How to Create or Update organizations with the Capsule API in Python
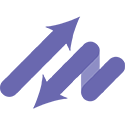
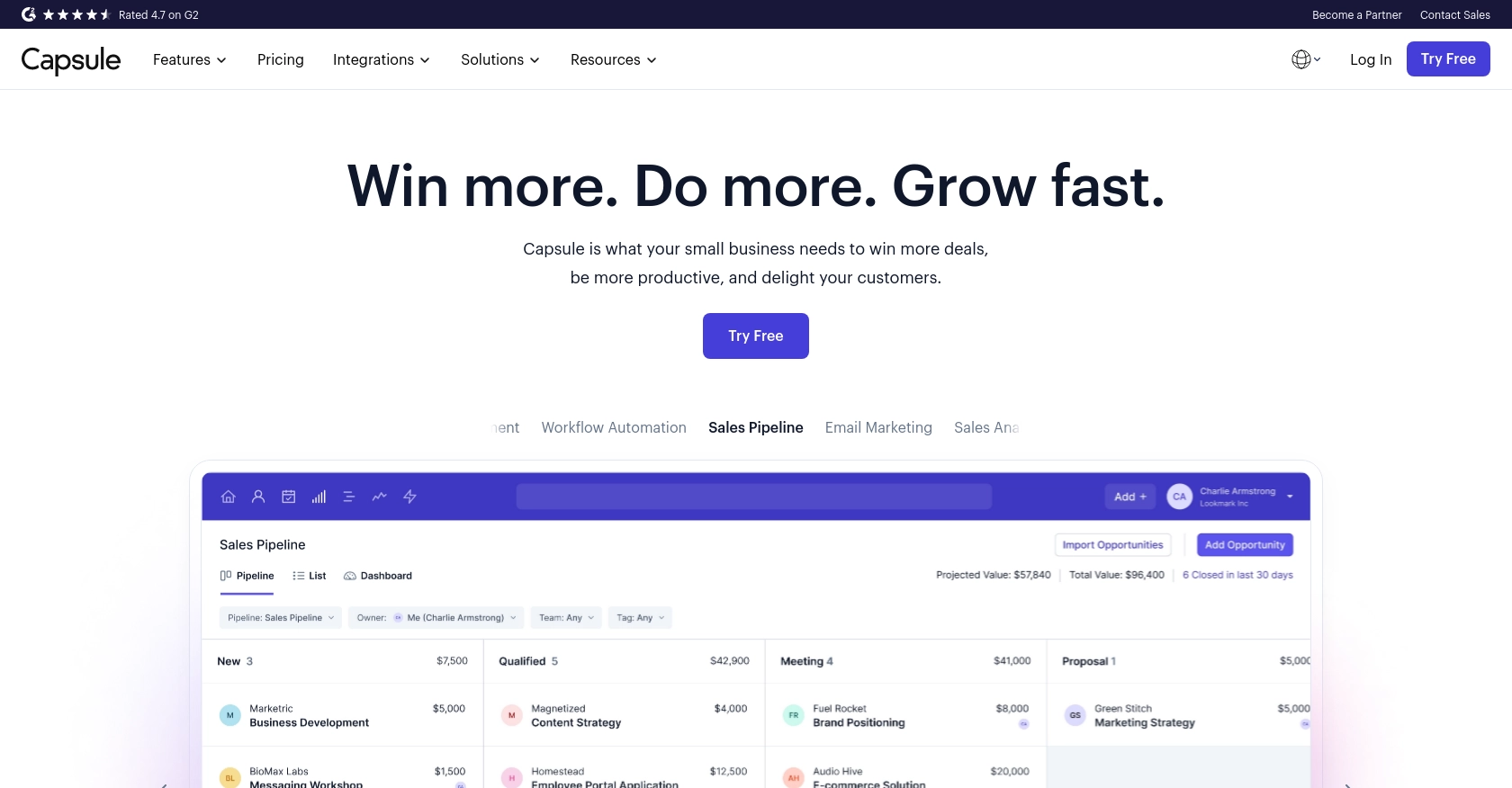
Introduction to Capsule CRM
Capsule CRM is a versatile customer relationship management platform designed to help businesses manage their contacts, sales opportunities, and customer interactions efficiently. With its user-friendly interface and robust features, Capsule CRM is a popular choice for businesses looking to streamline their CRM processes.
Integrating with Capsule's API allows developers to automate and enhance CRM functionalities, such as creating or updating organizations within the platform. For example, a developer might use the Capsule API to automatically update organization details from an external database, ensuring that the CRM data remains current and accurate.
Setting Up Your Capsule CRM Test Account for API Integration
Before you can start integrating with Capsule CRM's API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data.
