How to Create Records with the NetHunt API in Javascript
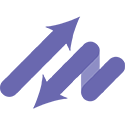
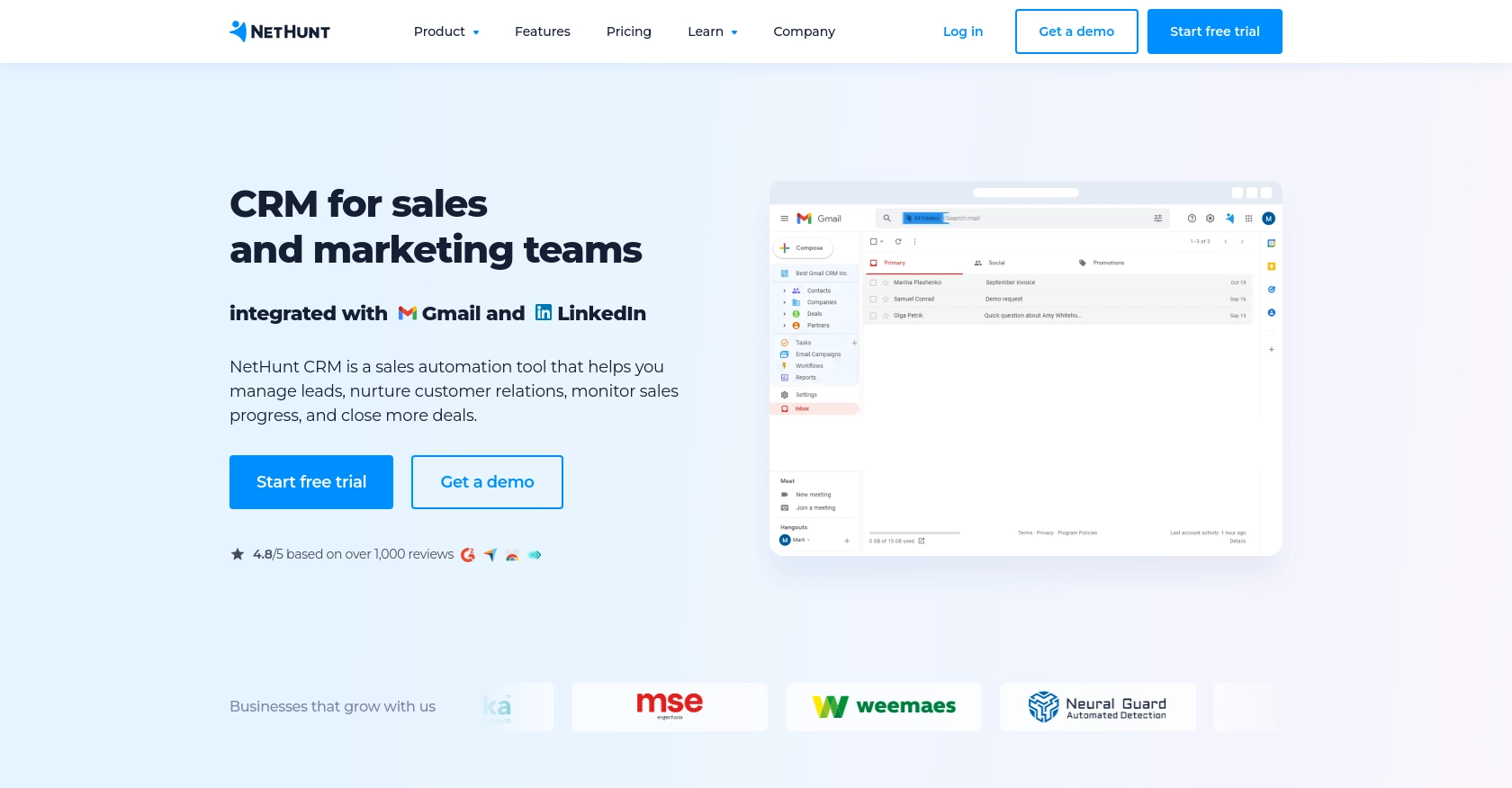
Introduction to NetHunt CRM
NetHunt CRM is a powerful customer relationship management tool that seamlessly integrates with Gmail, providing businesses with an efficient way to manage their customer interactions and sales processes. With features like email campaigns, lead management, and sales automation, NetHunt CRM is designed to enhance productivity and streamline workflows for businesses of all sizes.
