How to Get Users with the Outreach API in Python
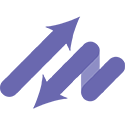
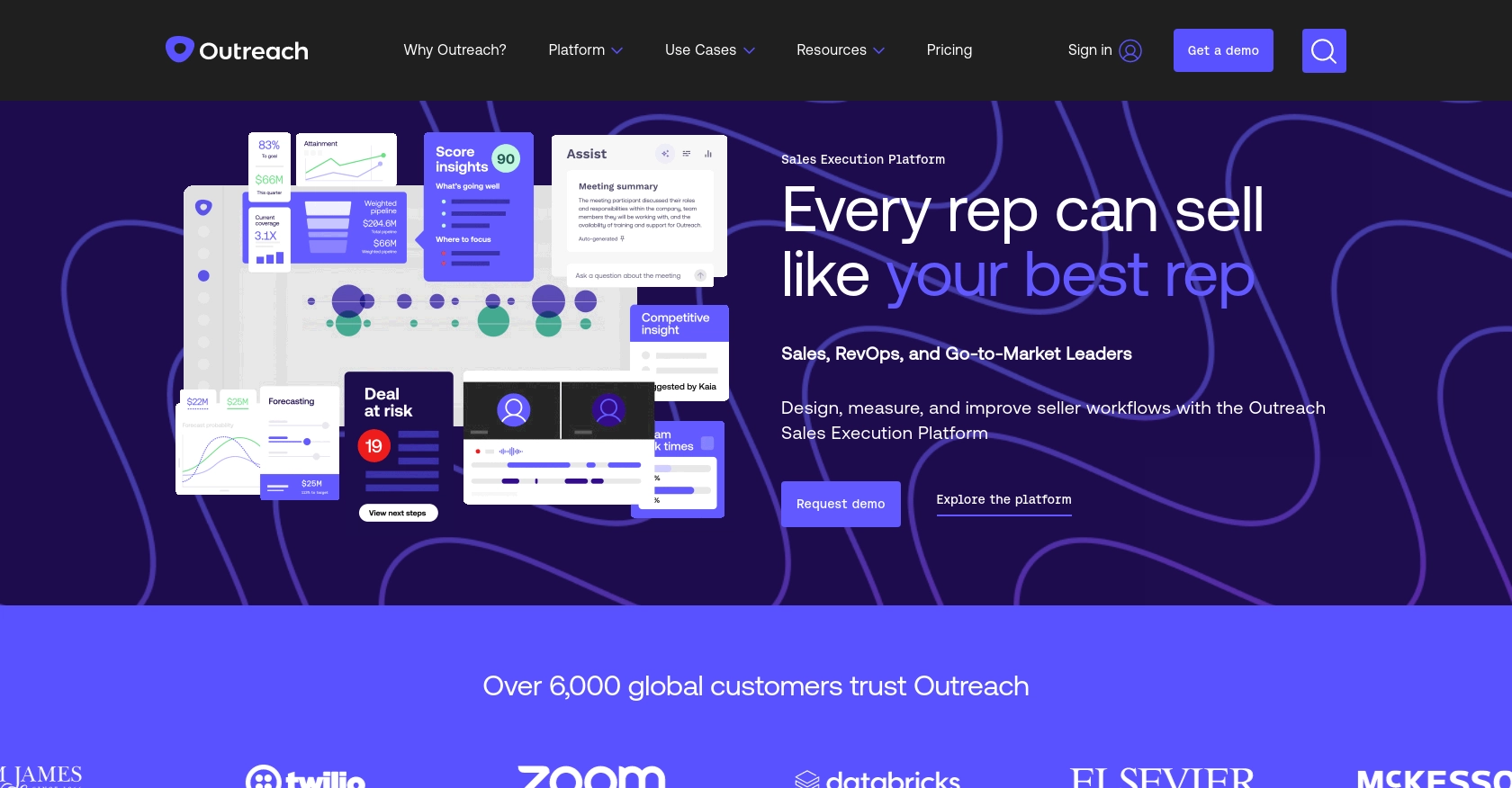
Introduction to Outreach API
Outreach is a powerful sales engagement platform designed to streamline communication and enhance productivity for sales teams. It offers a suite of tools that help businesses manage customer interactions, automate workflows, and improve sales performance.
