Using the Salesloft API to Create or Update Accounts (with Javascript examples)
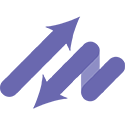
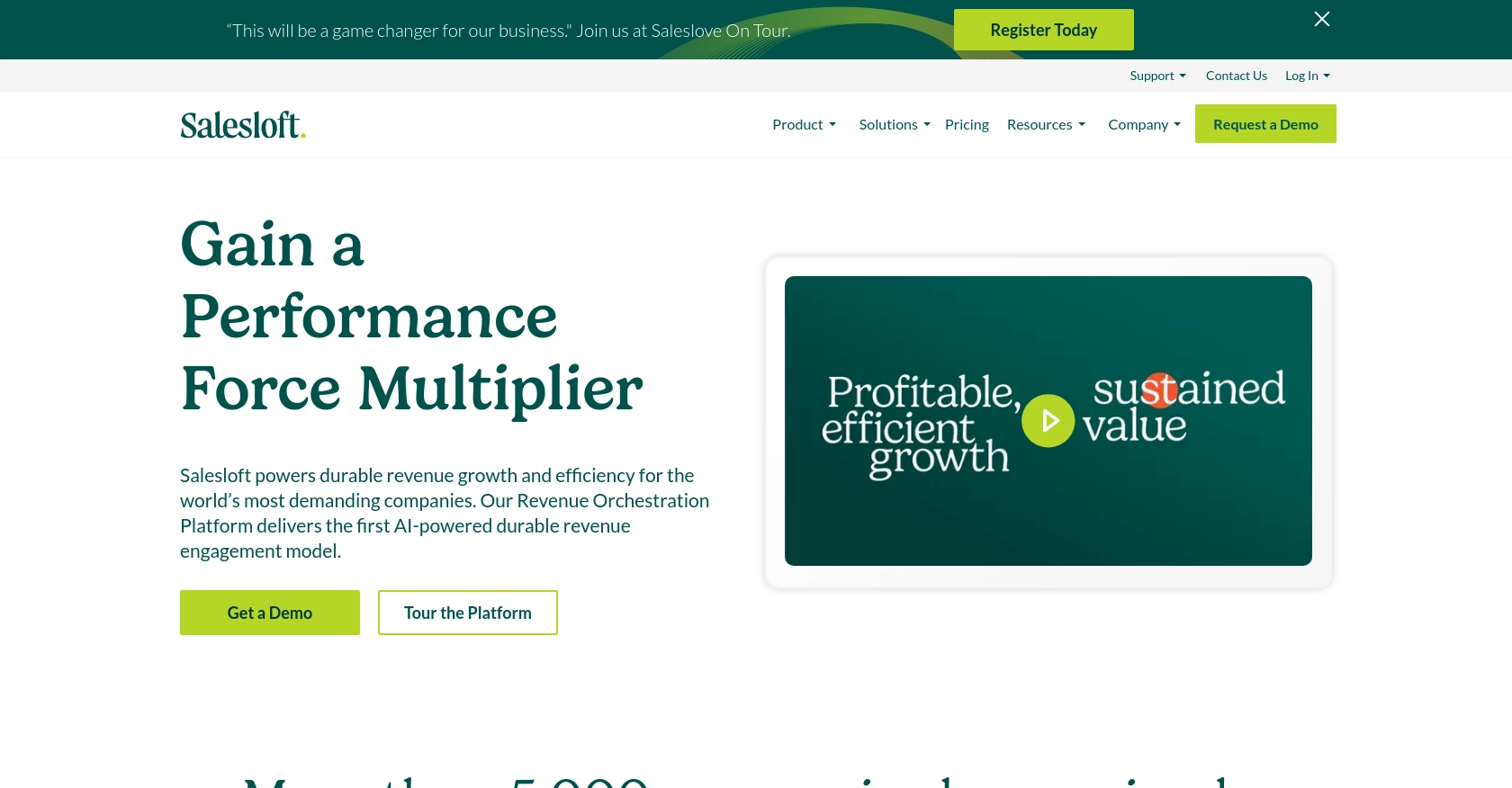
Introduction to Salesloft API Integration
Salesloft is a powerful sales engagement platform designed to enhance the productivity and efficiency of sales teams. By providing tools for communication, analytics, and automation, Salesloft enables sales professionals to connect with prospects more effectively and close deals faster.
Integrating with the Salesloft API allows developers to automate and streamline various sales processes. For example, you can use the API to create or update account information directly from your application, ensuring that your sales data is always up-to-date and accessible.
This article will guide you through using JavaScript to interact with the Salesloft API, focusing on creating and updating accounts. By following this tutorial, you can enhance your application's capabilities and improve your team's sales operations.