Developers may want to integrate with NetHunt's API to automate CRM tasks, such as creating new records directly from external applications. For example, a developer could use the NetHunt API to automatically add new leads from a web form into the CRM, ensuring that sales teams have immediate access to potential customer information.
Setting Up Your NetHunt CRM Account for API Access
Before you can start creating records with the NetHunt API using JavaScript, you need to set up your NetHunt CRM account to access the API. This involves generating an API key, which is essential for authenticating your requests.
Creating a NetHunt CRM Account
If you don't already have a NetHunt CRM account, you can sign up for a free trial on the NetHunt website. This will give you access to the CRM features and allow you to explore the platform's capabilities.
Generating Your NetHunt API Key
To interact with the NetHunt API, you'll need an API key. Follow these steps to generate your API key:
- Log in to your NetHunt CRM account.
- Navigate to the Settings menu.
- Select Integrations from the options available.
- Find the section for API access and click on Generate API Key.
- Copy the generated API key and store it securely, as you'll need it to authenticate your API requests.
For more detailed instructions, you can refer to the NetHunt Help Page.
Understanding NetHunt API Authentication
NetHunt API uses Basic authentication, which requires encoding your email and API key in base64 format. Here's how you can set it up:
// Example of setting up Basic authentication
const email = 'your-email@example.com';
const apiKey = 'your-api-key';
const authString = btoa(`${email}:${apiKey}`); // Base64 encode
const headers = {
'Authorization': `Basic ${authString}`,
'Content-Type': 'application/json'
};
With your API key and authentication headers ready, you can now proceed to make API calls to create records in NetHunt CRM.
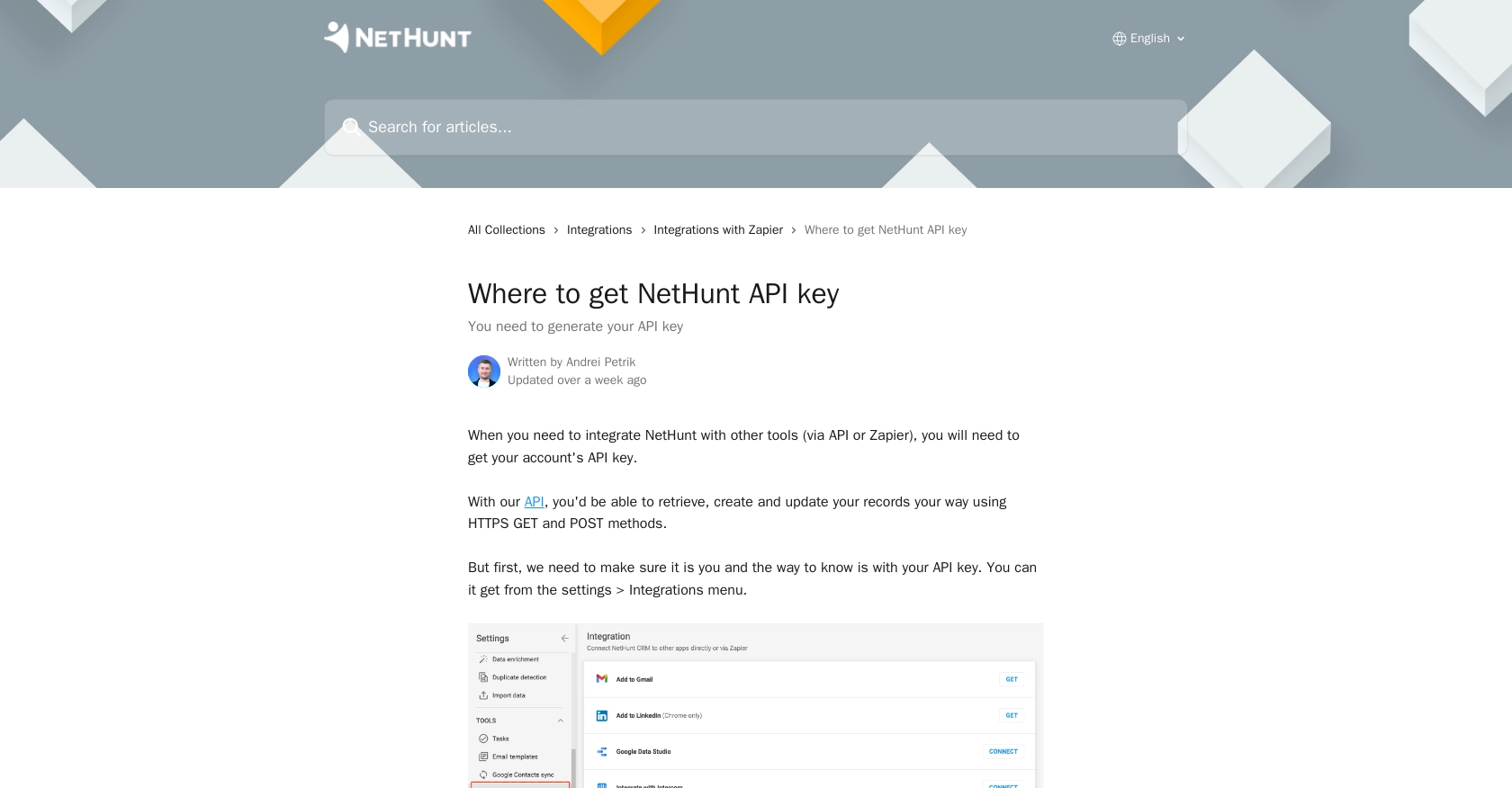
sbb-itb-96038d7
Making API Calls to Create Records in NetHunt CRM Using JavaScript
Once you have your NetHunt API key and authentication headers set up, you can proceed to create records in NetHunt CRM using JavaScript. This section will guide you through the process of making API calls to add new records to your CRM.
Setting Up Your JavaScript Environment for NetHunt API
Before making API calls, ensure you have a JavaScript environment ready. You can use Node.js or a browser-based environment. Make sure you have access to the fetch
API or a similar HTTP client like Axios.
Creating a New Record in NetHunt CRM
To create a new record, you'll need to specify the folder ID where the record will be stored and the data fields for the record. Here's a step-by-step guide:
- Identify the folder ID where you want to create the record. You can list folders using the NetHunt API to find the appropriate folder ID.
- Prepare the data fields for the record. This includes all necessary information such as name, email, and any custom fields you have set up in NetHunt.
