Using the Intercom API to Get Companies (with Javascript examples)
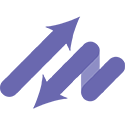
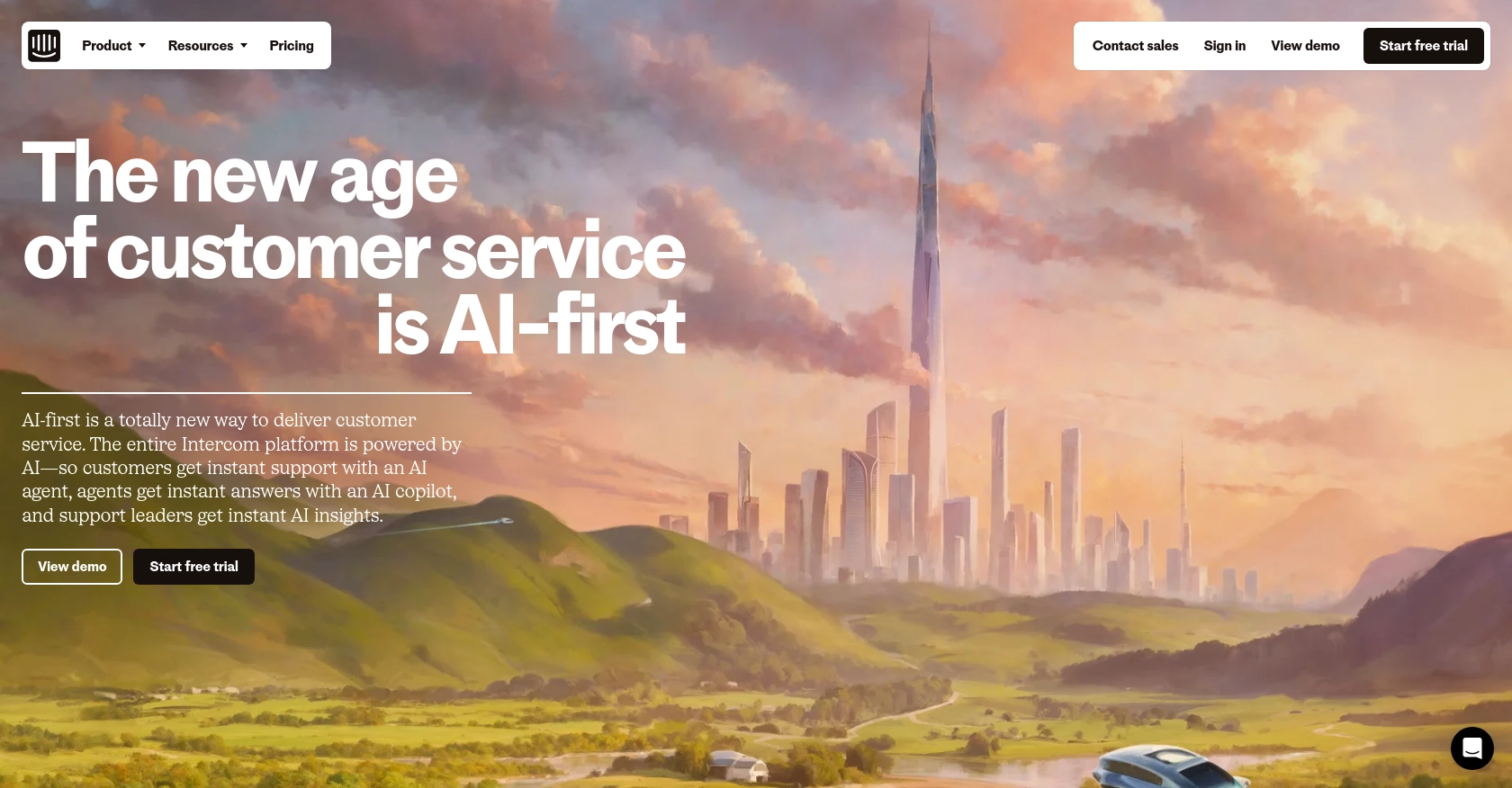
Introduction to Intercom API
Intercom is a powerful customer communication platform that enables businesses to engage with their customers through messaging, automation, and personalized experiences. It offers a suite of tools designed to enhance customer support, marketing, and sales efforts.
