Using the Hubspot API to Get Contacts (with Javascript examples)
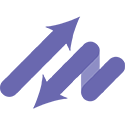
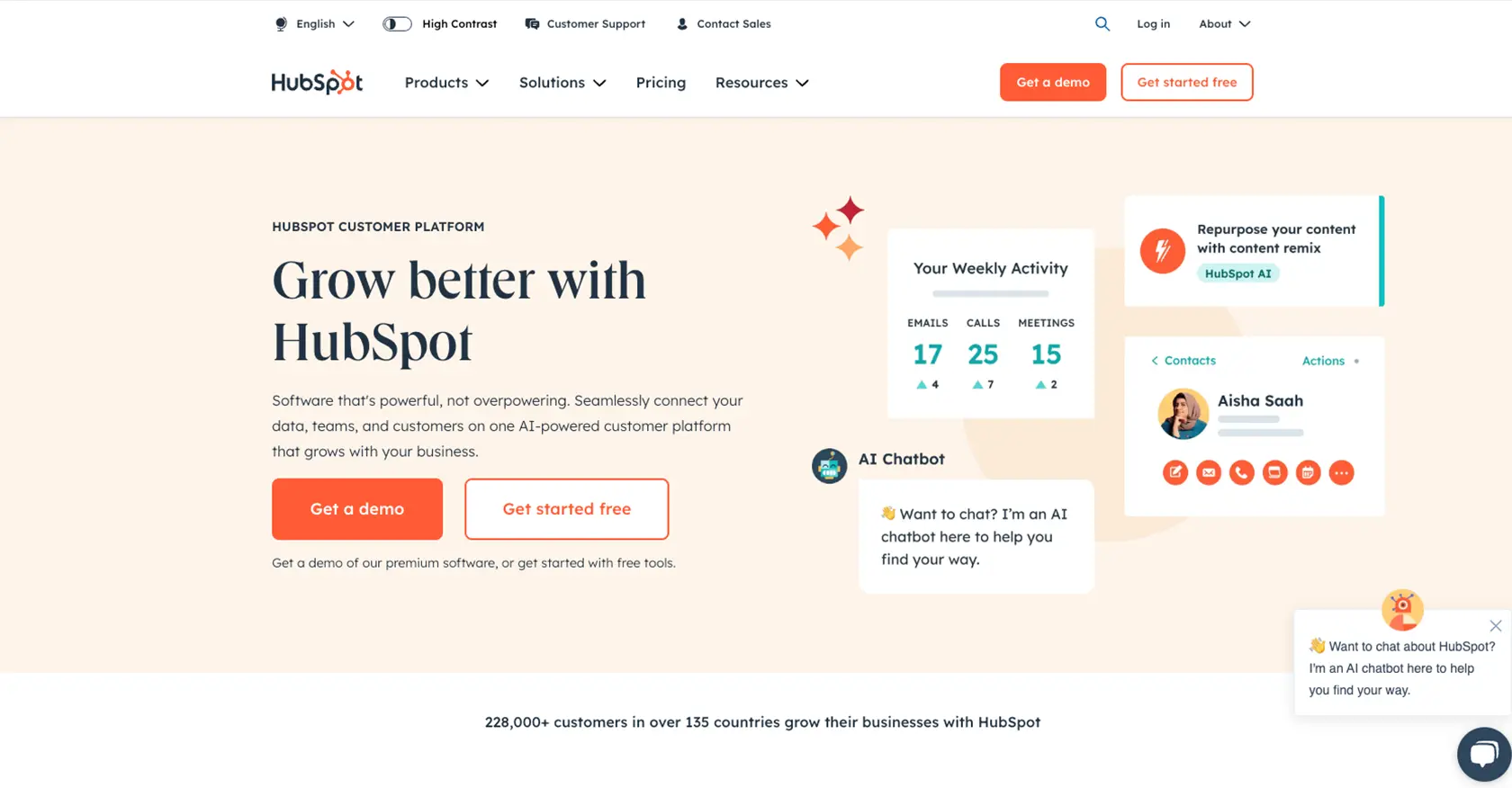
Introduction to HubSpot API Integration
HubSpot is a comprehensive CRM platform that offers a wide range of tools to help businesses manage their marketing, sales, and customer service efforts. Known for its user-friendly interface and robust features, HubSpot is a popular choice for companies looking to enhance their customer relationship management.
Developers often seek to integrate with HubSpot's API to access and manage customer data efficiently. By leveraging the HubSpot API, developers can automate tasks such as retrieving contact information, which can be used to personalize marketing campaigns or streamline customer service operations.
