Using the FreeAgent API to Create or Update Contacts in Python
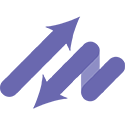
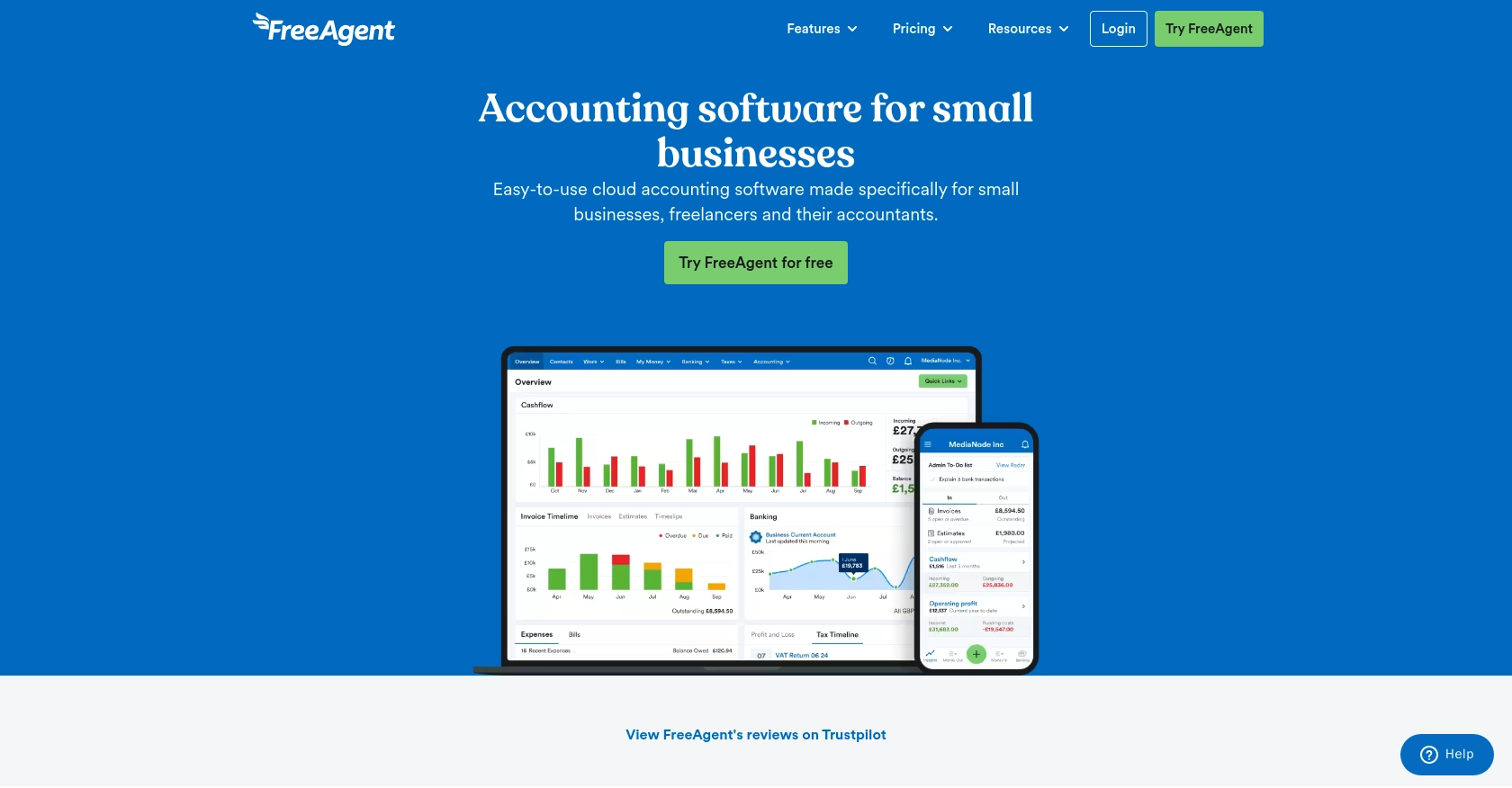
Introduction to FreeAgent API Integration
FreeAgent is a comprehensive accounting software platform designed to simplify financial management for small businesses and freelancers. It offers a wide range of features, including invoicing, expense tracking, and project management, making it a popular choice for businesses looking to streamline their accounting processes.
Integrating with the FreeAgent API allows developers to automate and enhance financial workflows. For example, you can use the API to create or update contact information directly from your application, ensuring that your client database is always up-to-date and accurate.
