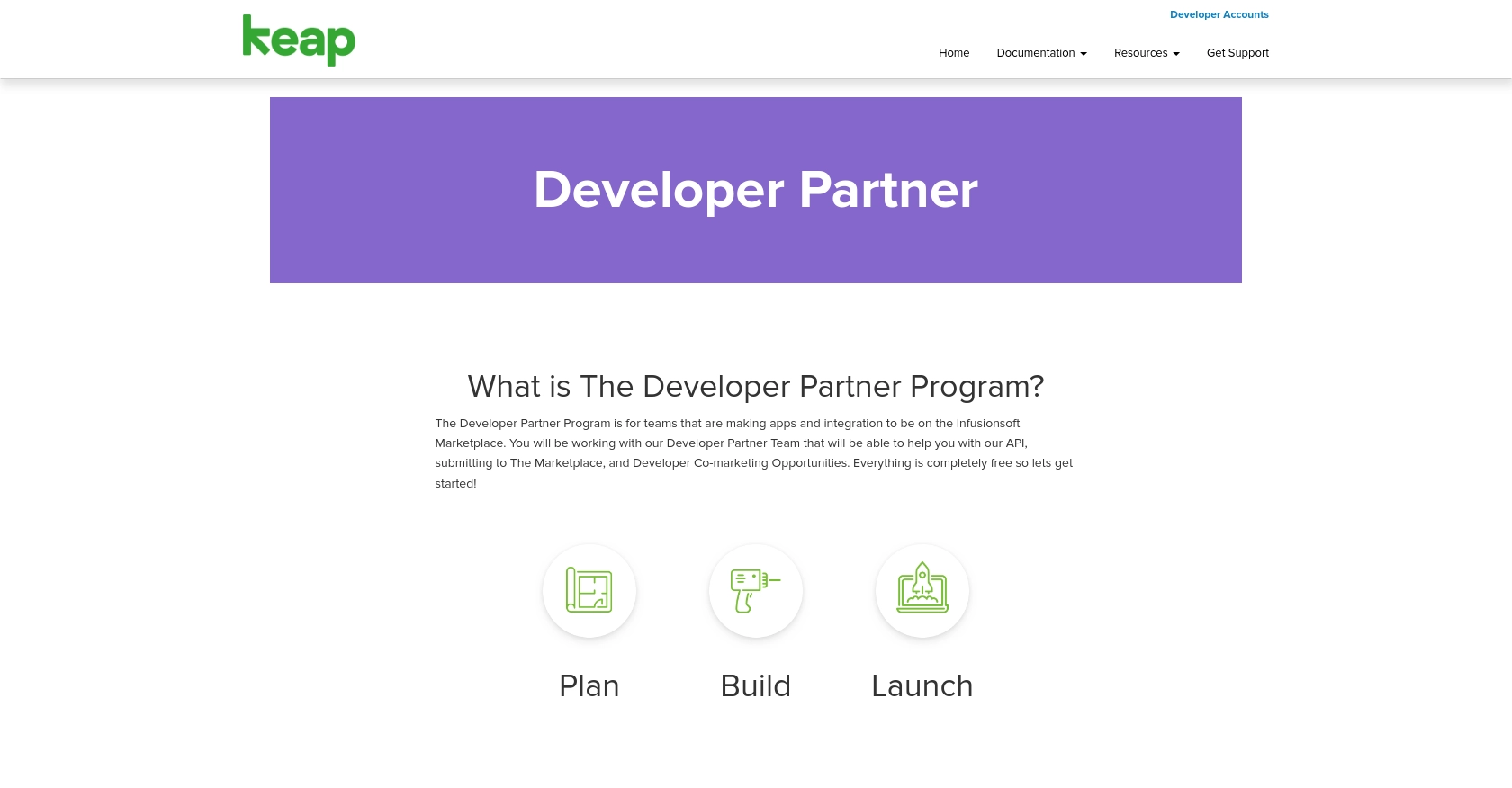
sbb-itb-96038d7
Making API Calls to Create or Update Companies with Keap API Using Python
With your Keap developer account and sandbox app ready, it's time to dive into making API calls using Python. This section will guide you through the process of setting up your Python environment, writing the code to interact with the Keap API, and handling responses effectively.
Setting Up Your Python Environment for Keap API Integration
Before you start coding, ensure your Python environment is properly set up. You'll need Python 3.x and the necessary libraries to make HTTP requests and handle JSON data.
- Ensure Python 3.x is installed on your machine. You can download it from the official Python website.
- Install the required libraries using pip:
pip install requests
Writing Python Code to Create or Update Companies in Keap
Now, let's write the Python code to interact with the Keap API. We'll focus on creating or updating company records. Replace Your_Client_ID
, Your_Client_Secret
, and Your_Access_Token
with your actual credentials.
import requests
# Set the API endpoint for creating or updating companies
url = "https://api.infusionsoft.com/crm/rest/v1/companies"
# Set the request headers
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json"
}
# Define the company data
company_data = {
"company_name": "Example Company",
"email": "contact@example.com",
"phone": "123-456-7890"
}
# Make the POST request to create or update the company
response = requests.post(url, json=company_data, headers=headers)
# Check the response status
if response.status_code == 200:
print("Company created or updated successfully.")
else:
print(f"Failed to create or update company. Status code: {response.status_code}")
print(response.json())
In this code, we use the requests
library to make a POST request to the Keap API. The company_data
dictionary contains the information for the company you want to create or update. The response is checked to ensure the operation was successful.
Verifying API Call Success and Handling Errors
After executing the API call, it's crucial to verify the success of the operation. You can do this by checking the response status code and reviewing the returned data.
- If the status code is
200
, the company was successfully created or updated. - If the status code indicates an error, review the response JSON for more details.
Handling errors gracefully is essential. Ensure your application can manage different error scenarios, such as invalid data or authentication issues.
By following these steps, you can efficiently create or update company records in Keap using Python. This integration allows you to automate and streamline your business processes, ensuring data consistency across platforms.
Best Practices for Keap API Integration in Python
Integrating with the Keap API using Python can significantly enhance your business processes by automating tasks and ensuring data consistency. Here are some best practices to consider when working with the Keap API:
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of the API rate limits to avoid exceeding the allowed number of requests. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that data fields are standardized across your systems to maintain consistency. This will help in seamless data integration and reduce errors.
- Implement Error Handling: Develop robust error handling to manage different scenarios, such as network issues or invalid data. Log errors for troubleshooting and improve your application's resilience.
Streamlining Integrations with Endgrate
While integrating with the Keap API can be highly beneficial, managing multiple integrations can become complex and time-consuming. This is where Endgrate can make a difference.
Endgrate offers a unified API endpoint that connects to various platforms, including Keap, allowing you to manage all your integrations through a single interface. By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations, simplifying your development process.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can streamline your integration efforts and help you scale your business efficiently. Visit Endgrate to learn more and get started today.
Read More
Ready to get started?