Setting Up Your Salesloft Test Account for API Integration
Before diving into the Salesloft API integration, you need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Salesloft provides a sandbox environment where developers can test their applications.
Creating a Salesloft Account
If you don't already have a Salesloft account, you can sign up for a free trial on the Salesloft website. Follow the instructions to create your account and log in to access the dashboard.
Setting Up OAuth Authentication for Salesloft API
Salesloft uses OAuth 2.0 for authentication, which is the preferred method for secure API access. Follow these steps to set up OAuth authentication:
- Navigate to Your Applications in your Salesloft account.
- Select OAuth Applications and click on Create New.
- Fill out the required fields and click Save.
- After saving, you will receive your Application Id (Client Id), Secret (Client Secret), and Redirect URI (Callback URL).
These credentials are essential for the OAuth authorization process.
Obtaining Authorization Code and Access Tokens
To interact with the Salesloft API, you need to obtain an access token. Here's how:
- Generate a request to the authorization endpoint using your
Client Id
andRedirect URI
: - Authorize the application when prompted.
- Upon approval, your
redirect_uri
will receive a code. Use this code to request an access token: - Receive a JSON response containing the
access_token
andrefresh_token
.
https://accounts.salesloft.com/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&response_type=code
POST https://accounts.salesloft.com/oauth/token
{
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET",
"code": "YOUR_AUTHORIZATION_CODE",
"grant_type": "authorization_code",
"redirect_uri": "YOUR_REDIRECT_URI"
}
Store these tokens securely, as they are required for making API requests.
Refreshing Access Tokens
Access tokens have a limited lifespan. When they expire, use the refresh token to obtain a new access token without user intervention:
POST https://accounts.salesloft.com/oauth/token
{
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET",
"grant_type": "refresh_token",
"refresh_token": "YOUR_REFRESH_TOKEN"
}
Ensure you update your stored refresh token with the new one provided in the response.
With your test account and OAuth setup complete, you're ready to start integrating with the Salesloft API.
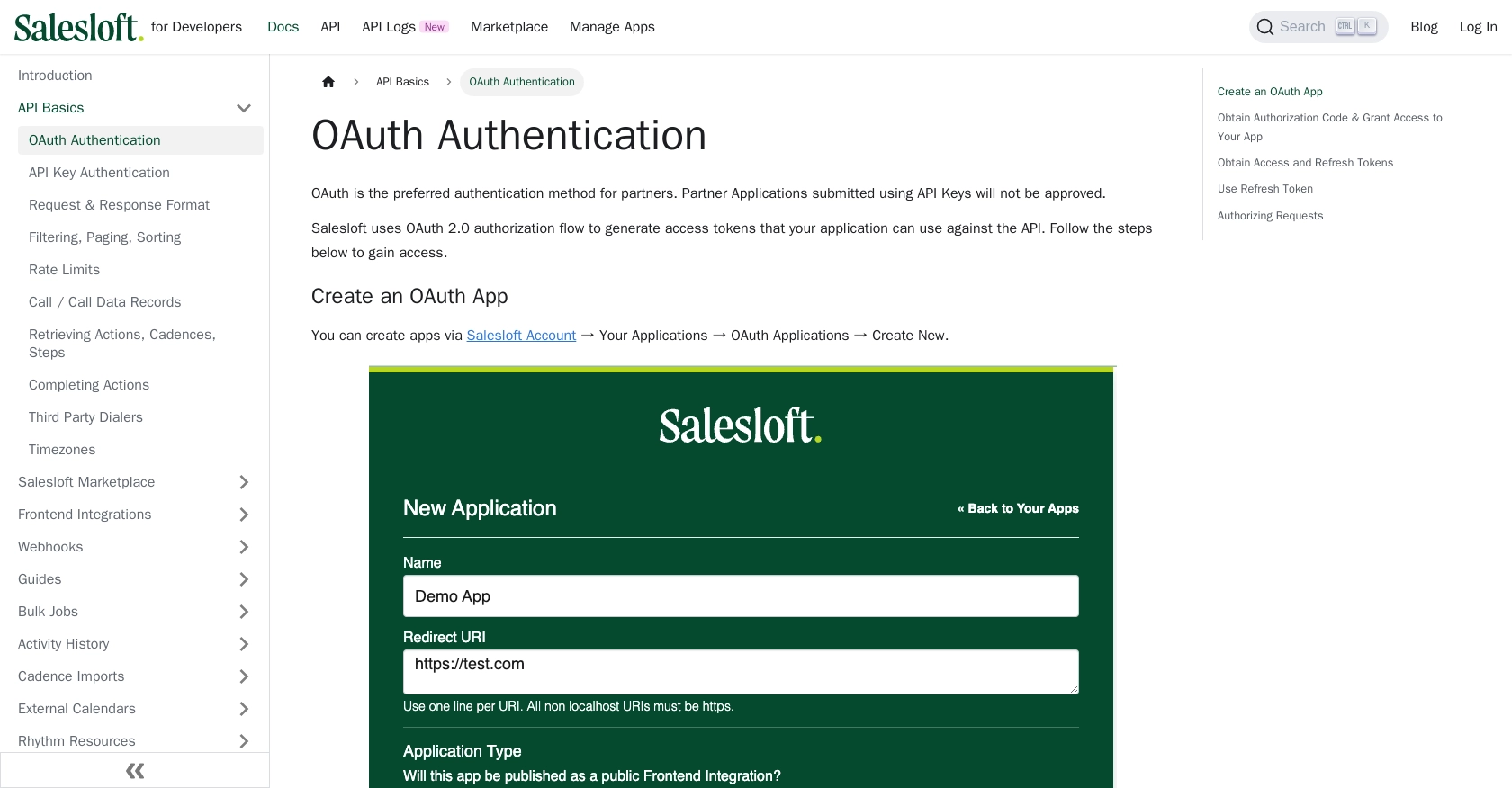
sbb-itb-96038d7
Making API Calls to Salesloft for Creating or Updating Accounts with JavaScript
With your Salesloft test account and OAuth authentication set up, you can now proceed to make API calls to create or update accounts. This section will guide you through the process using JavaScript, ensuring your application can interact seamlessly with the Salesloft API.
Prerequisites for JavaScript Integration with Salesloft API
