Using the Pipeliner CRM API to Create Or Update Contacts (with PHP examples)
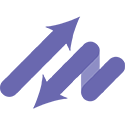
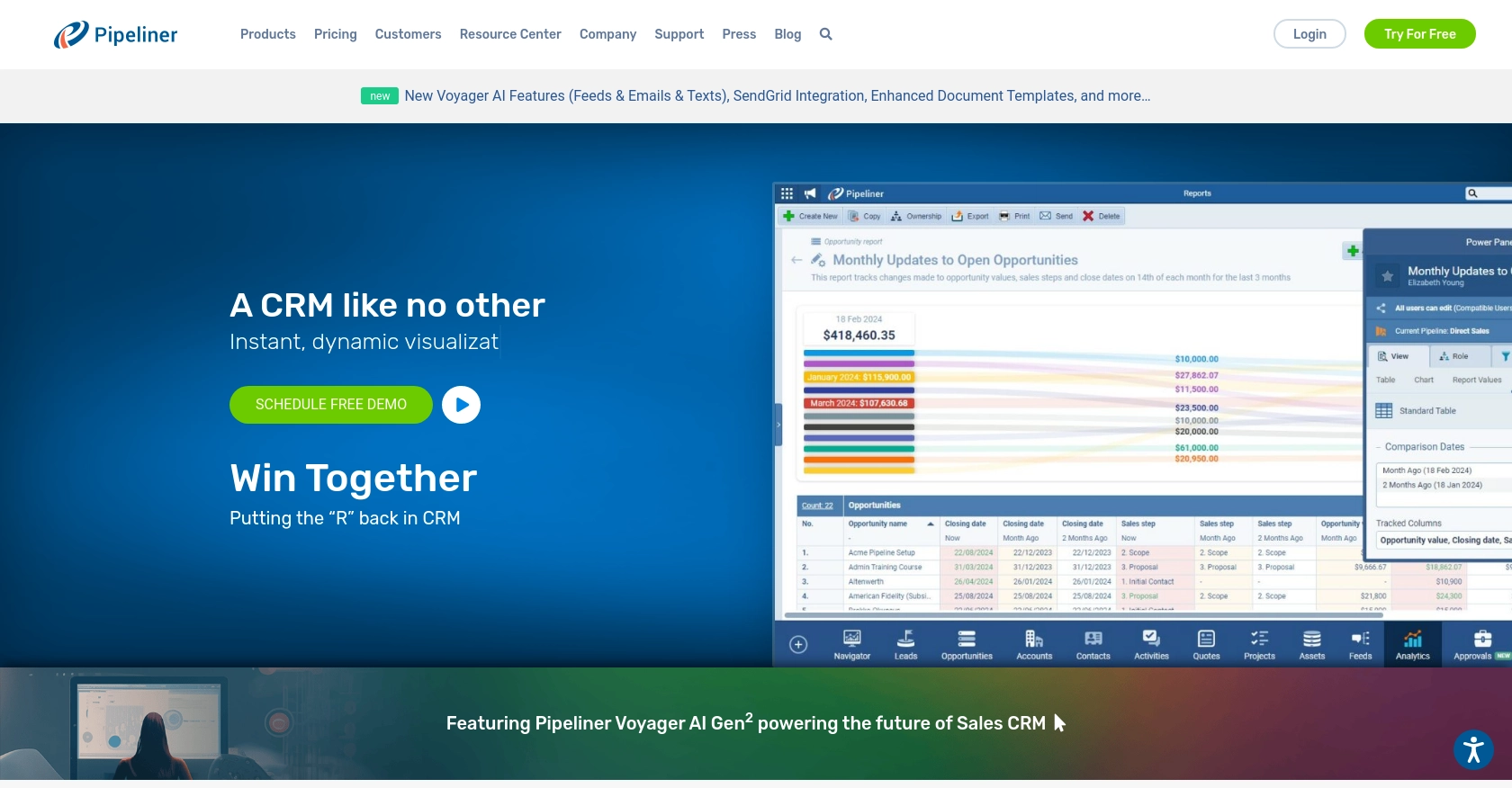
Introduction to Pipeliner CRM
Pipeliner CRM is a robust customer relationship management platform designed to enhance sales processes and improve team collaboration. With its intuitive interface and powerful features, Pipeliner CRM helps businesses manage their sales pipelines, track customer interactions, and drive growth.
Integrating with Pipeliner CRM's API allows developers to automate and streamline CRM tasks, such as creating or updating contact information. For example, a developer might use the Pipeliner CRM API to automatically update contact details from an external data source, ensuring that the sales team always has access to the most current information.