Integrating with the Outreach API allows developers to access and manage user data efficiently. For example, you can retrieve user information to personalize sales strategies or automate reporting processes. This integration can significantly enhance the capabilities of your B2B SaaS product by providing seamless access to user data.
Setting Up Your Outreach Test/Sandbox Account
Before you can start interacting with the Outreach API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Create an Outreach Developer Account
If you don't already have an Outreach account, you'll need to sign up for one. Visit the Outreach Developer Portal to create a free developer account. This account will give you access to the necessary tools and resources to build and test your integrations.
Set Up OAuth for Outreach API Access
The Outreach API uses OAuth 2.0 for authentication. Follow these steps to set up OAuth:
- Log in to your Outreach Developer account.
- Navigate to the "API Access" section in the developer portal.
- Create a new application by providing the required details such as application name and description.
- Specify one or more redirect URIs. These URIs are where Outreach will redirect users after they authorize your application.
- Select the OAuth scopes your application will need. For accessing user data, ensure you include scopes like
users.read
. - Save your application to generate the client ID and client secret. Note that the client secret will only be displayed once, so make sure to store it securely.
Generate OAuth Tokens
Once your application is set up, you can generate OAuth tokens to authenticate API requests:
- Redirect users to the following URL to obtain an authorization code:
- After the user authorizes the application, they will be redirected to your specified redirect URI with an authorization code.
- Exchange the authorization code for an access token by making a POST request to the token endpoint:
- Store the access token securely, as it will be used to authenticate your API requests.
https://api.outreach.io/oauth/authorize?client_id=<client_id>&redirect_uri=<redirect_uri>&response_type=code&scope=users.read
