How to Create or Update Customers with the Sage 100 API in PHP
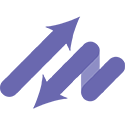
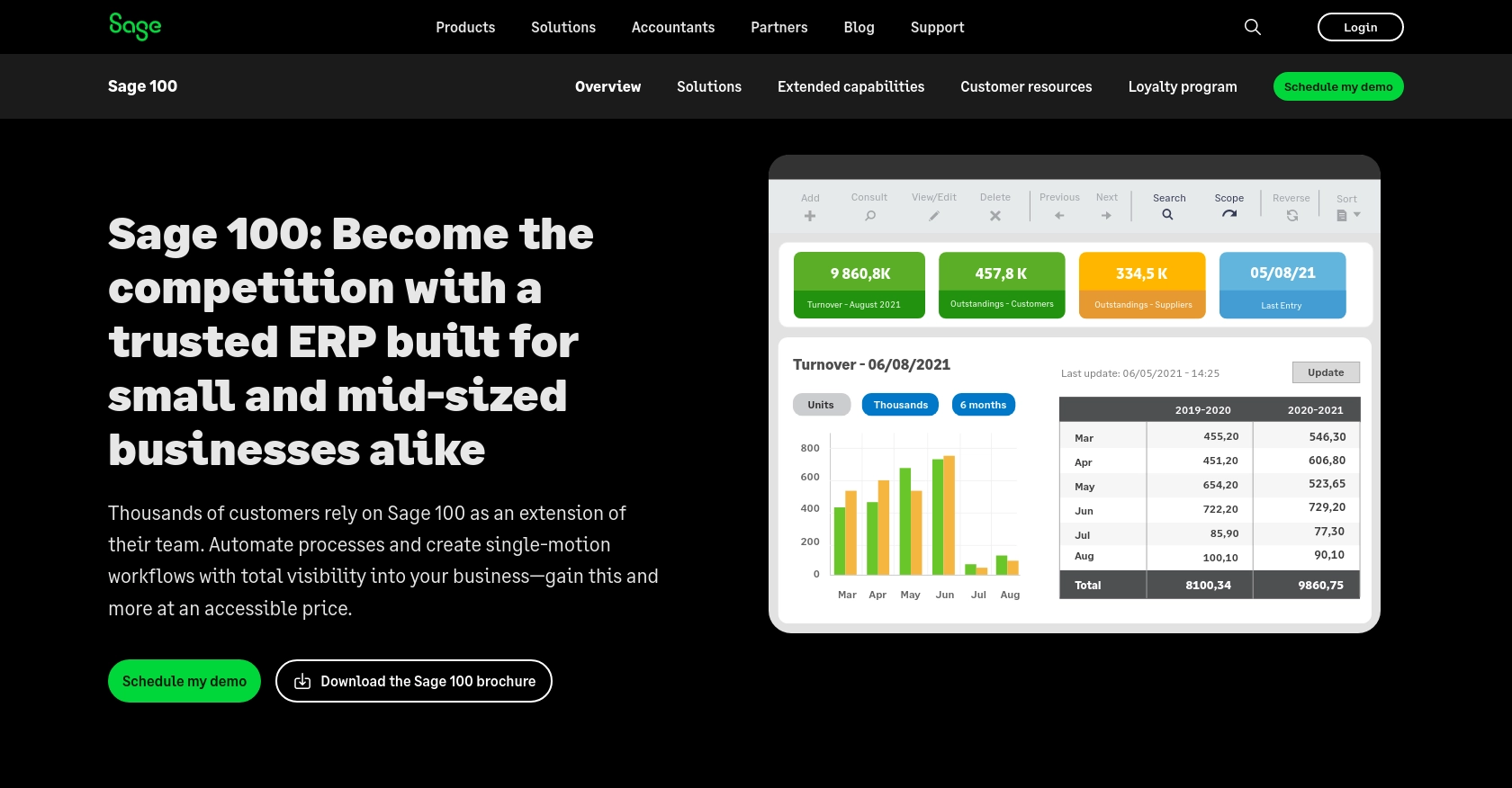
Introduction to Sage 100 API Integration
Sage 100 is a comprehensive ERP solution tailored for small to medium-sized businesses, offering robust features for accounting, inventory management, and customer relationship management. Its flexibility and scalability make it a preferred choice for businesses looking to streamline their operations and improve efficiency.
Integrating with the Sage 100 API allows developers to automate and enhance various business processes, such as managing customer data. For example, you can use the Sage 100 API to create or update customer records directly from your application, ensuring that your customer information is always up-to-date and accurate.
