Using the FreshDesk API to Create or Update Companies in Python
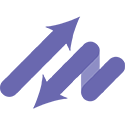
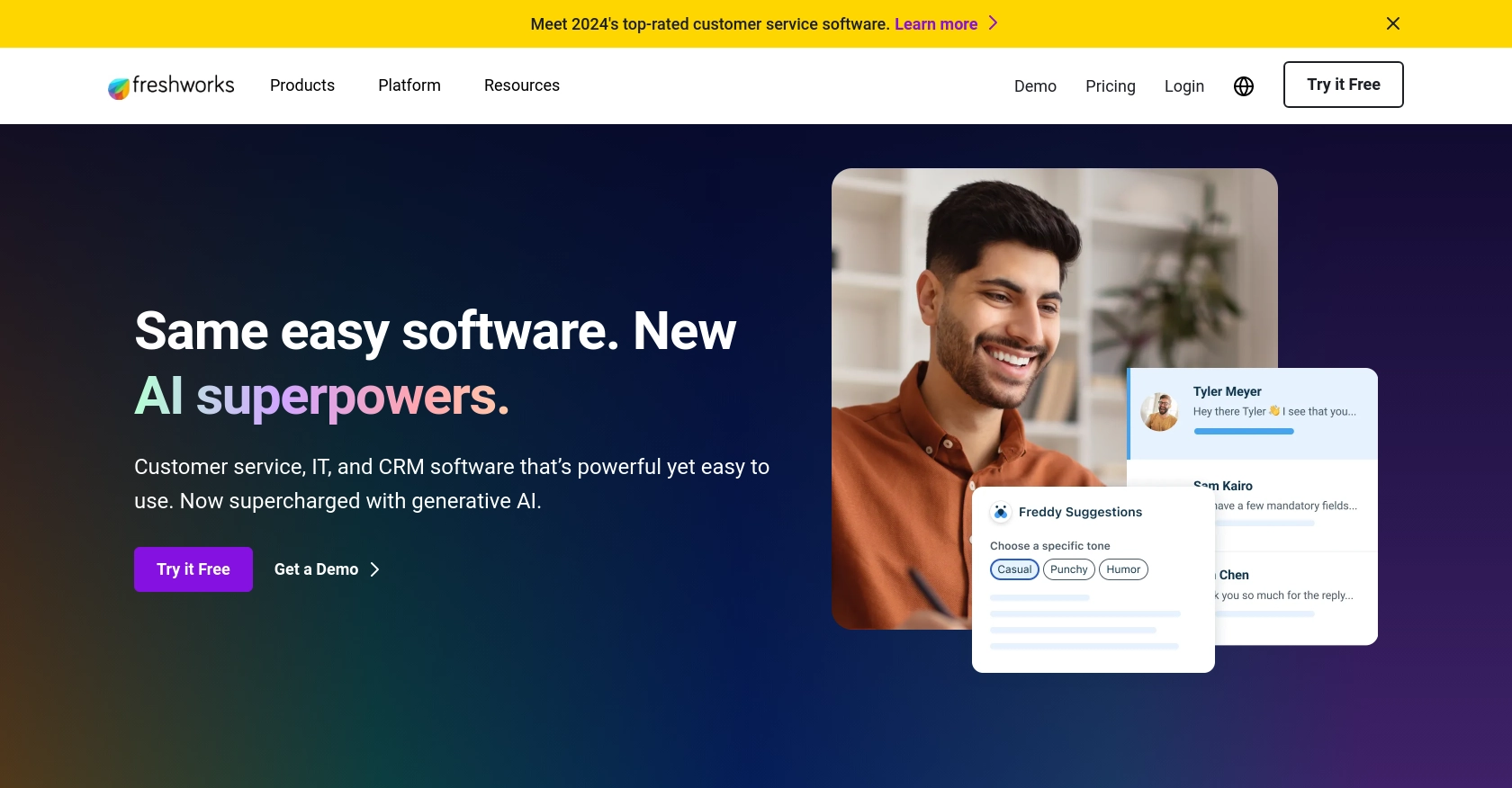
Introduction to FreshDesk API Integration
FreshDesk is a powerful customer support software that helps businesses manage customer interactions across multiple channels. It offers a comprehensive suite of tools for ticketing, collaboration, and automation, making it a popular choice for companies looking to enhance their customer service operations.
Developers may want to integrate with FreshDesk's API to automate and streamline customer support processes. For example, using the FreshDesk API, a developer can create or update company records in the FreshDesk system, ensuring that customer information is always up-to-date and accessible for support teams.