Creating a Capsule CRM Account
If you don't already have a Capsule CRM account, you can sign up for a free trial on the Capsule CRM website. This trial will give you access to all the features you need to test the API integration.
- Visit the Capsule CRM signup page.
- Fill out the registration form with your details.
- Verify your email address to activate your account.
Generating OAuth Credentials for Capsule API
Capsule CRM uses OAuth 2.0 for authentication, which means you'll need to create an application within your Capsule account to obtain the necessary credentials.
- Log in to your Capsule CRM account.
- Navigate to My Preferences and select API Authentication Tokens.
- Click on Create New Token to generate a token for your application.
- Note down the Client ID and Client Secret as you'll need these for API authentication.
Setting Up OAuth 2.0 Authorization
To authenticate API requests, follow these steps to implement the OAuth 2.0 flow:
- Redirect users to the Capsule authorization URL:
https://api.capsulecrm.com/oauth/authorise
. - After user approval, Capsule will redirect back to your specified URL with an authorization code.
- Exchange this code for an access token by making a POST request to
https://api.capsulecrm.com/oauth/token
.
import requests
# Exchange authorization code for access token
url = "https://api.capsulecrm.com/oauth/token"
data = {
"code": "your_authorization_code",
"client_id": "your_client_id",
"client_secret": "your_client_secret",
"grant_type": "authorization_code"
}
response = requests.post(url, data=data)
tokens = response.json()
access_token = tokens['access_token']
Once you have the access token, you can use it to authenticate your API requests by including it in the Authorization header as a Bearer token.
Testing Your Capsule CRM API Setup
With your test account and OAuth credentials set up, you're ready to start making API calls to Capsule CRM. Ensure that you verify the success of your requests by checking the responses and the Capsule CRM dashboard.
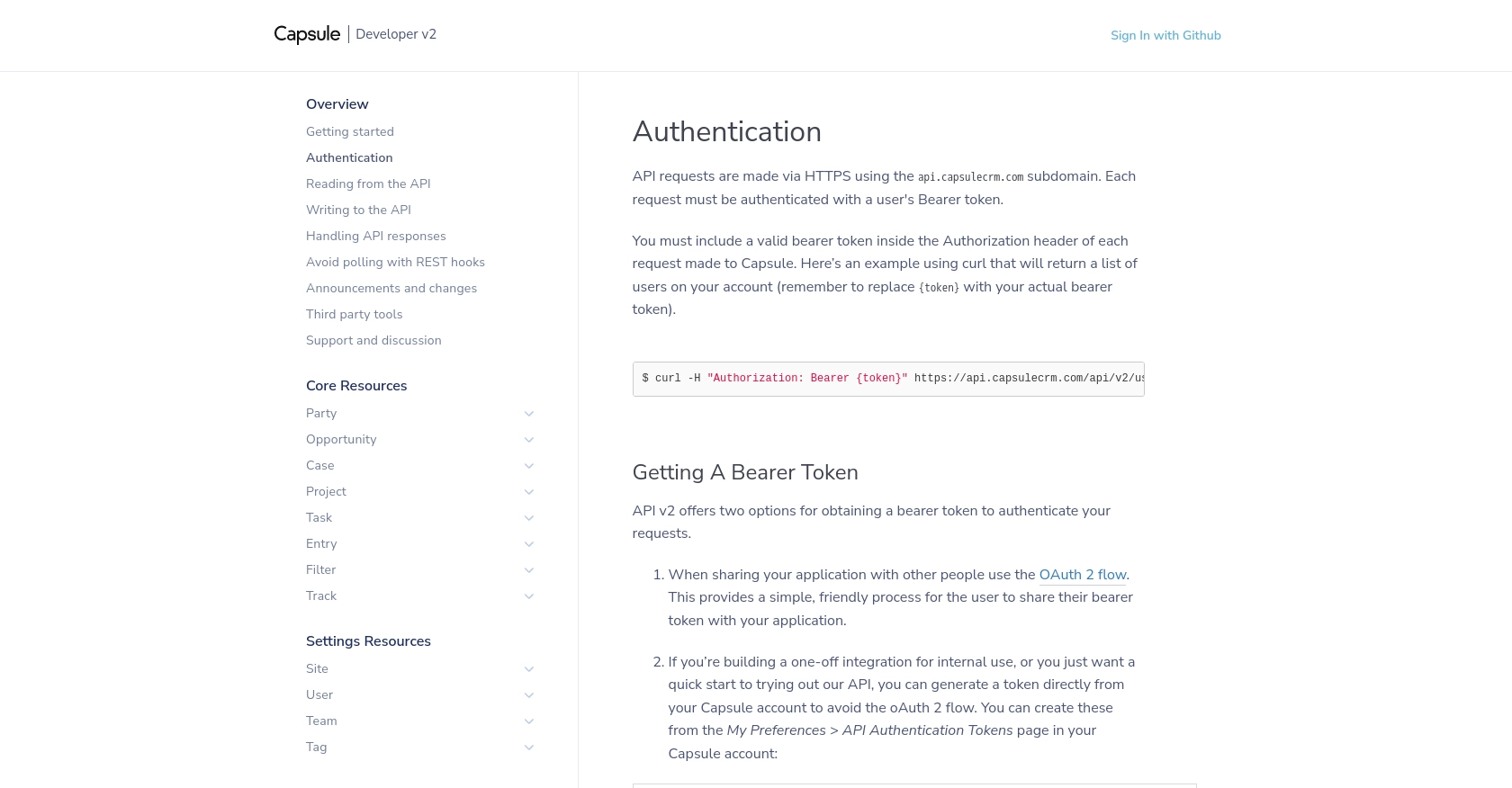
sbb-itb-96038d7
Making API Calls to Create or Update Organizations with Capsule API in Python
To interact with Capsule CRM's API for creating or updating organizations, you'll need to use Python and make HTTP requests to the appropriate endpoints. This section will guide you through the process of setting up your Python environment, writing the code to make API calls, and handling responses effectively.
