How to Create Invoices with the Avalara API in PHP
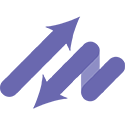
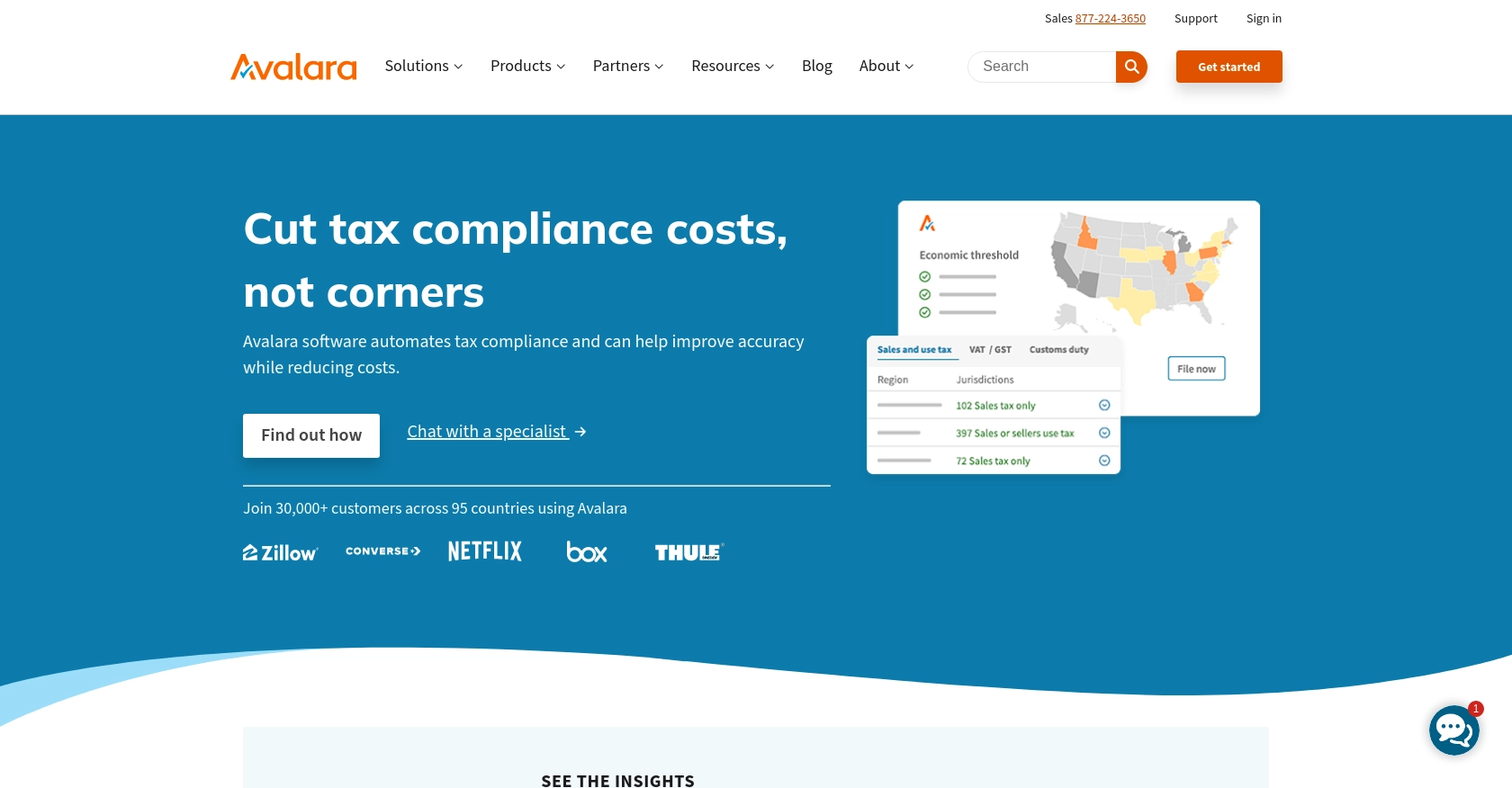
Introduction to Avalara and Its API for Invoice Creation
Avalara is a renowned provider of cloud-based tax compliance solutions, offering a comprehensive suite of tools to automate tax calculations, returns, and compliance processes for businesses of all sizes. Its flagship product, AvaTax, is widely used for accurate tax calculations across various industries, including retail, ecommerce, and manufacturing.
