Using the SMTP API to Send Messages in Javascript
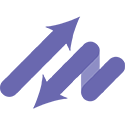
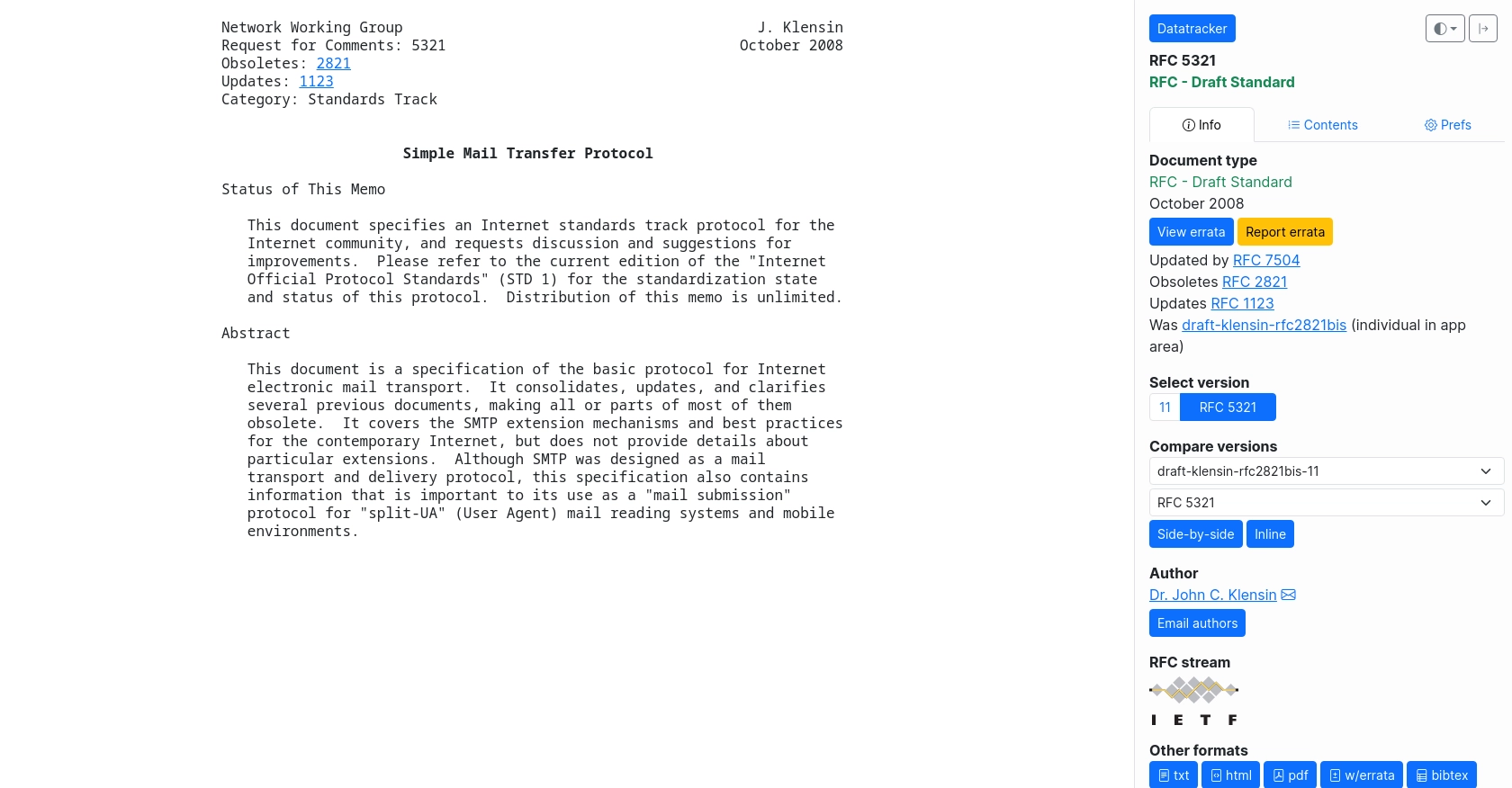
Introduction to SMTP and Its Role in Messaging
SMTP, or Simple Mail Transfer Protocol, is a fundamental protocol used for sending emails across the Internet. It serves as the backbone for email communication, enabling the transmission of messages from one server to another. SMTP is widely used by email clients and servers to deliver messages reliably and efficiently.
For developers, integrating with SMTP allows for programmatic control over email sending, which can be crucial for applications that require automated notifications, newsletters, or transactional emails. By leveraging SMTP in JavaScript, developers can build robust solutions for sending messages directly from their applications.
Consider a scenario where a developer needs to send confirmation emails to users after they sign up for a service. Using SMTP, the developer can automate this process, ensuring timely and consistent communication with users.
Setting Up Your SMTP Test Environment for JavaScript Integration
Before you can start sending messages using SMTP in JavaScript, it's essential to set up a test environment. This will allow you to safely experiment with sending emails without affecting your production environment. Here's how you can get started:
Create a Test Email Account
To begin, you'll need a test email account. Many email service providers offer free accounts that you can use for testing purposes. Ensure that the account you choose supports SMTP access, as this is crucial for integration.
