Using the Front API to Get Conversations (with Javascript examples)
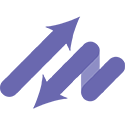
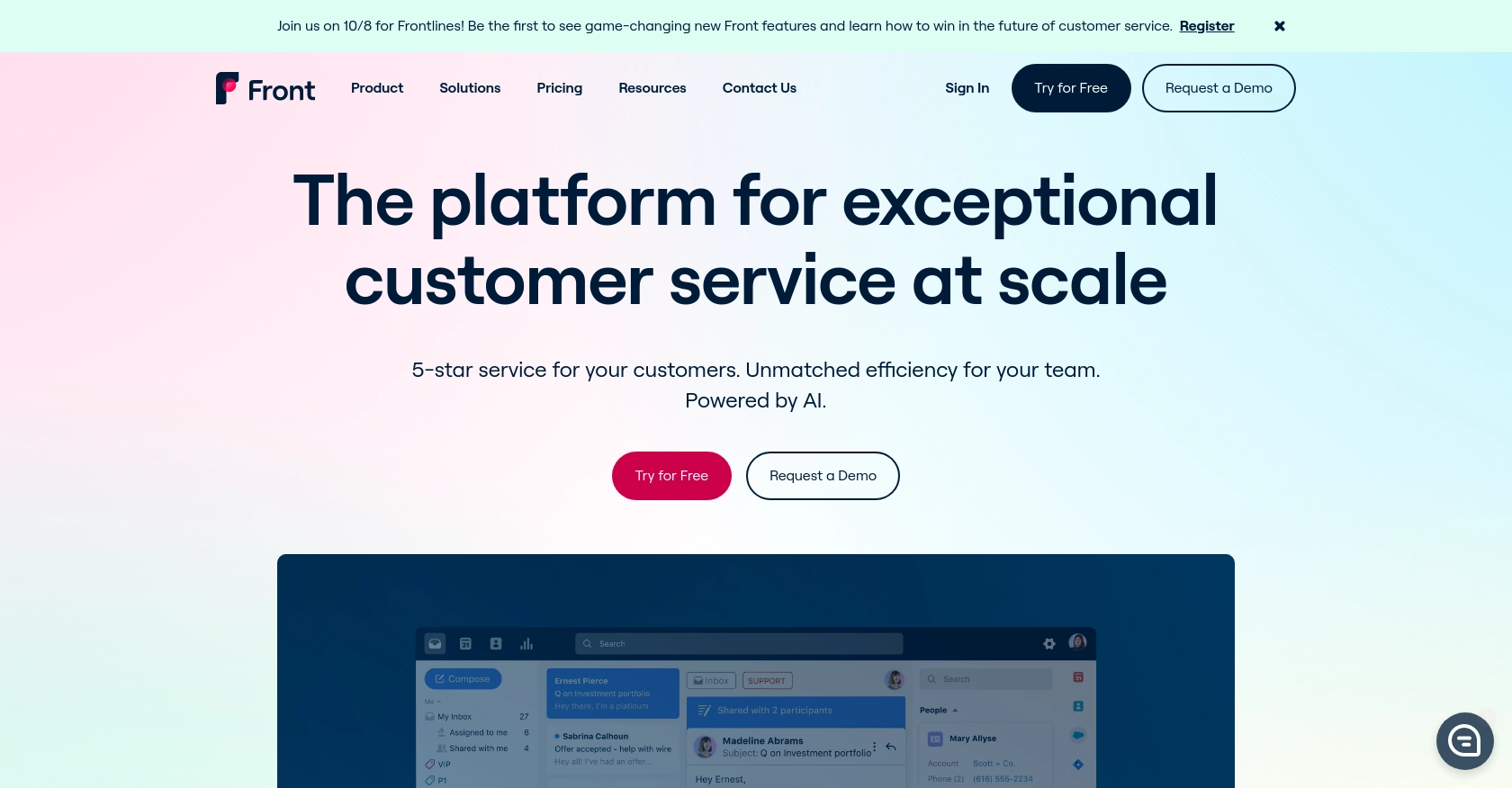
Introduction to Front API for Conversations
Front is a powerful communication platform that centralizes emails, messages, and other communication channels into a single collaborative interface. It is widely used by businesses to streamline customer interactions and enhance team collaboration.