Integrating with Avalara's API allows developers to streamline the invoicing process by automating tax calculations and compliance checks. For example, a developer might use the Avalara API to create invoices that automatically apply the correct tax rates based on the customer's location, ensuring compliance and reducing manual errors.
This article will guide you through the process of creating invoices using the Avalara API in PHP, providing step-by-step instructions and code examples to help you efficiently manage tax-related tasks in your applications.
Setting Up Your Avalara Sandbox Account for API Integration
Before diving into the Avalara API integration, it's essential to set up a sandbox account. This environment allows developers to test API calls without affecting live data, ensuring a smooth development process.
Creating an Avalara Sandbox Account
If you don't already have an Avalara account, you can sign up for a free trial or sandbox account on the Avalara website. This will give you access to the necessary tools and credentials for testing.
- Visit the Avalara Developer Portal.
- Click on "Sign Up" to create a new account.
- Follow the on-screen instructions to complete the registration process.
Generating API Credentials for Avalara
Once your sandbox account is set up, you'll need to generate API credentials to authenticate your requests. Avalara supports Basic HTTP Authentication, which requires a username and password.
- Log in to your Avalara account.
- Navigate to the "Settings" section.
- Select "Reset License Key" to generate a new license key. Note that this action is restricted to account administrators.
- Record your account ID and the newly generated license key. These will be used to authenticate API requests.
For more details on authentication, refer to the Avalara Authentication Documentation.
Configuring Your PHP Environment for Avalara API
To interact with the Avalara API using PHP, ensure your environment is correctly configured:
- Ensure PHP is installed on your machine. PHP 7.4 or later is recommended.
- Install the necessary PHP extensions, such as cURL, to handle HTTP requests.
With your sandbox account and API credentials ready, you can now proceed to make API calls to Avalara, starting with creating invoices.
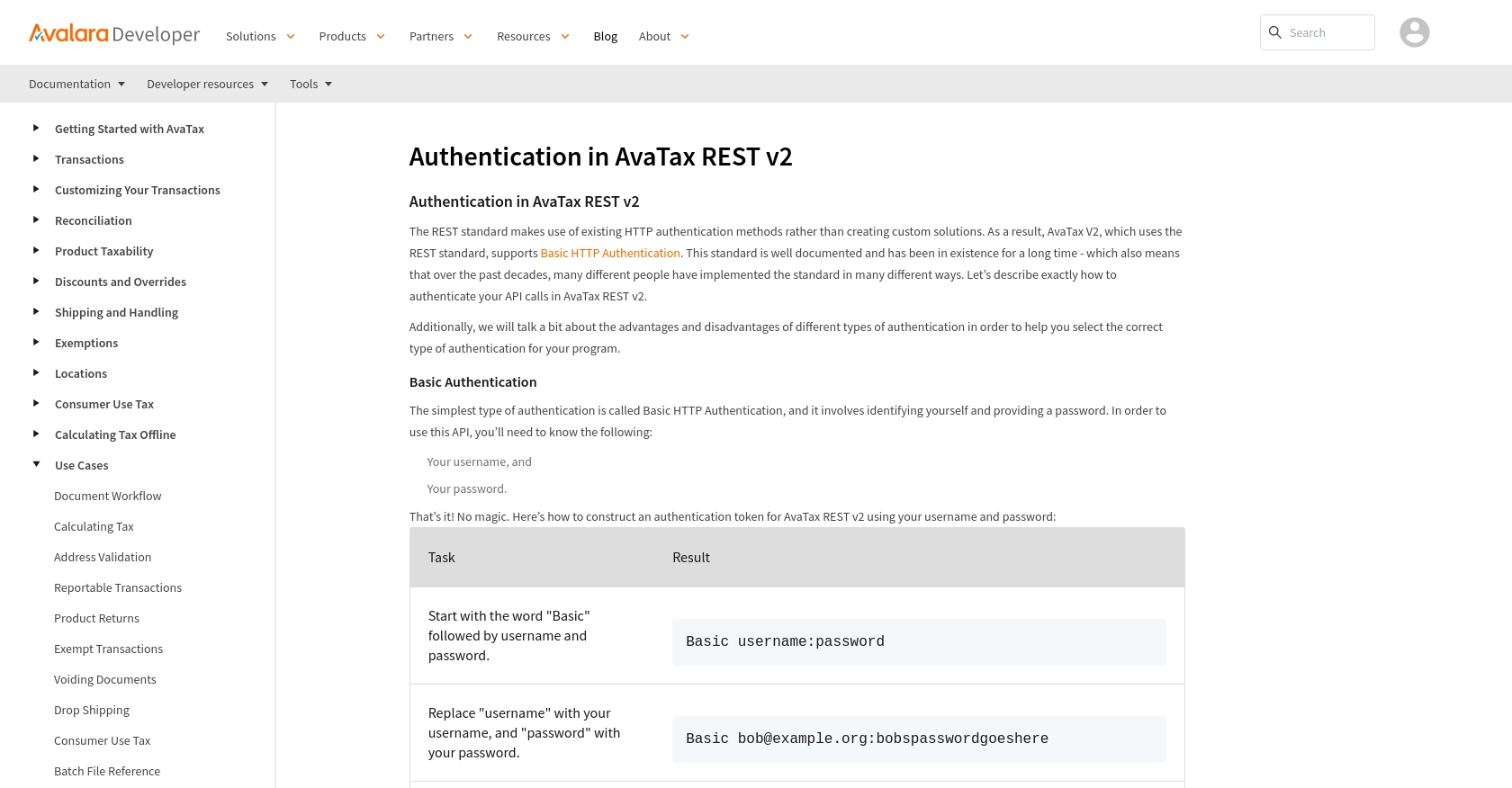
sbb-itb-96038d7
Making API Calls to Create Invoices with Avalara in PHP
To create invoices using the Avalara API in PHP, you need to ensure your environment is set up correctly and that you have the necessary credentials. This section will guide you through the process of making API calls to Avalara's CreateTransaction endpoint, which is essential for generating invoices.
