How to Get Records with the Google Sheets API in PHP
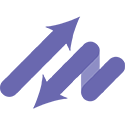
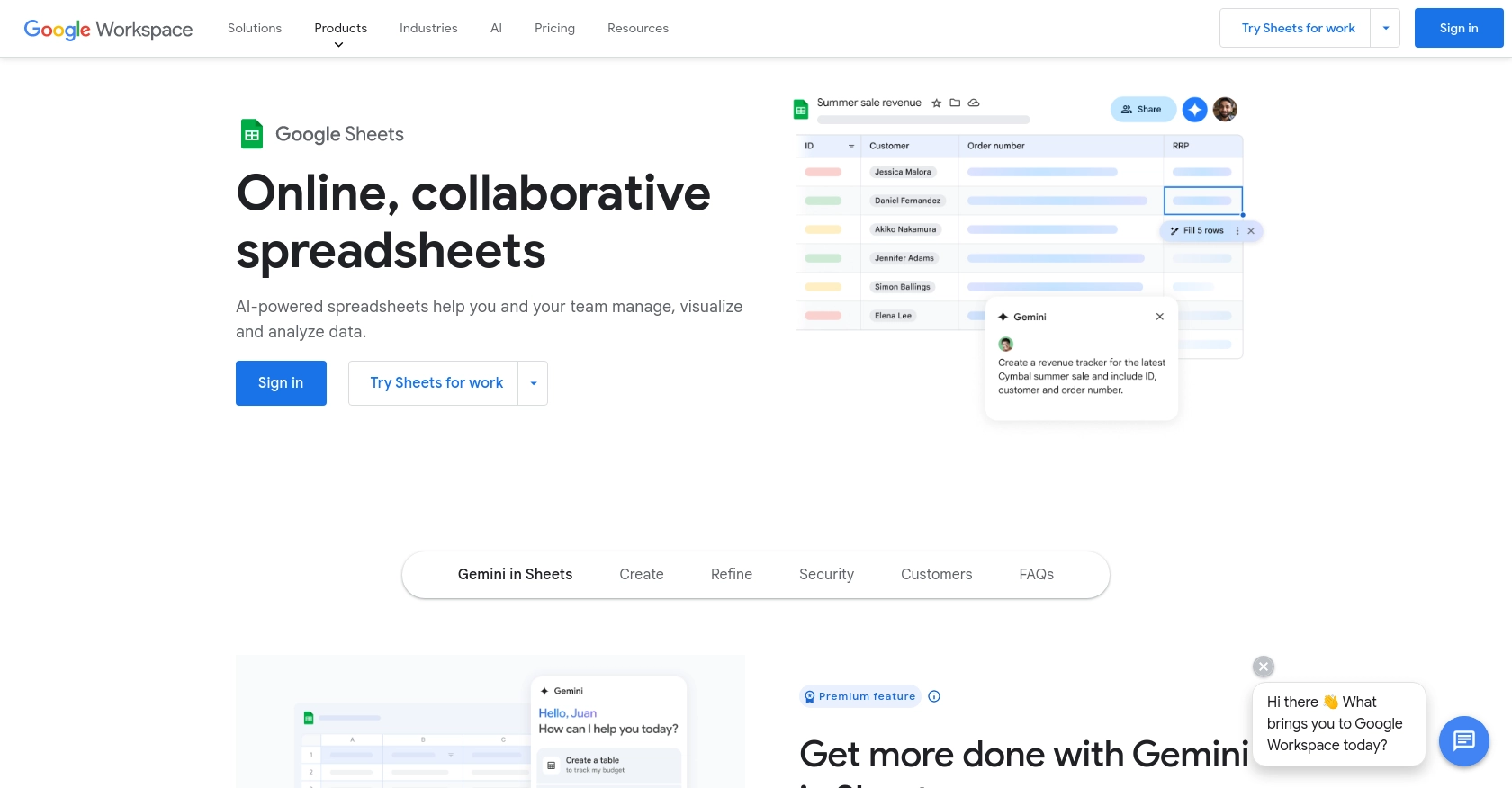
Introduction to Google Sheets API
Google Sheets is a powerful cloud-based spreadsheet application that allows users to create, edit, and collaborate on spreadsheets in real-time. It is part of the Google Workspace suite and is widely used for data analysis, project management, and reporting.
Integrating with the Google Sheets API enables developers to programmatically interact with Google Sheets, allowing for automation of data retrieval and manipulation. For example, a developer might use the Google Sheets API to fetch sales data from a shared spreadsheet and generate reports automatically, saving time and reducing manual errors.