Setting Up Your Sage 100 Test/Sandbox Account
Before you can begin integrating with the Sage 100 API, it's essential to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data. Follow these steps to configure your Sage 100 environment for development:
Install and Configure the Sage 100 ODBC Driver
To interact with the Sage 100 API, you need to ensure that the Sage 100 ODBC driver is installed and properly configured on your system. This involves setting up a Data Source Name (DSN) to connect to the Sage 100 ERP system.
- Access the ODBC Data Source Administrator on your system.
- Create a new DSN that connects to the Sage 100 database using the correct server, database, and authentication settings.
- Ensure the Sage 100 ODBC driver is running as an application or service, depending on your server configuration.
For detailed instructions, refer to the Sage 100 ODBC Driver Configuration Guide.
Configuring the Sage 100 Server
Once the ODBC driver is set up, configure the Sage 100 server to allow API interactions:
- Open the Server Manager on the Sage 100 Advanced server.
- Navigate to Services and locate the Sage 100 Client Server ODBC Driver Service.
- Set the Startup type to Automatic and start the service.
Workstation Configuration for Sage 100 API Access
To ensure your workstation can access the Sage 100 API, perform the following steps:
- In Sage 100 Advanced, go to Library Master > Setup > System Configuration.
- On the ODBC Driver tab, enable the C/S ODBC Driver.
- Enter the server name or IP address and configure the server port if necessary.
Test the ODBC data source to ensure connectivity. For more details, consult the Sage 100 Workstation Configuration Guide.
Creating a Sage 100 Sandbox Environment
To safely test API interactions, consider setting up a sandbox environment:
- Duplicate your Sage 100 database to create a separate testing environment.
- Ensure all API calls are directed to this sandbox to prevent data corruption in your live system.
With your Sage 100 test account and environment configured, you're ready to begin integrating and testing API calls using PHP.
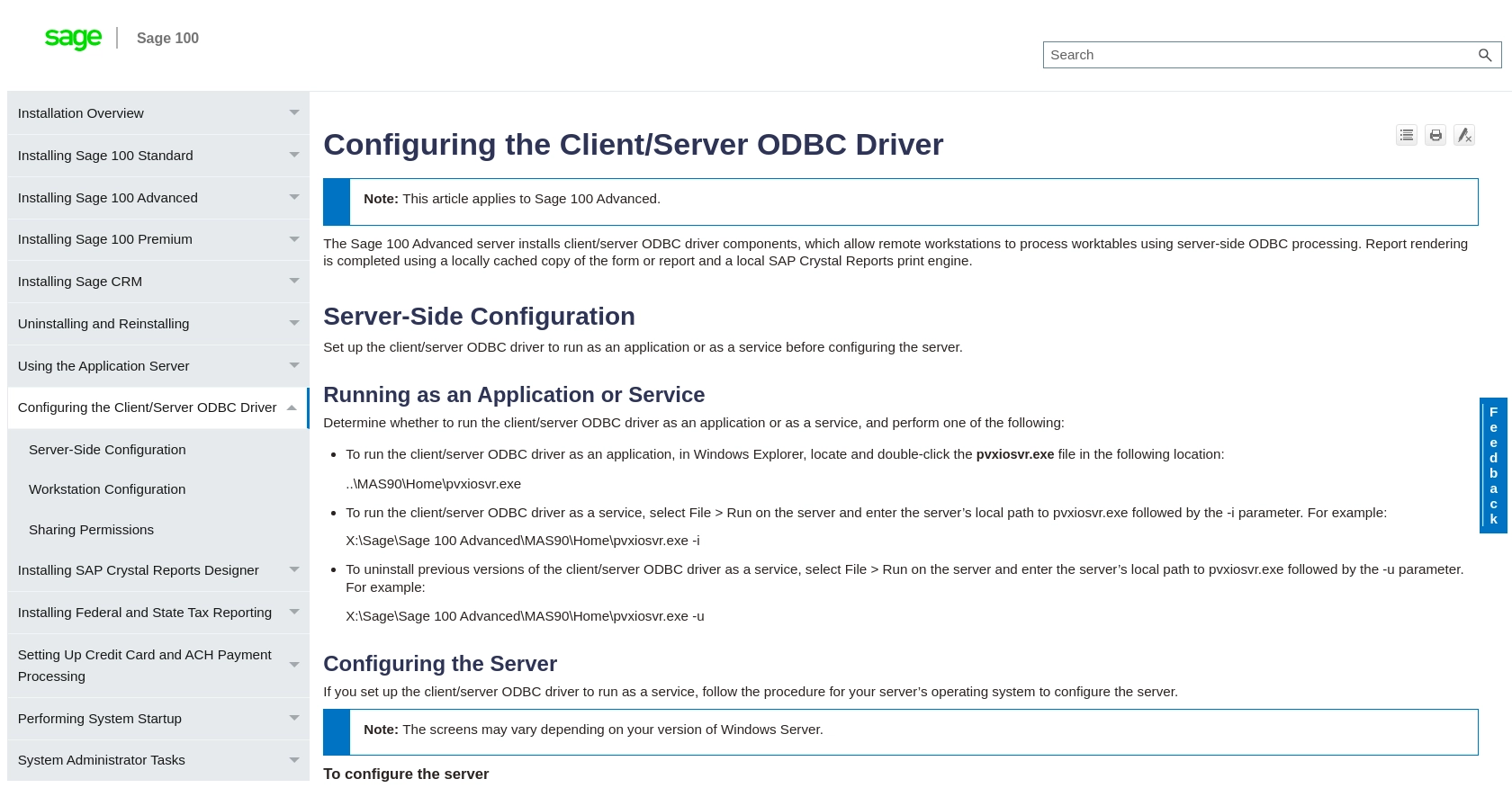
sbb-itb-96038d7
Making API Calls to Create or Update Customers in Sage 100 Using PHP
Prerequisites for PHP Integration with Sage 100 API
Before diving into the code, ensure you have the following prerequisites set up on your machine:
- PHP 7.4 or higher installed.