Setting Up Your PHP Environment for Avalara API Integration
Before making API calls, ensure your PHP environment is ready:
- Ensure PHP 7.4 or later is installed on your machine.
- Install the cURL extension for PHP to handle HTTP requests.
Creating an Invoice with Avalara's CreateTransaction API
To create an invoice, you'll use the CreateTransaction endpoint provided by Avalara. This endpoint allows you to record transactions, which include sales, purchases, and other taxable actions.
// Set your Avalara account credentials
$accountId = 'Your_Account_ID';
$licenseKey = 'Your_License_Key';
// Encode credentials for Basic Authentication
$credentials = base64_encode("$accountId:$licenseKey");
// Define the API endpoint
$url = 'https://sandbox-rest.avatax.com/api/v2/transactions/create';
// Prepare the invoice data
$data = [
'lines' => [
[
'number' => '1',
'quantity' => 1,
'amount' => 100,
'taxCode' => 'PS081282',
'itemCode' => 'Y0001',
'description' => 'Yarn'
]
],
'type' => 'SalesInvoice',
'companyCode' => 'DEFAULT',
'date' => '2024-09-06',
'customerCode' => 'ABC',
'purchaseOrderNo' => '2024-09-06-001',
'addresses' => [
'singleLocation' => [
'line1' => '2000 Main Street',
'city' => 'Irvine',
'region' => 'CA',
'country' => 'US',
'postalCode' => '92614'
]
],
'commit' => true,
'currencyCode' => 'USD',
'description' => 'Yarn'
];
// Initialize cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Basic ' . $credentials,
'Content-Type: application/json'
]);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the API call
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Decode the response
$result = json_decode($response, true);
echo 'Invoice Created Successfully: ' . $result['id'];
}
// Close cURL session
curl_close($ch);
Replace Your_Account_ID
and Your_License_Key
with your actual Avalara account ID and license key. The code above initializes a cURL session, sets the necessary headers for authentication, and sends a POST request to the Avalara API with the invoice data.
Verifying the API Call and Handling Errors
After executing the API call, you should verify the response to ensure the invoice was created successfully. The response will include details such as the transaction ID and status. If the request fails, handle errors appropriately by checking the error message returned by the API.
For more information on error codes and handling, refer to the Avalara API Documentation.
