Using the Apollo API to Create Or Update Contacts in Python
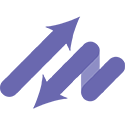
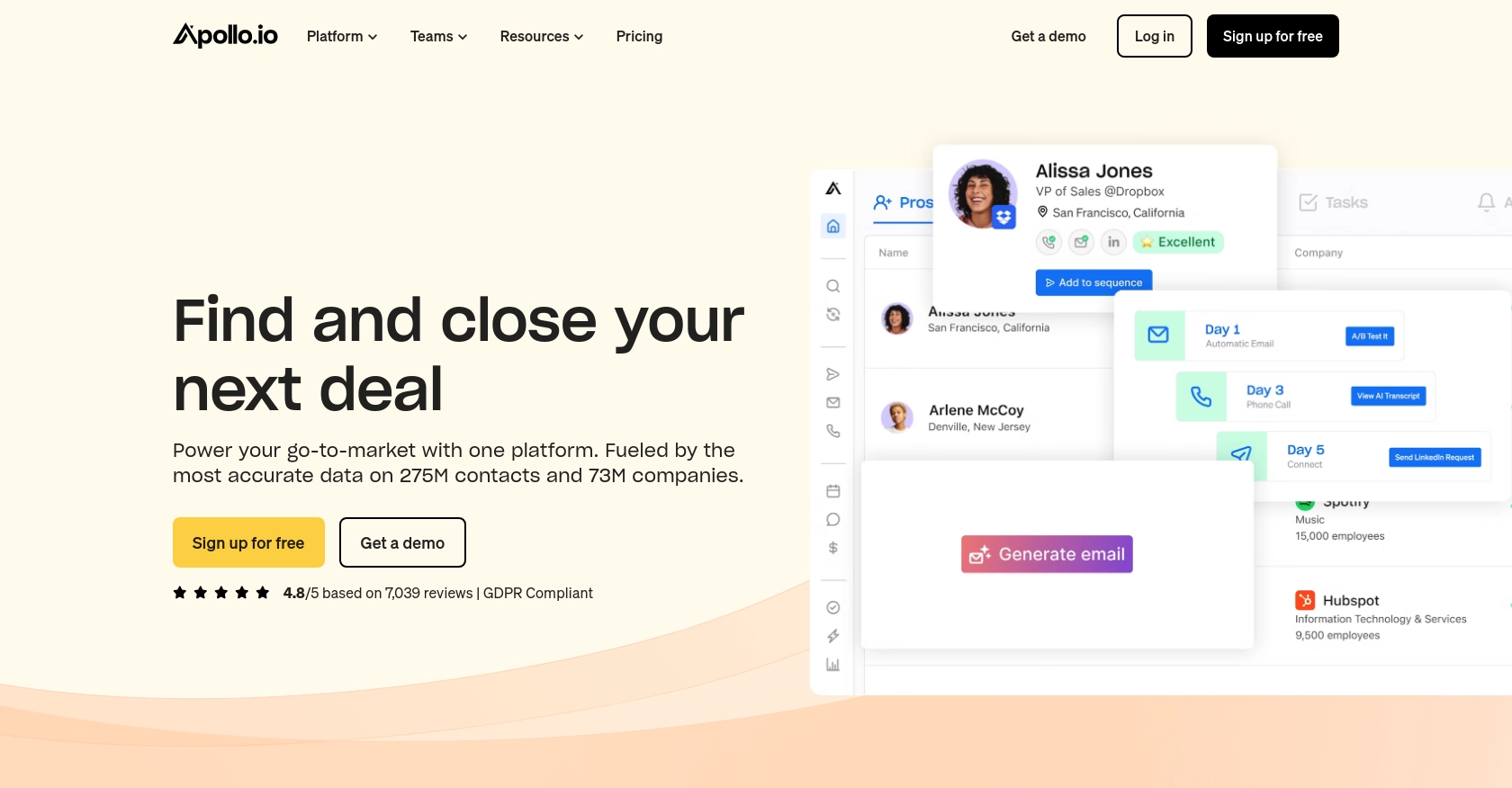
Introduction to Apollo API for Contact Management
Apollo is a powerful sales engagement platform that provides businesses with a comprehensive suite of tools to enhance their sales processes. With its extensive database and advanced features, Apollo helps sales teams identify potential leads, manage customer relationships, and optimize outreach strategies.
Integrating with Apollo's API allows developers to automate and streamline contact management tasks. By using the Apollo API, you can create or update contact information directly from your application, ensuring that your sales team has access to the most up-to-date data. For example, a developer might want to automatically update contact details in Apollo when changes occur in another system, ensuring consistency across platforms.