Setting Up Your Python Environment for Capsule API Integration
Before you begin, ensure that you have Python 3.x installed on your machine. Additionally, you'll need the requests
library to handle HTTP requests. You can install it using pip:
pip install requests
Creating an Organization with Capsule API
To create a new organization in Capsule CRM, you'll make a POST request to the /api/v2/parties
endpoint. Here's a sample code snippet to create an organization:
import requests
# Set the API endpoint and headers
url = "https://api.capsulecrm.com/api/v2/parties"
headers = {
"Authorization": "Bearer your_access_token",
"Content-Type": "application/json"
}
# Define the organization data
data = {
"party": {
"type": "organisation",
"name": "New Organization",
"emailAddresses": [{"type": "Work", "address": "info@neworg.com"}],
"addresses": [{"city": "New York", "country": "United States"}]
}
}
# Make the POST request
response = requests.post(url, json=data, headers=headers)
# Check the response
if response.status_code == 201:
print("Organization created successfully:", response.json())
else:
print("Failed to create organization:", response.json())
Replace your_access_token
with the access token obtained from the OAuth 2.0 flow. The response will include the details of the newly created organization if successful.
Updating an Existing Organization with Capsule API
To update an existing organization, you'll use a PUT request to the /api/v2/parties/{partyId}
endpoint. Here's how you can update an organization:
import requests
# Set the API endpoint and headers
party_id = "your_organization_id"
url = f"https://api.capsulecrm.com/api/v2/parties/{party_id}"
headers = {
"Authorization": "Bearer your_access_token",
"Content-Type": "application/json"
}
# Define the updated organization data
data = {
"party": {
"name": "Updated Organization Name",
"emailAddresses": [{"type": "Work", "address": "contact@updatedorg.com"}]
}
}
# Make the PUT request
response = requests.put(url, json=data, headers=headers)
# Check the response
if response.status_code == 200:
print("Organization updated successfully:", response.json())
else:
print("Failed to update organization:", response.json())
Ensure you replace your_organization_id
and your_access_token
with the appropriate values. The response will confirm the update if successful.
Handling API Responses and Errors
When making API calls, it's crucial to handle responses and potential errors effectively. Capsule CRM's API will return various status codes to indicate the result of your request:
- 201 Created: The organization was successfully created.
- 200 OK: The organization was successfully updated.
- 400 Bad Request: The request was malformed or contained invalid data.
- 401 Unauthorized: The access token is invalid or expired.
- 403 Forbidden: The request is not allowed due to insufficient permissions.
- 429 Too Many Requests: The rate limit has been exceeded. Capsule allows up to 4,000 requests per hour.
For more details on handling API responses, refer to the Capsule API documentation.