This article will guide you through the process of using Python to interact with the FreeAgent API, specifically focusing on creating and updating contacts. By following these steps, you can efficiently manage your contacts within the FreeAgent platform, saving time and reducing manual data entry.
Setting Up Your FreeAgent Sandbox Account for API Integration
Before you can start integrating with the FreeAgent API, you'll need to set up a sandbox account. This allows you to test your API interactions without affecting live data. Follow these steps to create a sandbox account and configure OAuth authentication.
Step 1: Create a FreeAgent Sandbox Account
To begin, you'll need a FreeAgent sandbox account. This account will serve as your testing environment for API interactions.
- Visit the FreeAgent Sandbox page.
- Sign up for a free temporary user account.
- Complete the setup stages for your company. This step is crucial to avoid unexpected errors when using the API.
Step 2: Create an App in the FreeAgent Developer Dashboard
Next, you'll need to create an app in the FreeAgent Developer Dashboard to obtain your OAuth credentials.
- Log in to the FreeAgent Developer Dashboard.
- Create a new app and take note of the OAuth Client ID and Client Secret.
Step 3: Configure OAuth 2.0 Playground
Use the Google OAuth 2.0 Playground to generate access and refresh tokens for your app.
- Go to the Google OAuth 2.0 Playground.
- Enter the OAuth Client ID and Client Secret from your FreeAgent app.
- Set the OAuth Authorization Endpoint to
https://api.sandbox.freeagent.com/v2/approve_app
. - Set the OAuth Token Endpoint to
https://api.sandbox.freeagent.com/v2/token_endpoint
.
Step 4: Authorize API Usage and Generate Tokens
Finally, authorize the API usage and generate the necessary tokens.
- Specify a scope in the OAuth Playground and click "Authorize APIs".
- Log in to your FreeAgent sandbox account and approve the app.
- Click "Exchange Authorization Code for Tokens" to generate access and refresh tokens.
With these tokens, you can now access the FreeAgent API in your sandbox environment. For more details, refer to the FreeAgent API documentation.
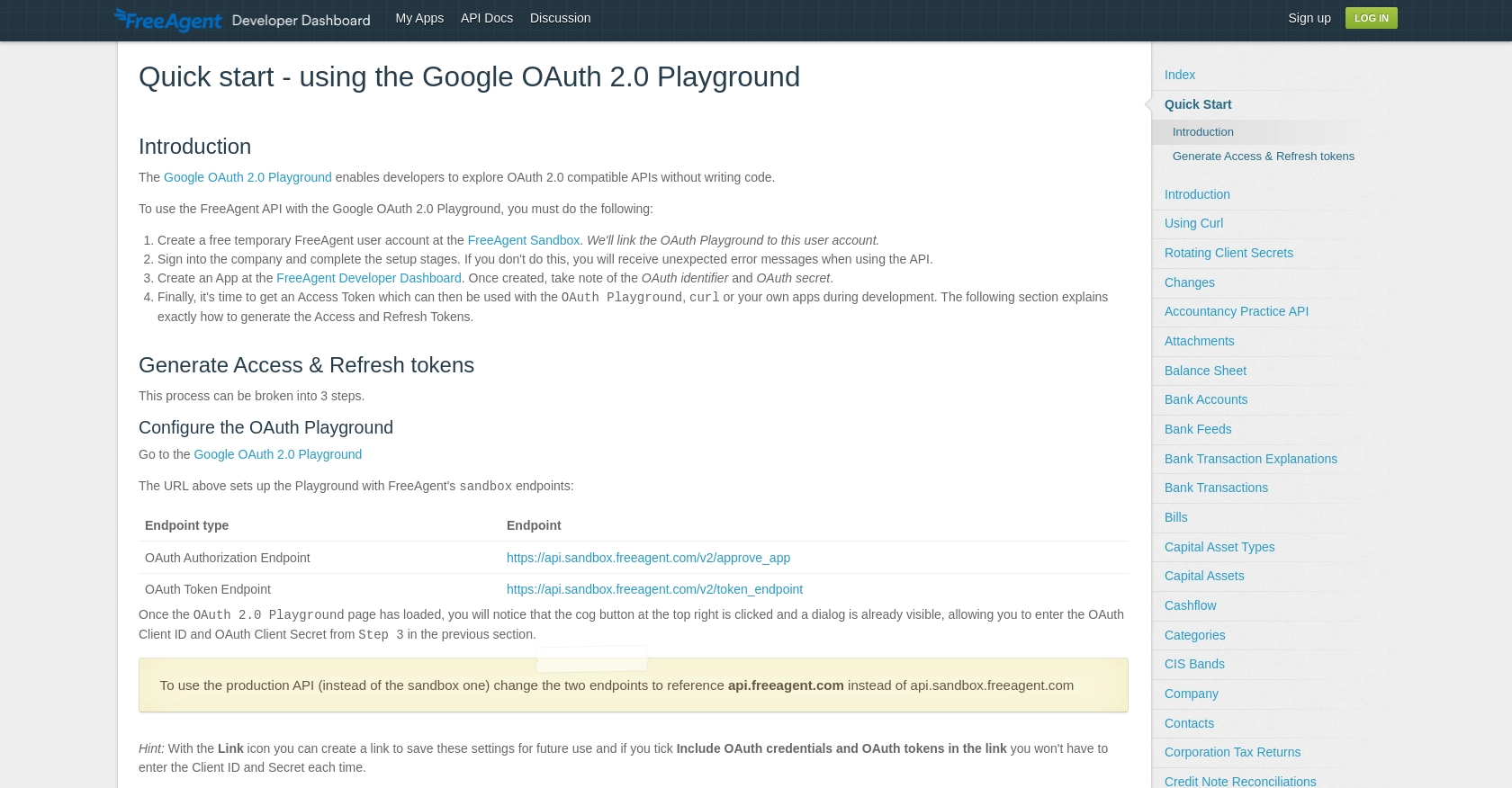
sbb-itb-96038d7
Making API Calls to FreeAgent for Contact Management Using Python
In this section, we'll explore how to interact with the FreeAgent API using Python to create or update contact information. This process involves setting up your development environment, writing the necessary code, and handling potential errors effectively.
Setting Up Your Python Environment for FreeAgent API Integration
Before making API calls, ensure your Python environment is correctly configured. You'll need Python 3.11.1 and the requests