Setting Up Your Pipeliner CRM Test Account
Before diving into the integration with Pipeliner CRM, it's essential to set up a test account to safely experiment with the API. This allows developers to test their code without affecting live data, ensuring a smooth integration process.
Creating a Pipeliner CRM Account
If you don't already have a Pipeliner CRM account, follow these steps to create one:
- Visit the Pipeliner CRM website and sign up for an account.
- Follow the on-screen instructions to complete the registration process.
- Once registered, log in to your Pipeliner CRM account.
Generating Pipeliner CRM API Keys for Authentication
Pipeliner CRM uses Basic Authentication, which requires a username and password. These credentials are unique to your team space and are essential for making API calls. Follow these steps to obtain your API keys:
- Log in to your Pipeliner CRM account and select your team space.
- Navigate to the administration section.
- Under the "Unit, Users & Roles" tab, open "Applications."
- Create a new application and click "Show API Access" to generate your API keys.
- Store the username and password securely, as they are generated only once.
For more details, refer to the Pipeliner CRM authentication documentation.
Understanding Pipeliner CRM API Authentication
With your API keys ready, you can now authenticate your API requests. The username and password will be used in the Basic Authentication header for each API call. Ensure that these credentials are kept confidential and stored securely.
By setting up your test account and obtaining the necessary API keys, you're now prepared to start integrating with Pipeliner CRM's API. In the next section, we'll explore how to make API calls using PHP to create or update contacts.
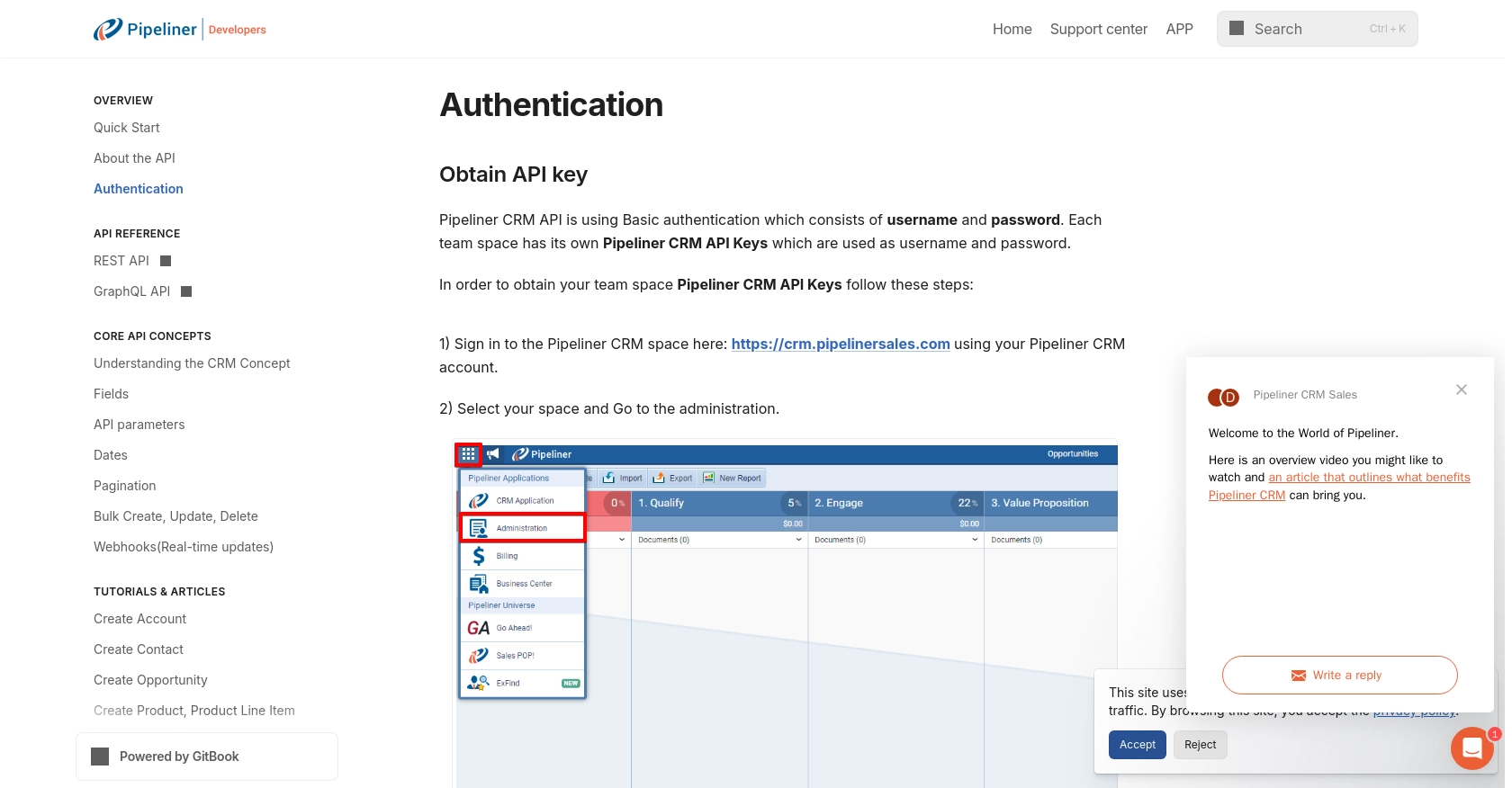
sbb-itb-96038d7
Making API Calls with PHP to Create or Update Contacts in Pipeliner CRM
To interact with the Pipeliner CRM API using PHP, you'll need to set up your environment and write code that can handle API requests. This section will guide you through the process of creating or updating contacts using PHP, ensuring a seamless integration with Pipeliner CRM.