For example, a developer might use the HubSpot API to fetch contact details and integrate them into a custom dashboard, providing sales teams with real-time insights into customer interactions. This article will guide you through using JavaScript to interact with the HubSpot API, focusing on retrieving contact information.
Setting Up Your HubSpot Test Account for API Integration
Before diving into the HubSpot API with JavaScript, it's essential to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data. HubSpot offers a developer account that provides access to create apps and test integrations.
Creating a HubSpot Developer Account
If you don't already have a HubSpot developer account, follow these steps to create one:
- Visit the HubSpot Developer Portal.
- Click on "Create a developer account" and fill in the required details.
- Once your account is set up, log in to access the developer dashboard.
Setting Up OAuth Authentication for HubSpot API
HubSpot uses OAuth for secure API authentication. Follow these steps to set up OAuth for your application:
- In your HubSpot developer account, navigate to the "Apps" section.
- Click on "Create app" to start a new application.
- Provide the necessary details such as app name, description, and redirect URL.
- Under the "Auth" tab, select "OAuth 2.0" as the authentication method.
- Configure the required scopes for accessing contact information, such as
crm.objects.contacts.read
. - Save your app to generate the Client ID and Client Secret, which you'll use for authentication.
Generating Access Tokens Using OAuth
With your app set up, you can now generate access tokens to authenticate API requests:
- Direct users to the HubSpot authorization URL, including your Client ID and requested scopes.
- Once users authorize, they'll be redirected to your specified URL with an authorization code.
- Exchange this authorization code for an access token by making a POST request to HubSpot's token endpoint.
// Example POST request to exchange authorization code for access token
const axios = require('axios');
const tokenUrl = 'https://api.hubapi.com/oauth/v1/token';
const data = {
grant_type: 'authorization_code',
client_id: 'Your_Client_ID',
client_secret: 'Your_Client_Secret',
redirect_uri: 'Your_Redirect_URI',
code: 'Authorization_Code'
};
axios.post(tokenUrl, data)
.then(response => {
console.log('Access Token:', response.data.access_token);
})
.catch(error => {
console.error('Error fetching access token:', error);
});
Store the access token securely, as it will be used to authenticate your API requests. Remember to handle token expiration by refreshing tokens as needed.
