Using the Insightly API to Create or Update Contacts (with Python examples)
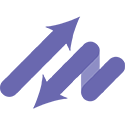
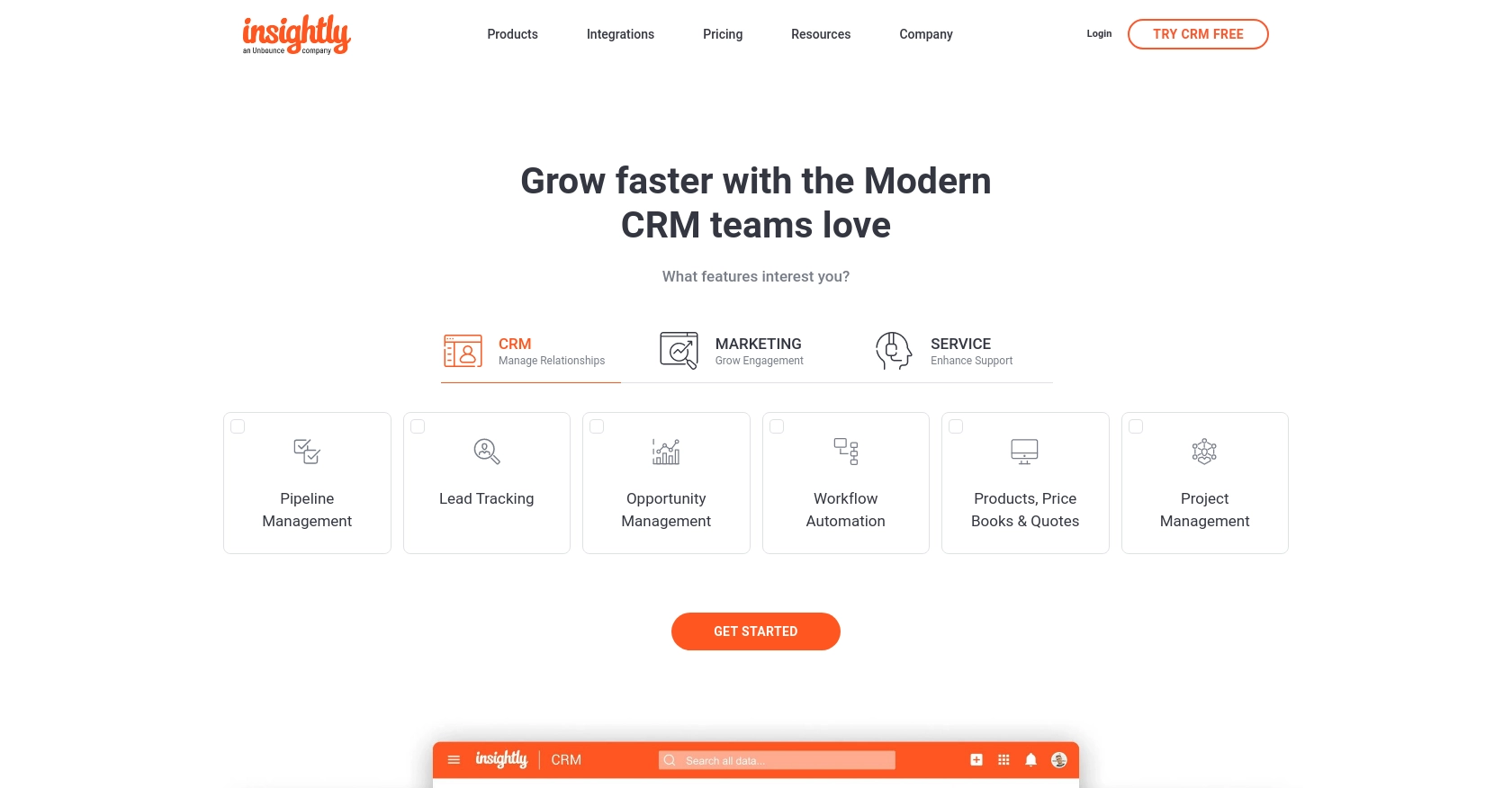
Introduction to Insightly API Integration
Insightly is a powerful CRM platform designed to help businesses manage customer relationships and streamline their sales processes. With features like project management, contact management, and workflow automation, Insightly is a popular choice for businesses looking to enhance their customer engagement strategies.
Integrating with the Insightly API allows developers to automate and enhance CRM functionalities, such as creating or updating contact information. For example, a developer might use the Insightly API to automatically update contact details from an external data source, ensuring that the CRM always contains the most current information.
Setting Up Your Insightly Test Account for API Integration
Before you can start integrating with the Insightly API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Insightly offers a straightforward way to access your API key, which is essential for authentication.
Creating an Insightly Account
If you don't already have an Insightly account, you can sign up for a free trial on the Insightly website. This will give you access to the CRM features and the ability to test API integrations.