library to handle HTTP requests.
- Ensure Python 3.11.1 is installed on your machine.
- Install the
requests
library using pip:
pip install requests
Creating a Contact in FreeAgent Using Python
To create a new contact in FreeAgent, you'll need to make a POST request to the FreeAgent API endpoint with the contact details. Here's a step-by-step guide:
import requests
# Set the API endpoint
url = "https://api.sandbox.freeagent.com/v2/contacts"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the contact information
contact_data = {
"contact": {
"first_name": "John",
"last_name": "Doe",
"email": "john.doe@example.com",
"organisation_name": "Example Corp"
}
}
# Make the POST request to create the contact
response = requests.post(url, json=contact_data, headers=headers)
# Check if the contact was created successfully
if response.status_code == 201:
print("Contact created successfully:", response.json())
else:
print("Failed to create contact:", response.status_code, response.text)
Replace Your_Access_Token
with the access token you obtained earlier. This code sends a POST request to the FreeAgent API to create a new contact. If successful, it will return the contact details.
Updating an Existing Contact in FreeAgent Using Python
To update an existing contact, use a PUT request with the contact's ID and the updated information:
import requests
# Set the API endpoint with the contact ID
contact_id = "2" # Example contact ID
url = f"https://api.sandbox.freeagent.com/v2/contacts/{contact_id}"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the updated contact information
updated_contact_data = {
"contact": {
"email": "new.email@example.com"
}
}
# Make the PUT request to update the contact
response = requests.put(url, json=updated_contact_data, headers=headers)
# Check if the contact was updated successfully
if response.status_code == 200:
print("Contact updated successfully:", response.json())
else:
print("Failed to update contact:", response.status_code, response.text)
This code updates the email address of an existing contact. Ensure you replace Your_Access_Token
and contact_id
with the appropriate values.
Handling Errors and Verifying API Requests
When interacting with the FreeAgent API, it's crucial to handle errors gracefully. Common HTTP status codes include:
- 201 Created: The contact was created successfully.
- 200 OK: The contact was updated successfully.
- 400 Bad Request: The request was malformed.
- 401 Unauthorized: Authentication failed. Check your access token.
- 429 Too Many Requests: Rate limit exceeded. Implement a back-off strategy.
For more information on handling errors, refer to the FreeAgent API documentation.