Developers might want to integrate with Intercom's API to access and manage company data, which can be crucial for tailoring customer interactions and improving business insights. For example, a developer could use the Intercom API to retrieve a list of companies associated with their product, allowing them to analyze user engagement and optimize marketing strategies.
Setting Up Your Intercom Developer Account
Before you can start using the Intercom API to retrieve company data, you'll need to set up a developer account with Intercom. This involves creating a development workspace and configuring OAuth for authentication. Follow these steps to get started:
Create a Free Intercom Development Workspace
- Visit the Intercom Developer Hub and sign up for a free developer account.
- Once registered, create a new development workspace. This workspace will allow you to test your integration without affecting live data.
Configure OAuth for Intercom API Access
Intercom uses OAuth for secure authentication. Follow these steps to set up OAuth:
- Navigate to the Developer Hub and select your development workspace.
- Go to the "Authentication" section and enable the "Use OAuth" option.
- Provide the necessary information, including redirect URLs. Ensure these URLs are HTTPS as required by Intercom.
- Select the permissions your application needs. For accessing company data, ensure you have the "Read and list users and companies" scope selected.
- Save your changes to generate your
client_id
andclient_secret
.
For more detailed instructions, refer to the Intercom OAuth setup guide.
Generate an Access Token
With OAuth configured, you can now generate an access token to authenticate API requests:
- Direct users to the following URL to authorize your app:
https://app.intercom.com/oauth?client_id=YOUR_CLIENT_ID&state=YOUR_STATE
. - Once authorized, users will be redirected to your specified URL with a code parameter.
- Exchange this code for an access token by making a POST request to
https://api.intercom.io/auth/eagle/token
with yourclient_id
,client_secret
, and the received code.
Keep your access token secure, as it will be used to authenticate your API calls.
