How to Get Messages with the Re:amaze API in Python
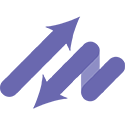
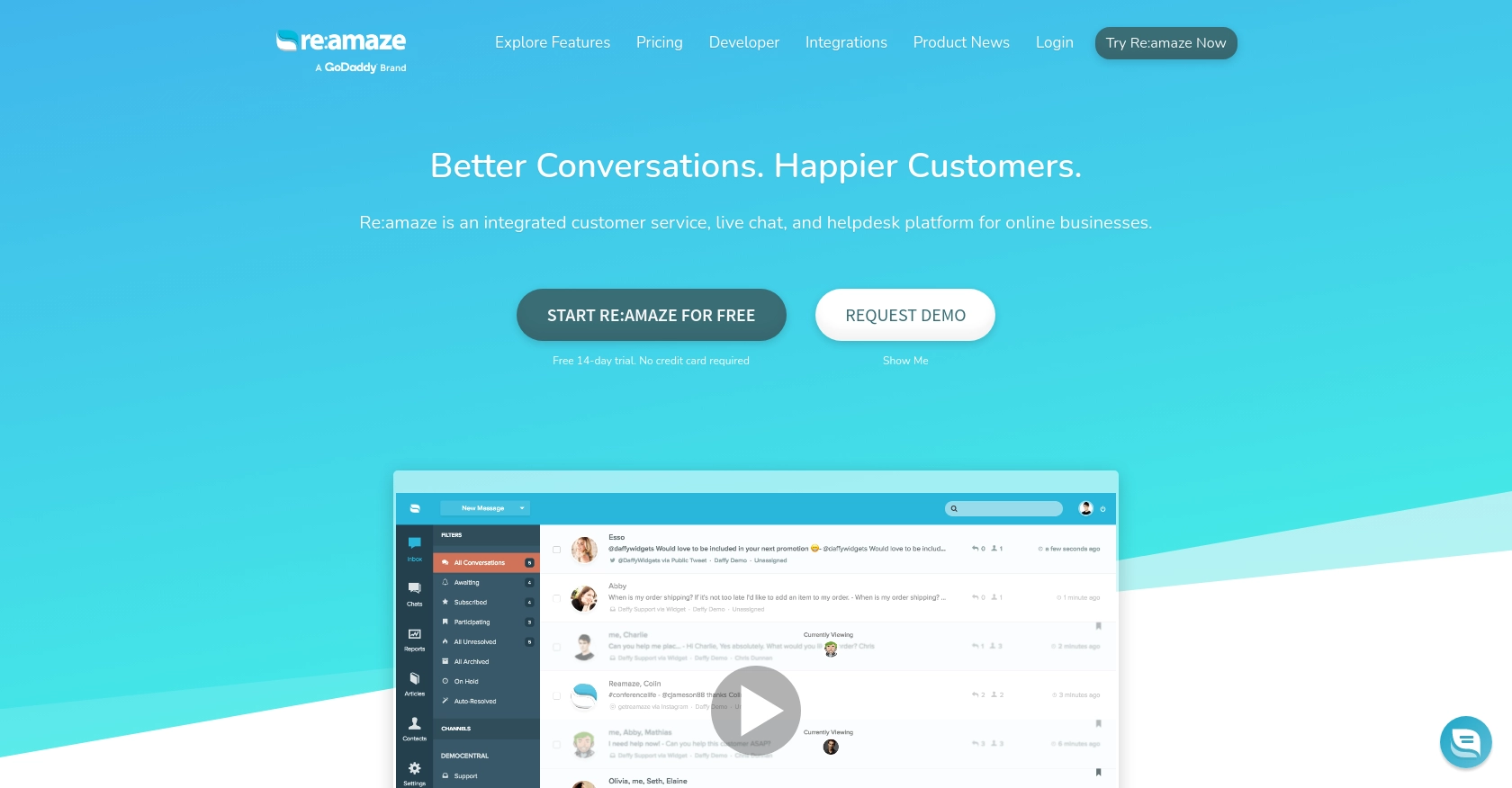
Introduction to Re:amaze API
Re:amaze is a versatile customer support platform that offers a unified inbox for managing customer interactions across various channels. It provides tools for live chat, chatbots, email, and social media, making it a comprehensive solution for businesses looking to enhance their customer service experience.
