Using the CSV API to Import Data from CSV in Javascript
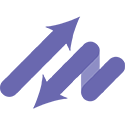
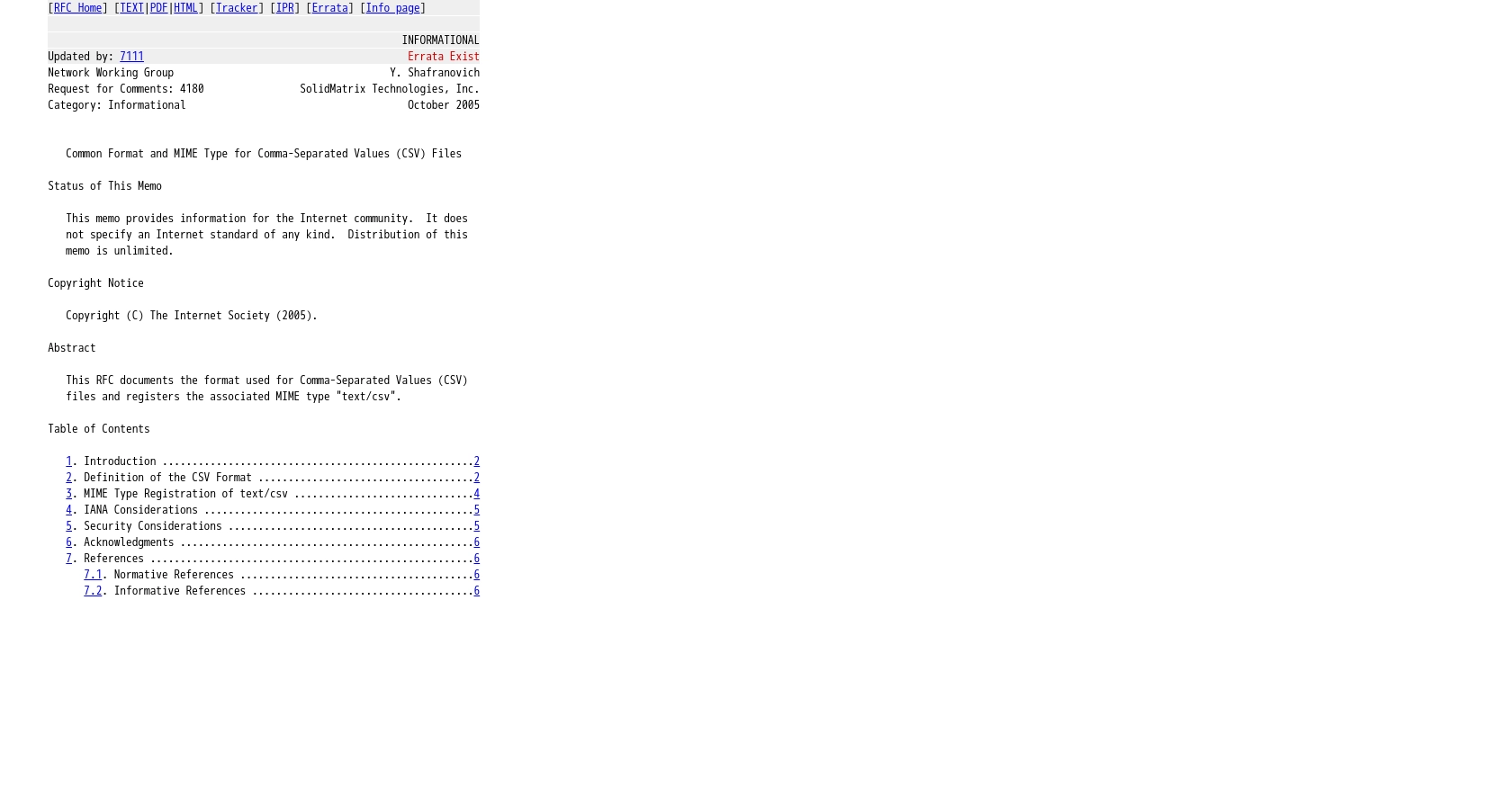
Introduction to CSV Integration for Data Import
CSV (Comma-Separated Values) files are a widely used format for storing and exchanging data due to their simplicity and compatibility with various applications. Many businesses rely on CSV files to manage and transfer data between systems, making CSV integration a crucial aspect of modern software development.
Integrating with a CSV API allows developers to automate the import and export of data from CSV files, streamlining processes and reducing manual effort. For example, a developer might use a CSV API to import customer data from a CSV file into a web application, enabling seamless data management and analysis.
This article will guide you through using JavaScript to interact with a CSV API, focusing on importing data from CSV files efficiently. You'll learn how to handle CSV data in chunks and ensure smooth data transfer, leveraging JavaScript's capabilities to enhance your integration workflows.