- ODBC extension enabled in your PHP configuration.
- Access to the Sage 100 ODBC Data Source Name (DSN) configured as per the Sage 100 ODBC Driver Configuration Guide.
Installing Necessary PHP Dependencies
To interact with the Sage 100 API, you need to ensure that your PHP environment is equipped with the necessary libraries. Use Composer to manage dependencies:
composer require ext-odbc
Creating a Customer in Sage 100 Using PHP
To create a new customer record in Sage 100, follow the steps below:
<?php
// Establish ODBC connection
$dsn = 'SOTAMAS90'; // Replace with your DSN
$user = 'your_username'; // Replace with your Sage 100 username
$password = 'your_password'; // Replace with your Sage 100 password
$conn = odbc_connect($dsn, $user, $password);
if (!$conn) {
die('Connection failed: ' . odbc_errormsg());
}
// Define SQL query to create a customer
$sql = "INSERT INTO AR_Customer (CustomerNo, CustomerName, AddressLine1) VALUES ('CUST001', 'New Customer', '123 Main St')";
// Execute the query
$result = odbc_exec($conn, $sql);
if ($result) {
echo 'Customer created successfully.';
} else {
echo 'Failed to create customer: ' . odbc_errormsg($conn);
}
// Close the connection
odbc_close($conn);
?>
Replace your_username
and your_password
with your actual Sage 100 credentials. This script inserts a new customer into the Sage 100 database. Ensure the fields match your database schema.
Updating a Customer in Sage 100 Using PHP
To update an existing customer record, modify the SQL query as shown below:
<?php
// Establish ODBC connection
$dsn = 'SOTAMAS90'; // Replace with your DSN
$user = 'your_username'; // Replace with your Sage 100 username
$password = 'your_password'; // Replace with your Sage 100 password
$conn = odbc_connect($dsn, $user, $password);
if (!$conn) {
die('Connection failed: ' . odbc_errormsg());
}
// Define SQL query to update a customer
$sql = "UPDATE AR_Customer SET CustomerName = 'Updated Customer' WHERE CustomerNo = 'CUST001'";
// Execute the query
$result = odbc_exec($conn, $sql);
if ($result) {
echo 'Customer updated successfully.';
} else {
echo 'Failed to update customer: ' . odbc_errormsg($conn);
}
// Close the connection
odbc_close($conn);
?>
This script updates the customer name for the customer with CustomerNo
'CUST001'. Adjust the fields and values as needed for your use case.
Verifying API Call Success in Sage 100
After executing the API calls, verify the changes in your Sage 100 sandbox environment:
- Log in to the Sage 100 interface.
- Navigate to the Accounts Receivable module.
- Check the customer records to confirm the creation or update.
Handling Errors and Exceptions
It's crucial to handle potential errors during API interactions. Use try-catch blocks to manage exceptions and log errors for troubleshooting:
<?php
try {
// Your code here
} catch (Exception $e) {
error_log('Error: ' . $e->getMessage());
echo 'An error occurred. Please check the logs.';
}
?>
Implementing error handling ensures that your application can gracefully manage issues without crashing.
