How to Get Contacts with the Active Campaign API in PHP
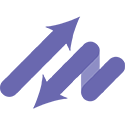
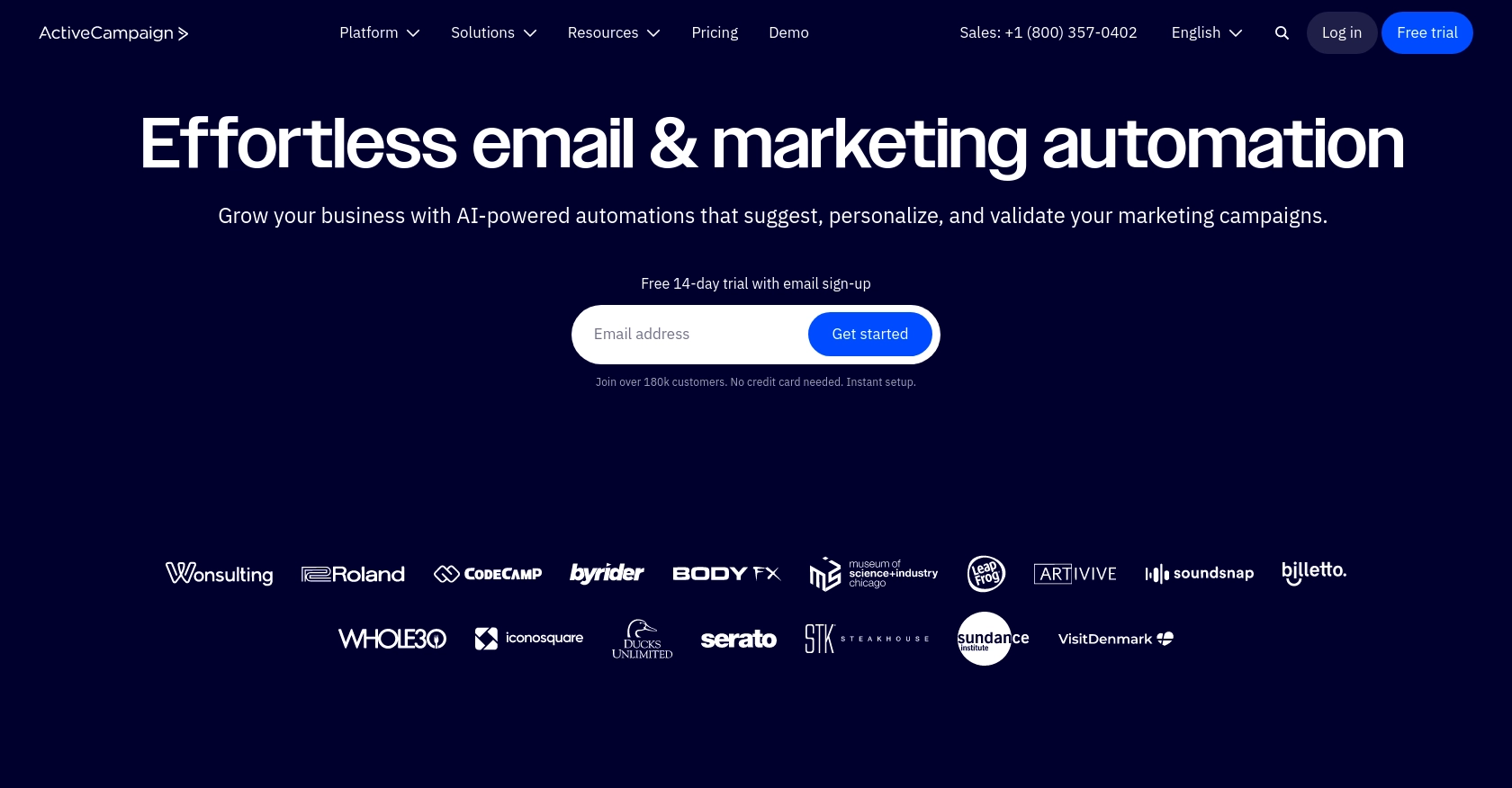
Introduction to Active Campaign API
Active Campaign is a powerful marketing automation platform that combines email marketing, automation, and CRM tools to help businesses engage with their customers more effectively. It offers a comprehensive suite of features designed to streamline marketing efforts and enhance customer relationships.
Developers may want to integrate with Active Campaign's API to access and manage contact data, automate marketing workflows, and enhance customer engagement. For example, a developer could use the Active Campaign API to retrieve contact information and use it to personalize email campaigns based on customer behavior and preferences.
