Using the Chargebee API to Create or Update Product Catalog (with Javascript examples)
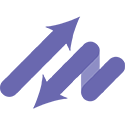
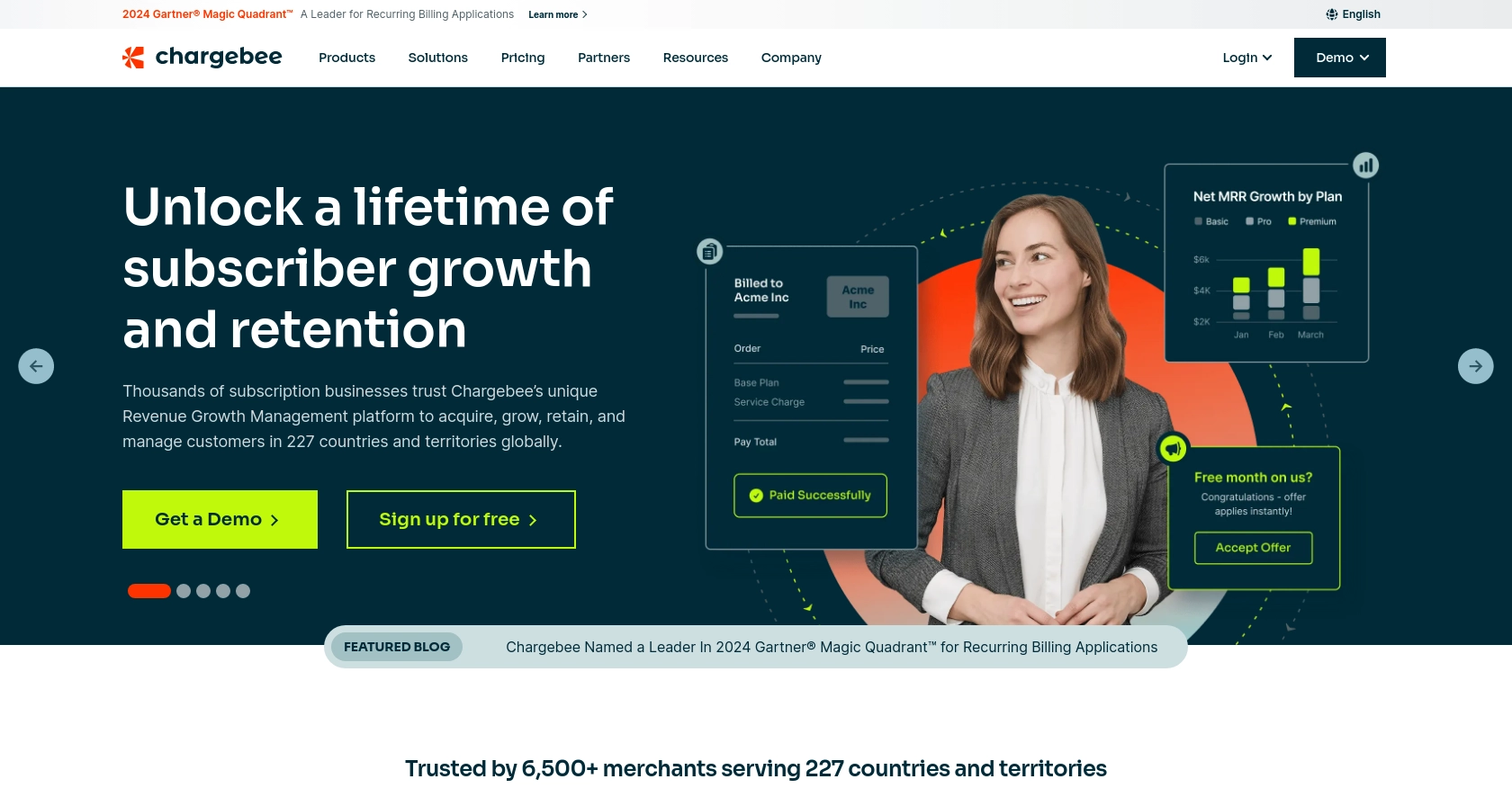
Introduction to Chargebee API for Product Catalog Management
Chargebee is a robust subscription billing and revenue management platform designed to help businesses streamline their billing processes. It offers a comprehensive suite of tools for managing subscriptions, invoicing, and revenue analytics, making it a popular choice for SaaS companies and other subscription-based businesses.
Integrating with Chargebee's API allows developers to efficiently manage product catalogs, including creating and updating items such as plans, addons, and charges. This integration is essential for businesses looking to automate and customize their billing workflows.