Developers may want to integrate with Front's API to automate and manage conversations efficiently. For example, using the Front API, a developer can retrieve conversation data to analyze customer interactions or integrate with other systems for enhanced workflow automation.
This article will guide you through using JavaScript to interact with the Front API, specifically focusing on retrieving conversations. You'll learn how to set up your environment, authenticate requests, and handle API responses effectively.
Setting Up Your Front Developer Account and API Access
Before you can start interacting with the Front API to retrieve conversations, you'll need to set up a developer account and obtain an API token. This section will guide you through the process of creating a test environment and generating the necessary credentials.
Create a Front Developer Account
If you don't already have a Front account, you can sign up for a free developer account. This account provides a sandbox environment where you can safely test your integrations without affecting production data.
- Visit the Front Developer Portal and click on the sign-up link.
- Follow the instructions to create your account. Once registered, you'll have access to the developer environment.
Generate an API Token for Front API Access
Front uses API tokens for authentication, allowing you to make secure requests to their API. Follow these steps to generate your API token:
- Log in to your Front developer account.
- Navigate to the API tokens section in the dashboard.
- Click on "Create API Token" and select the appropriate scopes for your integration. For retrieving conversations, ensure you have the necessary read permissions.
- Copy the generated API token and store it securely, as you'll need it to authenticate your API requests.
With your developer account set up and your API token ready, you're now prepared to start making requests to the Front API. In the next section, we'll explore how to use JavaScript to interact with the API and retrieve conversation data.
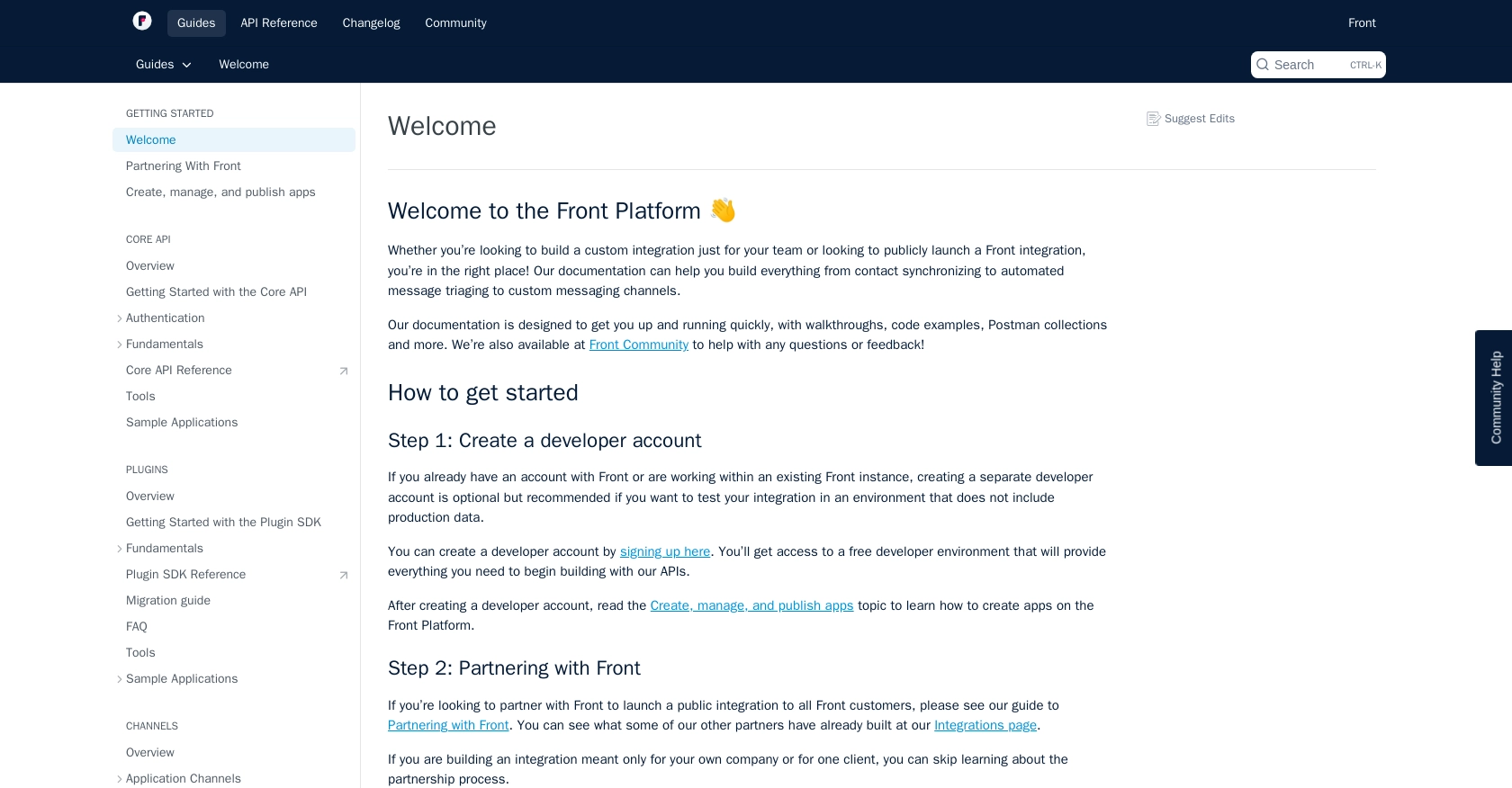
sbb-itb-96038d7
Making API Calls to Retrieve Conversations Using Front API with JavaScript
To interact with the Front API and retrieve conversations, you'll need to set up your JavaScript environment and make HTTP requests using the API token you generated. This section will guide you through the necessary steps to make successful API calls and handle responses effectively.
