Using the Keap API to Create or Update Contacts (with Python examples)
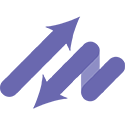
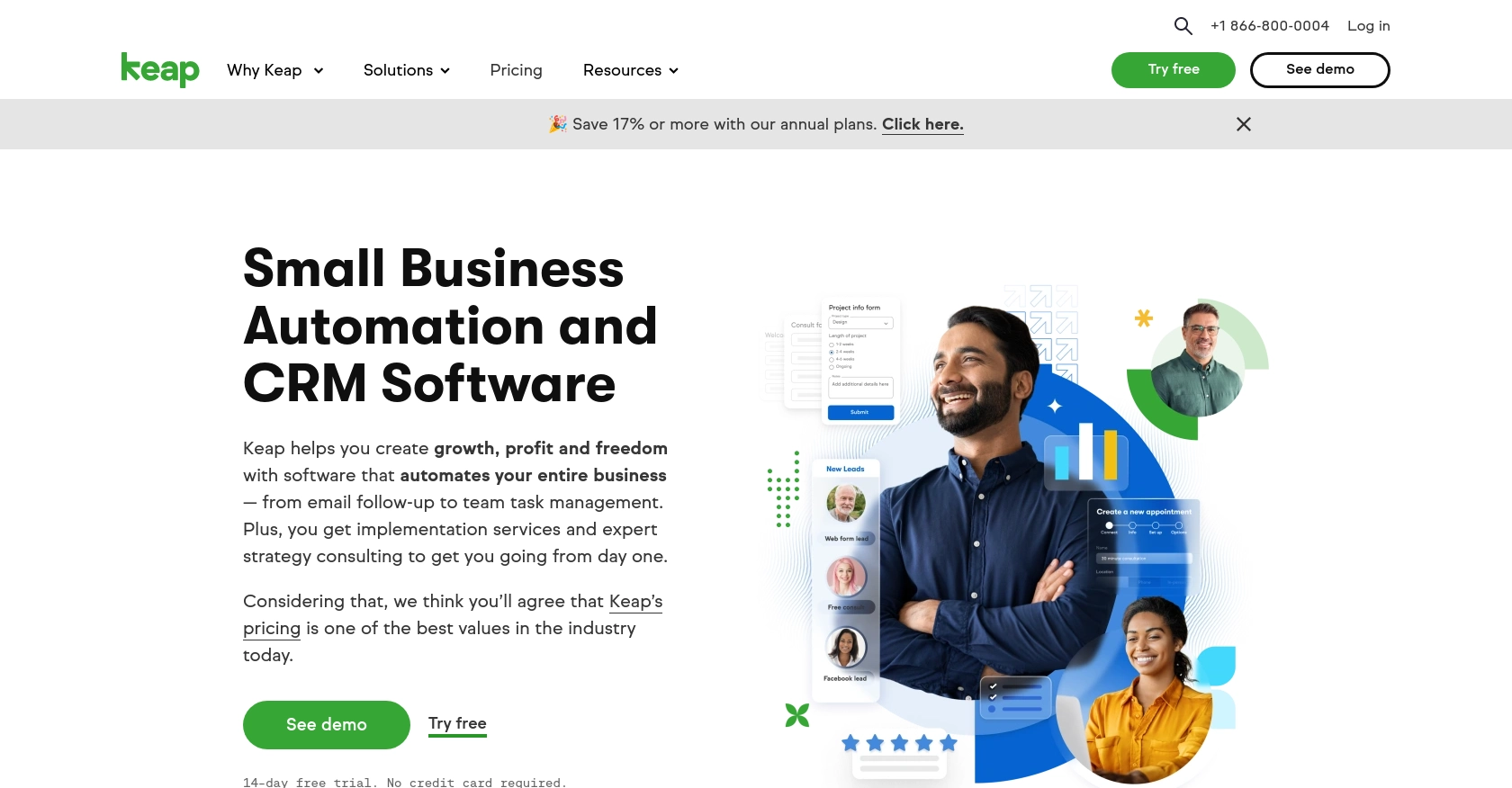
Introduction to Keap API Integration
Keap, formerly known as Infusionsoft, is a powerful CRM and marketing automation platform designed to help small businesses streamline their sales and marketing efforts. With features like contact management, email marketing, and sales automation, Keap enables businesses to nurture leads and convert them into loyal customers.
Integrating with Keap's API allows developers to automate and enhance various business processes. For example, you can use the Keap API to create or update contact information directly from your application, ensuring that your CRM data is always up-to-date and accurate. This integration can be particularly useful for businesses looking to synchronize customer data across multiple platforms, automate marketing campaigns, or manage customer interactions more effectively.