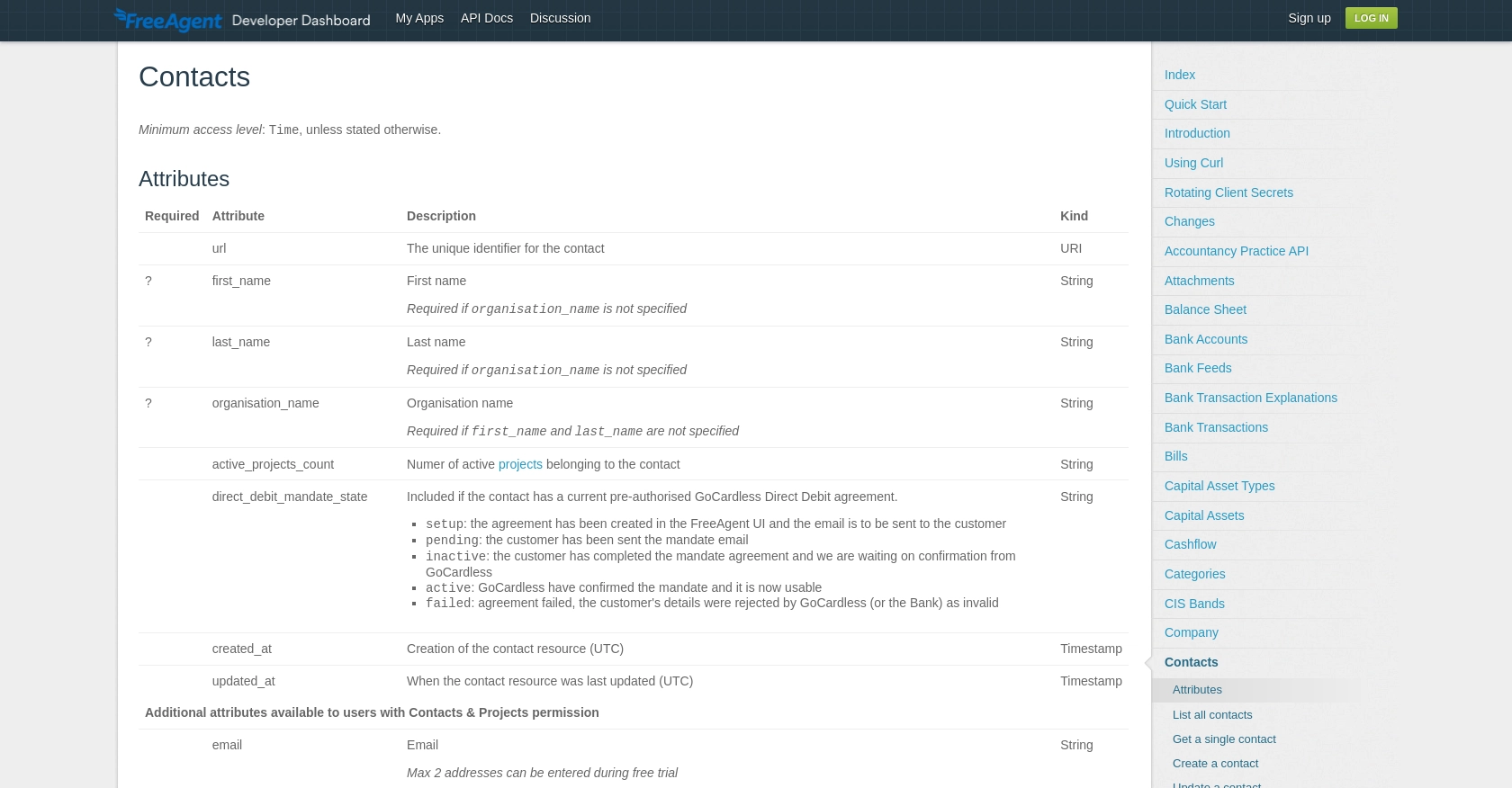
Conclusion and Best Practices for FreeAgent API Integration
Integrating with the FreeAgent API using Python provides a powerful way to automate and streamline contact management within your application. By following the steps outlined in this guide, you can efficiently create and update contacts, ensuring your client database remains accurate and up-to-date.
Best Practices for Secure and Efficient FreeAgent API Usage
- Securely Store Credentials: Always store your OAuth credentials and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of FreeAgent's rate limits, which are 120 requests per minute and 3600 requests per hour. Implement a back-off strategy to handle the
429 Too Many Requests
error gracefully. For more details, refer to the FreeAgent API documentation. - Validate API Responses: Always check the status codes of API responses to ensure successful operations. Handle errors appropriately to improve the reliability of your integration.
- Standardize Data Fields: Ensure that the data you send to FreeAgent is consistent and follows the required format. This helps in maintaining data integrity across your systems.
Enhance Your Integration Strategy with Endgrate
While integrating with FreeAgent can significantly improve your financial workflows, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including FreeAgent.
By leveraging Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers, enhancing user satisfaction.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate today.
Read More
Ready to get started?