How to Get Responses with the Google Forms API in PHP
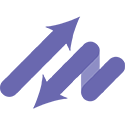
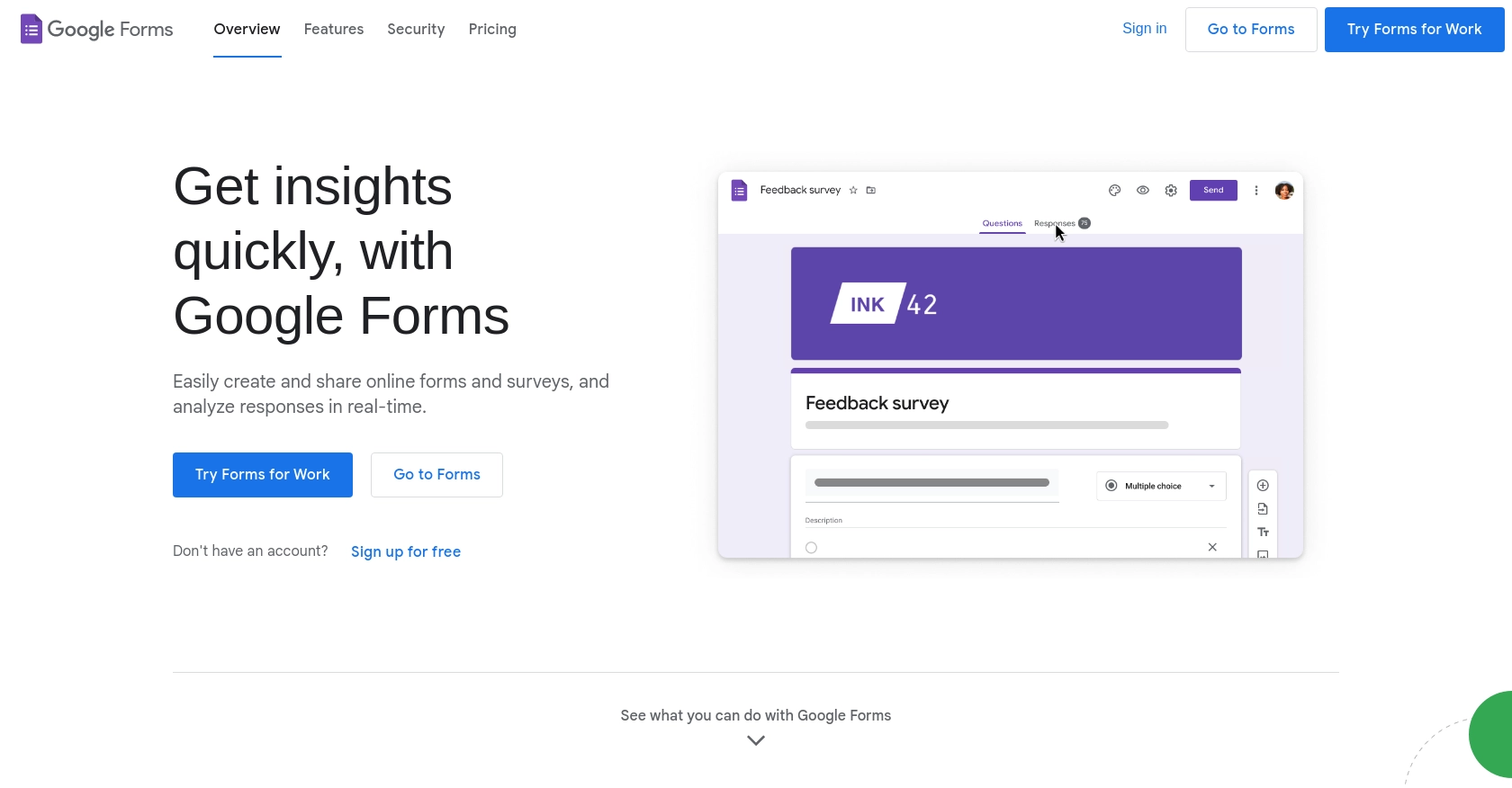
Introduction to Google Forms API Integration
Google Forms is a versatile tool within the Google Workspace suite, enabling users to create surveys, quizzes, and forms with ease. Its integration capabilities allow developers to automate data collection and processing, making it an essential tool for businesses and educational institutions.