This article will guide you through the process of using PHP to interact with the Active Campaign API, specifically focusing on retrieving contact information. By following this tutorial, you will learn how to efficiently access and manage contacts within the Active Campaign platform using PHP.
Setting Up Your Active Campaign Test Account
Before you can start integrating with the Active Campaign API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Create an Active Campaign Account
If you don't already have an Active Campaign account, you can sign up for a free trial on their website. This trial will provide you with access to the platform's features, including API access.
- Visit the Active Campaign website and click on the "Start Your Free Trial" button.
- Fill in the required information, such as your name, email, and company details.
- Follow the instructions to complete the registration process.
Accessing the Developer Settings
Once your account is set up, you'll need to access the Developer settings to obtain your API key and Base URL, which are essential for making API calls.
- Log in to your Active Campaign account.
- Navigate to the "Settings" section by clicking on your account name in the top right corner and selecting "Settings" from the dropdown menu.
- In the Settings menu, click on the "Developer" tab.
- Here, you'll find your API key and Base URL. Make sure to keep these credentials secure, as they are used to authenticate your API requests.
Generating Your API Key
Active Campaign uses API key-based authentication. Each user in your account has a unique API key, which you will use to authenticate your requests.
- In the Developer tab, locate the "API Key" section.
- Copy the API key provided. This key will be used in the headers of your API requests.
For more information on authentication, refer to the Active Campaign Authentication Documentation.
Setting Up a Sandbox Environment
While Active Campaign does not provide a dedicated sandbox environment, you can use your trial account as a testing ground. Ensure that you use test data to avoid any unintended consequences on real customer data.
By following these steps, you'll be ready to start making API calls to the Active Campaign platform using PHP. In the next section, we'll cover how to make these calls effectively.
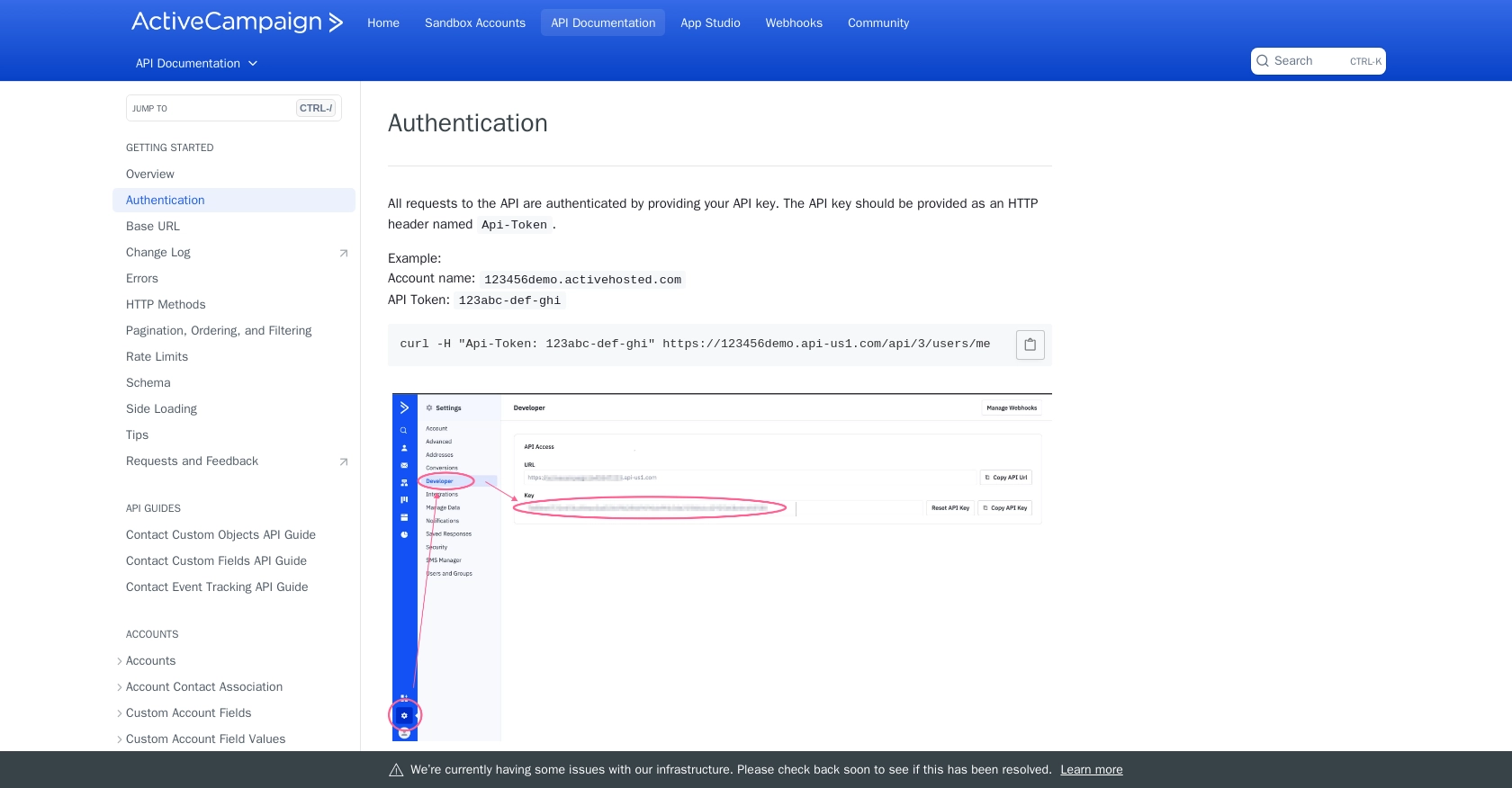
sbb-itb-96038d7
Making API Calls to Retrieve Contacts with Active Campaign API Using PHP
To interact with the Active Campaign API and retrieve contact information, you'll need to use PHP to make HTTP requests. This section will guide you through setting up your PHP environment, installing necessary dependencies, and executing API calls to fetch contact data.