Configure SMTP Settings
Once you have your test email account, you'll need to configure the SMTP settings. These settings typically include:
- SMTP Server: The address of the SMTP server provided by your email service.
- Port: Commonly used ports are 587 for TLS/STARTTLS and 465 for SSL.
- Username: Your email address or a specific username provided by the service.
- Password: The password associated with your email account.
Utilize SMTP Libraries in JavaScript
To interact with the SMTP server, you'll need to use JavaScript libraries that support SMTP. Popular libraries include Nodemailer, which simplifies the process of sending emails from your application.
const nodemailer = require('nodemailer');
// Create a transporter object using SMTP settings
let transporter = nodemailer.createTransport({
host: 'smtp.example.com',
port: 587,
secure: false, // true for 465, false for other ports
auth: {
user: 'your-email@example.com',
pass: 'your-email-password'
}
});
Test Your SMTP Configuration
Before proceeding to send actual emails, it's a good practice to test your SMTP configuration. You can do this by sending a test email to ensure that your settings are correct and that the email is delivered successfully.
transporter.sendMail({
from: '"Test Sender" <your-email@example.com>',
to: 'recipient@example.com',
subject: 'Test Email',
text: 'This is a test email sent using SMTP in JavaScript.'
}, (error, info) => {
if (error) {
return console.log('Error occurred: ', error);
}
console.log('Email sent: ', info.response);
});
By following these steps, you can set up a reliable test environment for sending messages using SMTP in JavaScript, ensuring that your integration is both functional and secure.
Executing SMTP API Calls in JavaScript: A Step-by-Step Guide
To effectively send messages using the SMTP API in JavaScript, it's crucial to understand the necessary steps and configurations. This section will guide you through the process, ensuring you can seamlessly integrate SMTP into your JavaScript applications.
Prerequisites for SMTP Integration in JavaScript
Before diving into the code, ensure you have the following prerequisites set up:
- Node.js: Ensure you have Node.js installed on your machine, as it provides the runtime environment for executing JavaScript outside the browser.
- Nodemailer: This is a popular library for sending emails via SMTP in Node.js. Install it using npm:
npm install nodemailer
Setting Up SMTP Transporter in JavaScript
With Nodemailer installed, the next step is to set up the SMTP transporter. This object will handle the connection to the SMTP server and facilitate email sending.
const nodemailer = require('nodemailer');
// Configure the SMTP transporter
let transporter = nodemailer.createTransport({
host: 'smtp.example.com',
port: 587,
secure: false, // Use true for port 465
auth: {
user: 'your-email@example.com',
pass: 'your-email-password'
}
});
Replace smtp.example.com
, your-email@example.com
, and your-email-password
with your SMTP server details and credentials.
Sending Emails Using SMTP in JavaScript
Once the transporter is configured, you can proceed to send emails. Here's how you can send a basic email using the SMTP API:
transporter.sendMail({
from: '"Sender Name" <your-email@example.com>',
to: 'recipient@example.com',
subject: 'Hello from SMTP',
text: 'This is a message sent using the SMTP API in JavaScript.',
html: '<b>This is a message sent using the SMTP API in JavaScript.</b>'
}, (error, info) => {
if (error) {
return console.log('Error occurred: ', error);
}
console.log('Email sent: ', info.response);
});
This code sends an email with both plain text and HTML content. Adjust the from
, to
, subject
, text
, and html
fields as needed for your application.
Verifying Successful SMTP API Calls
After executing the email sending function, you should verify that the email was sent successfully. Check the console output for the success message or any errors. Additionally, confirm the email's delivery by checking the recipient's inbox.
Error Handling and Troubleshooting SMTP API Calls
Handling errors is crucial for a robust SMTP integration. Common errors include authentication failures, network issues, and incorrect SMTP settings. Ensure you log errors and handle them gracefully in your application:
if (error) {
console.error('Error occurred: ', error.message);
// Implement additional error handling logic here
}
By following these steps, you can effectively send messages using the SMTP API in JavaScript, ensuring reliable and efficient email communication within your applications.
Conclusion and Best Practices for SMTP Integration in JavaScript
Integrating SMTP for sending messages in JavaScript offers a powerful way to automate email communication within your applications. By following the steps outlined in this guide, you can establish a reliable and efficient email-sending process using SMTP.
Best Practices for Secure and Efficient SMTP Integration
- Secure Credentials: Always store your SMTP credentials securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Be aware of any rate limits imposed by your SMTP provider. Implement logic to handle rate limiting gracefully to avoid disruptions in email delivery.
- Data Standardization: Ensure that email content is standardized and formatted correctly to maintain consistency and professionalism in communication.
- Error Logging: Implement comprehensive error logging to quickly identify and resolve issues related to SMTP integration.
Enhancing Integration Capabilities with Endgrate
While integrating SMTP directly in JavaScript is effective, managing multiple integrations can become complex. Endgrate offers a streamlined solution by providing a unified API for various platforms, including SMTP. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can simplify your integration processes and provide an intuitive experience for your customers. Visit Endgrate to learn more about how you can optimize your integration strategy.
Read More
Ready to get started?