For example, a developer might use the Chargebee API to dynamically update product offerings based on customer demand or seasonal promotions, ensuring that the product catalog is always up-to-date and relevant.
Setting Up Your Chargebee Test/Sandbox Account for API Integration
Before you can start integrating with the Chargebee API to manage your product catalog, you need to set up a test or sandbox account. This environment allows you to safely test API calls without affecting your live data.
Creating a Chargebee Test Account
If you don't already have a Chargebee account, follow these steps to create a test account:
- Visit the Chargebee website and sign up for a free trial.
- During the sign-up process, ensure you select the option to create a test site. This will provide you with a sandbox environment to experiment with API calls.
- Once your account is set up, log in to the Chargebee dashboard.
Generating API Keys for Chargebee Authentication
Chargebee uses HTTP Basic authentication for API calls. You'll need to generate API keys to authenticate your requests:
- In the Chargebee dashboard, navigate to Settings > API Keys.
- Click on Create API Key to generate a new key.
- Make sure to copy the API key and store it securely, as it will be used as the username in your API requests. The password field should remain empty.
Note: API keys are different for your test and live sites. Ensure you use the test site key for sandbox testing.
Configuring OAuth for Chargebee API Access
Chargebee supports OAuth for secure API access. To configure OAuth:
- Navigate to Settings > OAuth Apps in the Chargebee dashboard.
- Click on Create App and fill in the necessary details, such as the app name and redirect URL.
- Once the app is created, note down the client ID and client secret, which will be used for OAuth authentication.
Testing Your Chargebee API Setup
With your test account and API keys ready, you can now proceed to test your API setup:
- Use a tool like Postman or cURL to make a test API call to Chargebee's sandbox environment.
- Verify that the response is successful and that you can interact with the product catalog as expected.
For more detailed information, refer to the Chargebee API documentation.
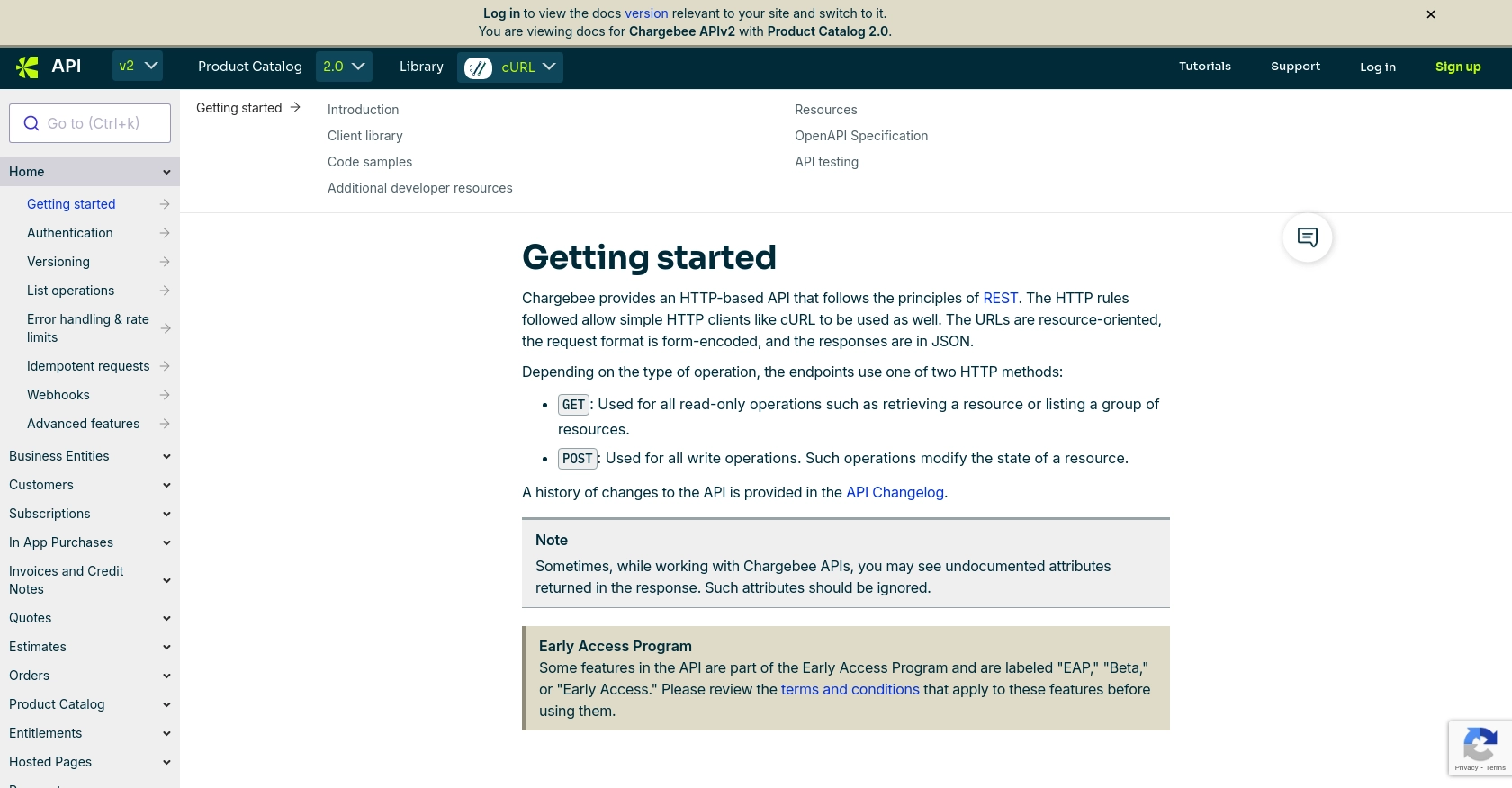
sbb-itb-96038d7
Making API Calls to Chargebee for Product Catalog Management Using JavaScript
To interact with Chargebee's API for creating or updating your product catalog, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the necessary code, and handling responses effectively.
Setting Up Your JavaScript Environment for Chargebee API Integration