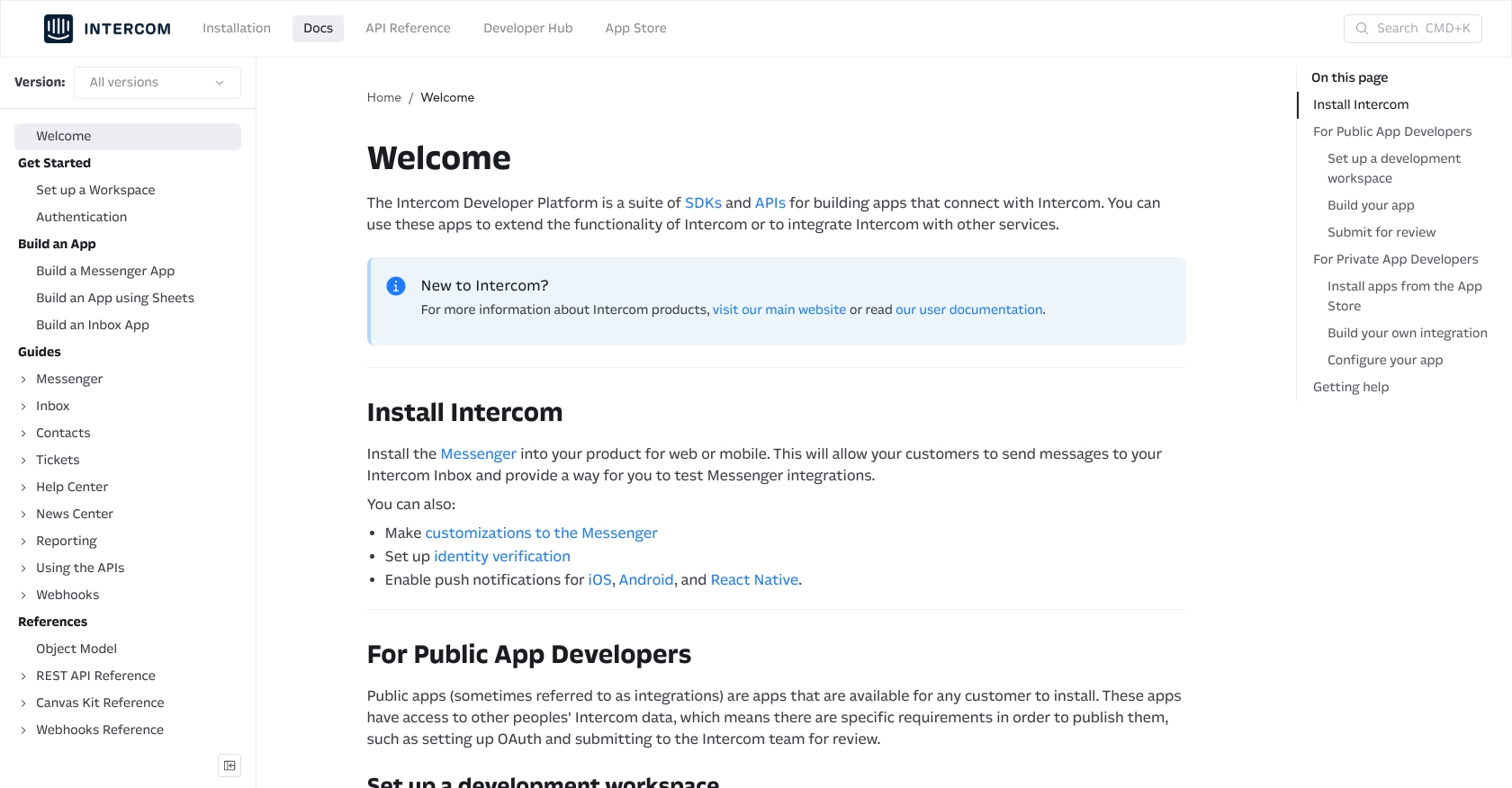
sbb-itb-96038d7
Making API Calls to Retrieve Companies Using Intercom API with JavaScript
To interact with the Intercom API and retrieve company data, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling responses and errors effectively.
Setting Up Your JavaScript Environment for Intercom API
Before making API calls, ensure you have Node.js installed on your machine. You can download it from the official Node.js website. Additionally, you'll need the axios
library to handle HTTP requests. Install it using npm:
npm install axios
Writing JavaScript Code to Fetch Companies from Intercom
Create a new JavaScript file named getCompanies.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.intercom.io/companies';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Intercom-Version': '2.11',
'Accept': 'application/json'
};
// Make a GET request to the API
axios.get(endpoint, { headers })
.then(response => {
// Handle successful response
const companies = response.data.data;
companies.forEach(company => {
console.log(`Company Name: ${company.name}, ID: ${company.id}`);
});
})
.catch(error => {
// Handle errors
if (error.response) {
console.error(`Error: ${error.response.status} - ${error.response.data.message}`);
} else {
console.error(`Error: ${error.message}`);
}
});
Replace YOUR_ACCESS_TOKEN
with the access token you generated earlier. This code makes a GET request to the Intercom API to retrieve a list of companies and logs their names and IDs to the console.
Running the JavaScript Code and Verifying Results
Run the script using Node.js:
node getCompanies.js
You should see the list of companies printed in the console. Verify the results by checking the data in your Intercom development workspace.
Handling Errors and Understanding Intercom API Error Codes
When making API calls, it's crucial to handle potential errors. The Intercom API may return various error codes, such as:
- 401 Unauthorized: Indicates an issue with authentication, such as an invalid access token.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server side.
Refer to the Intercom API documentation for more details on error handling.