Setting Up Your PHP Environment for Pipeliner CRM API Integration
Before making API calls, ensure you have the following prerequisites installed:
- PHP 7.4 or later
- cURL extension for PHP
These tools will allow you to send HTTP requests and handle responses from the Pipeliner CRM API.
Creating a Contact in Pipeliner CRM Using PHP
To create a contact in Pipeliner CRM, you'll need to send a POST request to the appropriate endpoint. Here's a step-by-step guide:
<?php
// Pipeliner CRM API credentials
$username = 'your_username';
$password = 'your_password';
// API endpoint for creating a contact
$url = 'https://api.pipelinersales.com/contacts';
// Contact data to be created
$data = [
'firstName' => 'John',
'lastName' => 'Doe',
'email' => 'john.doe@example.com'
];
// Initialize cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Content-Type: application/json'
]);
curl_setopt($ch, CURLOPT_USERPWD, "$username:$password");
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
echo 'Contact Created: ' . $response;
}
// Close cURL session
curl_close($ch);
?>
Replace your_username
and your_password
with your actual API credentials. This script initializes a cURL session, sets the necessary options, and sends a POST request to create a contact.
Updating a Contact in Pipeliner CRM Using PHP
To update an existing contact, you'll need to send a PUT request. Here's how you can do it:
<?php
// Pipeliner CRM API credentials
$username = 'your_username';
$password = 'your_password';
// API endpoint for updating a contact
$contactId = 'contact_id'; // Replace with the actual contact ID
$url = "https://api.pipelinersales.com/contacts/$contactId";
// Updated contact data
$data = [
'email' => 'new.email@example.com'
];
// Initialize cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, 'PUT');
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Content-Type: application/json'
]);
curl_setopt($ch, CURLOPT_USERPWD, "$username:$password");
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
echo 'Contact Updated: ' . $response;
}
// Close cURL session
curl_close($ch);
?>
Ensure you replace contact_id
with the actual ID of the contact you wish to update. This script sends a PUT request to update the specified contact's information.
Verifying API Request Success in Pipeliner CRM
After executing the API calls, you can verify the success of your requests by checking the response data. A successful creation or update will return a confirmation message or the updated contact details. Additionally, you can log in to your Pipeliner CRM account to visually confirm the changes.
Handling Errors and Error Codes in Pipeliner CRM API
It's crucial to handle potential errors in your API requests. Common error codes include:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 404 Not Found: The requested resource could not be found.
Implement error handling in your PHP code to manage these scenarios effectively, ensuring a robust integration with Pipeliner CRM.