By integrating with the Google Forms API, developers can programmatically access form responses, streamline data analysis, and automate workflows. For example, a developer might use the API to retrieve survey responses and automatically generate reports or trigger follow-up actions based on the data collected.
Setting Up Your Google Forms API Test Account
Before you can start interacting with the Google Forms API using PHP, you need to set up a Google Cloud project and configure OAuth 2.0 authentication. This will allow you to securely access form responses programmatically.
Create a Google Cloud Project for Google Forms API
- Go to the Google Cloud Console.
- Click on the menu icon and navigate to IAM & Admin > Create a Project.
- Enter a name for your project and click Create.
Enable Google Forms API in Your Project
- In the Google Cloud Console, go to APIs & Services > Library.
- Search for "Google Forms API" and click on it.
- Click the Enable button to activate the API for your project.
Configure OAuth Consent Screen for Google Forms API
- Navigate to APIs & Services > OAuth consent screen.
- Select the user type and fill out the required information, such as application name and support email.
- Click Save and Continue.
Create OAuth 2.0 Credentials for Google Forms API
- Go to APIs & Services > Credentials.
- Click Create Credentials and select OAuth client ID.
- Choose Web application as the application type.
- Enter a name for your credentials and set the authorized redirect URIs.
- Click Create to generate your client ID and client secret.
Make sure to securely store your client ID and client secret, as you will need them to authenticate API requests.
For more detailed instructions, refer to the official Google documentation: Create a Google Cloud Project, Enable Google Workspace APIs, Configure OAuth Consent, and Create Credentials.
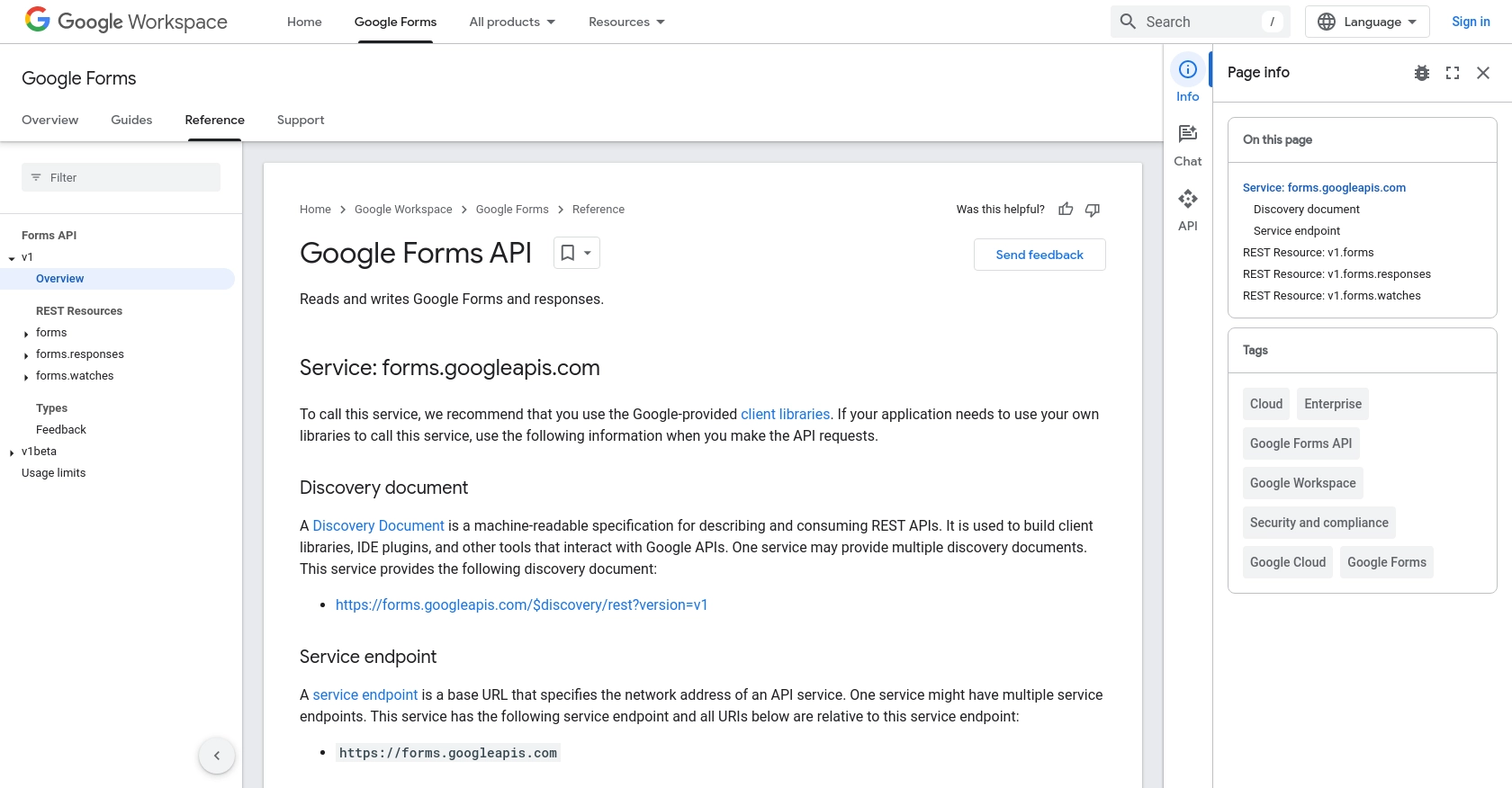
sbb-itb-96038d7
Making API Calls to Retrieve Google Forms Responses Using PHP
To interact with the Google Forms API and retrieve form responses using PHP, you need to set up your development environment and write the necessary code to make API requests. This section will guide you through the process, ensuring you can efficiently access and manage form data.
Setting Up Your PHP Environment for Google Forms API