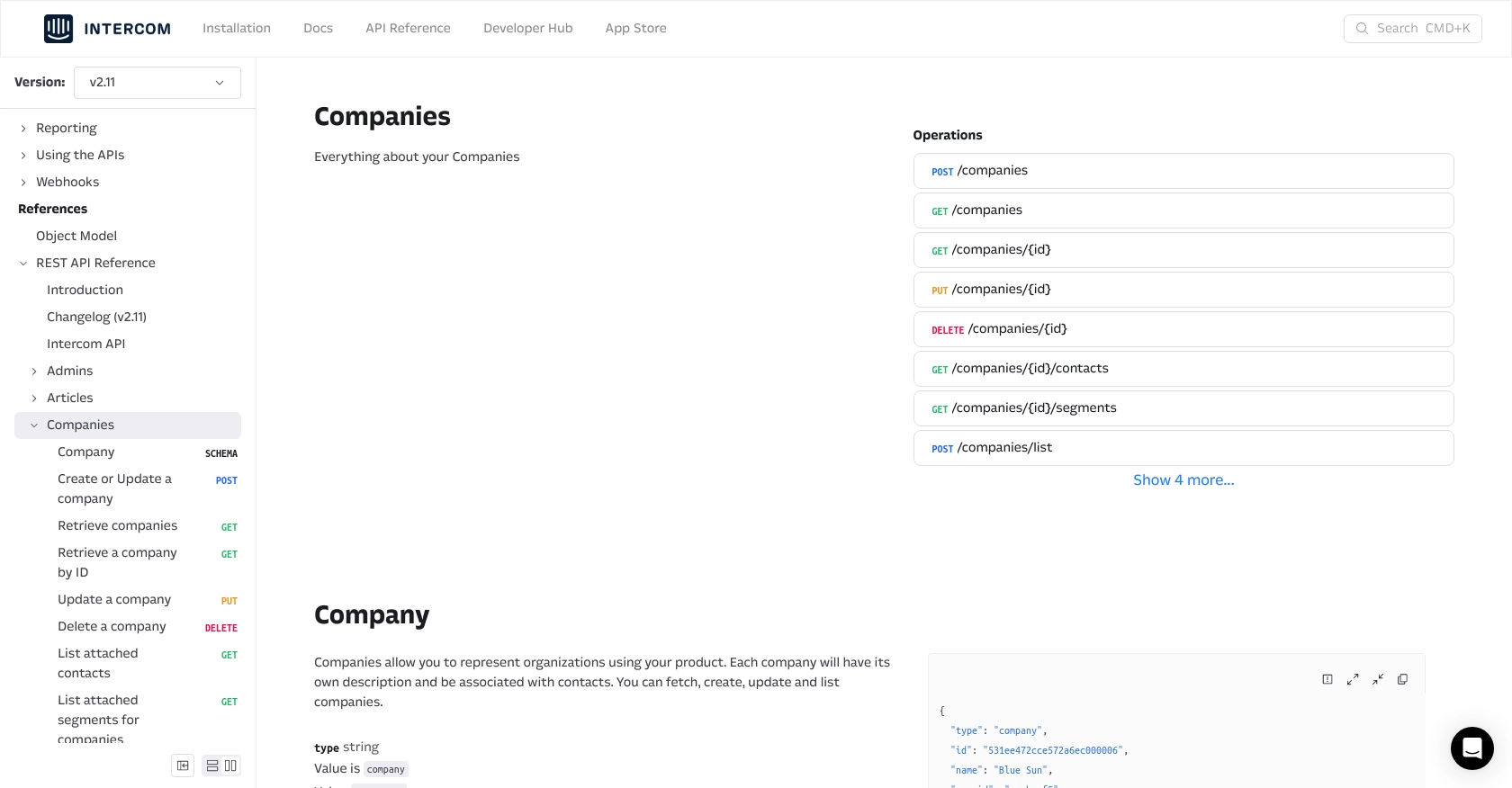
Conclusion and Best Practices for Using Intercom API with JavaScript
Integrating with the Intercom API allows developers to efficiently manage company data, enhancing customer interactions and business insights. By following the steps outlined in this guide, you can successfully authenticate and retrieve company information using JavaScript.
Best Practices for Secure and Efficient Intercom API Integration
- Secure Storage of Credentials: Always store your access tokens securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limiting: Be mindful of Intercom's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data retrieved from Intercom is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage API errors effectively. Refer to the Intercom API documentation for detailed error code explanations.
Streamlining Integrations with Endgrate
For developers looking to simplify and scale their integration efforts, consider using Endgrate. With Endgrate, you can streamline your integration process, saving time and resources by outsourcing complex integrations and focusing on your core product. Endgrate offers a unified API endpoint that connects to multiple platforms, including Intercom, providing an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?