Before you begin, ensure you have the following installed and set up on your development environment:
- Node.js (latest stable version)
- NPM (Node Package Manager)
Additionally, you will need the axios
library to handle HTTP requests. Install it using the following command:
npm install axios
Creating an Account in Salesloft Using JavaScript
To create an account in Salesloft, you will need to make a POST request to the Salesloft API endpoint. Below is a sample code snippet demonstrating how to achieve this:
const axios = require('axios');
const createAccount = async () => {
const url = 'https://api.salesloft.com/v2/accounts';
const token = 'YOUR_ACCESS_TOKEN'; // Replace with your access token
const accountData = {
name: 'New Account Name',
domain: 'example.com',
description: 'Description of the account',
phone: '1234567890',
website: 'https://example.com'
};
try {
const response = await axios.post(url, accountData, {
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${token}`
}
});
console.log('Account Created:', response.data);
} catch (error) {
console.error('Error creating account:', error.response.data);
}
};
createAccount();
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth setup. The accountData
object contains the necessary fields for creating an account.
Updating an Existing Account in Salesloft Using JavaScript
To update an existing account, you will need to make a PUT request to the Salesloft API. Here's how you can do it:
const updateAccount = async (accountId) => {
const url = `https://api.salesloft.com/v2/accounts/${accountId}`;
const token = 'YOUR_ACCESS_TOKEN'; // Replace with your access token
const updatedData = {
name: 'Updated Account Name',
description: 'Updated description of the account'
};
try {
const response = await axios.put(url, updatedData, {
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${token}`
}
});
console.log('Account Updated:', response.data);
} catch (error) {
console.error('Error updating account:', error.response.data);
}
};
updateAccount('ACCOUNT_ID');
Replace ACCOUNT_ID
with the ID of the account you wish to update. The updatedData
object contains the fields you want to modify.
Verifying API Call Success and Handling Errors
After making an API call, verify its success by checking the response status code and data. A successful creation or update will typically return a 200 status code along with the account details.
Handle errors by catching exceptions and inspecting the error response. Common error codes include 403 (Forbidden), 404 (Not Found), and 422 (Unprocessable Entity). Refer to the Salesloft API documentation for detailed error handling guidelines.
By following these steps, you can effectively create and update accounts in Salesloft using JavaScript, enhancing your application's integration capabilities.