Integrating with the Re:amaze API allows developers to programmatically access and manage customer messages, enabling automation and improved workflow efficiency. For example, a developer might use the Re:amaze API to retrieve messages from customer conversations and analyze them for sentiment or categorize them for better support prioritization.
Setting Up Your Re:amaze Account for API Access
Before you can start interacting with the Re:amaze API, you need to set up your account to obtain the necessary credentials. This involves creating an API token that will allow you to authenticate your requests securely.
Creating a Re:amaze Account
If you don't already have a Re:amaze account, you can sign up for a free account on the Re:amaze website. Follow the instructions to complete the registration process. Once your account is created, log in to access your dashboard.
Generating an API Token in Re:amaze
To interact with the Re:amaze API, you'll need an API token. Follow these steps to generate one:
- Log in to your Re:amaze account and navigate to the Settings section.
- Under the Developer menu, click on API Token.
- Click on Generate New Token to create a unique API token.
- Copy the generated token and store it securely, as you'll need it to authenticate your API requests.
Understanding Re:amaze API Authentication
The Re:amaze API uses HTTP Basic Authentication. This means you'll need to include your login email and API token in the request headers. Ensure that all requests are made over HTTPS to maintain security.
import requests
# Define your Re:amaze brand and credentials
brand = "your_brand"
login_email = "your_email@example.com"
api_token = "your_api_token"
# Set the API endpoint
endpoint = f"https://{brand}.reamaze.io/api/v1/messages"
# Make a GET request with authentication
response = requests.get(endpoint, auth=(login_email, api_token), headers={"Accept": "application/json"})
# Check the response
if response.status_code == 200:
print("Successfully authenticated and retrieved messages.")
else:
print("Failed to authenticate. Check your credentials.")
Replace your_brand
, your_email@example.com
, and your_api_token
with your actual Re:amaze brand, email, and API token.
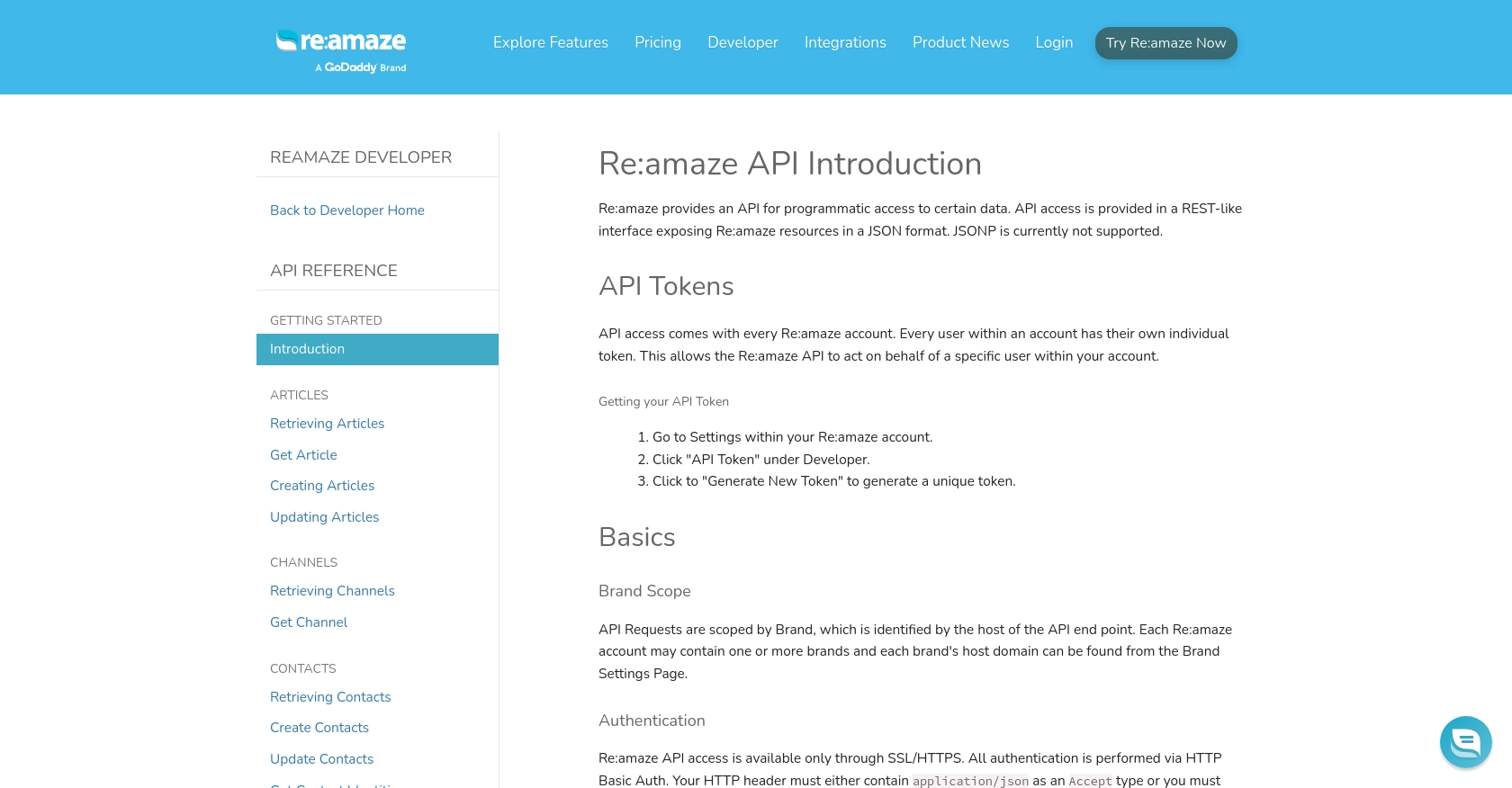
sbb-itb-96038d7
Making API Calls to Retrieve Messages with Re:amaze API in Python