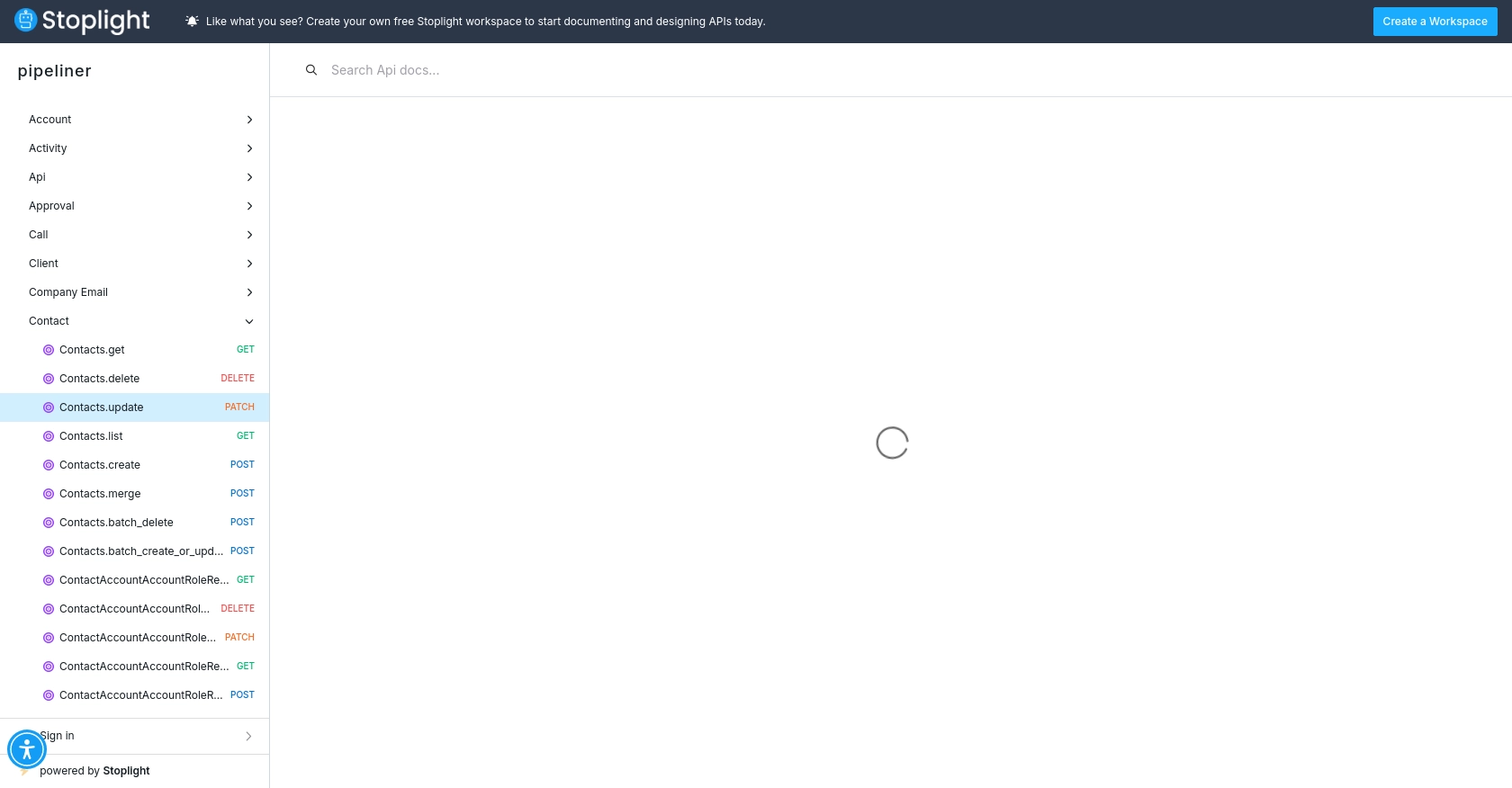
Best Practices for Integrating with Pipeliner CRM API
Successfully integrating with the Pipeliner CRM API requires attention to best practices to ensure security, efficiency, and maintainability. Here are some key recommendations:
- Securely Store Credentials: Always store your API credentials securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of Pipeliner CRM's rate limits to avoid throttling. Implement exponential backoff strategies to handle rate limit responses gracefully.
- Data Transformation and Standardization: Ensure that data formats are consistent between your application and Pipeliner CRM. Transform and standardize data fields as needed to maintain data integrity.
Leveraging Endgrate for Seamless Integration with Pipeliner CRM
While integrating directly with Pipeliner CRM's API is a powerful option, using a tool like Endgrate can simplify the process significantly. Endgrate provides a unified API endpoint that connects to multiple platforms, including Pipeliner CRM, allowing you to manage integrations more efficiently.
By utilizing Endgrate, you can:
- Save Time and Resources: Focus on your core product development while Endgrate handles the complexities of multiple integrations.
- Build Once, Deploy Everywhere: Create integration logic once and apply it across various platforms, reducing redundancy and maintenance efforts.
- Enhance Customer Experience: Offer your users a seamless and intuitive integration experience with minimal effort.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined integration process.
Read More
- https://endgrate.com/provider/pipelinercrm
- https://developers.pipelinersales.com/api-docs/overview/authentication
- https://developers.pipelinersales.com/api-docs/core-api-concepts/pagination
- https://pipeliner.stoplight.io/docs/api-docs/ah9798x4qjh7r-contacts-update
- https://pipeliner.stoplight.io/docs/api-docs/hds5thtgx28ti-contacts-create
Ready to get started?