Setting Up Your JavaScript Environment for Front API Integration
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine (version 14 or higher recommended).
- A code editor like Visual Studio Code for writing and running JavaScript code.
- The
axios
library for making HTTP requests. You can install it using npm:
npm install axios
Example Code to Retrieve Conversations from Front API
With your environment ready, you can now write JavaScript code to retrieve conversations from the Front API. Here's a step-by-step example:
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://api2.frontapp.com/conversations';
const headers = {
'Authorization': 'Bearer YOUR_API_TOKEN',
'Accept': 'application/json'
};
// Function to get conversations
async function getConversations() {
try {
const response = await axios.get(endpoint, { headers });
const conversations = response.data._results;
console.log('Conversations:', conversations);
} catch (error) {
console.error('Error fetching conversations:', error.response ? error.response.data : error.message);
}
}
// Call the function
getConversations();
Replace YOUR_API_TOKEN
with the API token you generated earlier. This code uses the axios
library to send a GET request to the Front API's conversations endpoint. If successful, it logs the retrieved conversations to the console.
Handling API Responses and Errors
After making the API call, it's crucial to handle responses and potential errors effectively:
- Check the response status code to ensure the request was successful (status code 200).
- Log any errors to understand what went wrong. Common error codes include 401 (Unauthorized) and 429 (Too Many Requests).
- Refer to the Front API documentation for detailed information on error codes and handling.
Verifying API Call Success in Front Sandbox Environment
To verify that your API call succeeded, you can check the sandbox environment in your Front developer account:
- Log in to your Front developer account and navigate to the Conversations section.
- Ensure the conversations retrieved by your API call match those displayed in the sandbox.
By following these steps, you can efficiently retrieve and manage conversations using the Front API with JavaScript. In the next section, we'll explore best practices for handling API rate limits and optimizing your integration.