Setting Up Your Apollo API Test Account
Before you can start integrating with the Apollo API, you need to set up a test account. This will allow you to safely experiment with API calls without affecting your live data. Follow these steps to get started:
Create an Apollo Account
If you don't already have an Apollo account, visit the Apollo website and sign up for a free trial or a demo account. This will give you access to the platform's features and the ability to generate an API key.
Generate Your Apollo API Key
Once your account is set up, you'll need to generate an API key to authenticate your requests. Follow these steps:
- Log in to your Apollo account.
- Navigate to the API settings section in your account dashboard.
- Click on the option to generate a new API key.
- Copy the generated API key and store it securely, as you'll need it for authentication in your API requests.
Configure API Key Authentication
With your API key ready, you can now configure your application to authenticate requests to the Apollo API. Use the following header format in your HTTP requests:
headers = {
'Cache-Control': 'no-cache',
'Content-Type': 'application/json',
'X-Api-Key': 'YOUR_API_KEY_HERE'
}
Replace YOUR_API_KEY_HERE
with the API key you generated earlier.
Understanding Apollo API Rate Limits
It's important to be aware of the rate limits imposed by Apollo to avoid exceeding them during your testing. According to Apollo's documentation, the rate limits are as follows:
- 50 requests per minute
- 100 requests per hour
- 300 requests per day
Ensure your application handles these limits appropriately to prevent disruptions in service.
With your test account and API key set up, you're now ready to start making API calls to create or update contacts in Apollo using Python.
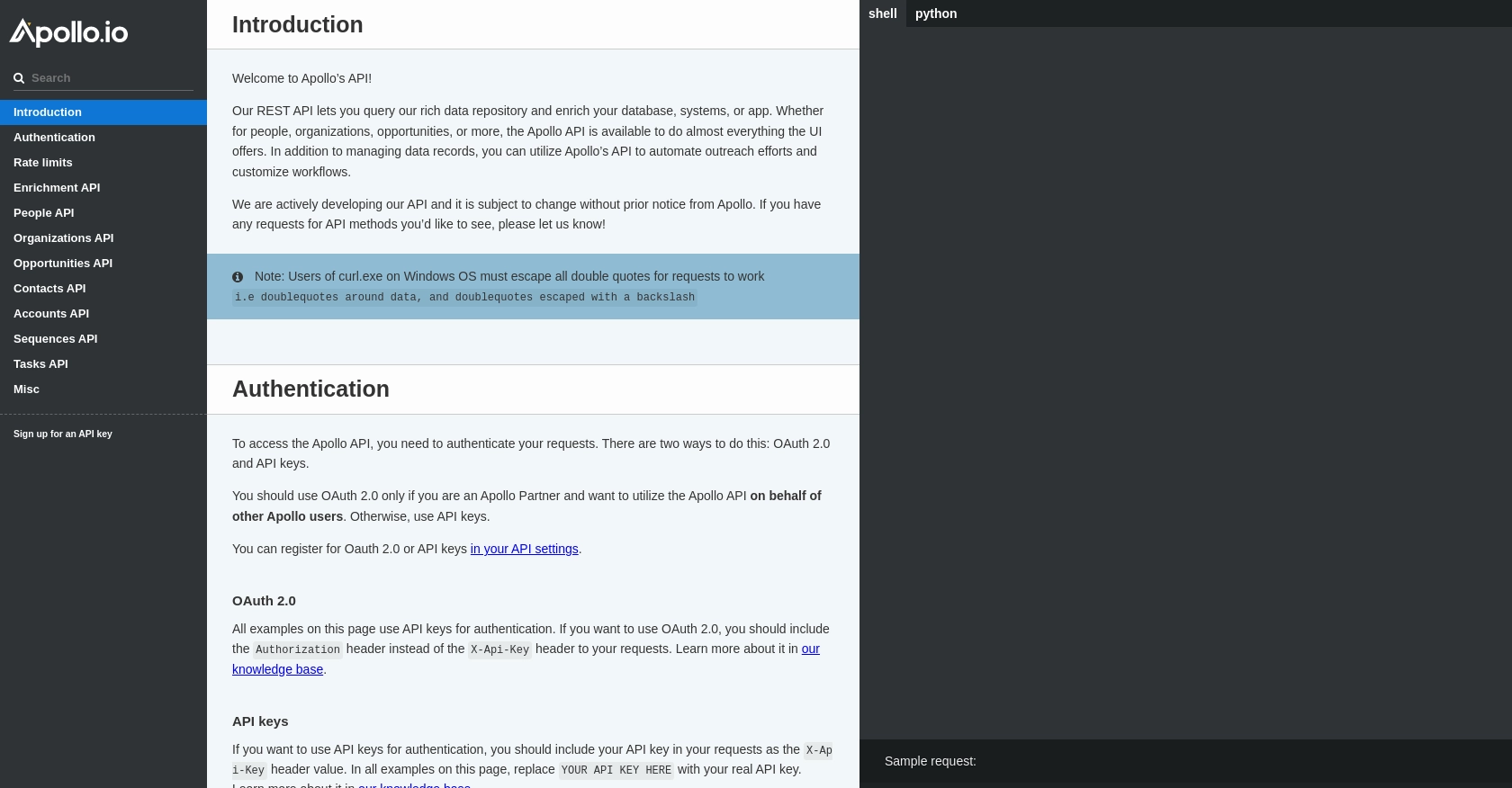
sbb-itb-96038d7
Making API Calls to Create or Update Contacts in Apollo Using Python
To interact with the Apollo API for creating or updating contacts, you'll need to use Python. This section will guide you through the process of setting up your environment, writing the necessary code, and handling responses effectively.