To effectively interact with the Re:amaze API and retrieve messages, you'll need to set up your Python environment and make authenticated requests. This section will guide you through the necessary steps, including setting up Python, installing dependencies, and executing the API call to fetch messages.
Setting Up Your Python Environment for Re:amaze API
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python 3.11.1 from the official Python website if you haven't already.
- Use the following command to install the
requests
library:
pip install requests
Executing the Re:amaze API Call to Retrieve Messages
With your environment set up, you can now proceed to make the API call to retrieve messages from Re:amaze. Follow the steps below to create a Python script that performs this task.
import requests
# Define your Re:amaze brand and credentials
brand = "your_brand"
login_email = "your_email@example.com"
api_token = "your_api_token"
# Set the API endpoint for retrieving messages
endpoint = f"https://{brand}.reamaze.io/api/v1/messages"
# Make a GET request with authentication
response = requests.get(endpoint, auth=(login_email, api_token), headers={"Accept": "application/json"})
# Check the response status
if response.status_code == 200:
messages = response.json().get("messages", [])
for message in messages:
print(f"Message: {message['body']}, From: {message['user']['name']}")
else:
print(f"Failed to retrieve messages. Status code: {response.status_code}")
Replace your_brand
, your_email@example.com
, and your_api_token
with your actual Re:amaze brand, email, and API token. This script makes a GET request to the Re:amaze API to fetch messages and prints each message's body and sender's name.
Verifying Successful API Requests in Re:amaze
After running the script, verify the retrieved messages by checking your Re:amaze dashboard. The messages printed by the script should match those in your Re:amaze account.
Handling Errors and Understanding Response Codes
It's crucial to handle potential errors when making API calls. The Re:amaze API may return various HTTP status codes indicating the result of your request:
- 200 OK: The request was successful, and messages were retrieved.
- 401 Unauthorized: Authentication failed. Check your email and API token.
- 429 Too Many Requests: Rate limit exceeded. Wait before making more requests.
For more details on error handling, refer to the Re:amaze API documentation.
