Using the Younium API to Create or Update Sales Orders (with PHP examples)
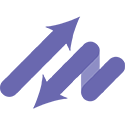
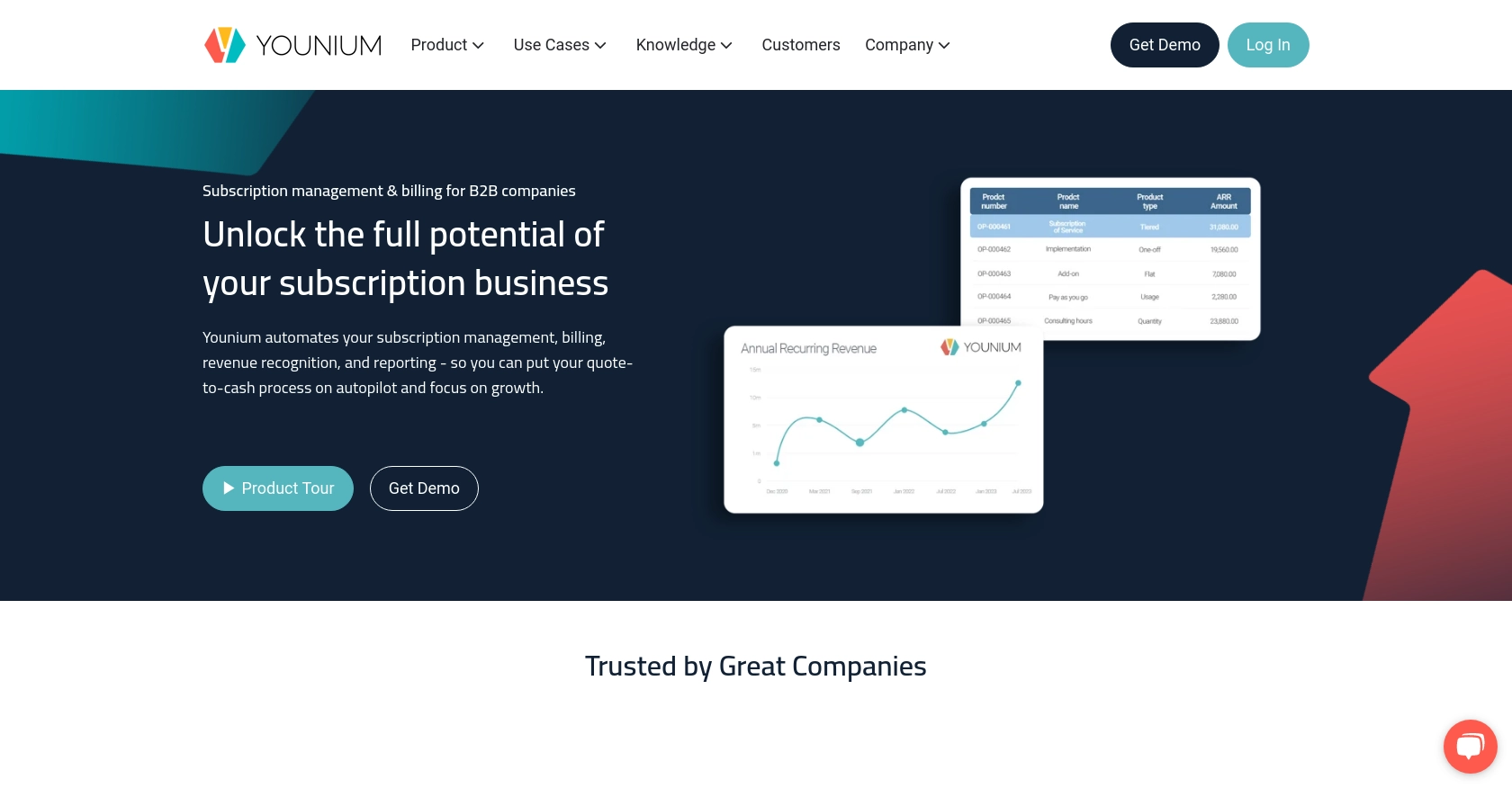
Introduction to Younium API for Sales Order Management
Younium is a comprehensive subscription management platform tailored for B2B companies, offering robust tools for handling billing, revenue recognition, and financial reporting. Its API provides developers with the flexibility to automate and streamline various business processes, including sales order management.
Integrating with the Younium API allows developers to efficiently create or update sales orders, ensuring seamless synchronization between their applications and Younium's platform. For example, a developer might automate the process of updating sales orders from an external CRM system, reducing manual data entry and minimizing errors.
Setting Up Your Younium Test/Sandbox Account for API Integration
Before you can start creating or updating sales orders using the Younium API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your production data.