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides the runtime environment needed to execute JavaScript code outside a browser.
- Download and install Node.js from the official website.
- Initialize a new Node.js project by running
npm init -y
in your terminal. - Install the
axios
library for making HTTP requests by runningnpm install axios
.
Creating a New Product Item in Chargebee Using JavaScript
To create a new product item in Chargebee, you'll need to make a POST request to the Chargebee API endpoint. Below is an example of how to do this using the axios
library.
const axios = require('axios');
const createProductItem = async () => {
try {
const response = await axios.post('https://{your-site}.chargebee.com/api/v2/items', {
id: 'new-product',
name: 'New Product',
type: 'plan',
item_applicability: 'all'
}, {
auth: {
username: '{your-api-key}',
password: ''
}
});
console.log('Product Created:', response.data);
} catch (error) {
console.error('Error creating product:', error.response.data);
}
};
createProductItem();
Replace {your-site}
with your Chargebee site name and {your-api-key}
with your API key. This script creates a new product item named "New Product" with a type of "plan".
Updating an Existing Product Item in Chargebee Using JavaScript
To update an existing product item, you'll make a POST request to the specific item endpoint. Here's how you can update an item:
const updateProductItem = async () => {
try {
const response = await axios.post('https://{your-site}.chargebee.com/api/v2/items/{item-id}', {
name: 'Updated Product Name',
description: 'Updated description for the product.'
}, {
auth: {
username: '{your-api-key}',
password: ''
}
});
console.log('Product Updated:', response.data);
} catch (error) {
console.error('Error updating product:', error.response.data);
}
};
updateProductItem();
Replace {item-id}
with the ID of the product you wish to update. This script updates the product's name and description.
Handling API Responses and Errors from Chargebee
When making API calls, it's crucial to handle responses and errors properly. Chargebee uses standard HTTP status codes to indicate success or failure:
- 2XX: Success. The API call was successful.
- 4XX: Client error. Check the request parameters.
- 5XX: Server error. Retry the request later.
For more detailed error handling, refer to the Chargebee error handling documentation.
Verifying API Requests in Chargebee Sandbox
After making API calls, verify the changes in your Chargebee sandbox account:
- Log in to your Chargebee dashboard.
- Navigate to the product catalog section to see the newly created or updated items.
For further details, consult the Chargebee API documentation.
