How to Get Invoices with the Chargebee API in Python
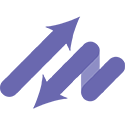
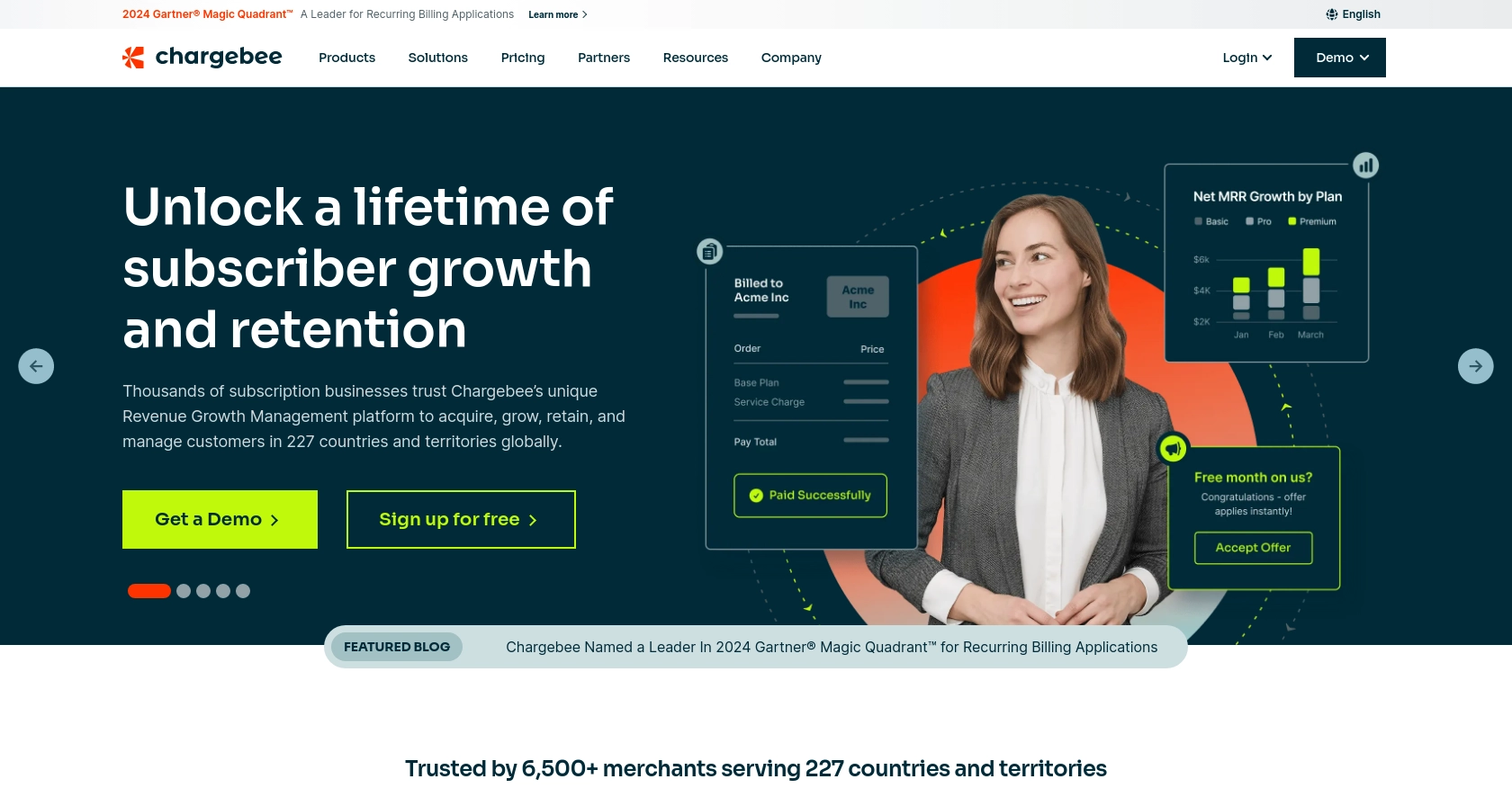
Introduction to Chargebee API for Invoice Management
Chargebee is a robust subscription billing and revenue management platform designed to streamline billing processes for businesses of all sizes. It offers a comprehensive suite of tools to manage subscriptions, invoicing, payments, and more, making it a popular choice among SaaS companies.