Creating a Younium Sandbox Account
To begin, you'll need to create a sandbox account on the Younium platform. Follow these steps:
- Visit the Younium Developer Portal.
- Sign up for a sandbox account by following the on-screen instructions.
- Once your account is set up, log in to access the sandbox environment.
Generating API Tokens and Client Credentials
To authenticate your API requests, you'll need to generate an API token and client credentials. Here's how:
- Click on your name in the top right corner to open the user profile menu and select "Privacy & Security".
- Navigate to "Personal Tokens" and click "Generate Token".
- Provide a relevant description for your token and click "Create".
- Copy the generated Client ID and Secret Key. These credentials are crucial for generating your JWT access token and will not be visible again.
Acquiring a JWT Access Token
With your client credentials ready, you can now acquire a JWT access token to authenticate your API requests:
// Set up the request to acquire a JWT token
$url = 'https://api.sandbox.younium.com/auth/token';
$headers = ['Content-Type: application/json'];
$body = json_encode([
'clientId' => 'Your_Client_ID',
'secret' => 'Your_Secret_Key'
]);
// Use cURL to make the POST request
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $body);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
// Decode the response to get the access token
$data = json_decode($response, true);
$accessToken = $data['accessToken'];
Replace Your_Client_ID
and Your_Secret_Key
with the credentials you generated earlier. The access token will be valid for 24 hours, after which you'll need to request a new one.
Handling Authentication Errors
If you encounter errors during authentication, refer to the following common error codes:
- 400 Bad Request: Check your request body for any missing or incorrect fields.
- 401 Unauthorized: Ensure your access token is correct and not expired.
- 403 Forbidden: Verify that the legal entity specified is valid and that your user has the necessary permissions.
For more details, visit the Younium Authentication Documentation.
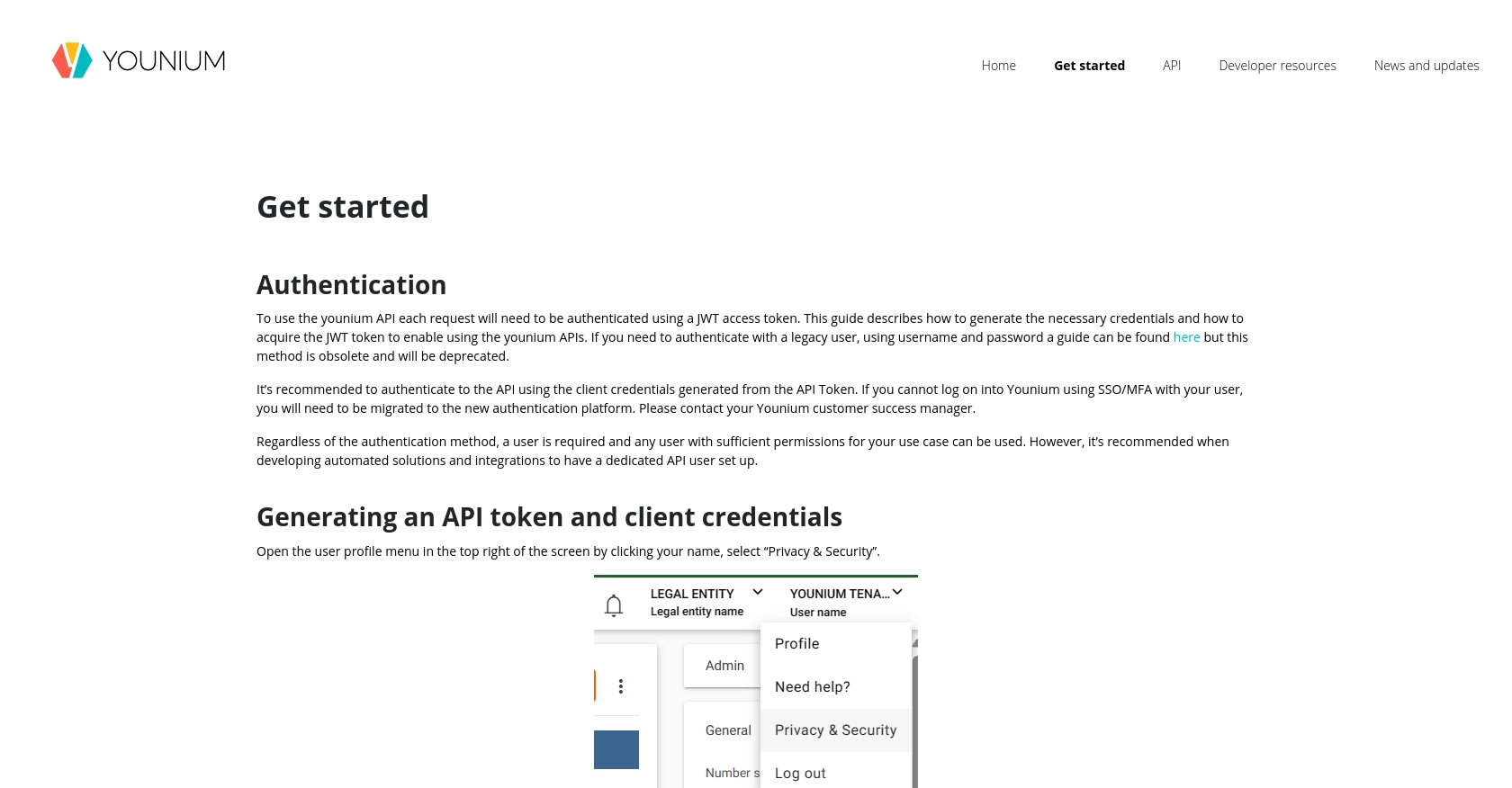
sbb-itb-96038d7
Making API Calls to Younium for Sales Order Management Using PHP
Once you have set up your Younium sandbox account and acquired a JWT access token, you can proceed to create or update sales orders using the Younium API. This section will guide you through the process of making authenticated API calls with PHP to manage sales orders effectively.