This article will guide you through using Python to interact with the FreshDesk API, specifically focusing on creating or updating company records. By following this tutorial, you'll learn how to efficiently manage company data within FreshDesk, enhancing your organization's ability to provide top-notch customer support.
Setting Up Your FreshDesk Test or Sandbox Account
Before you can start integrating with the FreshDesk API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data.
Creating a FreshDesk Account
If you don't already have a FreshDesk account, you can sign up for a free trial on the FreshDesk website. This trial will give you access to all the features you need to test the API integration.
- Visit the FreshDesk website and click on the "Sign Up" button.
- Fill in the required information, such as your email address and company name.
- Follow the instructions to complete the registration process.
Generating API Key for FreshDesk Authentication
FreshDesk uses a custom authentication method that requires an API key. Follow these steps to obtain your API key:
- Log in to your FreshDesk account.
- Navigate to your profile settings by clicking on your profile icon in the top right corner.
- Select "Profile Settings" from the dropdown menu.
- Scroll down to the "Your API Key" section and copy the API key provided.
Keep this API key secure, as it will be used to authenticate your API requests.
Configuring Your FreshDesk App for API Access
To interact with the FreshDesk API, you may need to configure certain settings within your FreshDesk account:
- Ensure that your account has the necessary permissions to create or update company records.
- Review the API documentation on the FreshDesk Developer Portal to understand the required scopes and permissions.
With your FreshDesk account and API key ready, you're now set to begin integrating with the FreshDesk API using Python. In the next section, we'll walk through the process of making API calls to create or update company records.
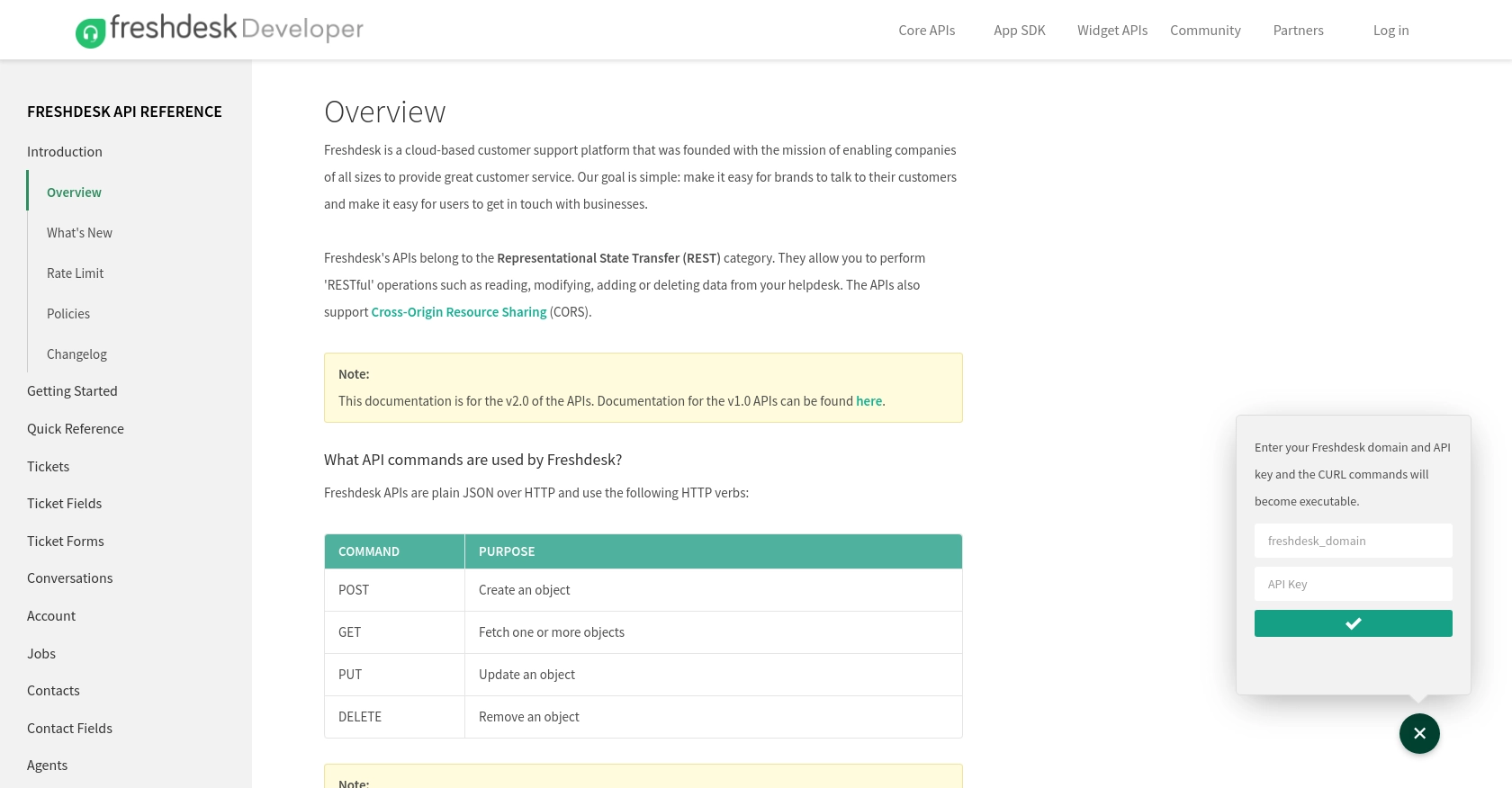
sbb-itb-96038d7
Making API Calls to Create or Update Companies in FreshDesk Using Python
To interact with the FreshDesk API and manage company records, you'll need to use Python to make HTTP requests. This section will guide you through the process of setting up your Python environment and making API calls to create or update companies in FreshDesk.
