Using the Fullstory API to Get Page Analytics in Javascript
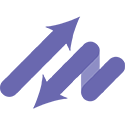
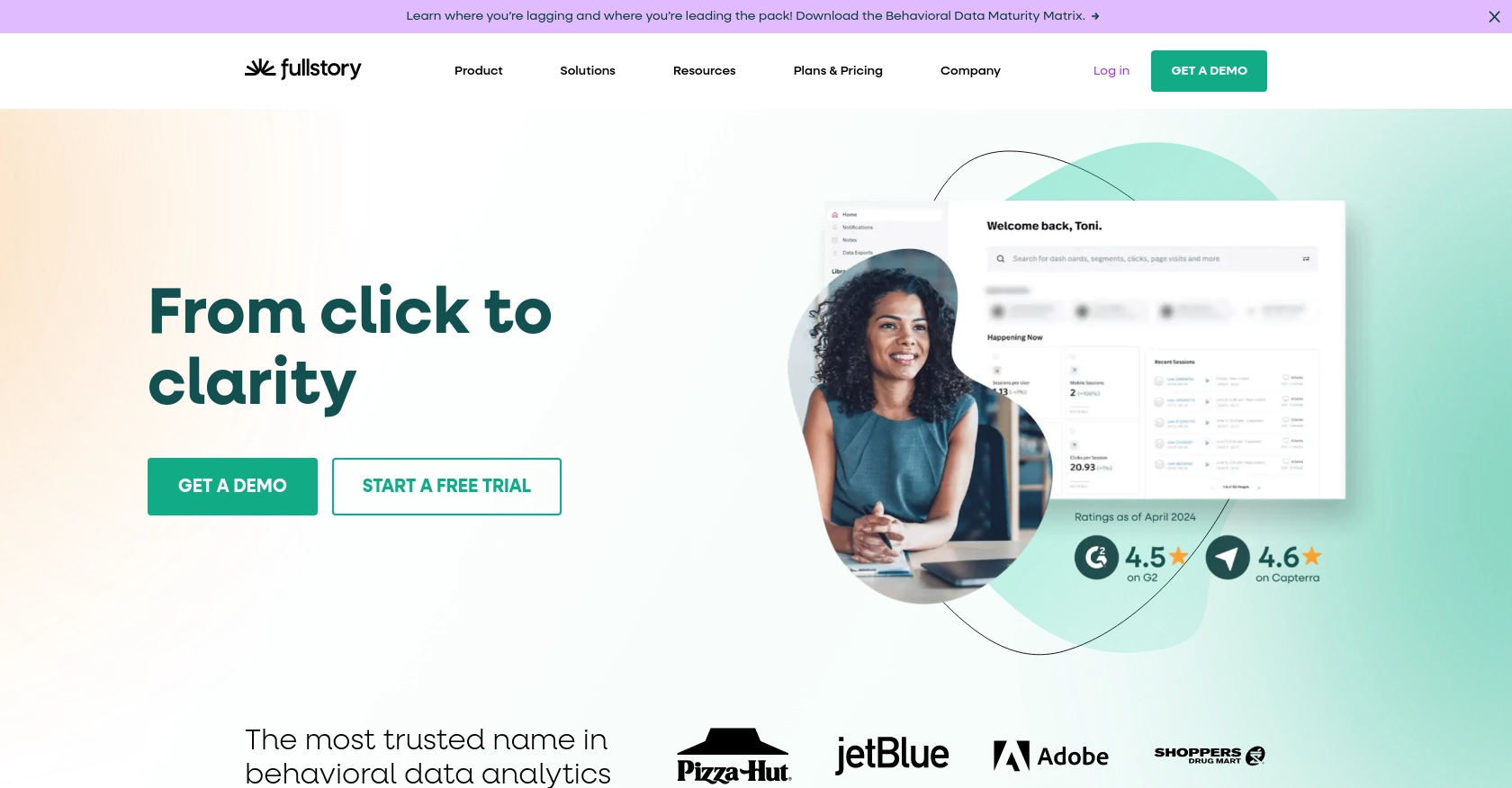
Introduction to Fullstory API for Page Analytics
Fullstory is a powerful analytics platform that provides comprehensive insights into user interactions on websites and applications. By capturing detailed session data, Fullstory enables businesses to understand user behavior, optimize user experience, and drive engagement.