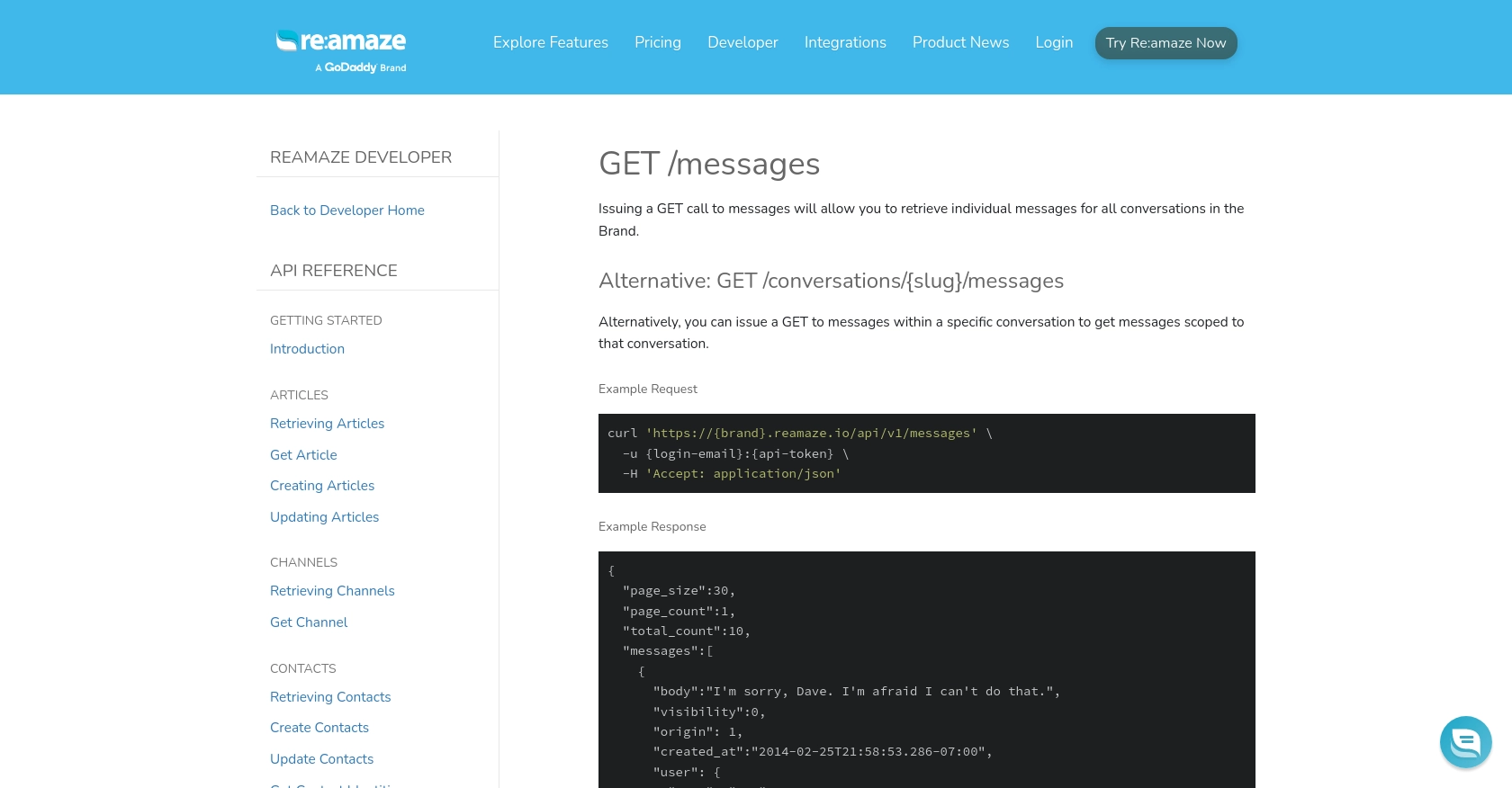
Conclusion and Best Practices for Using Re:amaze API in Python
Integrating with the Re:amaze API allows developers to efficiently manage customer interactions by retrieving and analyzing messages programmatically. This integration can significantly enhance customer support workflows and improve response times.
Best Practices for Secure and Efficient API Integration with Re:amaze
- Secure Storage of Credentials: Always store your API tokens and credentials securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of the Re:amaze API rate limits. Implement logic to handle HTTP 429 responses by pausing requests and retrying after a delay.
- Data Standardization: Ensure that the data retrieved from Re:amaze is standardized and transformed as needed for your application. This can help in maintaining consistency across different systems.
Enhance Your Integration Strategy with Endgrate
While integrating with Re:amaze API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate offers a unified API solution that simplifies this process, allowing you to focus on your core product development.
By using Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Save time and resources by outsourcing integrations, and ensure a seamless experience across all your platforms.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate today.
Read More
Ready to get started?