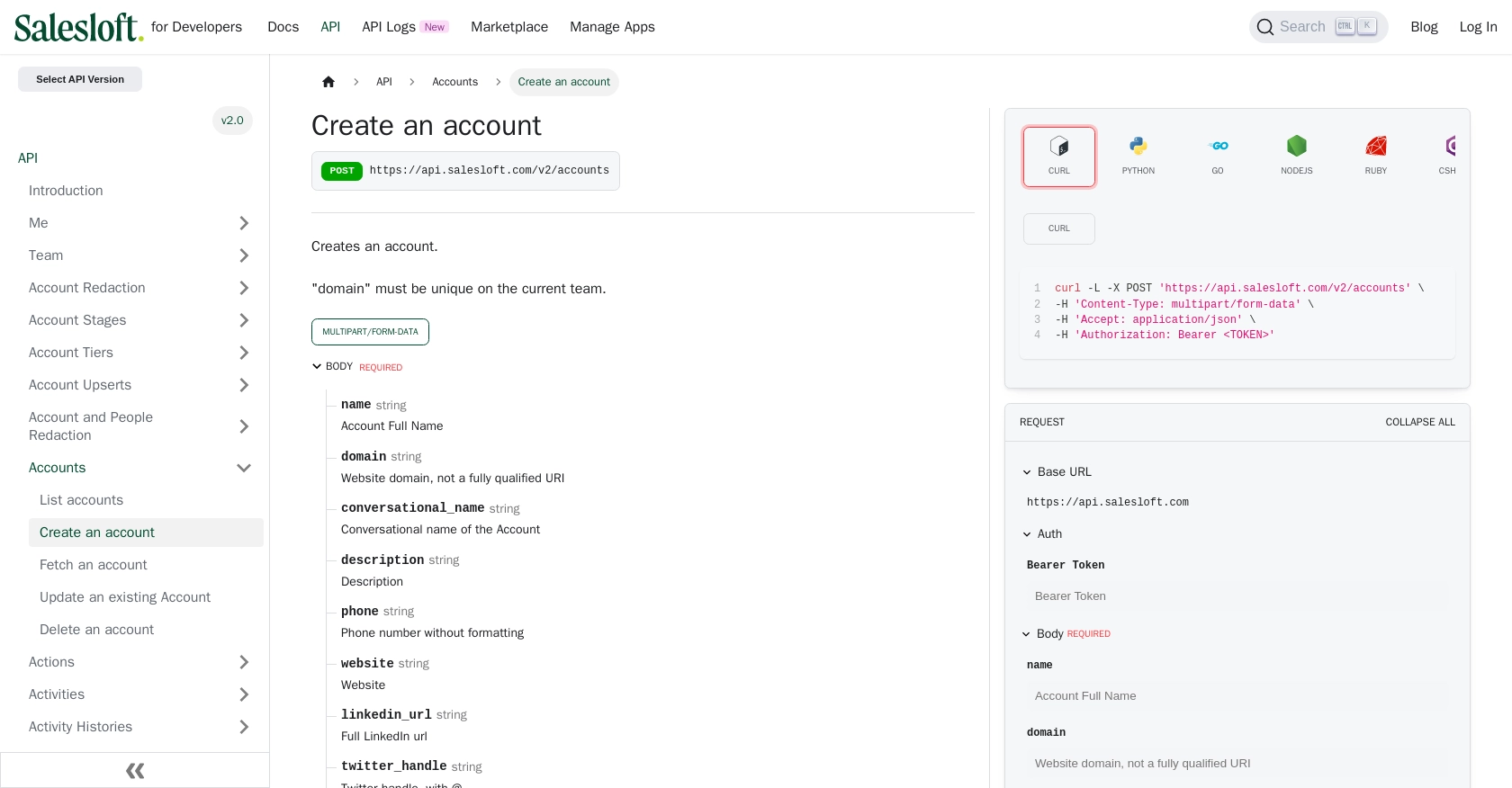
Conclusion and Best Practices for Salesloft API Integration
Integrating with the Salesloft API using JavaScript allows developers to automate account management, enhancing the efficiency of sales operations. By following the steps outlined in this guide, you can create and update accounts seamlessly, ensuring your sales data remains accurate and up-to-date.
Best Practices for Secure and Efficient Salesloft API Usage
- Secure Token Storage: Always store your access and refresh tokens securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Salesloft's rate limits, which are set at 600 cost per minute. Implement logic to handle rate limit responses and avoid exceeding these limits. For more details, refer to the Salesloft API rate limits documentation.
- Error Handling: Implement robust error handling to manage common HTTP status codes such as 403, 404, and 422. This will ensure your application can gracefully handle API errors and provide meaningful feedback to users.
- Data Standardization: Ensure that data fields are standardized and validated before making API calls. This will help maintain data integrity and prevent errors during account creation or updates.
Enhance Your Integration Strategy with Endgrate
While integrating with Salesloft is a powerful way to enhance your sales processes, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration management, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage a single API endpoint to connect with multiple platforms, including Salesloft. This not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/salesloft
- https://developers.salesloft.com/docs/platform/api-basics/oauth-authentication/
- https://developers.salesloft.com/docs/platform/api-basics/request-response-format/
- https://developers.salesloft.com/docs/platform/api-basics/filtering-paging-sorting/
- https://developers.salesloft.com/docs/platform/api-basics/rate-limits/
- https://developers.salesloft.com/docs/api/accounts-create/
Ready to get started?