Setting Up Your PHP Environment for Younium API Integration
Before making API calls, ensure your PHP environment is properly configured. You will need:
- PHP 7.4 or later
- cURL extension enabled
Verify your PHP version and ensure cURL is installed by running the following commands:
php -v
php -m | grep curl
Creating a Sales Order with Younium API
To create a sales order, you will need to send a POST request to the Younium API endpoint. Below is a sample PHP script to create a sales order:
// Define the API endpoint and headers
$url = 'https://api.sandbox.younium.com/salesorders';
$headers = [
'Content-Type: application/json',
'Authorization: Bearer ' . $accessToken,
'api-version: 2.1',
'legal-entity: Your_Legal_Entity'
];
// Define the sales order data
$salesOrderData = json_encode([
'customerId' => '12345',
'orderDate' => '2023-10-01',
'items' => [
[
'productId' => '67890',
'quantity' => 10,
'price' => 100
]
]
]);
// Initialize cURL session
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $salesOrderData);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
curl_close($ch);
// Decode and handle the response
$responseData = json_decode($response, true);
if (isset($responseData['id'])) {
echo "Sales Order Created Successfully. ID: " . $responseData['id'];
} else {
echo "Failed to Create Sales Order. Error: " . $responseData['message'];
}
Replace Your_Legal_Entity
with your actual legal entity ID or name. Ensure the customerId
and productId
are valid within your Younium account.
Updating a Sales Order with Younium API
To update an existing sales order, use a PUT request. Here's how you can update a sales order:
// Define the API endpoint for updating a sales order
$url = 'https://api.sandbox.younium.com/salesorders/{orderId}'; // Replace {orderId} with the actual order ID
$headers = [
'Content-Type: application/json',
'Authorization: Bearer ' . $accessToken,
'api-version: 2.1',
'legal-entity: Your_Legal_Entity'
];
// Define the updated sales order data
$updateData = json_encode([
'items' => [
[
'productId' => '67890',
'quantity' => 15, // Updated quantity
'price' => 95 // Updated price
]
]
]);
// Initialize cURL session
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, 'PUT');
curl_setopt($ch, CURLOPT_POSTFIELDS, $updateData);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
curl_close($ch);
// Decode and handle the response
$responseData = json_decode($response, true);
if (isset($responseData['id'])) {
echo "Sales Order Updated Successfully. ID: " . $responseData['id'];
} else {
echo "Failed to Update Sales Order. Error: " . $responseData['message'];
}
Ensure you replace {orderId}
with the actual ID of the sales order you wish to update.
Verifying API Call Success in Younium Sandbox
After executing the API calls, verify the success by checking the sales orders in your Younium sandbox account. Navigate to the sales orders section to confirm the creation or update of the orders.
Handling Common API Errors
While making API calls, you might encounter errors. Here are some common error codes and their meanings:
- 400 Bad Request: The request body may have invalid or missing fields.
- 401 Unauthorized: The access token might be expired or incorrect.
- 403 Forbidden: The specified legal entity might be invalid, or the user lacks necessary permissions.
For detailed error handling, refer to the Younium API Documentation.