For more detailed information on OAuth setup, refer to the HubSpot Authentication Documentation.
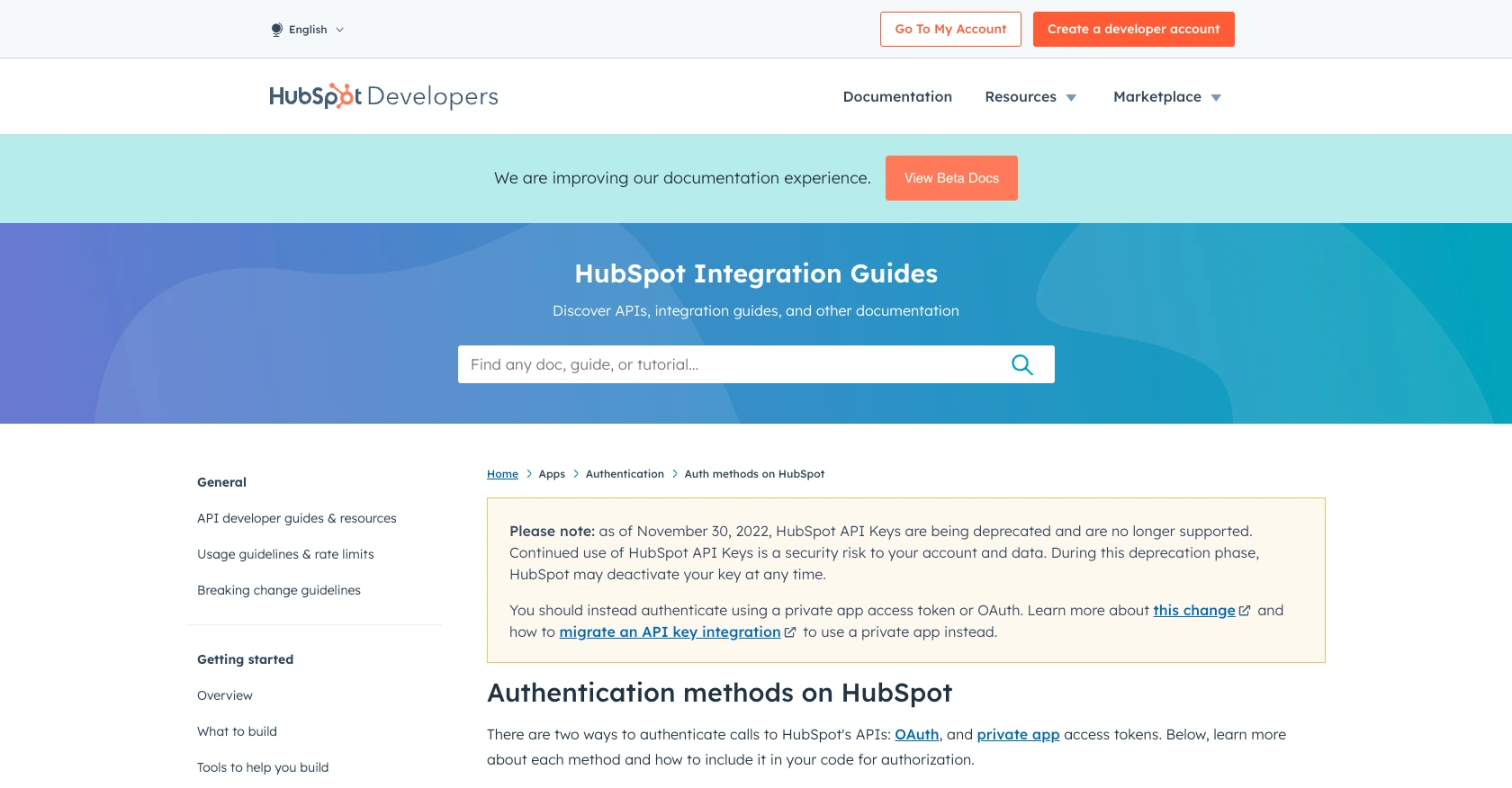
sbb-itb-96038d7
Making API Calls to Retrieve HubSpot Contacts Using JavaScript
To interact with the HubSpot API and retrieve contact information using JavaScript, you'll need to ensure your development environment is set up correctly. This involves using Node.js and installing necessary dependencies. Let's walk through the process step by step.
Setting Up Your JavaScript Environment for HubSpot API
Before making API calls, ensure you have Node.js installed on your machine. You can download it from the official Node.js website. Once installed, use npm (Node Package Manager) to install the Axios library, which simplifies HTTP requests.
npm install axios
Writing JavaScript Code to Fetch HubSpot Contacts
With your environment ready, you can now write the JavaScript code to make API calls to HubSpot. The following example demonstrates how to retrieve contacts using Axios:
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://api.hubapi.com/crm/v3/objects/contacts';
const headers = {
'Authorization': 'Bearer Your_Access_Token',
'Content-Type': 'application/json'
};
// Make a GET request to the HubSpot API
axios.get(endpoint, { headers })
.then(response => {
console.log('Contacts:', response.data.results);
})
.catch(error => {
console.error('Error fetching contacts:', error.response.data);
});
Replace Your_Access_Token
with the access token obtained from the OAuth process. This code sets up the API endpoint and headers, then makes a GET request to retrieve contacts. The response data is logged to the console.
Verifying Successful API Requests in HubSpot
After running the code, verify the request's success by checking the console output for contact data. You can also log into your HubSpot test account to ensure the contacts match the retrieved data.
Handling Errors and Understanding HubSpot API Error Codes
When making API calls, it's crucial to handle potential errors. The HubSpot API may return various error codes, such as 401 for unauthorized access or 429 for rate limits exceeded. Here's how you can handle these errors in your code:
axios.get(endpoint, { headers })
.then(response => {
console.log('Contacts:', response.data.results);
})
.catch(error => {
if (error.response) {
console.error('Error:', error.response.status, error.response.data.message);
} else {
console.error('Error:', error.message);
}
});
This code checks for errors in the response and logs the status code and message, helping you diagnose issues quickly.
For more details on error codes, refer to the HubSpot Contacts API Documentation.