curl https://api.outreach.io/oauth/token \
-X POST \
-d client_id=<client_id> \
-d client_secret=<client_secret> \
-d redirect_uri=<redirect_uri> \
-d grant_type=authorization_code \
-d code=<authorization_code>
For more detailed information on setting up OAuth, refer to the Outreach OAuth documentation.
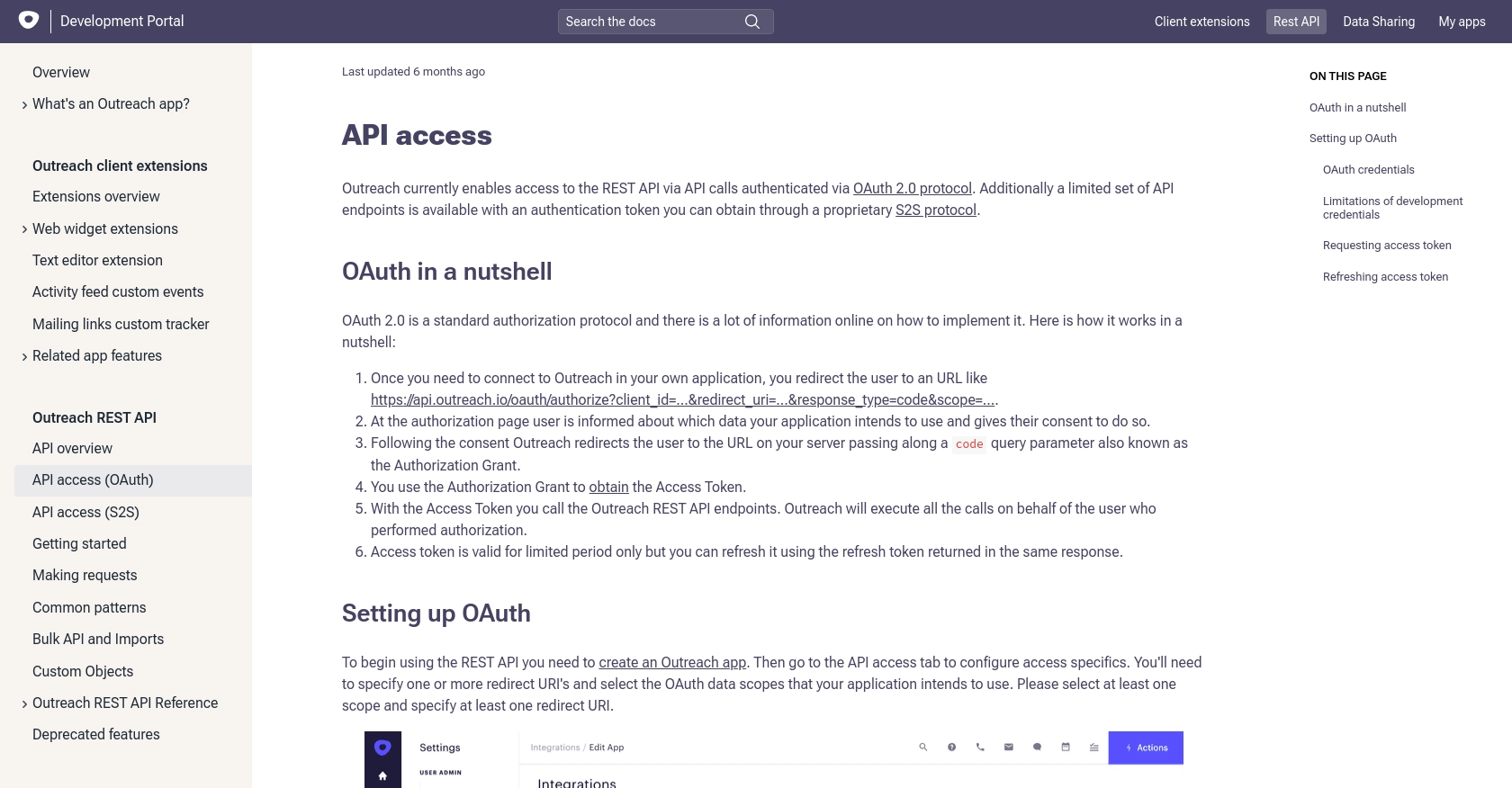
sbb-itb-96038d7
Making API Calls to Retrieve Users with the Outreach API in Python
To interact with the Outreach API and retrieve user data, you'll need to make authenticated API calls using Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for Outreach API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using pip:
pip install requests
Writing Python Code to Retrieve Users from Outreach
Create a new Python file named get_outreach_users.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://api.outreach.io/api/v2/users"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/vnd.api+json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON data from the response
users = response.json()["data"]
for user in users:
print(f"User ID: {user['id']}, Name: {user['attributes']['name']}")
else:
print(f"Failed to retrieve users: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the access token obtained from the OAuth process.
Understanding the Sample Output and Verifying Success
When you run the script using the command python get_outreach_users.py
, you should see a list of users with their IDs and names. This confirms that the API call was successful and the data was retrieved correctly.
Handling Errors and Common Error Codes in Outreach API
It's crucial to handle errors gracefully. The Outreach API may return various HTTP status codes indicating different issues:
- 401 Unauthorized: Check if your access token is valid and not expired.
- 403 Forbidden: Ensure your OAuth scopes include
users.read
. - 429 Too Many Requests: You have exceeded the rate limit of 10,000 requests per hour. Consider implementing a retry mechanism.
For more details on error handling, refer to the Outreach API documentation.
