How to Get Invoices with the Quickbooks API in Javascript
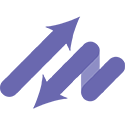
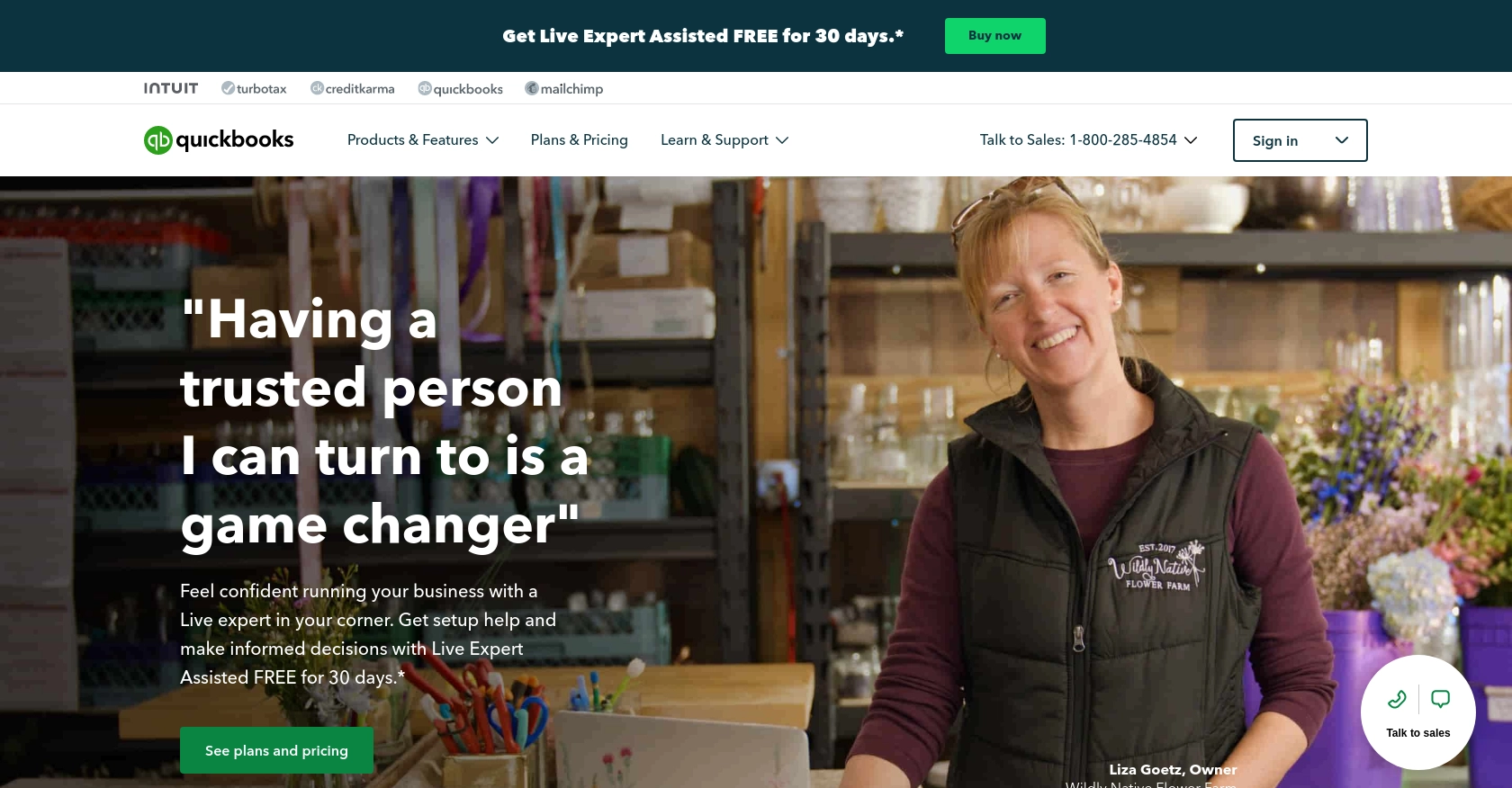
Introduction to QuickBooks API Integration
QuickBooks is a renowned accounting software that offers comprehensive solutions for managing finances, payroll, and other business-related tasks. It is widely used by small to medium-sized businesses to streamline their accounting processes and maintain accurate financial records.
Integrating with the QuickBooks API allows developers to automate and enhance financial operations by accessing various data points, such as invoices, customers, and payments. For example, a developer might want to retrieve invoices using the QuickBooks API in JavaScript to automate billing processes or generate financial reports.
