Using the Quickbooks API to Get Sales Orders (with Javascript examples)
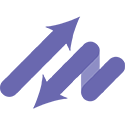
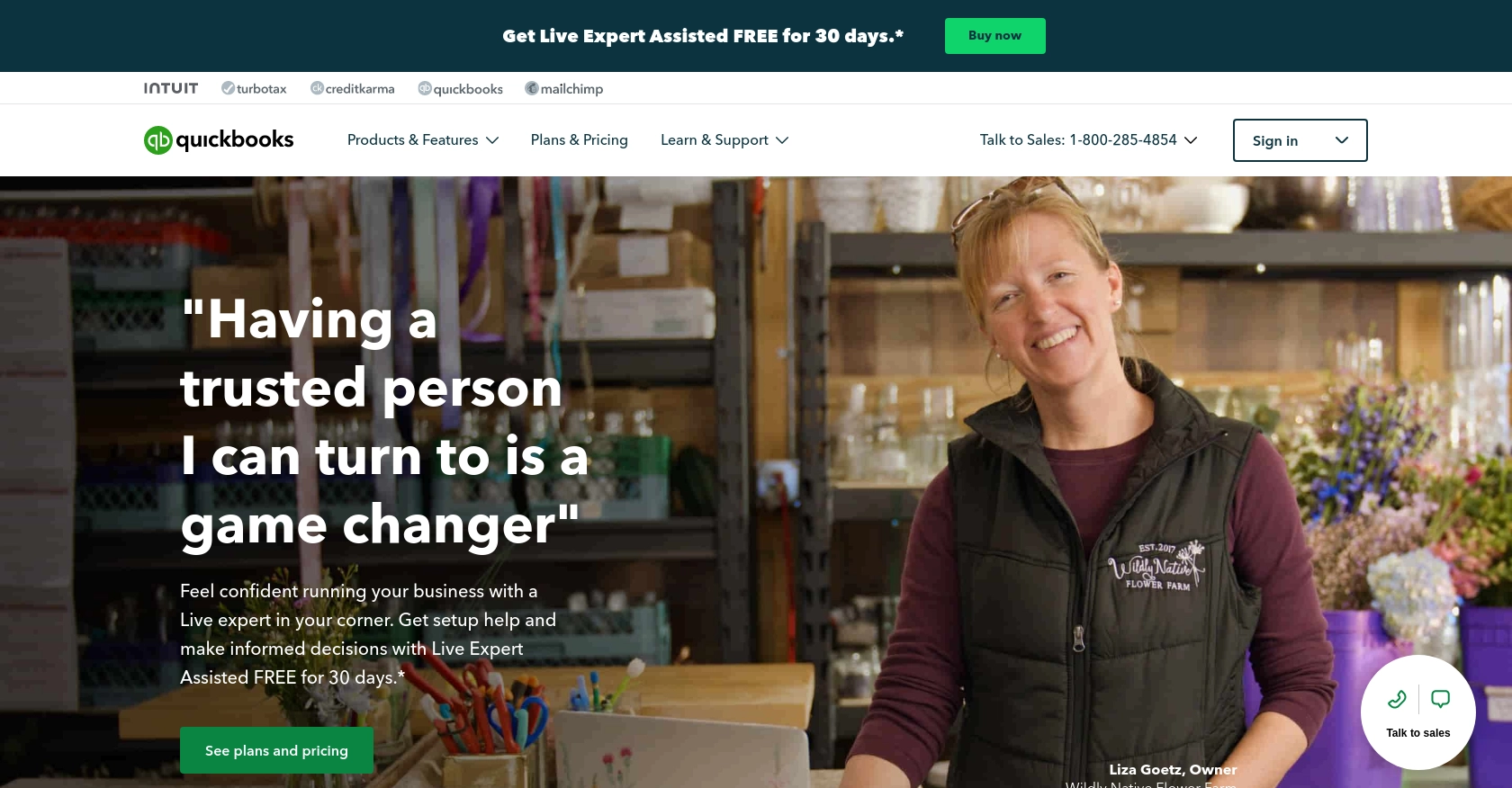
Introduction to QuickBooks API
QuickBooks is a widely-used accounting software that helps businesses manage their finances efficiently. It offers a range of features including invoicing, expense tracking, and financial reporting, making it an essential tool for small to medium-sized enterprises.
