Using the Salesforce Sandbox API to Get Records in Javascript
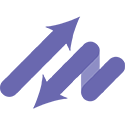
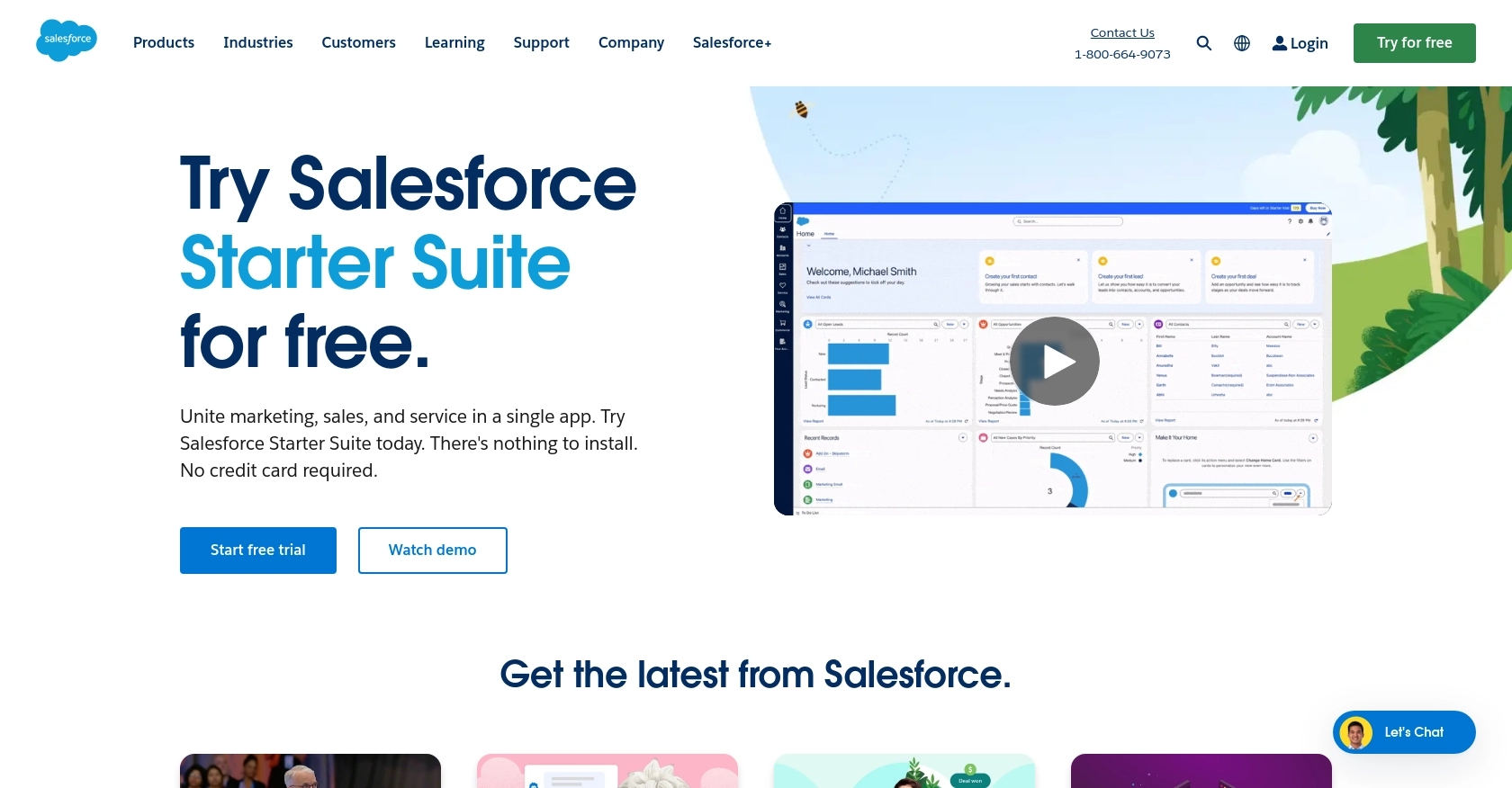
Introduction to Salesforce Sandbox API
Salesforce Sandbox is a powerful tool that allows developers to create and test applications in a secure environment that mirrors their production setup. It provides a safe space to experiment with new features, integrations, and customizations without affecting live data.
Integrating with the Salesforce Sandbox API is essential for developers looking to access and manage Salesforce data programmatically. By connecting with the API, developers can automate data retrieval and manipulation, enabling seamless integration with other systems. For example, a developer might use the Salesforce Sandbox API to fetch customer records and synchronize them with an external CRM system, ensuring data consistency across platforms.
Setting Up Your Salesforce Sandbox Account for API Integration
Before you can start interacting with the Salesforce Sandbox API, you'll need to set up a Salesforce Sandbox account. This environment allows you to test and develop without impacting your live data, providing a safe space to experiment with API integrations.