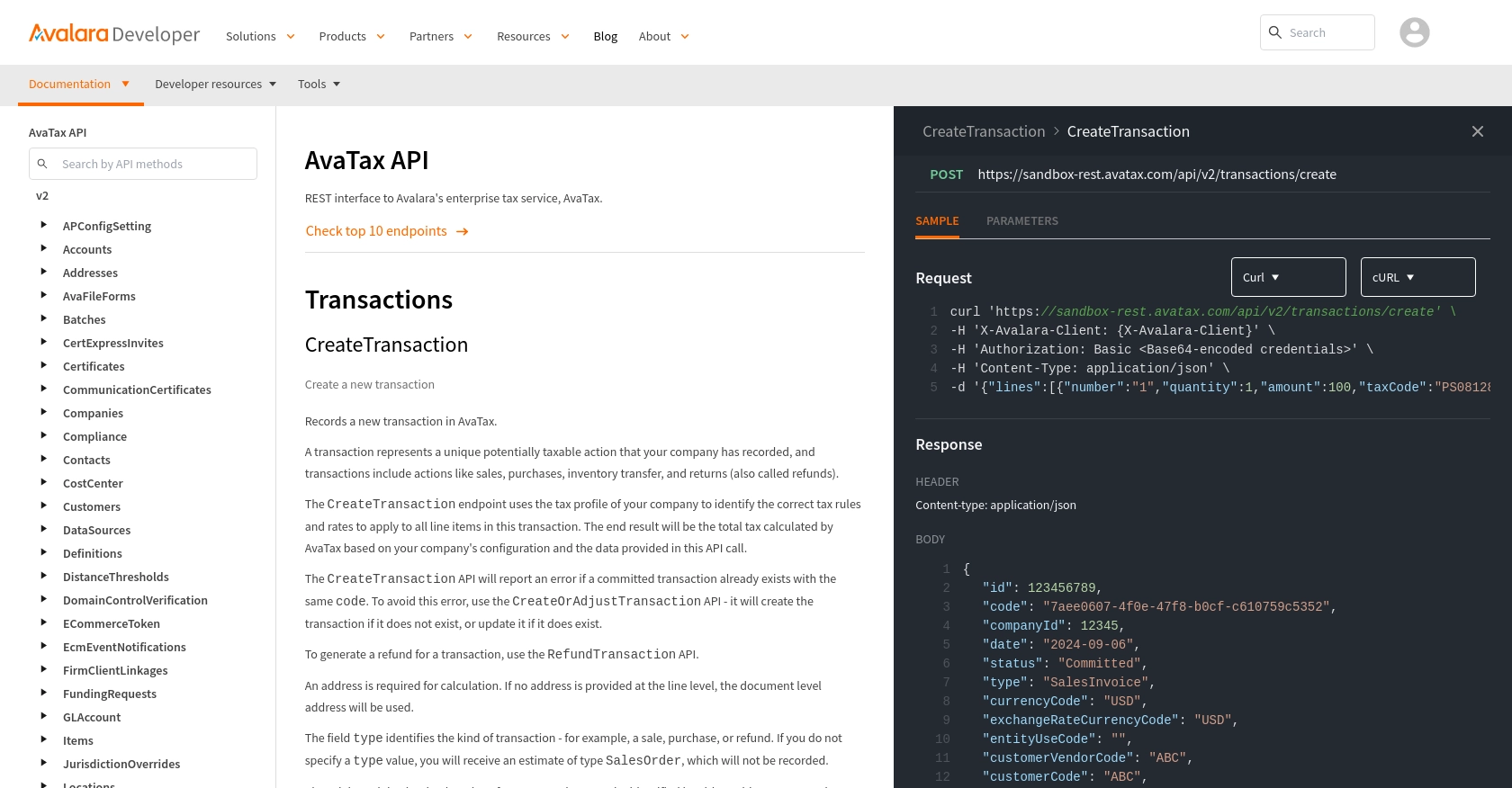
Best Practices for Using Avalara API in PHP
When integrating with the Avalara API, it's crucial to follow best practices to ensure security, efficiency, and reliability. Here are some recommendations:
- Secure Storage of Credentials: Always store your Avalara account ID and license key securely. Use environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Avalara may impose rate limits on API requests. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that the data you send to Avalara is correctly formatted and standardized to avoid errors and ensure accurate tax calculations.
- Error Handling: Implement robust error handling to manage API errors effectively. Log errors for troubleshooting and provide meaningful feedback to users.
Streamlining Integration with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development.
With Endgrate, you can:
- Save time and resources by outsourcing integration tasks.
- Build once for each use case and apply it across multiple integrations.
- Provide an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
Ready to get started?