Integrating with the QuickBooks API allows developers to automate and streamline financial processes, such as retrieving sales orders. For example, a developer might use the QuickBooks API to automatically fetch sales orders and integrate them into a custom dashboard, providing real-time insights into sales performance.
Setting Up Your QuickBooks Sandbox Account for API Integration
Before you can start interacting with the QuickBooks API, you need to set up a sandbox account. This allows you to test API calls without affecting live data, ensuring a safe environment for development and experimentation.
Creating a QuickBooks Developer Account
To begin, you'll need a QuickBooks Developer account. If you don't have one, follow these steps:
- Visit the Intuit Developer Portal.
- Click on "Sign Up" and fill in the required information to create your developer account.
- Once registered, log in to your account to access the developer dashboard.
Setting Up a QuickBooks Sandbox Company
After creating your developer account, you can set up a sandbox company:
- Navigate to the "Sandbox" section in the developer dashboard.
- Select "Add Sandbox" to create a new sandbox environment.
- Choose the type of company you want to simulate and complete the setup process.
This sandbox company will serve as your testing ground for API interactions.
Generating OAuth Credentials for QuickBooks API
QuickBooks API uses OAuth for authentication. Follow these steps to generate the necessary credentials:
- Go to the App Management section in your developer account.
- Click on "Create an App" and provide the required details.
- Once your app is created, navigate to the "Keys & OAuth" tab.
- Here, you'll find your Client ID and Client Secret. Make sure to store these securely as they are essential for authenticating API requests.
Configuring OAuth Scopes for Access
To ensure your application has the necessary permissions, configure the OAuth scopes:
- In the "Keys & OAuth" tab, scroll down to the "Scopes" section.
- Select the appropriate scopes that your application requires, such as "Sales" for accessing sales orders.
- Save your changes to update the app settings.
With your sandbox account and OAuth credentials set up, you're ready to start making API calls to QuickBooks. This setup ensures that you can securely authenticate and interact with the QuickBooks API in a controlled environment.
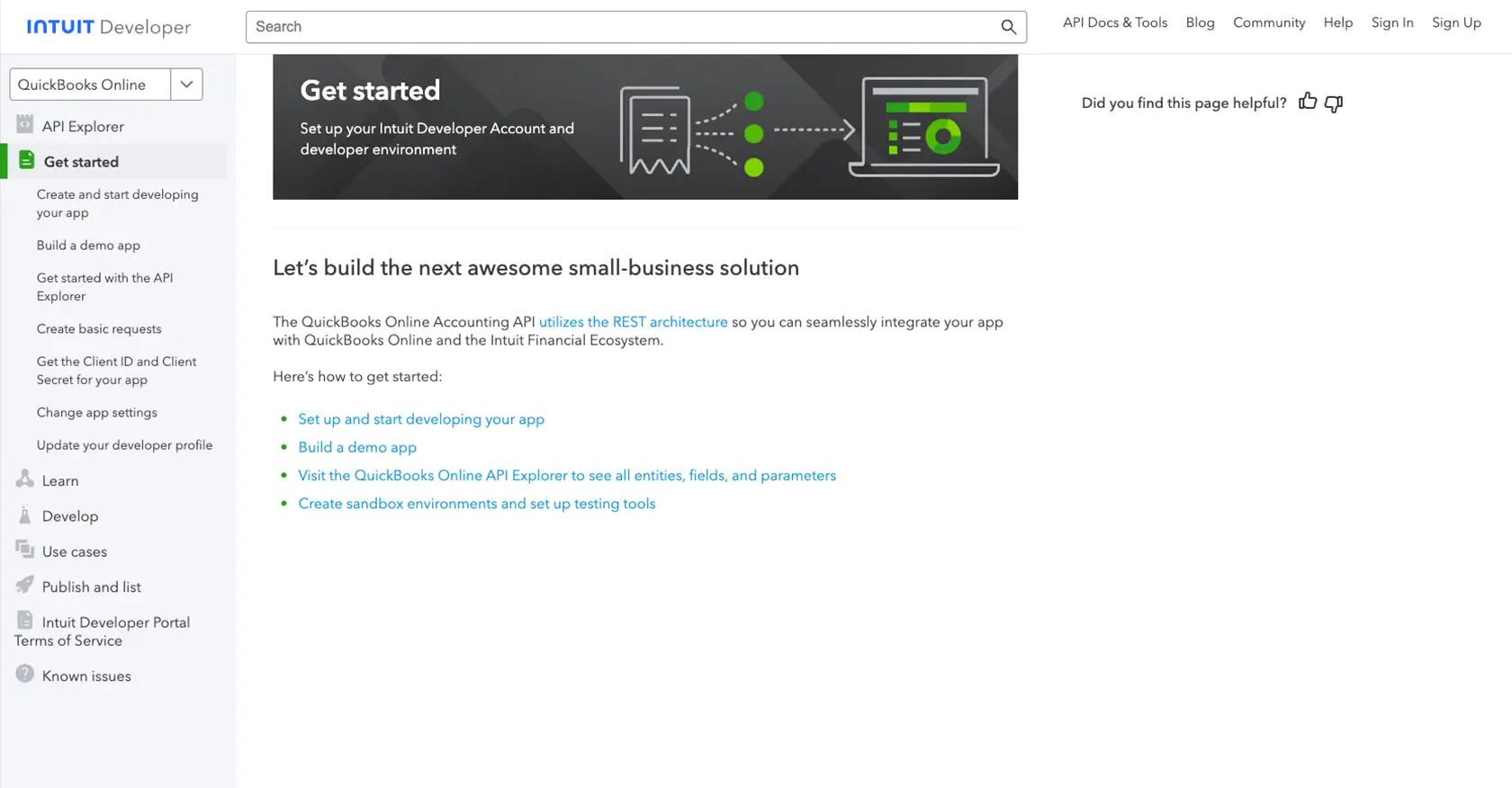
sbb-itb-96038d7