Developers may want to integrate with Fullstory's API to access detailed page analytics, which can be used to enhance data-driven decision-making processes. For example, a developer might use the Fullstory API to retrieve page view statistics and user interaction data, allowing for the creation of personalized user experiences or targeted marketing campaigns.
Setting Up Your Fullstory Account for API Access
Before you can start using the Fullstory API to gather page analytics, you'll need to set up your Fullstory account and obtain the necessary API key. This key will allow you to authenticate your requests and access the data you need.
Creating a Fullstory Account
If you don't already have a Fullstory account, you can sign up for a free trial or a demo account on the Fullstory website. This will give you access to the platform's features and allow you to explore its capabilities.
- Visit the Fullstory website and click on "Get Started" to create an account.
- Follow the on-screen instructions to complete the registration process.
- Once your account is set up, log in to access the Fullstory dashboard.
Generating a Fullstory API Key
To interact with the Fullstory API, you'll need to generate an API key with the appropriate permissions. Follow these steps to create your API key:
- Navigate to the "Settings" section in the Fullstory dashboard.
- Locate the "API Keys" section and click on "Create key" to generate a new API key.
- Enter a meaningful name for your API key and select the permission level. Ensure it has Admin or Architect level permissions for full access.
- Click "Save API Key" and copy the key value from the modal that appears. Store it securely, as you won't be able to view it again.
For more details on managing API keys, refer to the Fullstory Developer Guide.
Understanding Fullstory API Authentication
Fullstory's API uses API key-based authentication. You'll need to include your API key in the Authorization header of your requests. Here's the format you should use:
Authorization: Basic {YOUR_API_KEY}
Ensure that your API key is kept confidential and only shared with trusted parties. For more information, visit the Fullstory Authentication Guide.
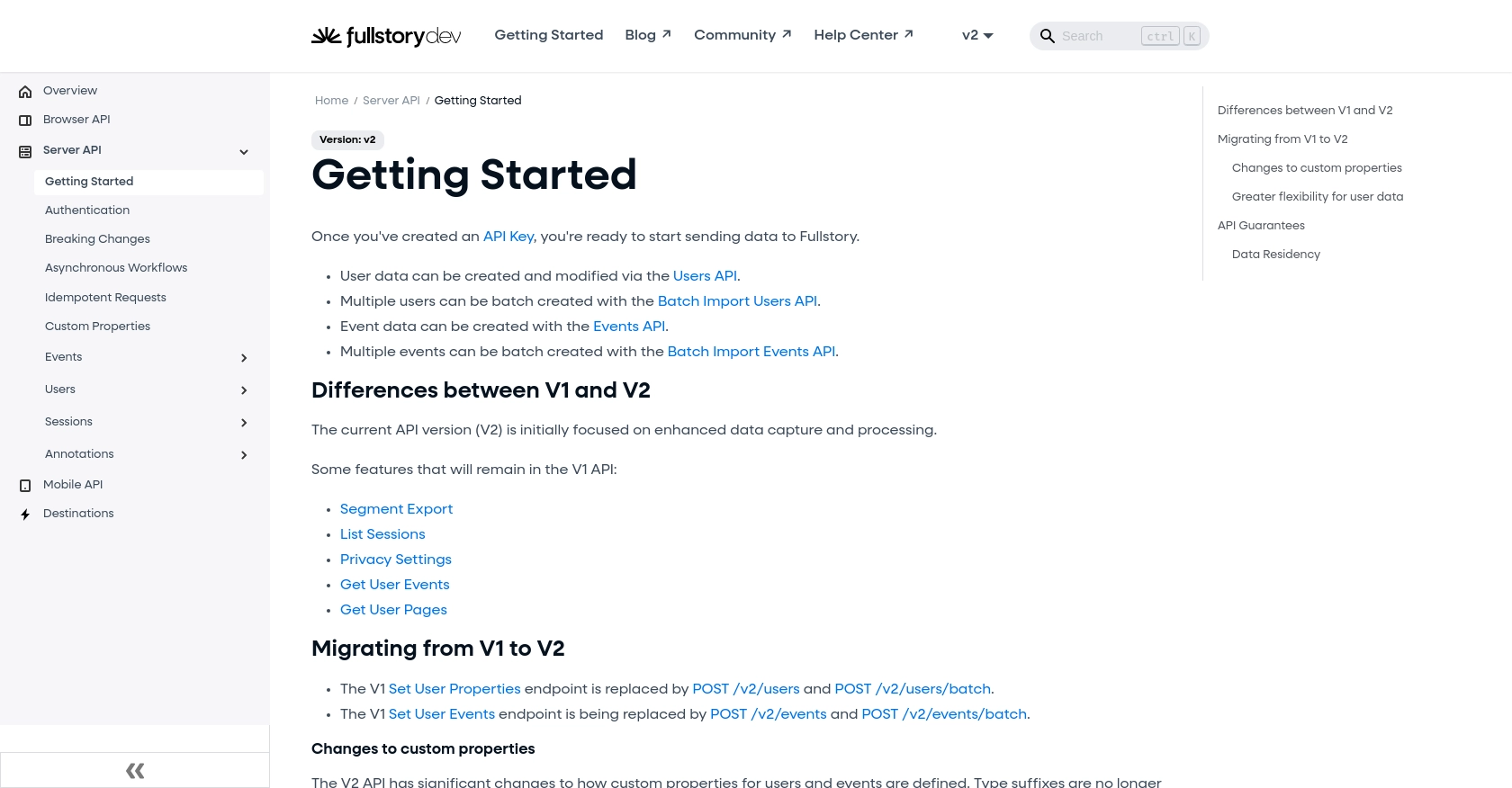
sbb-itb-96038d7
Making API Calls to Fullstory for Page Analytics Using JavaScript