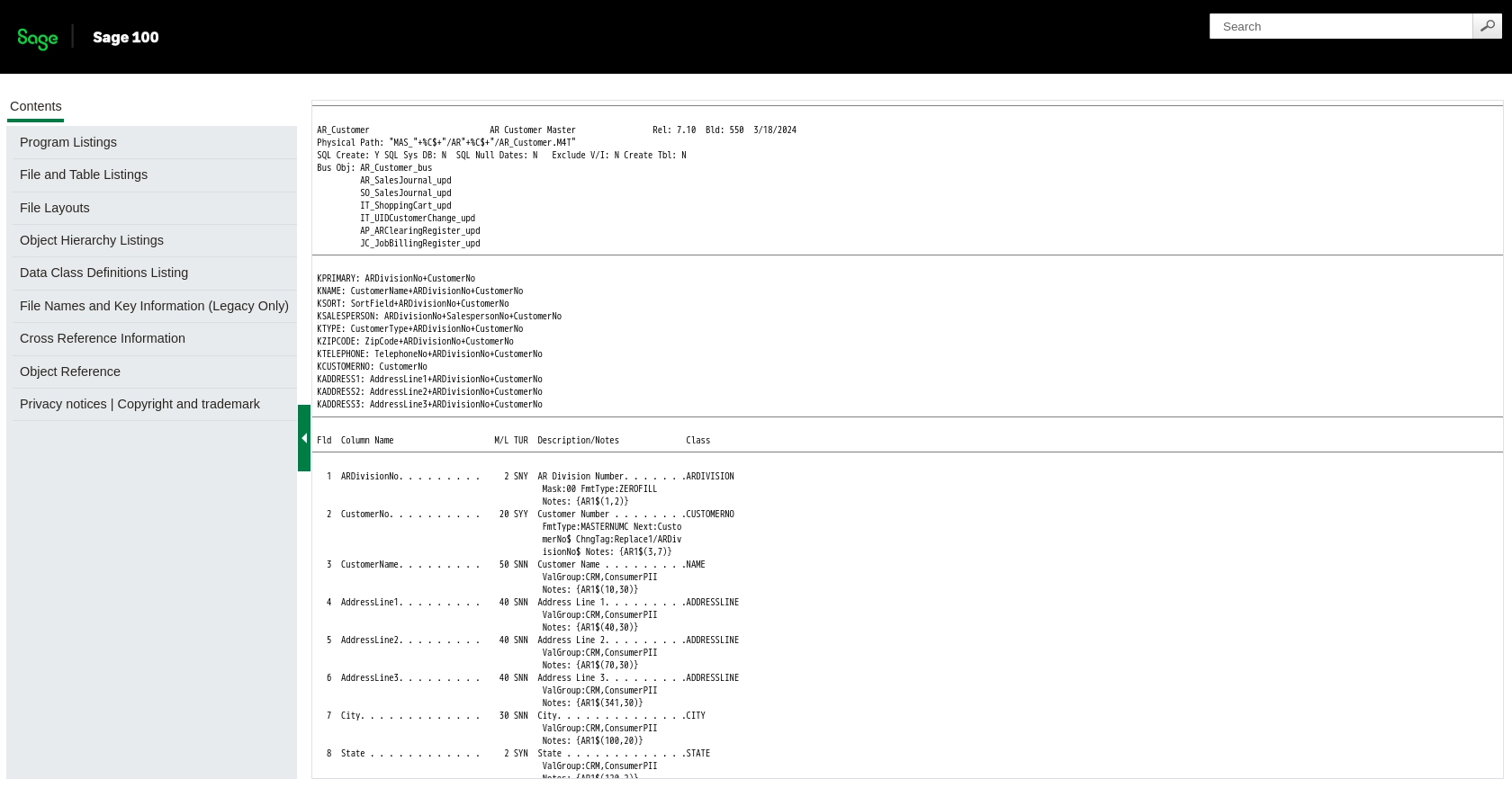
Conclusion and Best Practices for Sage 100 API Integration
Integrating with the Sage 100 API using PHP provides a powerful way to automate and streamline customer management processes. By following the steps outlined in this guide, you can efficiently create and update customer records, ensuring your data remains accurate and up-to-date.
Best Practices for Secure and Efficient Sage 100 API Usage
- Secure Credential Storage: Always store user credentials securely, using encryption and secure storage solutions to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Sage 100 API and implement logic to handle retries or backoff strategies to avoid exceeding these limits.
- Data Validation and Transformation: Ensure that data being sent to the API is validated and transformed as needed to match the expected formats and standards of Sage 100.
- Error Logging and Monitoring: Implement comprehensive error logging and monitoring to quickly identify and resolve issues that may arise during API interactions.
Enhancing Integration Efficiency with Endgrate
While integrating with Sage 100 directly can be effective, using a tool like Endgrate can further enhance your integration capabilities. Endgrate offers a unified API endpoint that simplifies the process of connecting to multiple platforms, including Sage 100. This allows you to focus on your core product while outsourcing complex integration tasks.
By leveraging Endgrate, you can save time and resources, build integrations once for multiple use cases, and provide an intuitive integration experience for your customers. Explore how Endgrate can streamline your integration processes by visiting Endgrate's website.
Read More
Ready to get started?