This article will guide you through the steps to effectively interact with the QuickBooks API using JavaScript, focusing on retrieving invoice data to optimize your financial workflows.
Setting Up Your QuickBooks Sandbox Account for API Integration
Before you can start interacting with the QuickBooks API, you need to set up a sandbox account. This allows you to test your integration without affecting live data. QuickBooks provides a sandbox environment that mimics the production environment, enabling developers to experiment and test their applications safely.
Creating a QuickBooks Developer Account
To begin, you'll need to create a QuickBooks Developer account. Follow these steps:
- Visit the QuickBooks Developer Portal.
- Click on "Sign Up" to create a new account or "Sign In" if you already have one.
- Fill in the required information and complete the registration process.
Setting Up a QuickBooks Sandbox Company
Once your developer account is ready, you can set up a sandbox company:
- Log in to your QuickBooks Developer account.
- Navigate to the "Sandbox" section in the dashboard.
- Click on "Add Sandbox" to create a new sandbox company.
- Follow the prompts to configure your sandbox environment.
Creating a QuickBooks App for OAuth Authentication
To interact with the QuickBooks API, you'll need to create an app that uses OAuth for authentication:
- In your QuickBooks Developer account, go to the "My Apps" section.
- Click on "Create an App" and select "QuickBooks Online and Payments."
- Fill in the necessary details for your app, such as name and description.
- Once the app is created, navigate to the "Keys & OAuth" section.
- Here, you'll find your Client ID and Client Secret. These are crucial for authenticating API requests.
For more detailed instructions, refer to the QuickBooks OAuth Setup Guide.
Configuring OAuth Scopes for Invoice Access
Ensure your app has the correct permissions to access invoice data:
- In the "Scopes" section of your app settings, select the necessary scopes for invoices.
- Make sure to include both read and write permissions if needed.
For additional configuration options, visit the QuickBooks App Settings Documentation.
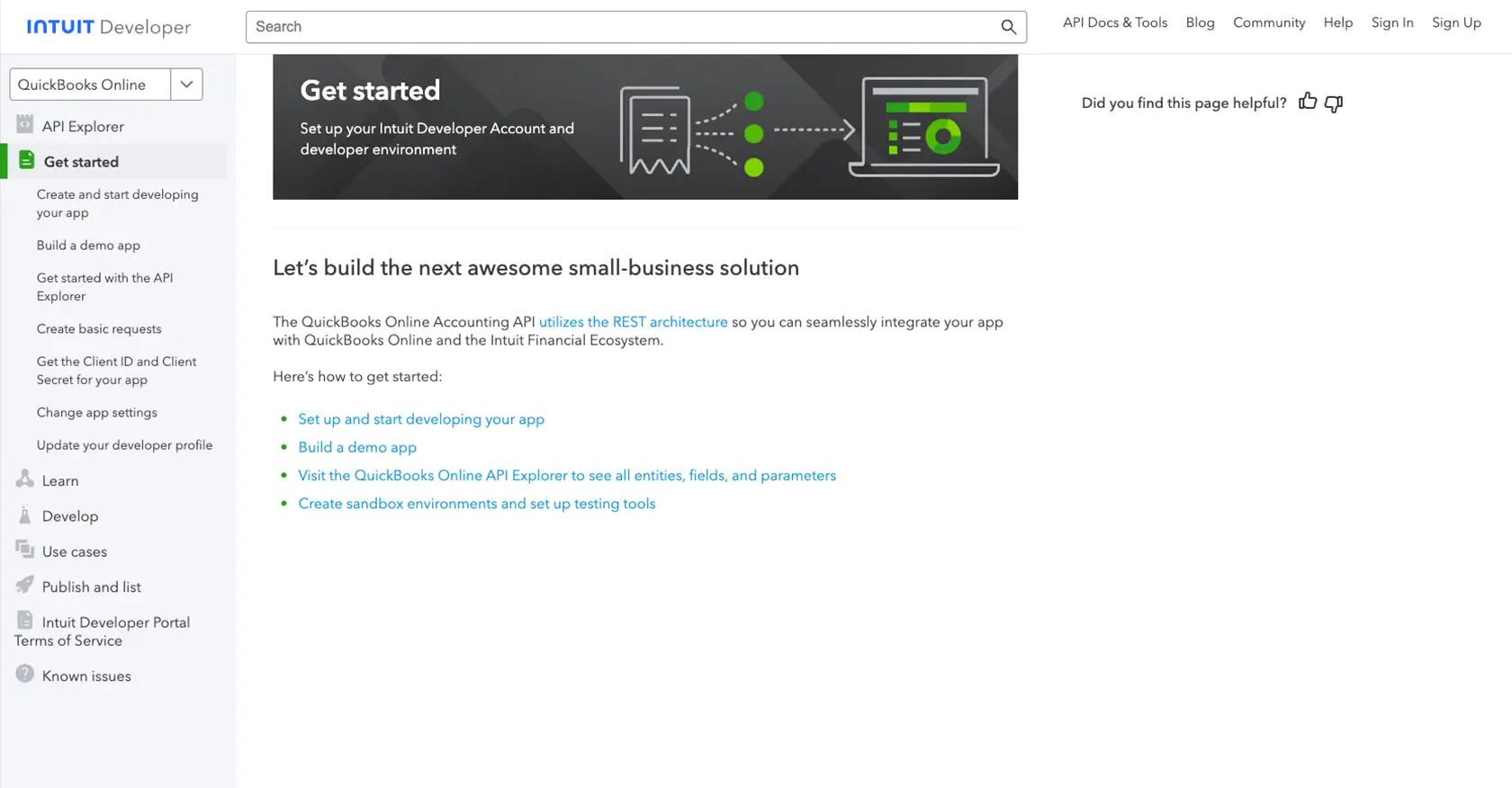
sbb-itb-96038d7
Making API Calls to Retrieve Invoices from QuickBooks Using JavaScript
To interact with the QuickBooks API and retrieve invoice data using JavaScript, you'll need to set up your development environment and write the necessary code to make API requests. This section will guide you through the process, ensuring you have everything you need to successfully access invoice data.
Setting Up Your JavaScript Environment for QuickBooks API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Node.js (version 14.x or later)
- NPM (Node Package Manager)