Integrating with Chargebee's API allows developers to automate and enhance billing operations, such as retrieving and managing invoices. For example, a developer might use the Chargebee API to fetch invoice details and integrate them into a custom dashboard, providing real-time financial insights to the business.
Setting Up Your Chargebee Test/Sandbox Account for API Integration
Before you can start using the Chargebee API to manage invoices, you need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your live data.
Creating a Chargebee Test Account
To begin, sign up for a Chargebee test account. Visit the Chargebee website and select the option to create a new account. Choose the test environment to ensure that your operations do not impact live data.
Generating API Keys for Chargebee
Once your test account is set up, you need to generate API keys to authenticate your API requests. Follow these steps:
- Log in to your Chargebee test account.
- Navigate to the API Keys section in the admin console.
- Click on Create API Key and provide a name for your key.
- Copy the generated API key and store it securely, as it will be used for authenticating your API requests.
Understanding Chargebee's Custom Authentication
Chargebee uses HTTP Basic authentication for API calls. The username is your API key, while the password is left empty. This method ensures secure communication between your application and Chargebee's servers.
Configuring OAuth for Chargebee API
Although Chargebee primarily uses API keys, you can also configure OAuth for additional security. To set up OAuth:
- Navigate to the OAuth Apps section in your Chargebee account.
- Create a new OAuth app and note down the client ID and client secret.
- Use these credentials to authenticate your API requests via OAuth.
With your Chargebee test account and API keys ready, you're all set to start integrating and managing invoices using the Chargebee API in Python.
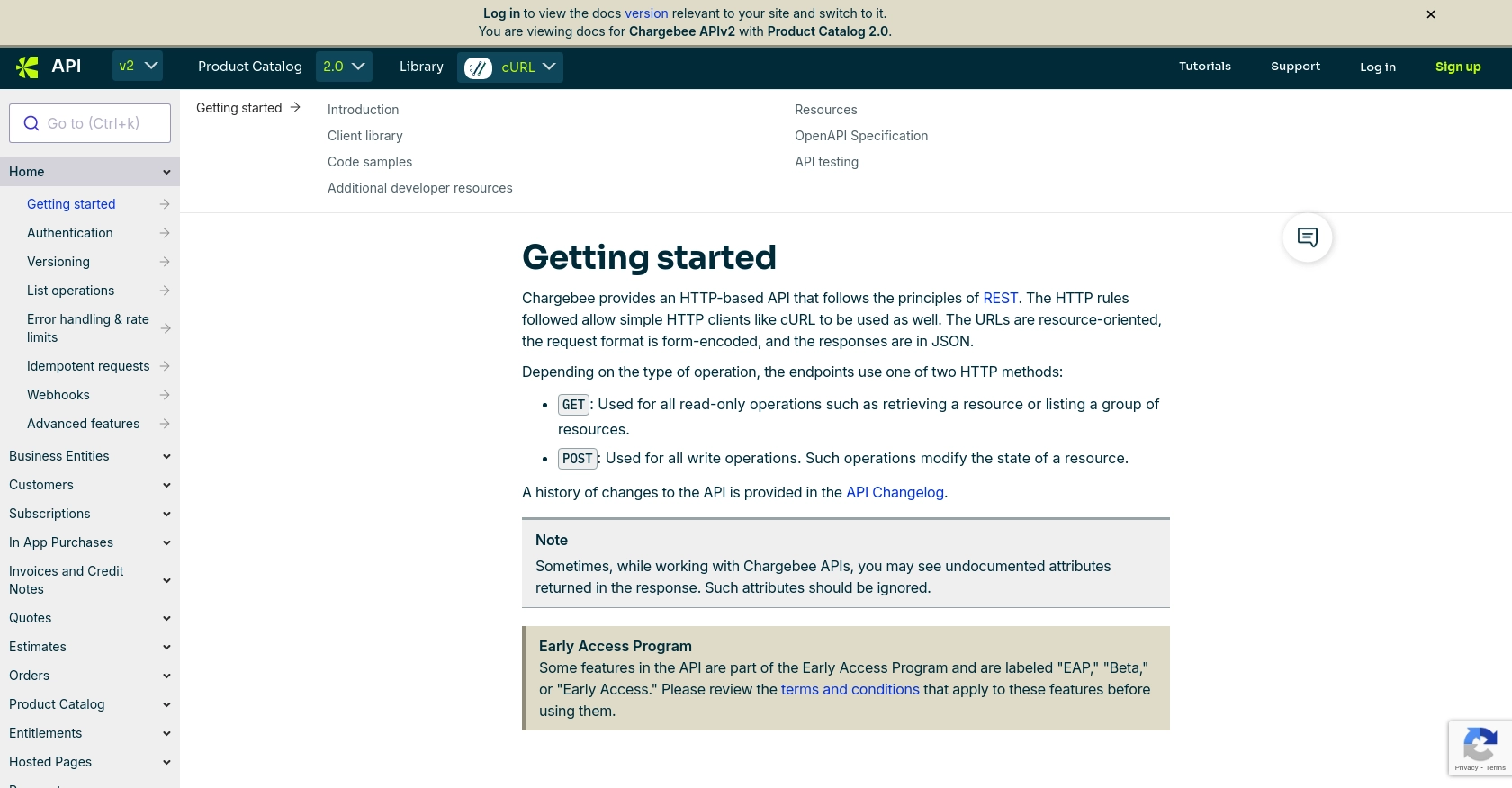
sbb-itb-96038d7
Making API Calls to Retrieve Invoices with Chargebee API in Python
To interact with the Chargebee API and retrieve invoices, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses.