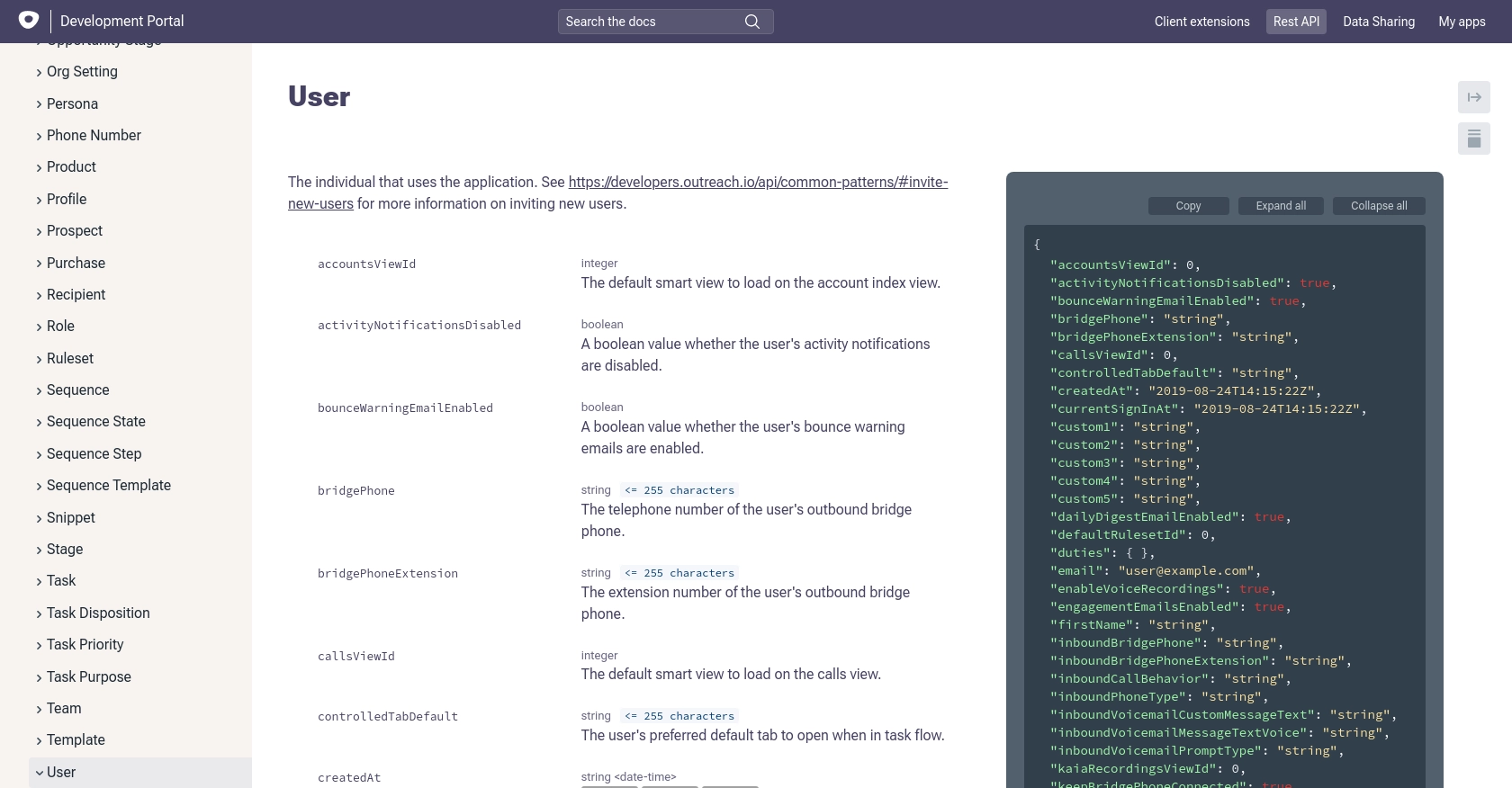
Conclusion and Best Practices for Using Outreach API in Python
Integrating with the Outreach API using Python provides a powerful way to access and manage user data, enhancing your B2B SaaS product's capabilities. By following the steps outlined in this guide, you can efficiently retrieve user information and leverage it to improve sales strategies and automate workflows.
Best Practices for Secure and Efficient API Integration with Outreach
- Secure Storage of Credentials: Always store your OAuth client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault service.
- Handling Rate Limits: The Outreach API has a rate limit of 10,000 requests per hour. Implement a retry mechanism and monitor the
X-RateLimit-Remaining
header to avoid exceeding this limit. - Refreshing Tokens: Access tokens are short-lived. Use the refresh token to obtain new access tokens before they expire, ensuring uninterrupted API access.
- Data Standardization: Standardize and transform data fields as needed to maintain consistency across different integrations and systems.
Enhance Your Integration Strategy with Endgrate
While building integrations with the Outreach API can be rewarding, it can also be time-consuming and complex. Endgrate simplifies this process by offering a unified API endpoint that connects to multiple platforms, including Outreach. By using Endgrate, you can focus on your core product while outsourcing integrations, saving time and resources.
Visit Endgrate to learn more about how you can streamline your integration efforts and provide an intuitive experience for your customers.
Read More
Ready to get started?