Setting Up Your Keap Developer Account and Sandbox Environment
Before you can start integrating with the Keap API, you'll need to set up a developer account and create a sandbox environment. This will allow you to test your API interactions without affecting live data. Follow these steps to get started:
Register for a Keap Developer Account
To begin, you'll need to register for a Keap developer account. This account will give you access to the necessary tools and resources to build and test your integrations.
- Visit the Keap Developer Portal.
- Click on the "Register" button to create a new developer account.
- Fill out the registration form with your details and submit it.
- Once registered, log in to your developer account to access the dashboard.
Create a Keap Sandbox App
With your developer account set up, the next step is to create a sandbox app. This sandbox environment will allow you to safely test your API calls.
- Navigate to the "Apps" section in your developer dashboard.
- Click on "Create a New App" and select "Sandbox" as the app type.
- Provide a name and description for your sandbox app.
- Click "Create" to generate your sandbox app.
Set Up OAuth Authentication for Keap API
The Keap API uses OAuth 2.0 for authentication. You'll need to create an app within your sandbox environment to obtain the client ID and client secret required for OAuth.
- In your sandbox app, navigate to the "API Keys" section.
- Click on "Create New Key" to generate a client ID and client secret.
- Note down these credentials as you'll need them for authenticating API requests.
With your developer account, sandbox app, and OAuth credentials set up, you're ready to start making API calls to Keap. This setup ensures that you can test your integrations thoroughly before deploying them in a live environment.
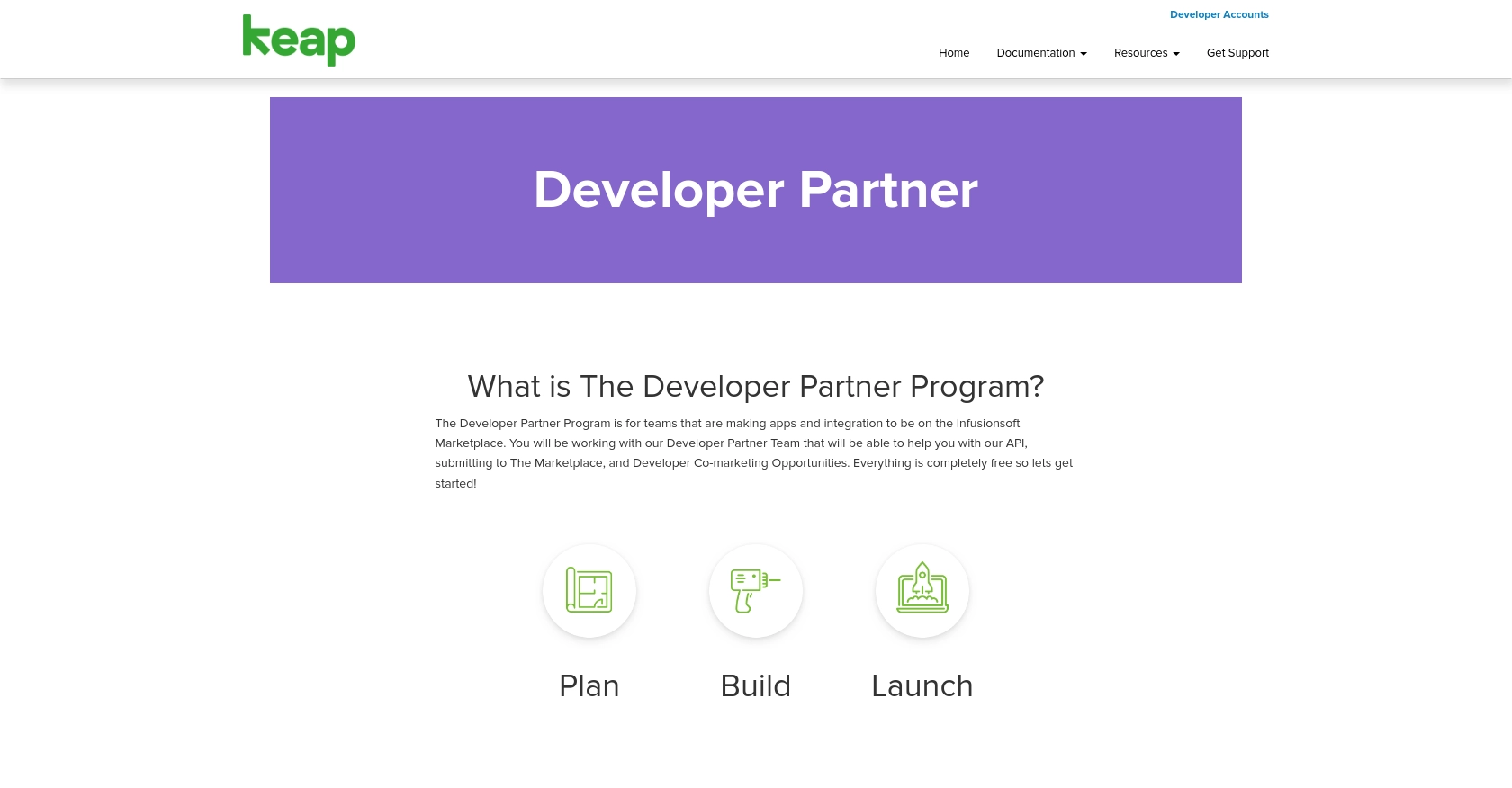
sbb-itb-96038d7
Making API Calls to Keap Using Python
With your Keap developer account and sandbox environment set up, you can now proceed to make API calls. In this section, we will guide you through the process of creating or updating contacts using Python. This will involve setting up your Python environment, writing the code to interact with the Keap API, and handling potential errors.
