How to Get Users with the Insightly API in PHP
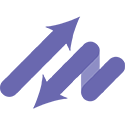
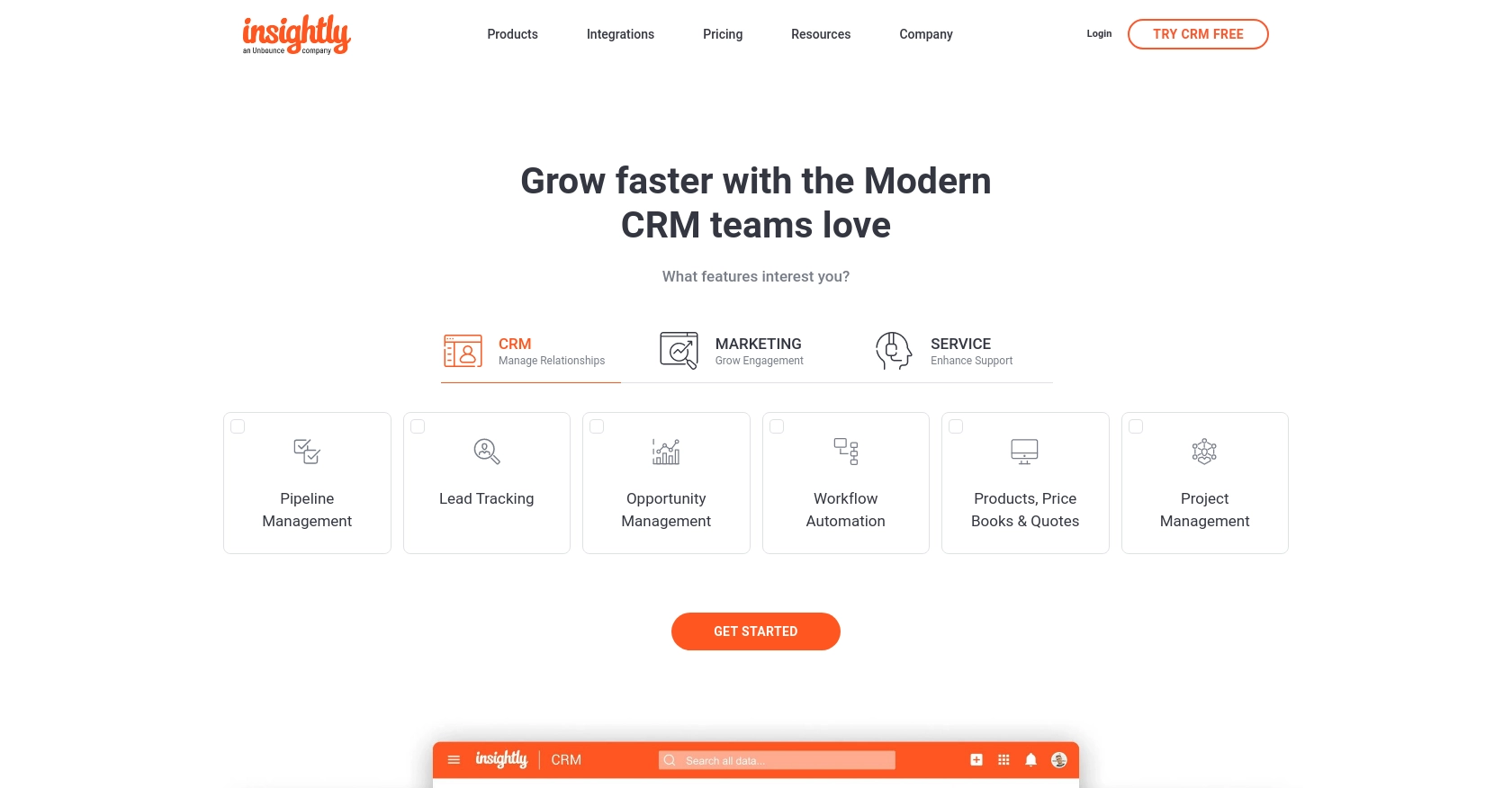
Introduction to Insightly CRM
Insightly is a powerful CRM platform designed to help businesses manage customer relationships and streamline their sales processes. With its robust set of features, Insightly enables companies to track leads, manage projects, and automate workflows, making it a popular choice for businesses looking to enhance their CRM capabilities.