To effectively utilize the Fullstory API for retrieving page analytics, you'll need to make API calls using JavaScript. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses and errors.
Setting Up Your JavaScript Environment for Fullstory API
Before making API calls, ensure you have a suitable JavaScript environment. You can use Node.js or a browser-based environment. For this tutorial, we'll use Node.js. Ensure you have Node.js installed on your machine.
- Download and install Node.js from the official website.
- Verify the installation by running
node -v
in your terminal.
Next, you'll need to install the axios
library to handle HTTP requests:
npm install axios
Writing JavaScript Code to Retrieve Fullstory Page Analytics
With your environment set up, you can now write the JavaScript code to make API calls to Fullstory. Create a new file named getPageAnalytics.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const segmentId = 'your_segment_id'; // Replace with your actual segment ID
const endpoint = `https://api.fullstory.com/segments/v1/${segmentId}`;
const headers = {
'Authorization': 'Basic YOUR_API_KEY', // Replace with your actual API key
'Accept': 'application/json'
};
// Make a GET request to the Fullstory API
axios.get(endpoint, { headers })
.then(response => {
console.log('Page Analytics:', response.data);
})
.catch(error => {
console.error('Error fetching page analytics:', error.response ? error.response.data : error.message);
});
Replace YOUR_API_KEY
with your actual API key and your_segment_id
with the segment ID you wish to analyze.
Understanding the Fullstory API Response
Upon a successful API call, the response will contain detailed analytics data for the specified segment. Here's an example of what the response might look like:
{
"id": "12345abcdefg",
"name": "Power Users (Web)",
"creator": "alice@example.com",
"created": "2020-10-01T12:34:56.123Z",
"url": "https://app.fullstory.com/ui/yourOrgId/segments/12345abcdefg"
}
This data includes the segment ID, name, creator, creation date, and a URL to view the segment in Fullstory.
Handling Errors and Troubleshooting Fullstory API Calls
When making API calls, it's crucial to handle potential errors gracefully. Common errors include authentication failures, invalid segment IDs, or network issues. The code snippet above includes basic error handling, which logs the error message to the console.
For more detailed error information, refer to the Fullstory API documentation.