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or later
- Composer (PHP dependency manager)
Use Composer to install the Google Client Library for PHP by running the following command in your terminal:
composer require google/apiclient:^2.0
Writing PHP Code to Access Google Forms Responses
Once your environment is set up, you can write the PHP script to authenticate and retrieve responses from a Google Form. Create a file named get_google_forms_responses.php
and add the following code:
require 'vendor/autoload.php';
use Google\Client;
use Google\Service\Forms;
// Initialize the Google Client
$client = new Client();
$client->setApplicationName('Google Forms API PHP');
$client->setScopes(['https://www.googleapis.com/auth/forms.responses.readonly']);
$client->setAuthConfig('path/to/your/credentials.json');
// Initialize the Forms service
$service = new Forms($client);
// Specify the form ID
$formId = 'your_form_id_here';
try {
// Retrieve the form responses
$responses = $service->forms_responses->listFormsResponses($formId);
foreach ($responses->getResponses() as $response) {
echo 'Response ID: ' . $response->getResponseId() . "\n";
echo 'Submitted Time: ' . $response->getCreateTime() . "\n";
// Access individual answers
foreach ($response->getAnswers() as $questionId => $answer) {
echo 'Question ID: ' . $questionId . "\n";
echo 'Answer: ' . $answer->getTextAnswers()->getAnswers()[0]->getValue() . "\n";
}
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace path/to/your/credentials.json
with the path to your OAuth 2.0 credentials JSON file, and your_form_id_here
with the ID of the Google Form you wish to access.
Running the PHP Script and Verifying Output
Execute the script using the following command in your terminal:
php get_google_forms_responses.php
If successful, the script will output the response IDs and submission times, along with the answers to each question in the form. Verify the data by checking the responses in your Google Forms dashboard.
Handling Errors and Troubleshooting
When making API calls, you may encounter errors such as authentication failures or insufficient permissions. Ensure your OAuth 2.0 credentials are correct and that the necessary API scopes are enabled. For detailed error codes and troubleshooting, refer to the Google Forms API documentation.