Setting Up Your CSV Integration Test Environment
Before you begin importing data using the CSV API in JavaScript, it's essential to set up a test environment to ensure smooth integration and data handling. This setup will allow you to experiment with CSV data importation without affecting your production environment.
Creating a CSV Test Account
To start, you'll need to create a test account or sandbox environment provided by your integration platform. This environment will enable you to upload and manage CSV files safely. Follow these steps to set up your test account:
- Visit the integration provider's website and sign up for a free trial or demo account.
- Once registered, log in to your account and navigate to the sandbox or test environment section.
- Ensure you have access to upload and download CSV files within this environment.
Configuring CSV API Authentication
The CSV API uses a custom integration approach, requiring specific configurations to authenticate and manage CSV data. Follow these steps to configure your authentication:
- Access the API settings in your test account dashboard.
- Locate the authentication section and follow the instructions to set up your API credentials.
- Ensure you have the necessary permissions to upload and download CSV files.
Preparing CSV Files for Testing
With your test environment ready, prepare sample CSV files to test the import process. Consider the following tips:
- Create CSV files with various data types and structures to test different scenarios.
- Ensure the CSV files are formatted correctly, with headers matching the expected data fields.
- Use a text editor or spreadsheet software to create and edit your CSV files.
By setting up a comprehensive test environment, you can confidently proceed with integrating the CSV API using JavaScript, ensuring efficient and error-free data importation.
Making API Calls to Import CSV Data Using JavaScript
JavaScript is a versatile language that allows developers to efficiently handle CSV data importation through API calls. In this section, we'll explore how to set up your JavaScript environment and execute API calls to import data from CSV files seamlessly.
Setting Up Your JavaScript Environment for CSV API Integration
Before making API calls, ensure your JavaScript environment is ready. Follow these steps to set up your environment:
- Ensure you have Node.js installed on your machine to run JavaScript code outside the browser.
- Use npm (Node Package Manager) to install necessary libraries, such as
csv-parser
for parsing CSV files. - Initialize your project with
npm init
and create apackage.json
file to manage dependencies.
Executing API Calls to Import CSV Data
Once your environment is set up, you can proceed with making API calls to import CSV data. Here's a step-by-step guide:
// Import required modules
const fs = require('fs');
const csv = require('csv-parser');
const axios = require('axios');
// Define the CSV file path and API endpoint
const csvFilePath = 'path/to/your/file.csv';
const apiEndpoint = 'https://api.yourintegrationprovider.com/upload';
// Function to read and upload CSV data
function uploadCSVData() {
const results = [];
// Read CSV file in chunks
fs.createReadStream(csvFilePath)
.pipe(csv())
.on('data', (data) => results.push(data))
.on('end', () => {
// Make API call to upload data
axios.post(apiEndpoint, results)
.then(response => {
console.log('Data uploaded successfully:', response.data);
})
.catch(error => {
console.error('Error uploading data:', error);
});
});
}
// Execute the function
uploadCSVData();
In the code above, we use the fs
module to read the CSV file and the csv-parser
library to parse it. The data is then sent to the API endpoint using axios
, a popular HTTP client for making requests.
Verifying Successful Data Importation
After executing the API call, verify the data importation by checking the test environment. Ensure that the uploaded data appears correctly in the system. If any errors occur, review the error messages and adjust your code or CSV file accordingly.
Handling Errors and Debugging API Calls
When working with API calls, it's crucial to handle potential errors gracefully. Consider the following tips:
- Implement error handling in your code to catch and log errors during the API call.
- Check the API documentation for specific error codes and their meanings.
- Use console logs to debug and trace issues in your code.
By following these steps, you can efficiently import CSV data using JavaScript and ensure a smooth integration process.
Conclusion and Best Practices for CSV API Integration with JavaScript
Integrating CSV APIs using JavaScript can significantly enhance your data management workflows by automating the import and export of CSV files. By following the steps outlined in this article, you can efficiently handle CSV data, ensuring smooth and error-free integration processes.
Best Practices for Secure and Efficient CSV API Integration
- Securely Store Credentials: Always store API credentials securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by the API provider. Implement retry logic and backoff strategies to handle rate limit errors gracefully.
- Data Validation and Transformation: Validate and transform CSV data before importing to ensure it matches the expected format and structure required by the API.
- Error Handling: Implement comprehensive error handling to catch and log errors during API calls, allowing for easier debugging and resolution.
Streamlining Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider using Endgrate to simplify the process. Endgrate allows you to build once for each use case, reducing the need for multiple integrations and enabling you to focus on your core product. With Endgrate, you can provide an intuitive integration experience for your customers, saving time and resources.
Visit Endgrate to learn more about how you can streamline your integration efforts and enhance your software development workflows.
Read More
Ready to get started?