Once you have Node.js and NPM installed, create a new project directory and initialize it with the following command:
npm init -y
This command will create a package.json
file in your project directory, which is essential for managing dependencies.
Installing Required Dependencies for QuickBooks API Calls
To make HTTP requests and handle OAuth authentication, you'll need to install the following packages:
npm install axios qs
Axios is a popular library for making HTTP requests, and qs is used for query string parsing and stringifying.
Writing JavaScript Code to Retrieve Invoices from QuickBooks API
With your environment set up, you can now write the JavaScript code to make API calls to QuickBooks. Create a file named getInvoices.js
and add the following code:
const axios = require('axios');
const qs = require('qs');
// Replace with your QuickBooks OAuth credentials
const clientId = 'Your_Client_ID';
const clientSecret = 'Your_Client_Secret';
const accessToken = 'Your_Access_Token';
// Set the QuickBooks API endpoint for invoices
const endpoint = 'https://quickbooks.api.intuit.com/v3/company/YOUR_COMPANY_ID/query';
// Define the query to retrieve invoices
const query = 'SELECT * FROM Invoice';
// Function to get invoices
async function getInvoices() {
try {
const response = await axios.post(endpoint, qs.stringify({ query }), {
headers: {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/x-www-form-urlencoded',
'Accept': 'application/json'
}
});
// Log the retrieved invoices
console.log('Invoices:', response.data.QueryResponse.Invoice);
} catch (error) {
console.error('Error retrieving invoices:', error.response ? error.response.data : error.message);
}
}
// Execute the function
getInvoices();
Replace Your_Client_ID
, Your_Client_Secret
, Your_Access_Token
, and YOUR_COMPANY_ID
with your actual QuickBooks credentials and company ID.
Running the JavaScript Code to Fetch QuickBooks Invoices
To execute the code and retrieve invoices, run the following command in your terminal:
node getInvoices.js
If successful, you should see the list of invoices printed in the console. Verify the data by checking your QuickBooks sandbox account to ensure the retrieved invoices match the data in your sandbox.
Handling Errors and QuickBooks API Error Codes
When making API calls, it's crucial to handle potential errors gracefully. The QuickBooks API may return various error codes, such as:
- 401 Unauthorized: Invalid or expired access token.
- 403 Forbidden: Insufficient permissions to access the resource.
- 404 Not Found: The requested resource does not exist.
Ensure your code checks for these errors and implements appropriate error-handling logic to provide meaningful feedback to users.
For more detailed information on error codes, refer to the QuickBooks API Documentation.