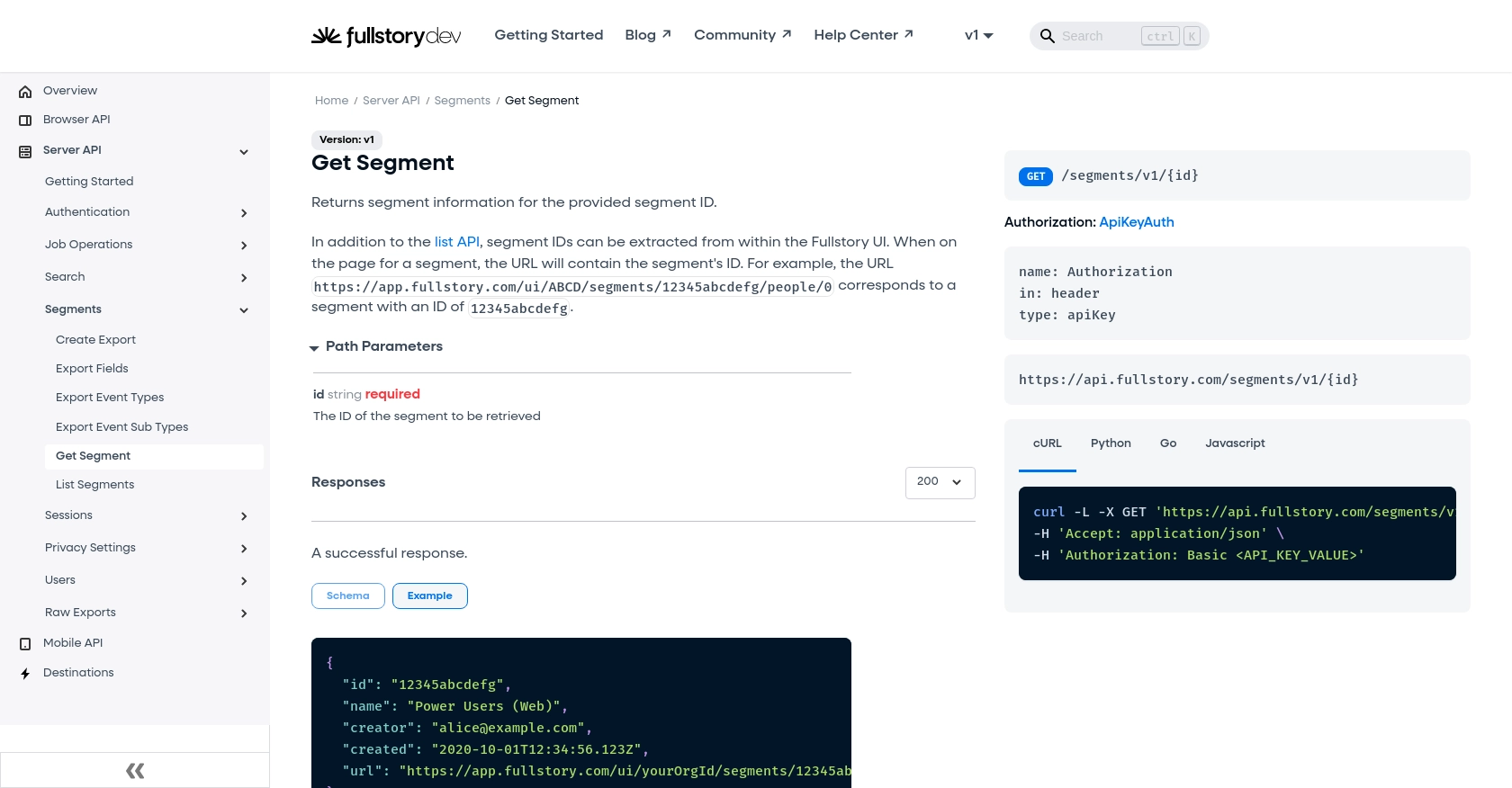
Conclusion and Best Practices for Using Fullstory API in JavaScript
Integrating Fullstory's API for page analytics using JavaScript provides developers with powerful insights into user interactions and behaviors. By following the steps outlined in this guide, you can effectively retrieve and utilize analytics data to enhance user experiences and drive informed business decisions.
Best Practices for Fullstory API Integration
- Secure API Keys: Always store your API keys securely and avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure consistency in data formats and structures when processing analytics data. This will aid in seamless integration with other systems.
- Error Handling: Implement robust error handling to manage potential issues such as network failures or invalid requests. Log errors for further analysis and debugging.
Enhancing Integration with Endgrate
For developers seeking to streamline their integration processes, consider leveraging Endgrate. By using Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product development. Endgrate provides a unified API endpoint that simplifies interactions with multiple platforms, including Fullstory, ensuring a seamless integration experience for your customers.
Explore how Endgrate can enhance your integration capabilities by visiting Endgrate's website and discover how it can help you build efficient, scalable integrations with ease.
Read More
Ready to get started?