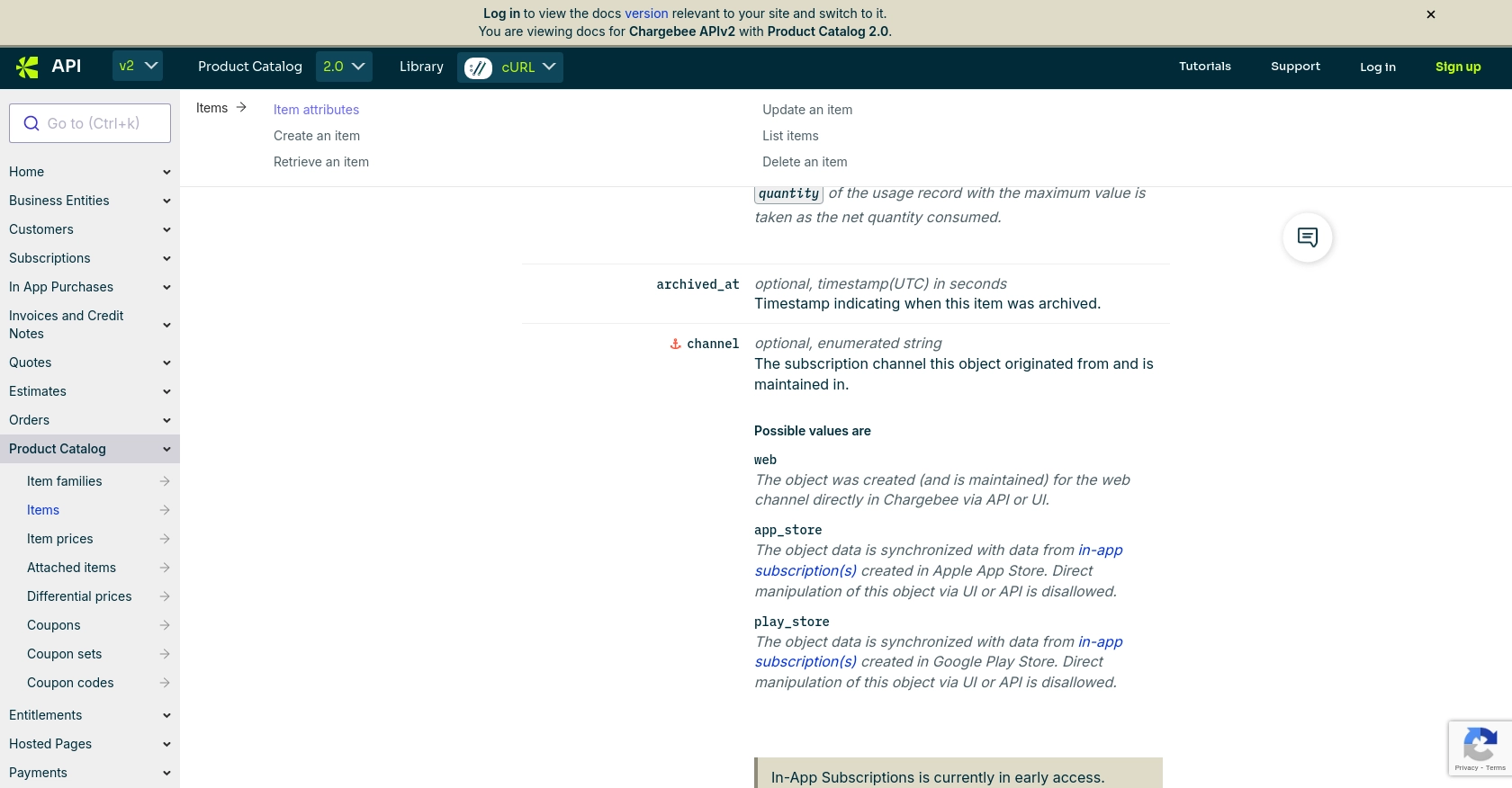
Conclusion and Best Practices for Chargebee API Integration
Integrating with Chargebee's API for managing your product catalog offers significant advantages in automating and customizing your billing workflows. By following the steps outlined in this guide, you can efficiently create and update product items using JavaScript, ensuring your catalog remains dynamic and responsive to business needs.
Best Practices for Secure and Efficient Chargebee API Usage
- Secure API Keys: Store your API keys securely and avoid including them in your codebase. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limits: Chargebee imposes rate limits on API requests. For test sites, the limit is approximately 750 API calls every 5 minutes, while live sites allow 150 calls per minute. Implement exponential backoff strategies to handle HTTP 429 errors effectively. For more details, refer to the Chargebee error handling documentation.
- Data Standardization: Ensure consistent data formats when interacting with the API to avoid errors and maintain data integrity across systems.
- Monitor API Usage: Regularly review API usage logs to identify any anomalies or unauthorized access attempts, and rotate API keys periodically to enhance security.
Streamlining Integrations with Endgrate
While integrating with Chargebee's API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Chargebee. This allows you to focus on your core product while outsourcing integration management.
By leveraging Endgrate, you can build once for each use case and enjoy an intuitive integration experience, saving both time and resources. Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
Ready to get started?