Setting Up Your Google Sheets API Test Account
Before you can start interacting with the Google Sheets API using PHP, you'll need to set up a Google Cloud project and configure OAuth 2.0 authentication. This process will allow your application to securely access Google Sheets on behalf of users.
Create a Google Cloud Project for Google Sheets API
- Go to the Google Cloud Console and sign in with your Google account.
- Navigate to Menu > IAM & Admin > Create a Project.
- Enter a descriptive name for your project and click Create.
Enable Google Sheets API
- In the Google Cloud Console, go to Menu > APIs & Services > Library.
- Search for "Google Sheets API" and click on it.
- Click the Enable button to activate the API for your project.
Configure OAuth Consent Screen for Google Sheets API
- In the Google Cloud Console, navigate to Menu > APIs & Services > OAuth consent screen.
- Select the user type for your app and click Create.
- Fill out the required fields, such as application name and support email, then click Save and Continue.
Create OAuth 2.0 Credentials for Google Sheets API
- Go to Menu > APIs & Services > Credentials in the Google Cloud Console.
- Click Create Credentials > OAuth client ID.
- Select Web application as the application type.
- Enter a name for the credential and add authorized redirect URIs related to your app.
- Click Create to generate your Client ID and Client Secret.
Make sure to securely store your Client ID and Client Secret, as you will need them to authenticate your API requests.
For more detailed instructions, refer to the official Google documentation: Create a Google Cloud project, Enable Google Workspace APIs, Configure OAuth consent, and Create access credentials.
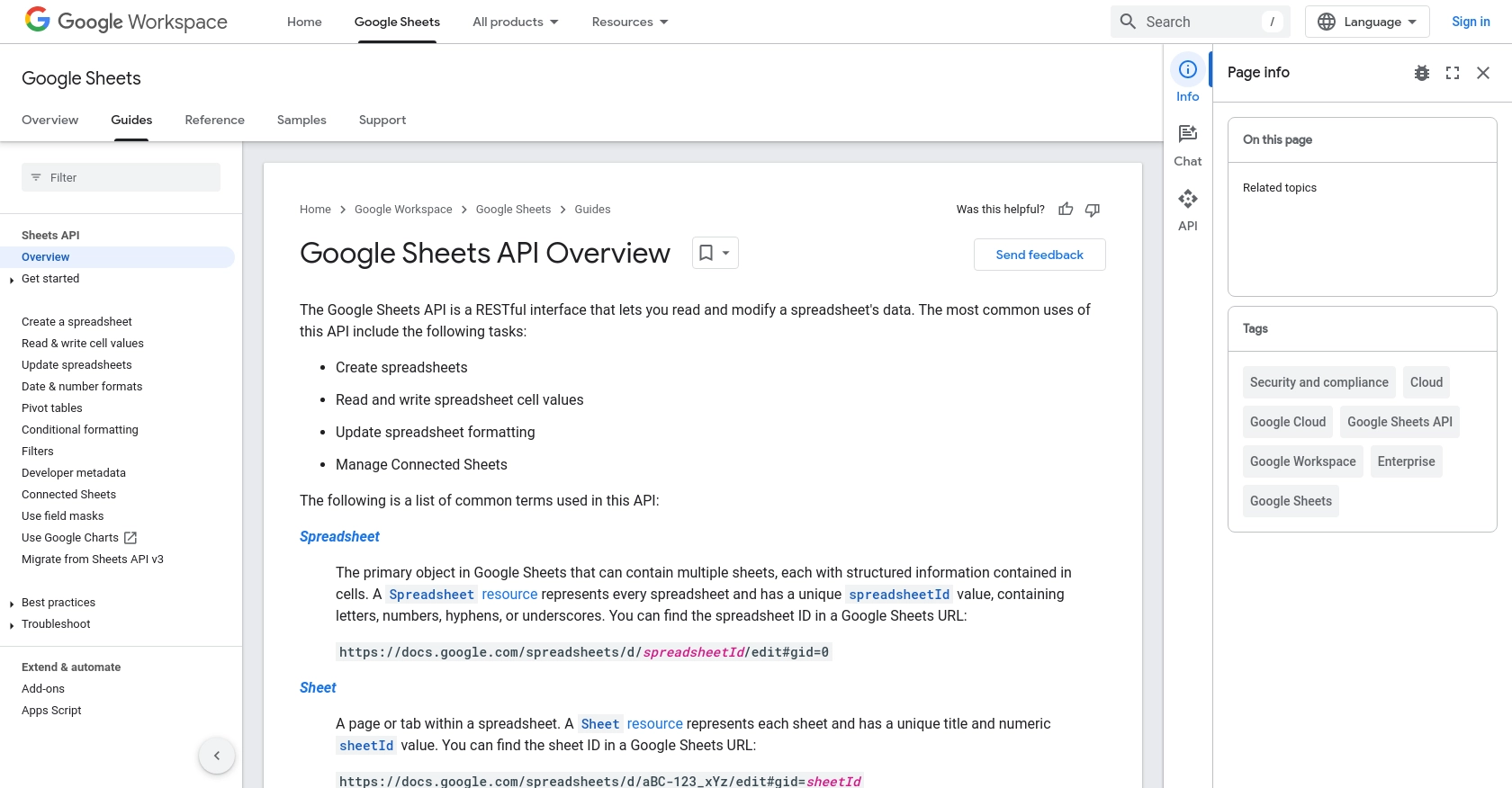
sbb-itb-96038d7
Making API Calls to Retrieve Records from Google Sheets Using PHP
To interact with the Google Sheets API using PHP, you'll need to set up your development environment and write code to authenticate and make requests. This section will guide you through the process of fetching records from a Google Sheet.