Creating a Salesforce Sandbox Account
If you don't already have a Salesforce Sandbox account, you can create one by following these steps:
- Log in to your Salesforce production account.
- Navigate to the Setup menu by clicking on the gear icon in the top right corner.
- In the Quick Find box, type Sandboxes and select it from the dropdown.
- Click on New Sandbox to create a new sandbox environment.
- Choose the type of sandbox you need (e.g., Developer, Developer Pro) and fill in the required details.
- Click Create to initiate the sandbox creation process.
Once the sandbox is created, you'll receive an email notification with login details.
Setting Up OAuth Authentication for Salesforce Sandbox API
Salesforce uses OAuth 2.0 for authentication, which requires setting up a connected app. Follow these steps to create a connected app and obtain the necessary credentials:
- Log in to your Salesforce Sandbox account.
- Go to the Setup menu and search for App Manager in the Quick Find box.
- Click on New Connected App in the top right corner.
- Fill in the required fields, such as Connected App Name and API Name.
- Under API (Enable OAuth Settings), check the box for Enable OAuth Settings.
- Enter a Callback URL (this can be a placeholder for now).
- Select the necessary OAuth scopes, such as Full Access or Access and manage your data (api).
- Click Save to create the connected app.
After saving, you'll receive a Consumer Key and Consumer Secret. Keep these credentials secure as they are required for API authentication.
For more detailed information on setting up a Salesforce Sandbox and OAuth authentication, refer to the official Salesforce documentation: Salesforce Sandbox Setup and OAuth and Connected Apps.
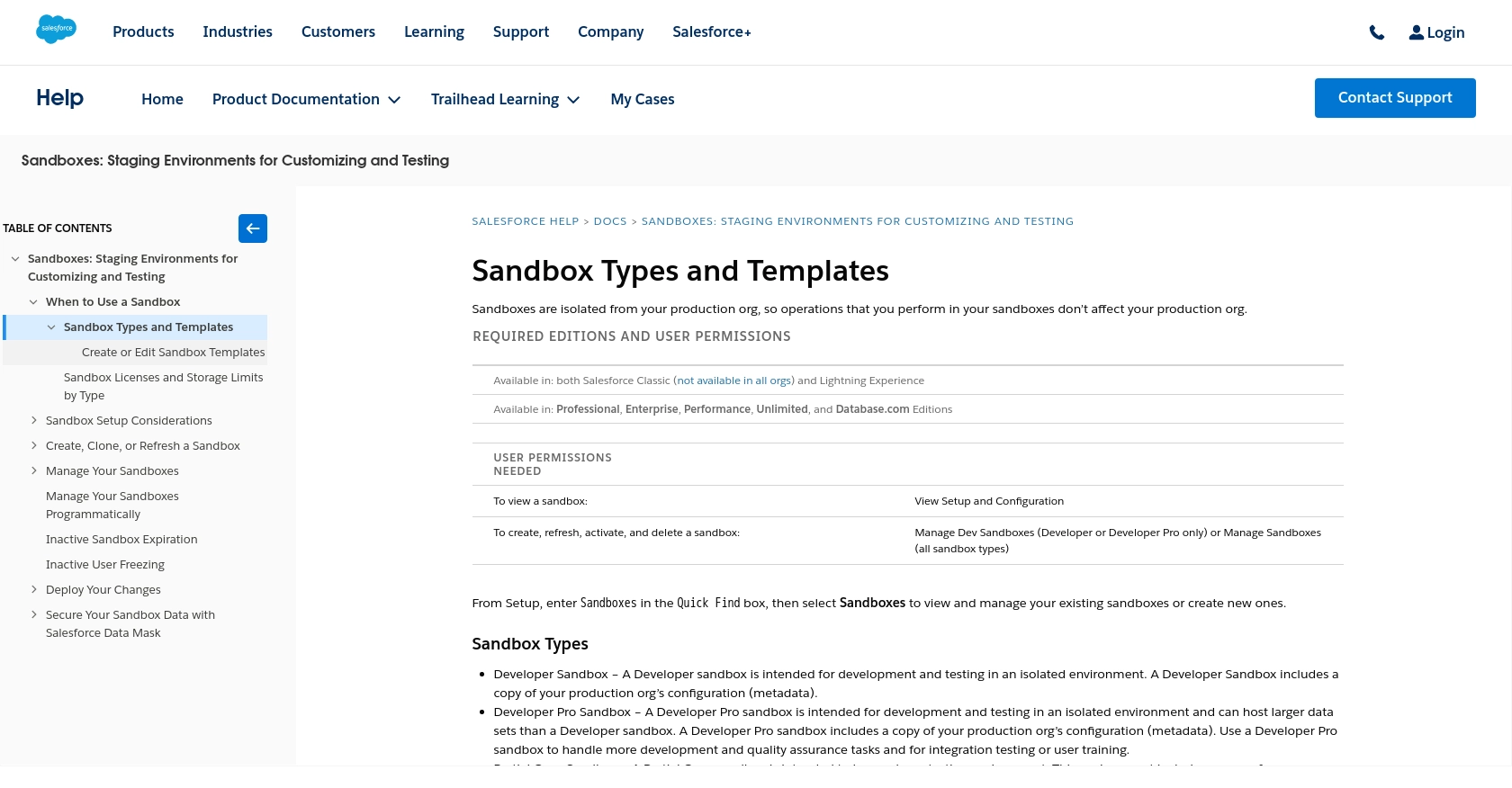
sbb-itb-96038d7
Making API Calls to Salesforce Sandbox Using JavaScript
To interact with the Salesforce Sandbox API using JavaScript, you'll need to set up your environment and write code to authenticate and make requests. This section will guide you through the process, including setting up your JavaScript environment, writing the code to make API calls, and handling responses and errors effectively.