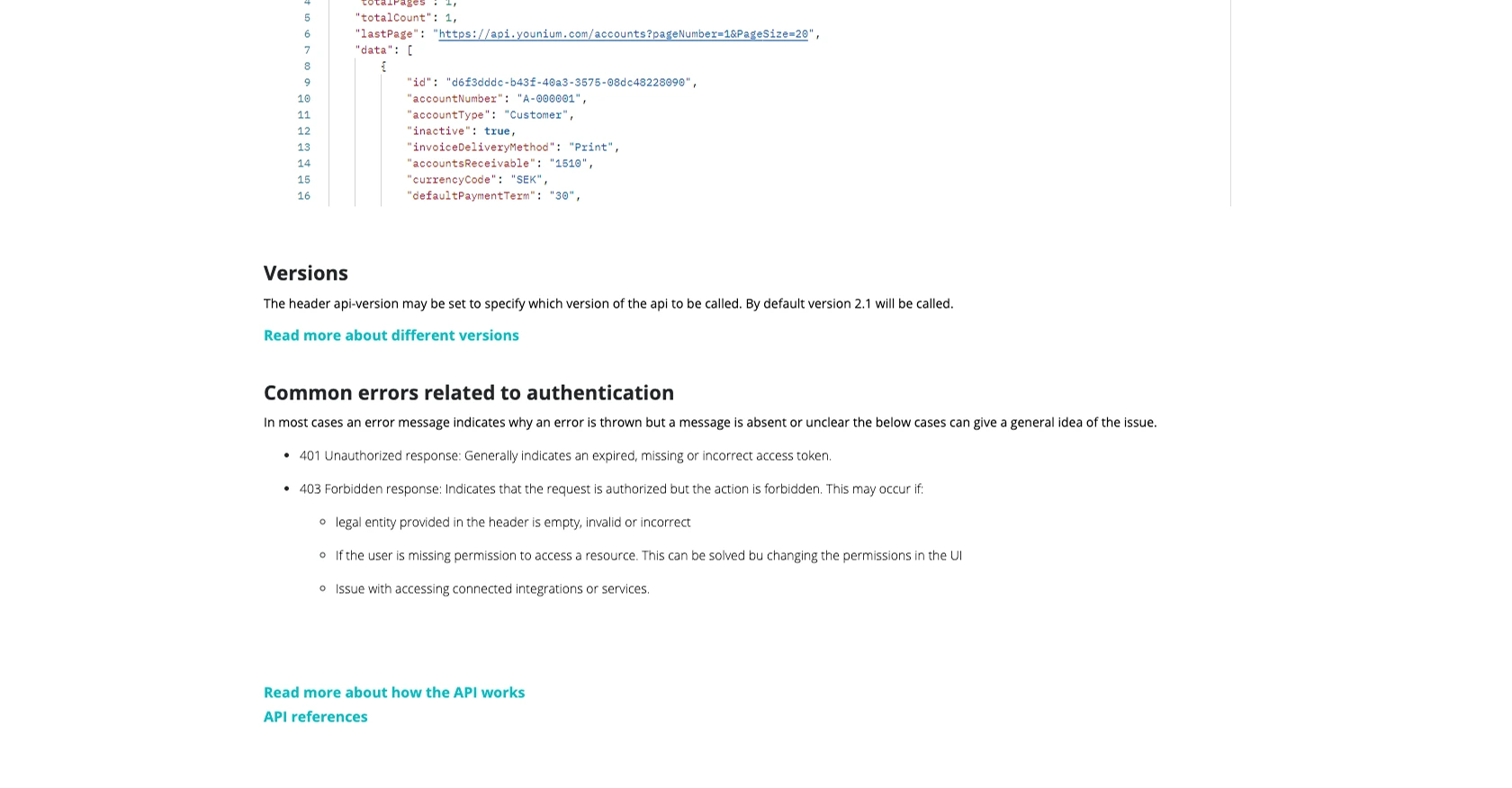
Conclusion and Best Practices for Younium API Integration
Integrating with the Younium API for sales order management can significantly enhance your business processes by automating and streamlining operations. By following the steps outlined in this guide, you can efficiently create and update sales orders using PHP, ensuring seamless synchronization with Younium's platform.
Best Practices for Secure and Efficient Younium API Usage
- Secure Storage of Credentials: Always store your API credentials, such as Client ID and Secret Key, securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handling Rate Limits: Be mindful of the API's rate limits to avoid throttling. Implement exponential backoff strategies to handle rate limit errors gracefully.
- Data Standardization: Ensure that data fields are standardized and validated before making API calls to prevent errors and maintain data integrity.
- Regular Token Refresh: Since the JWT access token is valid for 24 hours, implement a mechanism to refresh the token automatically to maintain uninterrupted API access.
Enhancing Integration Capabilities with Endgrate
While integrating with Younium's API can be highly beneficial, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including Younium.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. With Endgrate, you build once for each use case, reducing the need for multiple integrations and providing an intuitive experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website and discover the benefits of a unified integration approach.
Read More
Ready to get started?