Integrating with the Insightly API allows developers to access and manipulate data within the platform, providing opportunities for automation and customization. For example, a developer might use the Insightly API to retrieve user data and integrate it with other business applications, enhancing data consistency and operational efficiency.
Setting Up Your Insightly Test Account
Before you can start interacting with the Insightly API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Creating an Insightly Account
If you don't already have an Insightly account, you can sign up for a free trial on the Insightly website. Follow the instructions to create your account, and once completed, log in to access your dashboard.
Obtaining Your Insightly API Key
Insightly uses an API key for authentication, which you will need to include in your API requests. Follow these steps to obtain your API key:
- Log in to your Insightly account.
- Navigate to your user profile in the upper right corner and select User Settings.
- Scroll down to the API Key and URL section.
- Copy your API key. You will use this key in your API requests to authenticate your calls.
For more details on finding your API key, refer to the Insightly support article.
Configuring Your Test Environment
With your API key in hand, you can now configure your test environment. Ensure you have PHP installed on your machine, along with any necessary dependencies for making HTTP requests, such as cURL.
Testing API Calls in the Sandbox
Insightly provides a sandbox environment for testing API calls. You can use your API key directly in the sandbox without Base64 encoding. This allows you to test and debug your API interactions safely.
For more information on using the Insightly API, visit the Insightly API documentation.
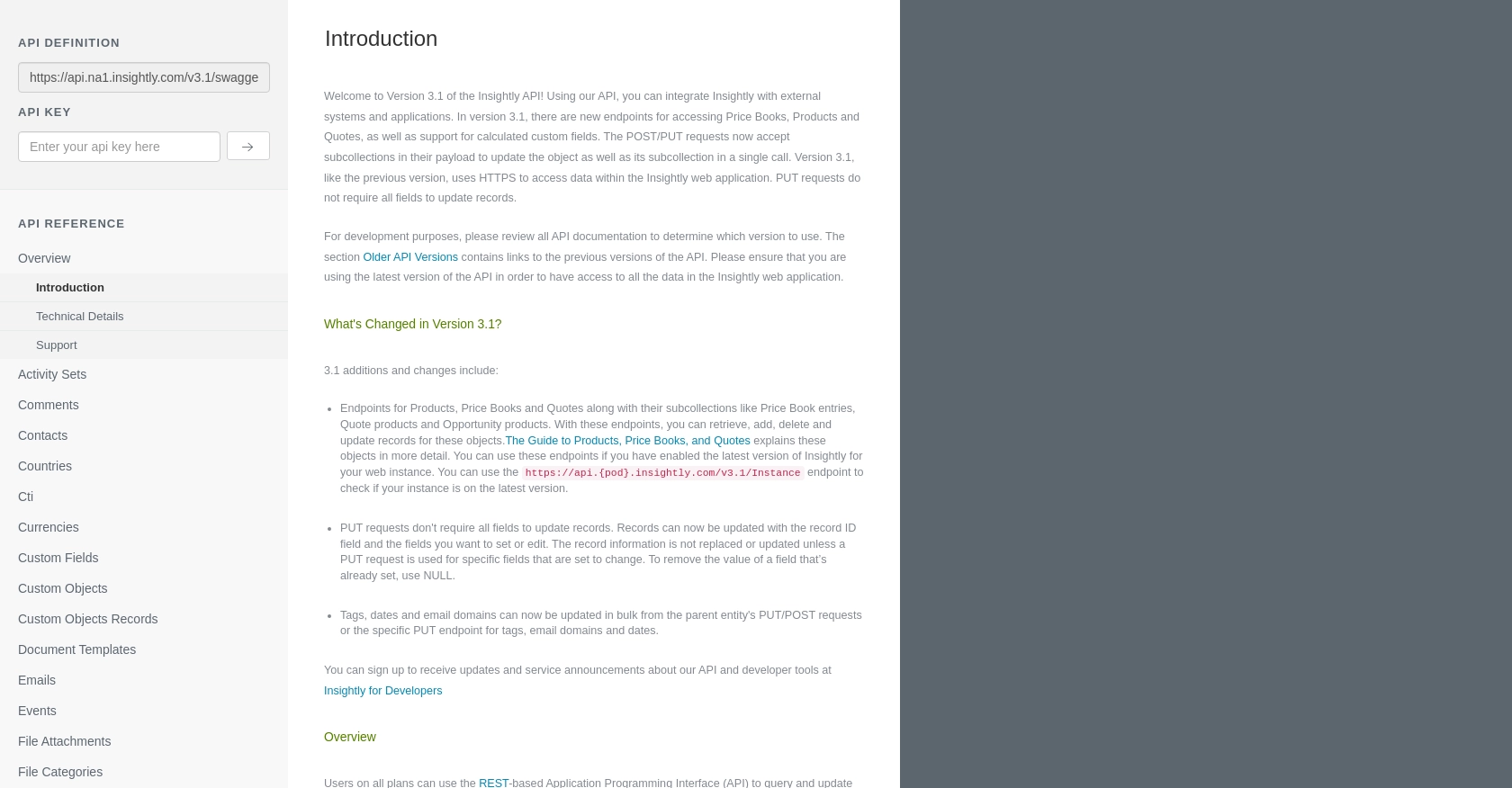
sbb-itb-96038d7
Setting Up PHP Environment for Insightly API Integration
Before making API calls to Insightly, ensure your PHP environment is properly configured. You'll need PHP installed on your machine, along with the cURL extension to handle HTTP requests. Verify your PHP version is 7.4 or higher for compatibility with modern libraries.
Installing Necessary PHP Dependencies
To interact with the Insightly API, you'll use PHP's cURL extension. Ensure it's enabled in your php.ini
file. You can check this by running the following command in your terminal:
php -m | grep curl
If cURL is not listed, enable it by uncommenting the line extension=curl
in your php.ini
file and restart your web server.
Making API Calls to Retrieve Users from Insightly
With your environment set up, you can now make API calls to retrieve user data from Insightly. The following example demonstrates how to use PHP and cURL to fetch users.
Example Code to Fetch Users from Insightly API
Create a new PHP file, get_insightly_users.php