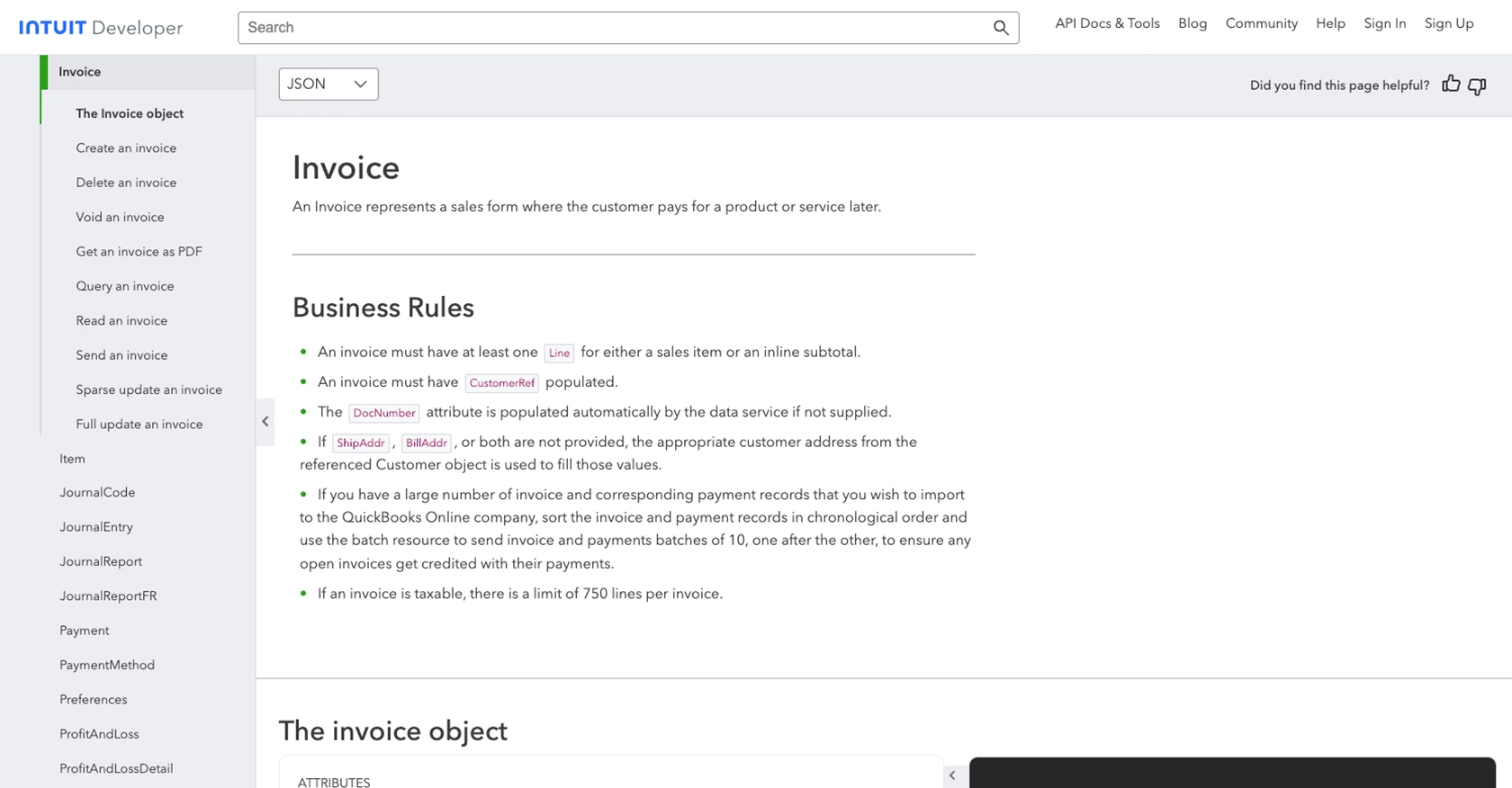
Conclusion and Best Practices for QuickBooks API Integration
Integrating with the QuickBooks API using JavaScript provides a powerful way to automate and enhance financial operations within your applications. By following the steps outlined in this guide, you can efficiently retrieve invoice data and streamline your billing processes.
Best Practices for Secure and Efficient QuickBooks API Usage
- Securely Store Credentials: Always store your Client ID, Client Secret, and Access Tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: QuickBooks API has rate limits in place. Ensure your application handles these limits gracefully by implementing retry logic or backoff strategies. For more details, refer to the QuickBooks API Documentation.
- Data Transformation and Standardization: When retrieving data, ensure it is transformed and standardized to fit your application's needs. This will help maintain consistency and improve data integrity.
- Error Handling: Implement robust error handling to manage API errors and provide meaningful feedback to users. This includes checking for common error codes and taking appropriate actions.
Streamline Your Integrations with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including QuickBooks. By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Provide an easy, intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration capabilities by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/all-entities/invoice
Ready to get started?