Setting Up Your PHP Environment for Google Sheets API
Before making API calls, ensure you have PHP installed on your machine. This tutorial uses PHP 7.4 or later. You'll also need the Composer package manager to install dependencies.
- Install Composer by following the instructions on the official Composer website.
- Create a new directory for your project and navigate to it in your terminal.
- Run the following command to initialize a new Composer project:
composer init
Follow the prompts to set up your project.
Installing Google Client Library for PHP
To interact with the Google Sheets API, you'll need the Google Client Library for PHP. Install it using Composer:
composer require google/apiclient:^2.0
Writing PHP Code to Fetch Records from Google Sheets
Now that your environment is set up, you can write the PHP code to authenticate and fetch records from a Google Sheet. Create a new PHP file, for example, get_google_sheets_records.php
, and add the following code:
require 'vendor/autoload.php';
use Google\Client;
use Google\Service\Sheets;
// Initialize the Google Client
$client = new Client();
$client->setApplicationName('Google Sheets API PHP Quickstart');
$client->setScopes(Sheets::SPREADSHEETS_READONLY);
$client->setAuthConfig('credentials.json');
$client->setAccessType('offline');
// Initialize the Sheets service
$service = new Sheets($client);
// Define the spreadsheet ID and range
$spreadsheetId = 'your_spreadsheet_id';
$range = 'Sheet1!A1:D10';
// Fetch the records
$response = $service->spreadsheets_values->get($spreadsheetId, $range);
$values = $response->getValues();
// Display the records
if (empty($values)) {
echo "No data found.\n";
} else {
foreach ($values as $row) {
echo implode(", ", $row) . "\n";
}
}
Replace your_spreadsheet_id
with the actual ID of your Google Sheet. You can find this ID in the URL of your Google Sheet.
Running the PHP Script to Retrieve Google Sheets Data
To run the script, execute the following command in your terminal:
php get_google_sheets_records.php
If successful, the script will output the data from the specified range in your Google Sheet.
Handling Errors and Verifying API Requests
It's important to handle potential errors when making API requests. The Google Sheets API may return various error codes, such as 400 for bad requests or 403 for permission errors. You can handle these errors using try-catch blocks in PHP.
Verify that your request succeeded by checking the output in your terminal and ensuring it matches the data in your Google Sheet. If you encounter errors, refer to the Google Sheets API documentation for troubleshooting tips.