- Visit the Insightly website and click on the "Sign Up" button.
- Follow the prompts to create your account. You'll need to provide some basic information such as your name, email, and company details.
- Once your account is created, log in to access the Insightly dashboard.
Locating Your Insightly API Key
To authenticate your API requests, you'll need your API key. Insightly uses HTTP Basic authentication, which requires this key.
- Navigate to your user profile by clicking on your profile icon in the top right corner of the Insightly dashboard.
- Select "User Settings" from the dropdown menu.
- In the "API Key and URL" section, you'll find your API key. Make sure to copy and store it securely, as you'll need it for API requests.
- For more details, refer to the Insightly support page.
Understanding Insightly API Authentication
Insightly's API uses HTTP Basic authentication. When making API calls, include your API key as the Base64-encoded username, leaving the password blank. This ensures that the API can identify the user making the request.
For testing purposes, you can paste your API key directly into the API key field without encoding when using the sandbox environment.
Testing API Calls in the Sandbox Environment
Once you have your API key, you can start testing API calls in the sandbox environment. This allows you to experiment with creating and updating contacts without impacting your live data.
- Use tools like Postman to make API requests. This can simplify the process of testing and troubleshooting your API integrations.
- Ensure that you are using the correct API endpoint format:
https://api.{pod}.insightly.com/v3.1/
. Replace{pod}
with your specific instance, which can be found in your API URL.
By following these steps, you can effectively set up your Insightly test account and begin integrating with the API to create or update contacts using Python.
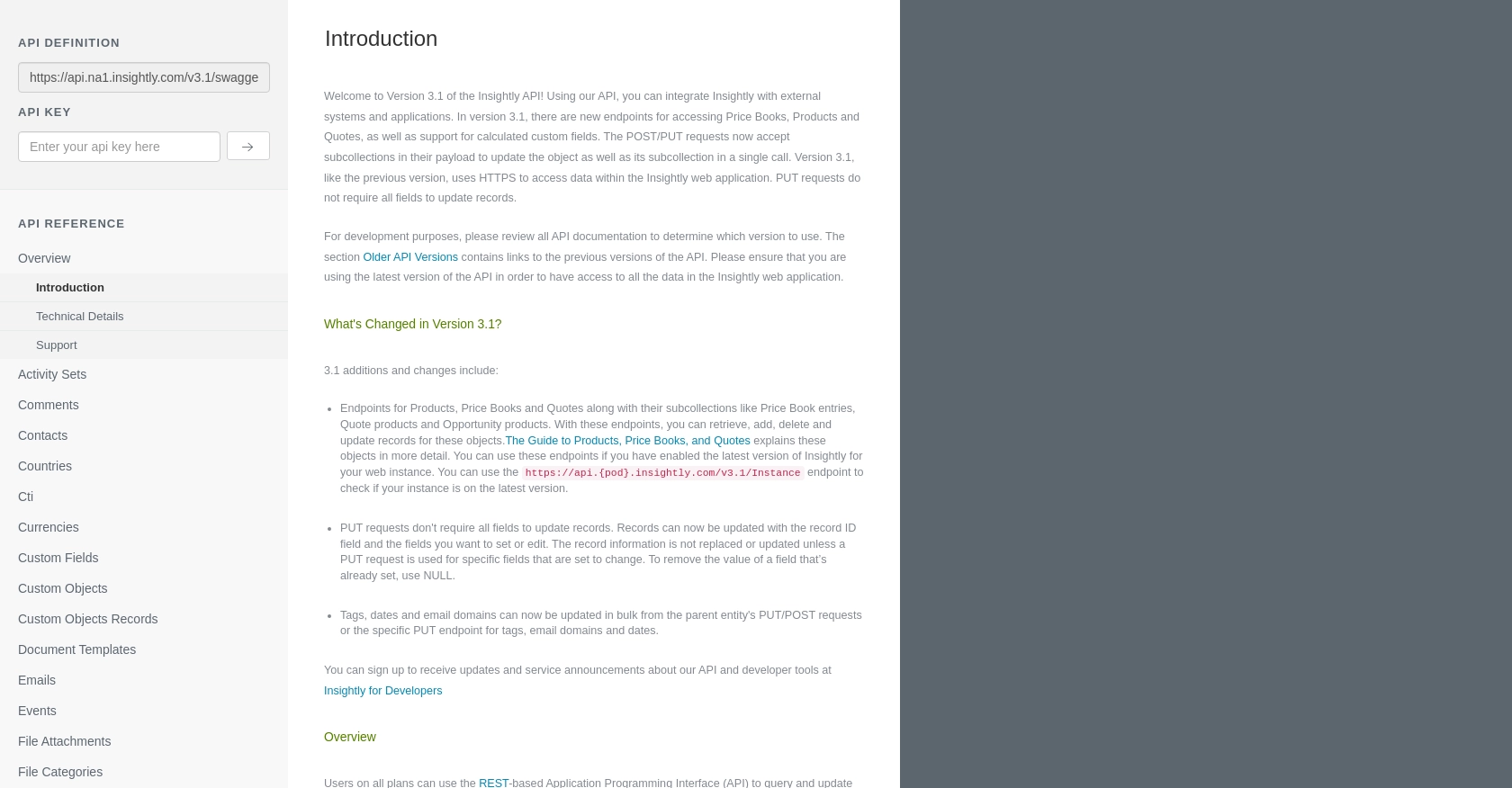
sbb-itb-96038d7
Making API Calls to Insightly for Contact Management Using Python
To interact with the Insightly API for creating or updating contacts, you'll need to use Python. This section will guide you through setting up your environment, making API calls, and handling responses effectively.