Setting Up Your Python Environment for Keap API Integration
Before you start coding, ensure you have the following prerequisites:
- Python 3.11.1 installed on your machine.
- The Python package installer
pip
.
Once you have these installed, open your terminal or command line and install the requests
library, which will be used to make HTTP requests to the Keap API:
pip install requests
Creating or Updating Contacts with Keap API
Now, let's write a Python script to create or update contacts in Keap. Start by creating a file named keap_contacts.py
and add the following code:
import requests
# Set the API endpoint for creating or updating contacts
url = "https://api.keap.com/crm/rest/v1/contacts"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Token"
}
# Define the contact data
contact_data = {
"email": "example@keap.com",
"given_name": "John",
"family_name": "Doe"
}
# Make a POST request to create or update the contact
response = requests.post(url, json=contact_data, headers=headers)
# Check the response status
if response.status_code == 200:
print("Contact created or updated successfully.")
else:
print(f"Failed to create or update contact. Status code: {response.status_code}")
print("Response:", response.json())
Replace Your_Token
with the OAuth token you obtained earlier. This script sends a POST request to the Keap API to create or update a contact with the specified email, given name, and family name.
Running the Python Script and Verifying Results
To execute the script, run the following command in your terminal:
python keap_contacts.py
If the request is successful, you should see the message "Contact created or updated successfully." To verify, check your Keap sandbox environment to ensure the contact appears as expected.
Handling Errors and Troubleshooting
When interacting with the Keap API, you may encounter errors. Common error codes include:
- 400 Bad Request: The request was invalid. Check your data formatting.
- 401 Unauthorized: Authentication failed. Verify your OAuth token.
- 404 Not Found: The endpoint URL is incorrect.
- 500 Internal Server Error: A server error occurred. Try again later.
For more detailed error information, refer to the response body returned by the API call.
Best Practices for Keap API Integration and Data Management
When integrating with the Keap API, it's essential to follow best practices to ensure efficient and secure data management. Here are some recommendations:
- Securely Store OAuth Credentials: Always store your OAuth client ID, client secret, and tokens securely. Avoid hardcoding them in your source code. Instead, use environment variables or secure vaults.
- Handle Rate Limiting: Keap's API may have rate limits to prevent abuse. Implement logic to handle HTTP 429 status codes by pausing requests and retrying after a specified time. Check the Keap API documentation for specific rate limit details.
- Validate and Sanitize Input: Always validate and sanitize data before sending it to the API to prevent errors and ensure data integrity.
- Implement Error Handling: Use try-except blocks in Python to catch exceptions and handle errors gracefully. Log errors for further analysis and debugging.
- Standardize Data Fields: Ensure that data fields are consistent across your application and Keap. This will help in maintaining data integrity and ease of integration.
Streamlining Integrations with Endgrate
Building and maintaining multiple integrations can be time-consuming and resource-intensive. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including Keap. This allows you to:
- Save Time and Resources: Focus on your core product while Endgrate handles the complexities of integration.
- Build Once, Use Everywhere: Develop a single integration for multiple platforms, reducing redundancy and maintenance efforts.
- Enhance Customer Experience: Provide a seamless and intuitive integration experience for your users.
Explore how Endgrate can simplify your integration processes by visiting Endgrate's website and discover how it can benefit your development workflow.
Read More
Ready to get started?