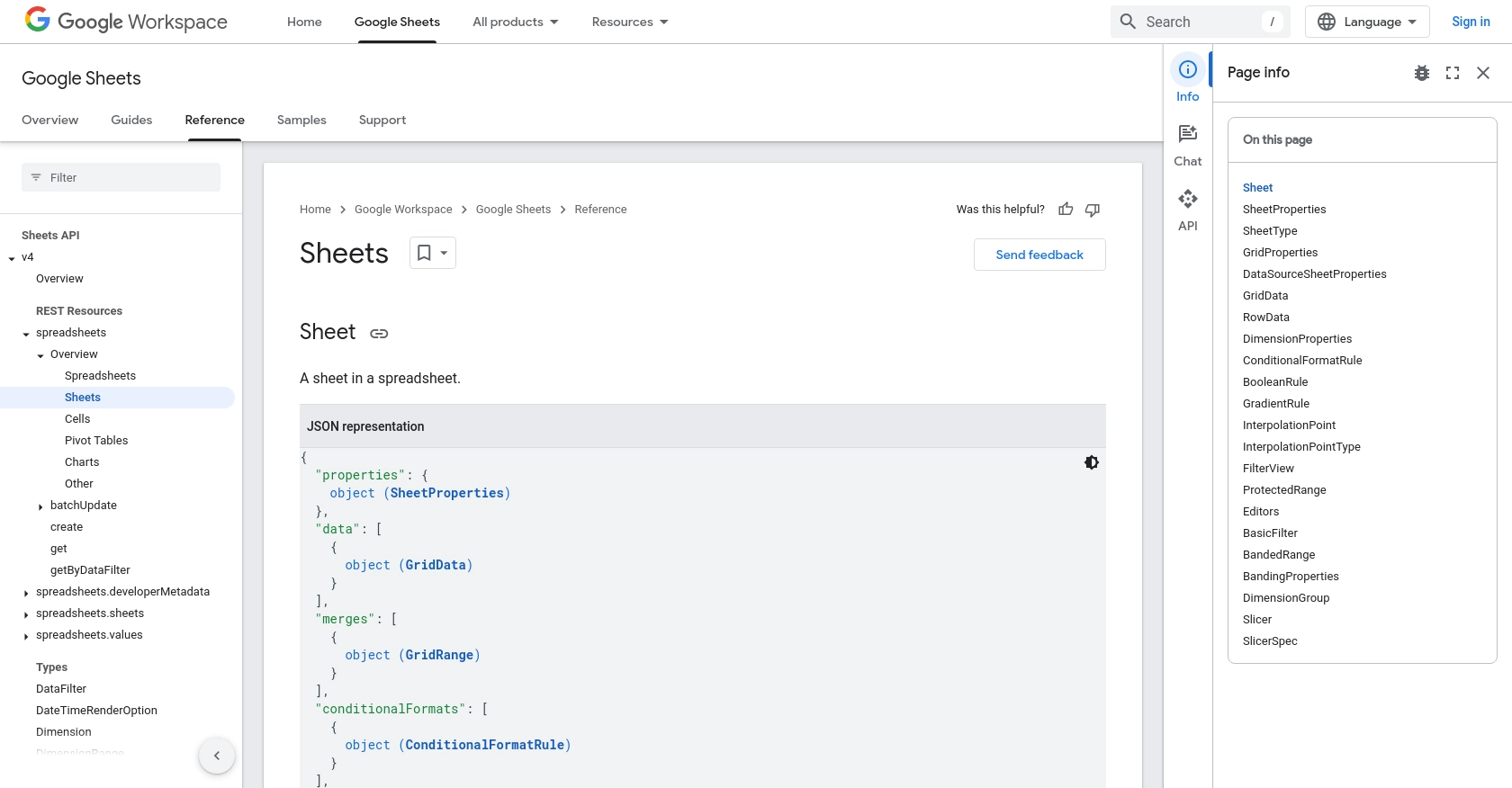
Conclusion and Best Practices for Using Google Sheets API with PHP
Integrating with the Google Sheets API using PHP can significantly enhance your application's ability to manage and manipulate spreadsheet data programmatically. By following the steps outlined in this guide, you can set up a secure connection to Google Sheets, retrieve data efficiently, and handle potential errors gracefully.
Best Practices for Secure and Efficient Google Sheets API Integration
- Securely Store Credentials: Always keep your OAuth credentials, such as Client ID and Client Secret, secure. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle API Rate Limits: Be mindful of the Google Sheets API rate limits to avoid exceeding them. Implement exponential backoff strategies for retrying requests when you encounter rate limit errors.
- Optimize Data Requests: Minimize the amount of data you request by specifying only the necessary ranges and fields. This will improve performance and reduce the load on the API.
- Implement Error Handling: Use try-catch blocks to manage exceptions and handle different error codes appropriately. This ensures your application can respond to issues without crashing.
- Regularly Review API Documentation: Stay updated with the latest changes and best practices by regularly reviewing the Google Sheets API documentation.
Streamline Your Integration Process with Endgrate
While integrating with Google Sheets API can be straightforward, managing multiple integrations across different platforms can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Save time and resources by outsourcing integrations and ensuring a seamless connection with Google Sheets and other platforms.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/googlesheets
- https://developers.google.com/sheets/api/guides/concepts
- https://developers.google.com/sheets/api/reference/rest
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/sheets/api/reference/rest/v4/spreadsheets/sheets
- https://developers.google.com/sheets/api/reference/rest/v4/spreadsheets/cells
Ready to get started?