Setting Up Your Python Environment for Insightly API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python 3.11.1 from the official Python website if you haven't already.
- Use the following command to install the
requests
library:
pip install requests
Creating a New Contact in Insightly Using Python
To create a new contact in Insightly, you'll use a POST request. Below is an example of how to do this using Python:
import requests
import base64
# Set your API key and encode it
api_key = 'your_api_key_here'
encoded_key = base64.b64encode(api_key.encode()).decode()
# Define the API endpoint
url = "https://api.na1.insightly.com/v3.1/Contacts"
# Set the request headers
headers = {
"Authorization": f"Basic {encoded_key}",
"Content-Type": "application/json"
}
# Define the contact data
contact_data = {
"FIRST_NAME": "John",
"LAST_NAME": "Doe",
"EMAIL_ADDRESS": "john.doe@example.com"
}
# Make the POST request
response = requests.post(url, json=contact_data, headers=headers)
# Check the response
if response.status_code == 201:
print("Contact created successfully.")
else:
print(f"Failed to create contact: {response.status_code} - {response.text}")
Replace your_api_key_here
with your actual API key. This script sends a POST request to create a new contact with the specified details. If successful, you'll see a confirmation message.
Updating an Existing Contact in Insightly Using Python
To update an existing contact, you'll use a PUT request. Here's how you can do it:
import requests
import base64
# Set your API key and encode it
api_key = 'your_api_key_here'
encoded_key = base64.b64encode(api_key.encode()).decode()
# Define the API endpoint with the contact ID
contact_id = 123456
url = f"https://api.na1.insightly.com/v3.1/Contacts/{contact_id}"
# Set the request headers
headers = {
"Authorization": f"Basic {encoded_key}",
"Content-Type": "application/json"
}
# Define the updated contact data
updated_contact_data = {
"FIRST_NAME": "Jane",
"LAST_NAME": "Doe",
"EMAIL_ADDRESS": "jane.doe@example.com"
}
# Make the PUT request
response = requests.put(url, json=updated_contact_data, headers=headers)
# Check the response
if response.status_code == 200:
print("Contact updated successfully.")
else:
print(f"Failed to update contact: {response.status_code} - {response.text}")
Replace contact_id
with the ID of the contact you wish to update. This script sends a PUT request to update the contact's information. A successful update will return a confirmation message.
Handling API Responses and Errors
When making API calls, it's crucial to handle responses and errors appropriately. Insightly's API will return various status codes to indicate the result of your request:
- 201 Created: The contact was successfully created.
- 200 OK: The contact was successfully updated.
- 400 Bad Request: The request was invalid. Check your data and try again.
- 401 Unauthorized: Authentication failed. Verify your API key.
- 429 Too Many Requests: Rate limit exceeded. Wait and retry later.
For more details on handling errors, refer to the Insightly API documentation.
Conclusion and Best Practices for Using the Insightly API
Integrating with the Insightly API can significantly enhance your CRM capabilities by automating contact management tasks. By following the steps outlined in this guide, you can efficiently create and update contacts using Python, ensuring your CRM data remains accurate and up-to-date.
Best Practices for Secure and Efficient API Integration
- Securely Store API Keys: Always store your API keys securely and avoid hardcoding them in your scripts. Consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of Insightly's rate limits, which allow up to 10 requests per second and vary daily based on your plan. Implement retry logic to handle HTTP 429 errors gracefully.
- Data Standardization: Ensure that data fields are standardized before sending them to the API to maintain consistency across your CRM.
- Error Handling: Implement robust error handling to manage different HTTP status codes and provide meaningful feedback to users or logs.
Streamline Your Integrations with Endgrate
While integrating with Insightly's API can be powerful, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including Insightly.
By leveraging Endgrate, you can:
- Save time and resources by outsourcing integration tasks and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations.
- Provide an intuitive integration experience for your customers, enhancing their satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?