Setting Up Your Python Environment for FreshDesk API Integration
Before you begin, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer, pip
Once you have these installed, open your terminal or command prompt and install the requests
library, which will be used to make HTTP requests:
pip install requests
Creating or Updating Companies in FreshDesk with Python
Now that your environment is ready, you can proceed to write the Python code to interact with the FreshDesk API. Below is an example of how to create or update a company record:
import requests
import json
# Set the FreshDesk API endpoint
url = "https://yourdomain.freshdesk.com/api/v2/companies"
# Set the request headers
headers = {
"Content-Type": "application/json"
}
# Set your FreshDesk API key
api_key = "Your_FreshDesk_API_Key"
# Define the company data
company_data = {
"name": "Example Company",
"domains": ["example.com"],
"description": "A sample company for demonstration purposes."
}
# Make the API request to create or update the company
response = requests.post(url, auth=(api_key, "X"), headers=headers, data=json.dumps(company_data))
# Check the response status
if response.status_code in [200, 201]:
print("Company created or updated successfully.")
else:
print(f"Failed to create or update company. Status Code: {response.status_code}")
print("Response:", response.json())
In this code:
- Replace
Your_FreshDesk_API_Key
with the API key you obtained from your FreshDesk account. - The
url
variable should be updated with your FreshDesk domain. - The
company_data
dictionary contains the information for the company you want to create or update.
Verifying the API Request Success in FreshDesk
After running the script, you can verify the success of your API request by checking the FreshDesk dashboard. Navigate to the Companies section to see if the company record has been created or updated as expected.
Handling Errors and Common Error Codes in FreshDesk API
When making API calls, it's crucial to handle potential errors. The FreshDesk API may return various status codes indicating the result of your request:
- 200 OK: The request was successful, and the company was updated.
- 201 Created: The company was successfully created.
- 400 Bad Request: The request was invalid, often due to missing or incorrect parameters.
- 401 Unauthorized: Authentication failed, usually due to an incorrect API key.
- 404 Not Found: The specified resource could not be found.
Ensure you handle these errors appropriately in your code to provide meaningful feedback and take corrective actions if needed.
Conclusion and Best Practices for FreshDesk API Integration
Integrating with the FreshDesk API to create or update company records can significantly enhance your customer support operations by ensuring that your team has access to the most current and accurate information. By following the steps outlined in this article, you can efficiently manage company data within FreshDesk using Python.
Best Practices for Secure and Efficient FreshDesk API Usage
- Secure API Key Storage: Always store your FreshDesk API key securely. Consider using environment variables or a secure vault to keep your credentials safe.
- Handle Rate Limiting: Be aware of FreshDesk's rate limits to avoid exceeding them. Implement exponential backoff or retry logic to handle rate limit responses gracefully.
- Data Standardization: Ensure that the data you send to FreshDesk is standardized and validated to prevent errors and maintain data integrity.
- Error Handling: Implement robust error handling in your code to manage different API response codes effectively. This will help you troubleshoot issues quickly and maintain a smooth integration.
Streamlining Integrations with Endgrate
While integrating with FreshDesk's API can be straightforward, managing multiple integrations across different platforms can become complex and time-consuming. This is where Endgrate can be a valuable asset.
Endgrate offers a unified API endpoint that connects to various platforms, including FreshDesk, allowing you to manage all your integrations through a single interface. By leveraging Endgrate, you can save time and resources, focus on your core product, and provide an intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration processes by visiting Endgrate's website and discover how you can build once for each use case instead of multiple times for different integrations.
Read More
Ready to get started?