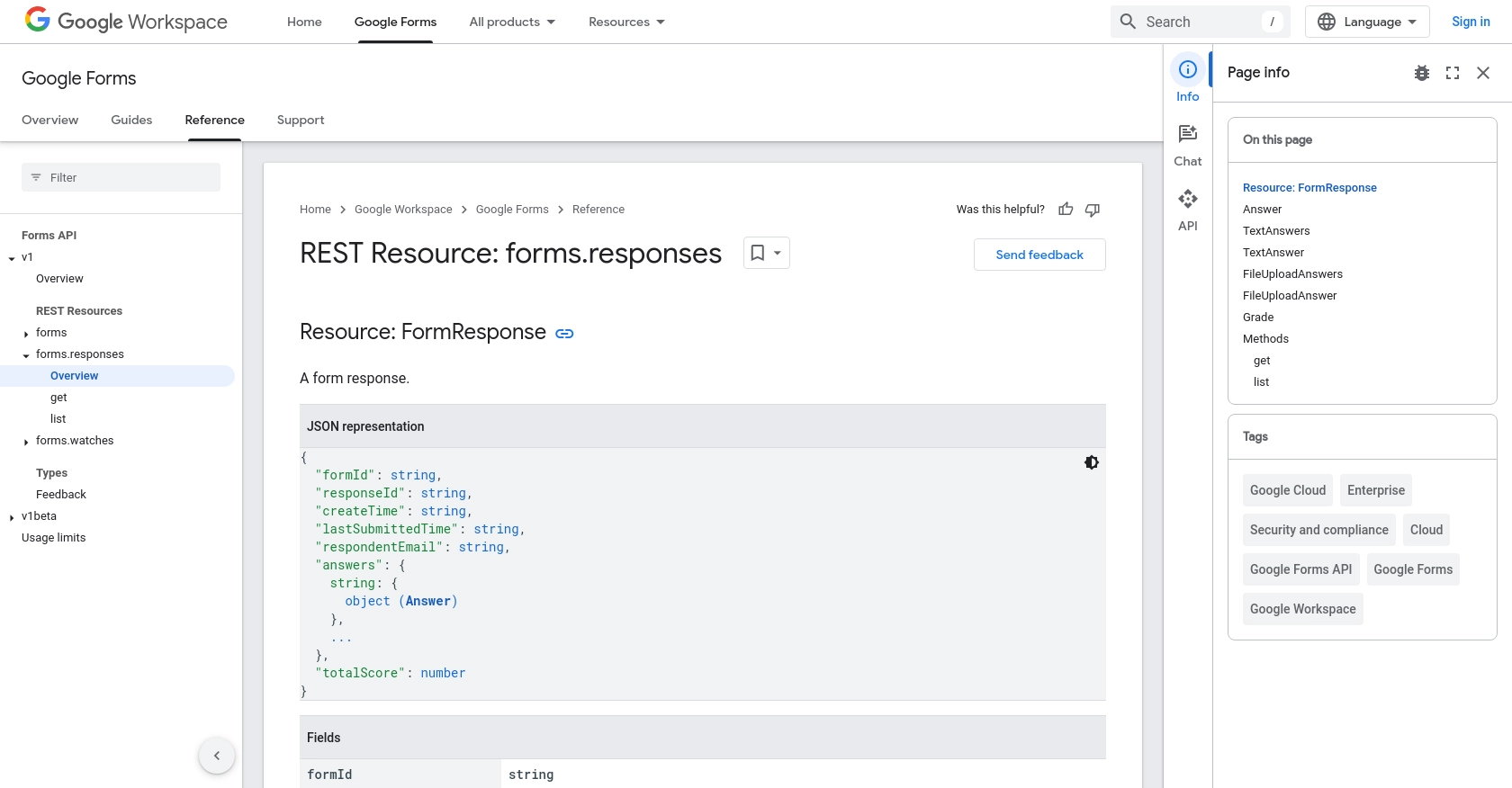
Conclusion and Best Practices for Using Google Forms API with PHP
Integrating Google Forms API with PHP offers a powerful way to automate data collection and streamline workflows. By following the steps outlined in this guide, you can efficiently retrieve and manage form responses, enhancing your application's capabilities.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always keep your OAuth 2.0 credentials secure. Use environment variables or secure vaults to store sensitive information like client IDs and secrets.
- Handle Rate Limiting: Be mindful of API rate limits to avoid service disruptions. Implement exponential backoff strategies to handle retries gracefully.
- Data Standardization: Ensure that the data retrieved from Google Forms is standardized and transformed as needed for your application's requirements.
- Error Handling: Implement robust error handling to manage API call failures. Refer to the Google Forms API documentation for detailed error codes and solutions.
Enhance Your Integration Strategy with Endgrate
While integrating with Google Forms API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate simplifies this process by providing a unified API endpoint for various platforms, including Google Forms. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can streamline your integration efforts and provide an intuitive experience for your customers. Visit Endgrate to learn more and start optimizing your integration strategy today.
Read More
- https://endgrate.com/provider/googleforms
- https://developers.google.com/forms/api/reference/rest
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/forms/api/reference/rest/v1/forms.responses
Ready to get started?