Setting Up Your JavaScript Environment for Salesforce Sandbox API
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides a runtime environment for executing JavaScript code outside a browser. You can download it from the official Node.js website.
Once Node.js is installed, you can use npm (Node Package Manager) to install the necessary dependencies. Open your terminal and run the following command to install the axios library, which will help you make HTTP requests:
npm install axios
Writing JavaScript Code to Authenticate and Fetch Records from Salesforce Sandbox
With your environment set up, you can now write the JavaScript code to authenticate with Salesforce Sandbox and fetch records. Here's a step-by-step guide:
const axios = require('axios');
// Define your Salesforce Sandbox credentials
const clientId = 'Your_Consumer_Key';
const clientSecret = 'Your_Consumer_Secret';
const username = 'Your_Sandbox_Username';
const password = 'Your_Sandbox_Password';
const tokenUrl = 'https://test.salesforce.com/services/oauth2/token';
// Function to authenticate and get an access token
async function getAccessToken() {
try {
const response = await axios.post(tokenUrl, null, {
params: {
grant_type: 'password',
client_id: clientId,
client_secret: clientSecret,
username: username,
password: password
}
});
return response.data.access_token;
} catch (error) {
console.error('Error fetching access token:', error.response.data);
}
}
// Function to fetch records from Salesforce Sandbox
async function fetchRecords() {
const accessToken = await getAccessToken();
if (!accessToken) return;
const instanceUrl = 'https://yourInstance.salesforce.com'; // Replace with your instance URL
const recordsUrl = `${instanceUrl}/services/data/vXX.X/sobjects/Your_Object_Name/`;
try {
const response = await axios.get(recordsUrl, {
headers: {
Authorization: `Bearer ${accessToken}`
}
});
console.log('Records:', response.data);
} catch (error) {
console.error('Error fetching records:', error.response.data);
}
}
// Execute the function to fetch records
fetchRecords();
Replace Your_Consumer_Key
, Your_Consumer_Secret
, Your_Sandbox_Username
, Your_Sandbox_Password
, and Your_Object_Name
with your actual Salesforce Sandbox credentials and the object you wish to query.
Verifying API Call Success and Handling Errors
After running the script, you should see the fetched records printed in your terminal. To verify the success of your API call, check the Salesforce Sandbox for the records you retrieved. If the call fails, the error message will be logged to the console, providing insights into what went wrong.
Common error codes include:
- 400 Bad Request: Check your request parameters and syntax.
- 401 Unauthorized: Verify your authentication credentials.
- 403 Forbidden: Ensure you have the necessary permissions.
For more detailed error handling, refer to the Salesforce API documentation.
Best Practices for Salesforce Sandbox API Integration
Successfully integrating with the Salesforce Sandbox API requires attention to best practices that ensure security, efficiency, and maintainability. Here are some key considerations:
- Securely Store Credentials: Always store your Salesforce credentials, such as the Consumer Key and Secret, in a secure location. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limiting: Salesforce imposes API rate limits to ensure fair usage. Be mindful of these limits and implement retry logic to handle rate limit errors gracefully. For more information on rate limits, refer to the Salesforce API documentation.
- Standardize Data Fields: When synchronizing data between Salesforce and other systems, ensure that data fields are standardized to maintain consistency and avoid data mismatches.
Leverage Endgrate for Streamlined Integration
Building and maintaining integrations with multiple platforms can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Salesforce Sandbox.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product development while Endgrate handles the integration complexities.
- Build Once, Use Everywhere: Develop a single integration for multiple platforms, reducing redundancy and effort.
- Enhance Customer Experience: Provide a seamless and intuitive integration experience for your users.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/salesforce-sandbox
- https://help.salesforce.com/s/articleView?id=sf.create_test_instance.htm&type=5
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/intro_oauth_and_connected_apps.htm
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/quickstart_dev_org.htm
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/resources_list.htm
Ready to get started?