, and add the following code:
<?php
// Set your Insightly API key
$apiKey = 'your_api_key_here';
// Set the API endpoint for retrieving users
$endpoint = 'https://api.na1.insightly.com/v3.1/Users';
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Basic ' . base64_encode($apiKey . ':')
]);
// Execute the cURL request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
// Decode the JSON response
$users = json_decode($response, true);
// Display user information
foreach ($users as $user) {
echo 'User ID: ' . $user['USER_ID'] . '<br>';
echo 'Name: ' . $user['FIRST_NAME'] . ' ' . $user['LAST_NAME'] . '<br>';
echo 'Email: ' . $user['EMAIL_ADDRESS'] . '<br><br>';
}
}
// Close cURL session
curl_close($ch);
?>
Replace your_api_key_here
with your actual Insightly API key. This script initializes a cURL session, sets the necessary headers for authentication, and retrieves user data from Insightly.
Running the PHP Script and Verifying Output
Run the script from your terminal or command line:
php get_insightly_users.php
You should see a list of users with their IDs, names, and email addresses. Verify the output by checking the users in your Insightly dashboard.
Handling Errors and Insightly API Rate Limits
When making API calls, handle potential errors by checking the response status and error messages. Insightly's API has rate limits of 10 requests per second and daily limits based on your plan. If you exceed these limits, you'll receive a 429 status code. Implement retry logic or backoff strategies to manage rate limiting effectively.
For more details on rate limits, refer to the Insightly API documentation.
Best Practices for Insightly API Integration
When working with the Insightly API, it's essential to follow best practices to ensure secure and efficient integration. Here are some recommendations:
- Securely Store API Keys: Always store your API keys securely in environment variables or a secure vault. Avoid hardcoding them in your source code.
- Handle Rate Limits: Be mindful of Insightly's rate limits. Implement retry logic with exponential backoff to handle 429 status codes gracefully.
- Data Transformation: Standardize and transform data fields as needed to maintain consistency across your applications.
- Error Handling: Implement robust error handling to manage API errors and ensure your application can recover gracefully.
Enhancing Integration Efficiency with Endgrate
Integrating multiple platforms can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Insightly. This allows you to focus on your core product while outsourcing integration complexities.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Save time and resources by letting Endgrate handle the heavy lifting of integrations.
Explore how Endgrate can streamline your integration processes by visiting Endgrate's website.
Read More
Ready to get started?