- Use the
POST
method to send a request to the NetHunt API endpoint for creating records.
Example Code to Create a Record with NetHunt API
// Example JavaScript code to create a record in NetHunt CRM
const folderId = 'your-folder-id'; // Replace with your folder ID
const apiUrl = `https://nethunt.com/api/v1/zapier/actions/create-record/${folderId}`;
const recordData = {
timeZone: 'Europe/London',
fields: {
Name: 'John Doe',
Birthday: '1980-01-01',
Employed: true,
Salary: 100000,
'Primary Email Address': 'john.doe@example.com'
}
};
fetch(apiUrl, {
method: 'POST',
headers: {
'Authorization': `Basic ${authString}`,
'Content-Type': 'application/json'
},
body: JSON.stringify(recordData)
})
.then(response => response.json())
.then(data => {
console.log('Record created successfully:', data);
})
.catch(error => {
console.error('Error creating record:', error);
});
In this code snippet, replace your-folder-id
with the actual folder ID where you want to create the record. The recordData
object contains the fields and values for the new record. The fetch
function is used to send the POST request to the NetHunt API.
Verifying Record Creation in NetHunt CRM
After executing the API call, you can verify the creation of the new record by checking the specified folder in your NetHunt CRM account. The newly created record should appear with the details you provided.
Handling Errors and Troubleshooting
If the API call fails, ensure that your authentication headers are correct and that the folder ID and data fields are properly specified. Check the console for error messages to help diagnose any issues.
For more detailed information on error codes and troubleshooting, refer to the NetHunt API Documentation.
Best Practices for Using NetHunt API in JavaScript
When working with the NetHunt API, it's crucial to follow best practices to ensure efficient and secure integration. Here are some recommendations:
- Securely Store API Credentials: Always store your API key and authentication details securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Validate API Responses: Always check the response status and handle errors appropriately. Use try-catch blocks to manage exceptions and log errors for troubleshooting.
- Standardize Data Fields: Ensure that the data fields you send to the API are consistent with your CRM's schema. This helps maintain data integrity and avoids errors during record creation.
Leveraging Endgrate for Seamless NetHunt Integrations
Building and maintaining integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including NetHunt.
By using Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the integration complexities.
- Build Once, Use Everywhere: Develop a single integration that works across various platforms, reducing redundancy.
- Enhance User Experience: Provide your customers with a seamless and intuitive integration experience.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website.
Read More
Ready to get started?