Setting Up Your PHP Environment for Active Campaign API Integration
Before making API calls, ensure your PHP environment is correctly configured. You'll need PHP version 7.4 or higher and the cURL extension enabled to handle HTTP requests.
- Verify your PHP version by running
php -v
in your terminal. - Ensure the cURL extension is enabled by checking your
php.ini
file or runningphp -m
to list installed modules.
Installing Required PHP Dependencies
To simplify HTTP requests, we recommend using the Guzzle HTTP client. Install it via Composer, a dependency manager for PHP.
composer require guzzlehttp/guzzle
If you don't have Composer installed, follow the instructions on the Composer website.
Writing PHP Code to Fetch Contacts from Active Campaign API
With your environment set up, you can now write PHP code to interact with the Active Campaign API. The following example demonstrates how to retrieve contacts:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiKey = 'Your_API_Key';
$baseUrl = 'https://youraccountname.api-us1.com/api/3/contacts';
$response = $client->request('GET', $baseUrl, [
'headers' => [
'Api-Token' => $apiKey,
'Accept' => 'application/json',
],
]);
$data = json_decode($response->getBody(), true);
foreach ($data['contacts'] as $contact) {
echo 'Name: ' . $contact['firstName'] . ' ' . $contact['lastName'] . "\n";
echo 'Email: ' . $contact['email'] . "\n\n";
}
Replace Your_API_Key
with the API key you obtained from the Developer settings. This script initializes a Guzzle client, sets the necessary headers, and sends a GET request to the Active Campaign API to retrieve contacts.
Understanding the API Response and Handling Errors
The API response will include contact details in JSON format. The example code parses this response and prints each contact's name and email. If the request fails, Guzzle will throw an exception, which you can catch and handle appropriately:
try {
$response = $client->request('GET', $baseUrl, [
'headers' => [
'Api-Token' => $apiKey,
'Accept' => 'application/json',
],
]);
} catch (\GuzzleHttp\Exception\RequestException $e) {
echo 'Request failed: ' . $e->getMessage();
}
For more details on error handling and response codes, refer to the Active Campaign API Documentation.
Verifying Successful API Requests in Active Campaign
To confirm that your API requests are successful, check the returned data against the contacts in your Active Campaign account. Ensure that the contact information matches and that no errors are reported in the response.
By following these steps, you can efficiently retrieve and manage contact data from Active Campaign using PHP, enhancing your marketing automation capabilities.