Making API Calls to Retrieve Sales Orders from QuickBooks Using JavaScript
With your QuickBooks sandbox and OAuth credentials ready, you can now proceed to make API calls to retrieve sales orders. This section will guide you through the process using JavaScript, ensuring you have the right setup and code to interact with the QuickBooks API effectively.
Prerequisites for QuickBooks API Integration with JavaScript
Before diving into the code, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A package manager like npm or yarn.
- Familiarity with JavaScript and asynchronous programming.
Installing Required Dependencies
To interact with the QuickBooks API, you'll need to install the axios
library for making HTTP requests. Run the following command in your terminal:
npm install axios
Example Code to Fetch Sales Orders from QuickBooks
Below is a sample JavaScript code snippet to fetch sales orders from QuickBooks:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://sandbox-quickbooks.api.intuit.com/v3/company/YOUR_COMPANY_ID/salesorder';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Accept': 'application/json'
};
// Function to get sales orders
async function getSalesOrders() {
try {
const response = await axios.get(endpoint, { headers });
const salesOrders = response.data.SalesOrder;
console.log('Sales Orders:', salesOrders);
} catch (error) {
console.error('Error fetching sales orders:', error.response ? error.response.data : error.message);
}
}
getSalesOrders();
Replace YOUR_COMPANY_ID
and YOUR_ACCESS_TOKEN
with your actual QuickBooks company ID and access token.
Verifying API Call Success in QuickBooks Sandbox
After running the code, you should see the sales orders printed in your console. To verify the success of the API call, you can cross-check the returned data with the sales orders listed in your QuickBooks sandbox environment.
Handling Errors and Common Error Codes
When making API calls, it's crucial to handle potential errors. The QuickBooks API may return various error codes, such as:
- 401 Unauthorized: Indicates an issue with authentication. Ensure your access token is valid.
- 403 Forbidden: You might not have the necessary permissions. Check your OAuth scopes.
- 500 Internal Server Error: A server-side issue. Try the request again later.
Implement error handling in your code to manage these scenarios gracefully.
For more detailed information on error codes, refer to the Intuit Developer Documentation.