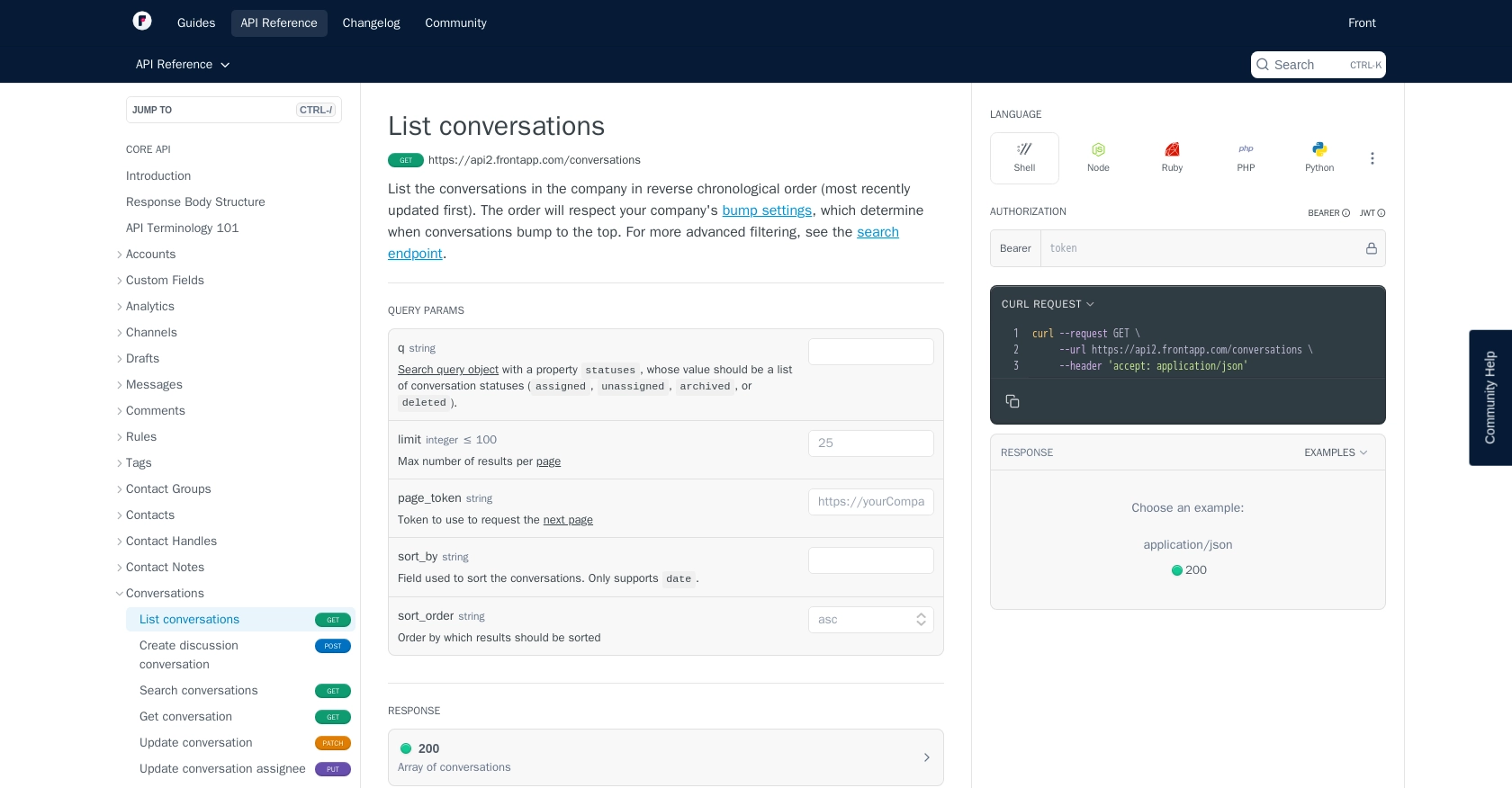
Conclusion and Best Practices for Using Front API with JavaScript
Integrating with the Front API to retrieve conversations using JavaScript can significantly enhance your application's capabilities by automating communication workflows and improving data management. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate requests, and handle API responses.
Best Practices for Secure and Efficient Front API Integration
- Securely Store API Tokens: Always store your API tokens securely and avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of Front's rate limits, which start at 50 requests per minute. Implement logic to handle the
429 Too Many Requests
error by respecting theRetry-After
header. For more details, refer to the Front API rate limiting documentation. - Implement Pagination: When retrieving large datasets, use pagination to manage the data efficiently. Refer to the Front API pagination documentation for guidance.
- Standardize Data Fields: Ensure that data fields retrieved from the API are standardized to match your application's data structure, facilitating seamless integration with other systems.
Enhance Your Integration Experience with Endgrate
While integrating with Front API can be straightforward, managing multiple integrations across different platforms can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes, allowing you to focus on your core product development.
With Endgrate, you can:
- Save time and resources by outsourcing integration management.
- Build once for each use case and apply it across multiple integrations.
- Provide an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate today.
Read More
- https://endgrate.com/provider/front
- https://dev.frontapp.com/docs/welcome
- https://dev.frontapp.com/docs/core-api-getting-started
- https://dev.frontapp.com/docs/oauth
- https://dev.frontapp.com/docs/rate-limiting
- https://dev.frontapp.com/docs/pagination
- https://dev.frontapp.com/reference/list-conversations
Ready to get started?