Setting Up Your Python Environment for Chargebee API Integration
Before you begin coding, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer
pip
Next, install the requests
library, which will be used to make HTTP requests to the Chargebee API:
pip install requests
Writing Python Code to Fetch Invoices from Chargebee
Create a new Python file named get_chargebee_invoices.py
and add the following code:
import requests
# Set the API endpoint and authentication details
endpoint = "https://{site}.chargebee.com/api/v2/invoices"
api_key = "your_api_key_here"
# Set the headers for the request
headers = {
"Authorization": f"Basic {api_key}:",
"Content-Type": "application/json"
}
# Make a GET request to the Chargebee API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
invoices = response.json()
for invoice in invoices['list']:
print(invoice['invoice']['id'], invoice['invoice']['status'])
else:
print(f"Failed to retrieve invoices: {response.status_code}")
Replace your_api_key_here
with the API key you generated in your Chargebee test account.
Understanding the Output and Verifying Success
When you run the script using the command python get_chargebee_invoices.py
, you should see a list of invoice IDs and their statuses printed to the console. This confirms that the API call was successful and that you have retrieved the invoice data from Chargebee.
Handling Errors and Chargebee API Error Codes
It's crucial to handle potential errors when making API calls. Chargebee uses standard HTTP status codes to indicate success or failure. Here are some common error codes you might encounter:
- 401 Unauthorized: Authentication failed. Check your API key.
- 404 Not Found: The requested resource could not be found.
- 429 Too Many Requests: Rate limit exceeded. Implement retry logic with exponential backoff.
For more detailed error handling, refer to the Chargebee API documentation: Chargebee Error Handling.
Checking the Chargebee Sandbox for Retrieved Invoices
After running your script, log in to your Chargebee sandbox account to verify that the invoices retrieved match those in your test environment. This ensures that your integration is functioning correctly.