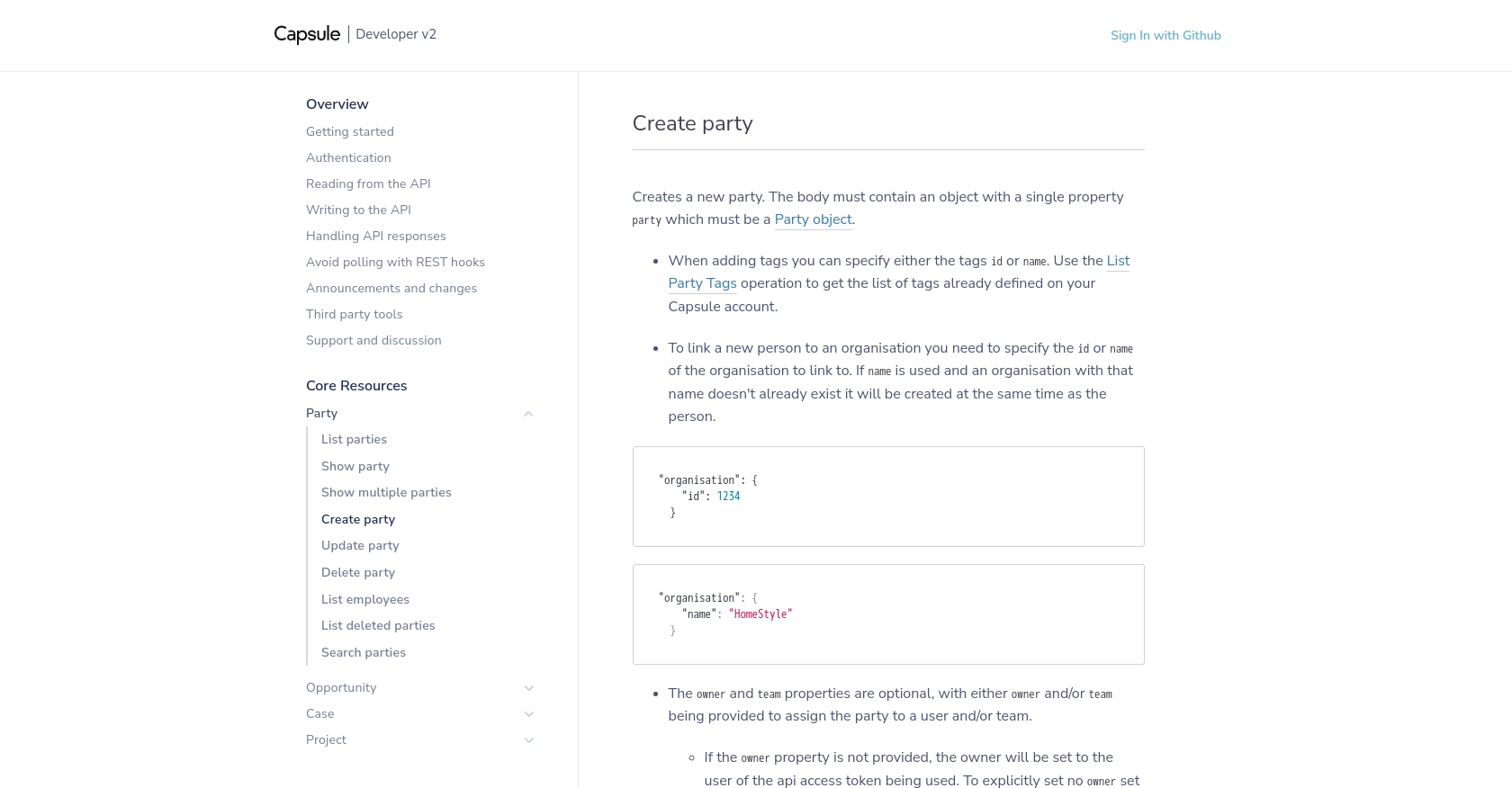
Conclusion and Best Practices for Capsule API Integration
Integrating with Capsule CRM's API offers a powerful way to automate and enhance your CRM processes. By following the steps outlined in this guide, you can efficiently create and update organizations using Python, ensuring your CRM data remains accurate and up-to-date.
Best Practices for Secure and Efficient Capsule API Usage
- Securely Store Credentials: Always keep your OAuth credentials, such as the client ID and client secret, secure. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limits: Capsule CRM allows up to 4,000 requests per hour. Monitor the
X-RateLimit-Remaining
header to avoid exceeding this limit and implement retry logic with exponential backoff if necessary. - Standardize Data Fields: Ensure that data fields are consistent across your systems to avoid discrepancies when syncing data between Capsule CRM and other platforms.
- Monitor API Responses: Regularly check API responses for errors and handle them gracefully. Use logging to track issues and improve the reliability of your integration.
Streamline Your Integration Process with Endgrate
Building and maintaining integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Capsule CRM. By leveraging Endgrate, you can focus on your core product while outsourcing integration tasks, saving both time and resources.
Explore how Endgrate can enhance your integration experience by visiting Endgrate's website and discover the benefits of a seamless, intuitive integration solution.
Read More
- https://endgrate.com/provider/capsulecrm
- https://developer.capsulecrm.com/v2/overview/authentication
- https://developer.capsulecrm.com/v2/overview/handling-api-responses
- https://developer.capsulecrm.com/v2/overview/avoid-polling-with-rest-hooks
- https://developer.capsulecrm.com/v2/operations/Party#createParty
Ready to get started?