Setting Up Your Python Environment for Apollo API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
Creating a Contact in Apollo Using Python
To create a contact in Apollo, you'll need to send a POST request to the appropriate endpoint. Here's a sample code snippet to help you get started:
import requests
# Define the API endpoint and headers
url = "https://api.apollo.io/v1/contacts"
headers = {
'Cache-Control': 'no-cache',
'Content-Type': 'application/json',
'X-Api-Key': 'YOUR_API_KEY_HERE'
}
# Define the contact data
data = {
"first_name": "Jon",
"last_name": "Snow",
"title": "Lord Commander",
"organization_name": "Westeros"
}
# Make the POST request
response = requests.post(url, headers=headers, json=data)
# Print the response
print(response.json())
Replace YOUR_API_KEY_HERE
with your actual API key. The response will include details of the created contact, which you can verify in your Apollo test account.
Updating a Contact in Apollo Using Python
To update an existing contact, you'll need the contact's ID. Use a PUT request to modify the contact's details. Here's how:
import requests
# Define the API endpoint and headers
contact_id = "YOUR_CONTACT_ID"
url = f"https://api.apollo.io/v1/contacts/{contact_id}"
headers = {
'Cache-Control': 'no-cache',
'Content-Type': 'application/json',
'X-Api-Key': 'YOUR_API_KEY_HERE'
}
# Define the updated contact data
data = {
"first_name": "Jon",
"last_name": "Snow",
"email": "jon.snow@westeros.com",
"title": "King in the North",
"organization_name": "Westeros"
}
# Make the PUT request
response = requests.put(url, headers=headers, json=data)
# Print the response
print(response.json())
Ensure you replace YOUR_CONTACT_ID
and YOUR_API_KEY_HERE
with the correct values. The response will confirm the update, and you can verify the changes in Apollo.
Handling Errors and Verifying API Requests
When making API calls, it's crucial to handle potential errors. Check the response status code to determine if the request was successful. Here's an example:
if response.status_code == 200:
print("Request was successful:", response.json())
else:
print("Failed to complete request:", response.status_code, response.text)
By checking the status code, you can implement logic to handle different scenarios, such as retrying the request or logging the error for further investigation.
With these steps, you can effectively create or update contacts in Apollo using Python, ensuring your sales data remains accurate and up-to-date.
Conclusion and Best Practices for Apollo API Integration
Integrating with the Apollo API to manage contacts offers significant benefits for developers and sales teams. By automating contact creation and updates, you ensure that your sales data is always current and accurate, which can enhance your outreach strategies and improve customer relationships.
Best Practices for Secure and Efficient Apollo API Usage
- Secure API Key Storage: Always store your API keys securely. Avoid hardcoding them in your source code. Instead, use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limits: Be mindful of Apollo's rate limits (50 requests per minute, 100 per hour, and 300 per day). Implement logic to handle rate limiting, such as exponential backoff or request queuing, to prevent disruptions.
- Data Standardization: Ensure that data fields are standardized across systems to maintain consistency. This can involve transforming data formats or validating inputs before making API calls.
- Error Handling: Implement robust error handling to manage API call failures. Log errors for troubleshooting and consider retry mechanisms for transient issues.
Streamlining Integrations with Endgrate
While integrating with Apollo's API can be highly beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects with various platforms, including Apollo. This allows you to build once for each use case, saving time and resources.
By leveraging Endgrate, you can focus on your core product while ensuring a seamless integration experience for your customers. Explore how Endgrate can simplify your integration processes and enhance your product offerings by visiting Endgrate.
Read More
Ready to get started?