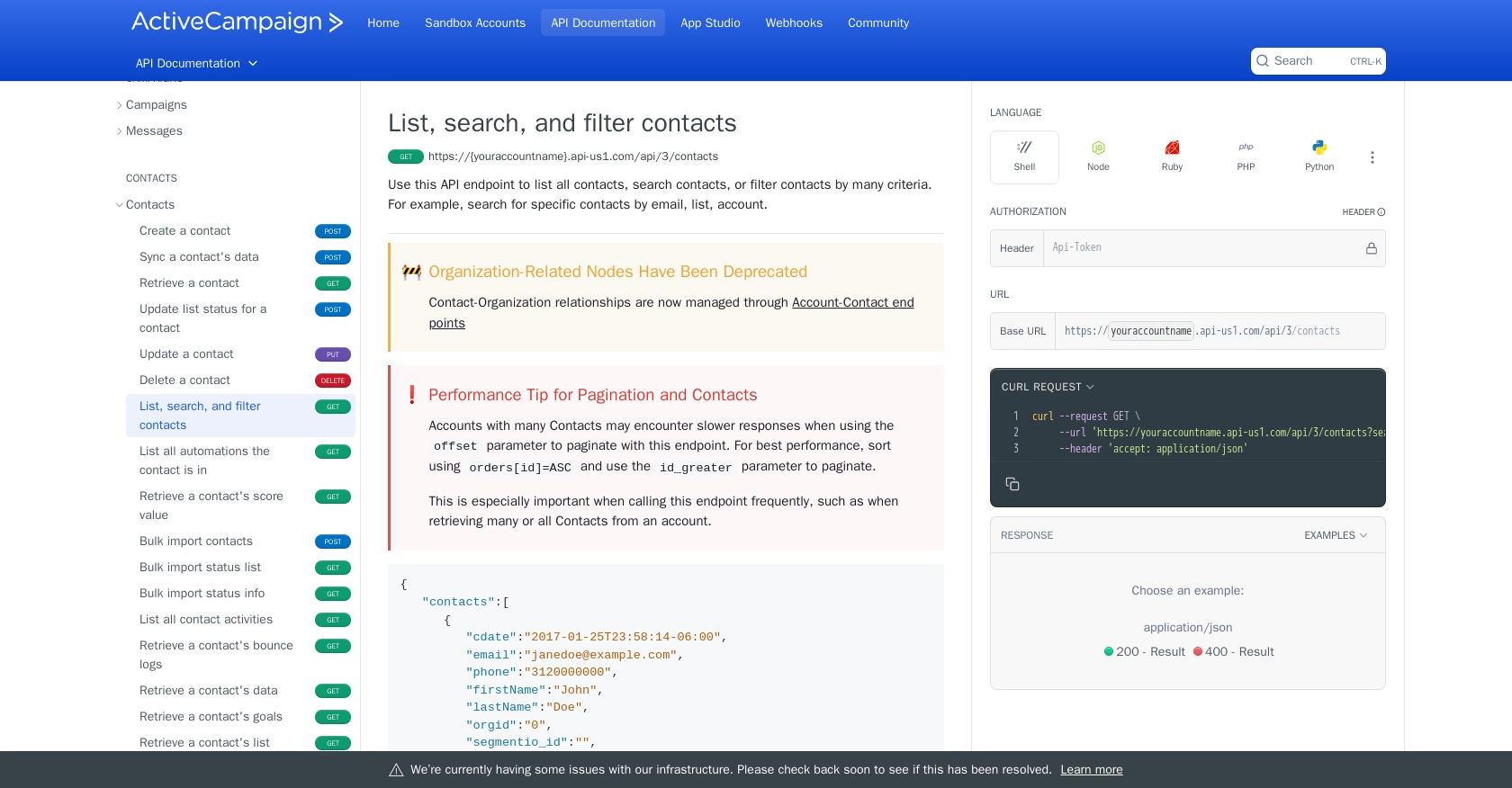
Conclusion and Best Practices for Using Active Campaign API with PHP
Integrating with the Active Campaign API using PHP allows developers to efficiently manage contact data and automate marketing workflows. By following the steps outlined in this guide, you can seamlessly retrieve and handle contact information, enhancing your marketing automation capabilities.
Best Practices for Secure and Efficient API Integration
- Secure API Credentials: Always keep your API key confidential. Avoid exposing it in client-side code and consider storing it securely in environment variables or a secure vault.
- Handle Rate Limits: Active Campaign's API has a rate limit of 5 requests per second per account. Implement logic to handle rate limiting gracefully, such as retrying requests after a delay. For more details, refer to the Active Campaign Rate Limits Documentation.
- Data Standardization: Ensure that contact data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage exceptions and API response errors. This ensures that your application can recover gracefully from unexpected issues.
Enhance Your Integration Strategy with Endgrate
While integrating with the Active Campaign API is a powerful way to enhance your marketing efforts, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including Active Campaign.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can streamline your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/activecampaign
- https://developers.activecampaign.com/reference/authentication
- https://developers.activecampaign.com/reference/url
- https://developers.activecampaign.com/reference/pagination
- https://developers.activecampaign.com/reference/rate-limits
- https://developers.activecampaign.com/reference/list-all-contacts
Ready to get started?