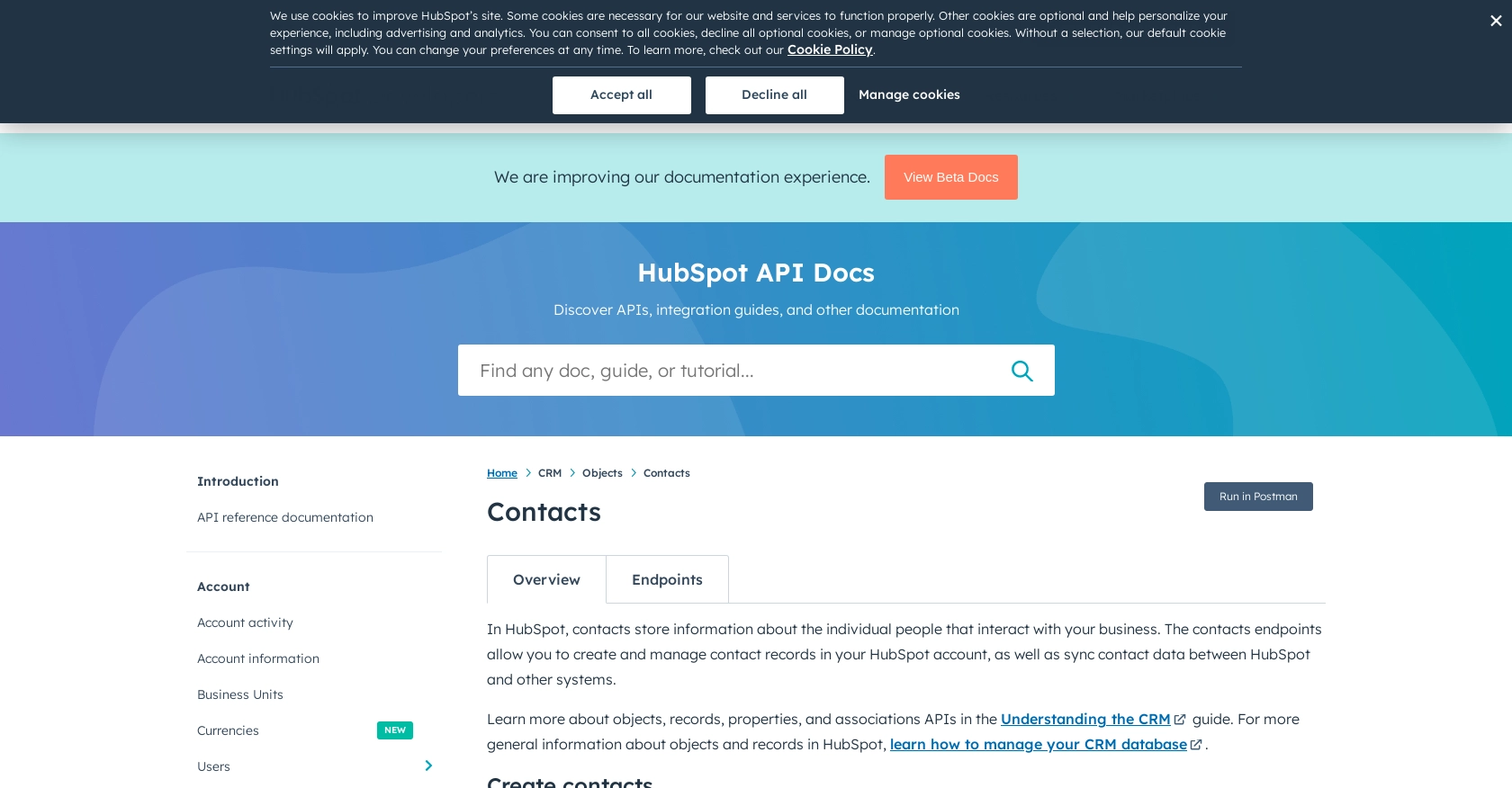
Conclusion and Best Practices for Using HubSpot API with JavaScript
Integrating with the HubSpot API using JavaScript offers developers a powerful way to manage and automate customer data processes. By following the steps outlined in this guide, you can efficiently retrieve contact information and integrate it into your applications, enhancing your CRM capabilities.
Best Practices for Secure and Efficient HubSpot API Integration
- Securely Store Access Tokens: Ensure that access tokens are stored securely and are not exposed in your codebase. Consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of HubSpot's rate limits, which allow 100 requests every 10 seconds for OAuth apps. Implement throttling to avoid hitting these limits. For more details, refer to the HubSpot API Usage Guidelines.
- Refresh Tokens Regularly: OAuth tokens expire, so implement a mechanism to refresh tokens before they expire to maintain seamless API access.
- Standardize Data Fields: When retrieving and storing contact data, ensure consistency in data fields to facilitate easy integration with other systems.
Enhance Your Integration Strategy with Endgrate
While building integrations with HubSpot can be rewarding, it can also be time-consuming and complex. Endgrate simplifies this process by offering a unified API endpoint that connects to multiple platforms, including HubSpot. This allows you to focus on your core product while outsourcing the intricacies of integration development.
Explore how Endgrate can streamline your integration efforts and provide an intuitive experience for your customers. Visit Endgrate to learn more and start optimizing your integration strategy today.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/contacts
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?