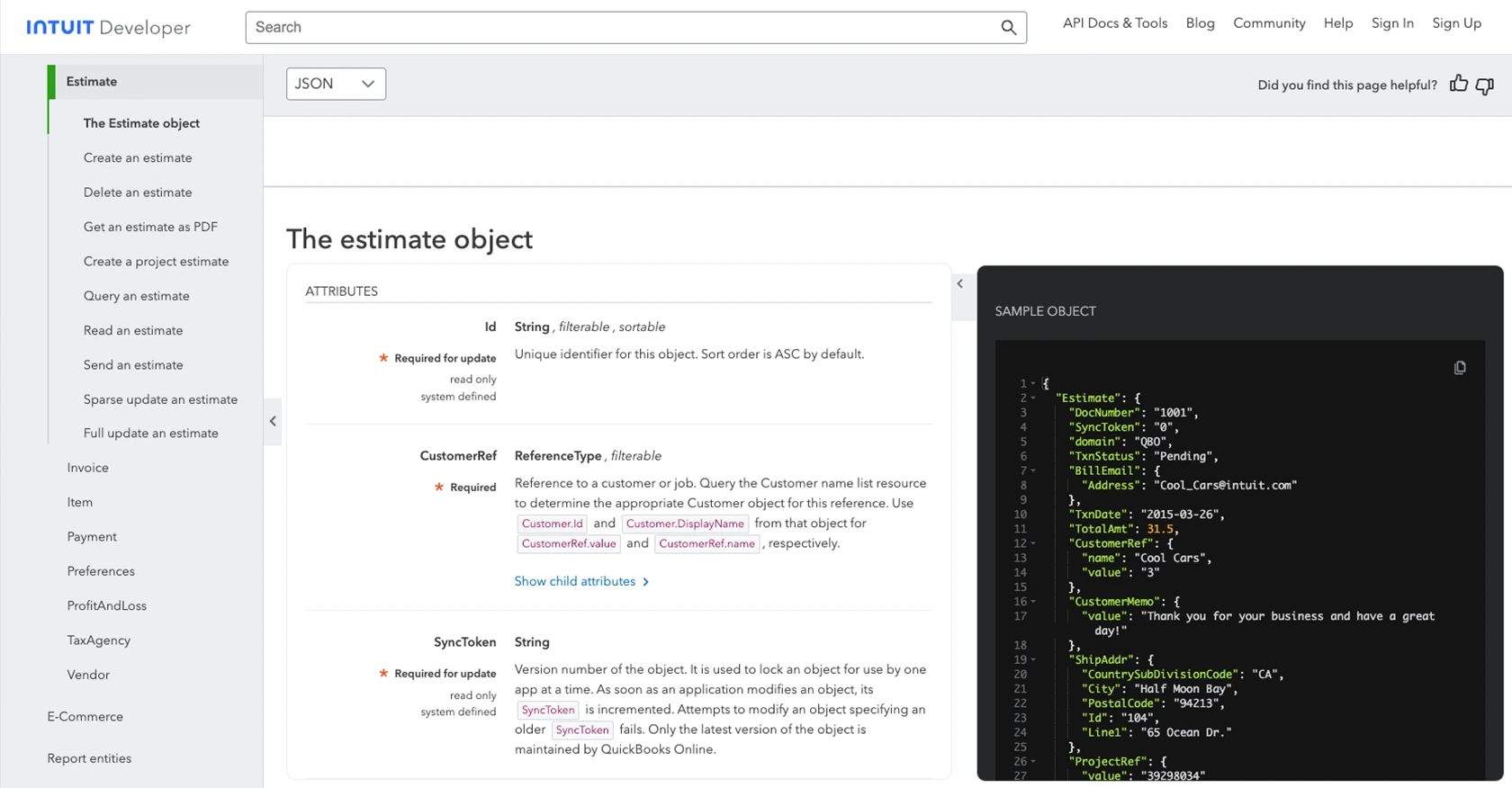
Conclusion: Best Practices for QuickBooks API Integration
Integrating with the QuickBooks API offers a powerful way to automate and enhance your financial processes. By following the steps outlined in this guide, you can efficiently retrieve sales orders using JavaScript, ensuring seamless interaction with QuickBooks.
Storing User Credentials Securely
When working with OAuth credentials, it's crucial to store your Client ID, Client Secret, and access tokens securely. Consider using environment variables or secure vaults to protect sensitive information from unauthorized access.
Handling QuickBooks API Rate Limiting
QuickBooks API enforces rate limits to ensure fair usage. Be mindful of these limits to avoid service disruptions. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
Transforming and Standardizing Data Fields
When integrating sales orders into your application, ensure that data fields are transformed and standardized to match your system's requirements. This practice helps maintain data consistency and integrity across platforms.
Streamlining Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider using Endgrate to simplify the process. Endgrate allows you to build once for each use case, offering a unified API endpoint that connects to various platforms, including QuickBooks. This approach saves time and resources, allowing you to focus on your core product.
By leveraging these best practices, you can optimize your QuickBooks API integration, providing a robust solution for your financial management needs. For more information on how Endgrate can enhance your integration strategy, visit Endgrate.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/most-commonly-used/estimate
Ready to get started?