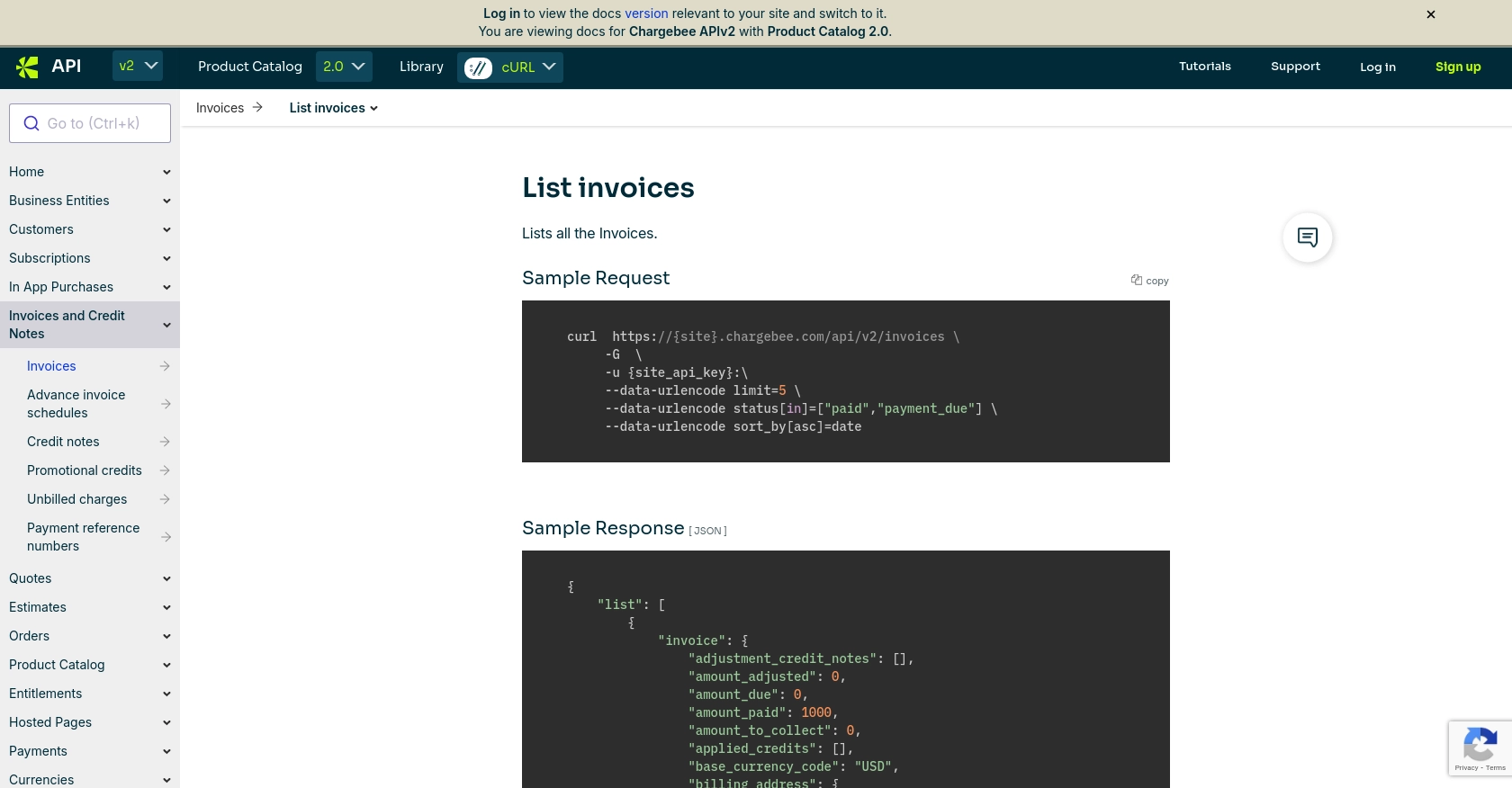
Conclusion and Best Practices for Chargebee API Integration in Python
Integrating with the Chargebee API using Python provides a powerful way to automate and manage your invoicing processes. By following the steps outlined in this guide, you can efficiently retrieve and handle invoice data, enhancing your business's financial operations.
Best Practices for Secure and Efficient Chargebee API Usage
- Secure API Keys: Always store your API keys securely and avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Implement Rate Limiting: Chargebee enforces rate limits on API requests. To avoid hitting these limits, implement retry logic with exponential backoff. For more details, refer to the Chargebee API Rate Limits.
- Data Standardization: Ensure that the data retrieved from Chargebee is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement comprehensive error handling to manage API call failures gracefully. Utilize Chargebee's error codes to provide meaningful feedback and troubleshooting information.
Streamline Your Integrations with Endgrate
While integrating with Chargebee's API can be highly beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration management across various platforms, including Chargebee.
By using Endgrate, you can save time and resources, allowing your team to focus on core product development while ensuring a seamless integration experience for your customers. Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
Ready to get started?