How to Create or Update Prospects with the Woodpecker API in PHP
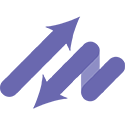
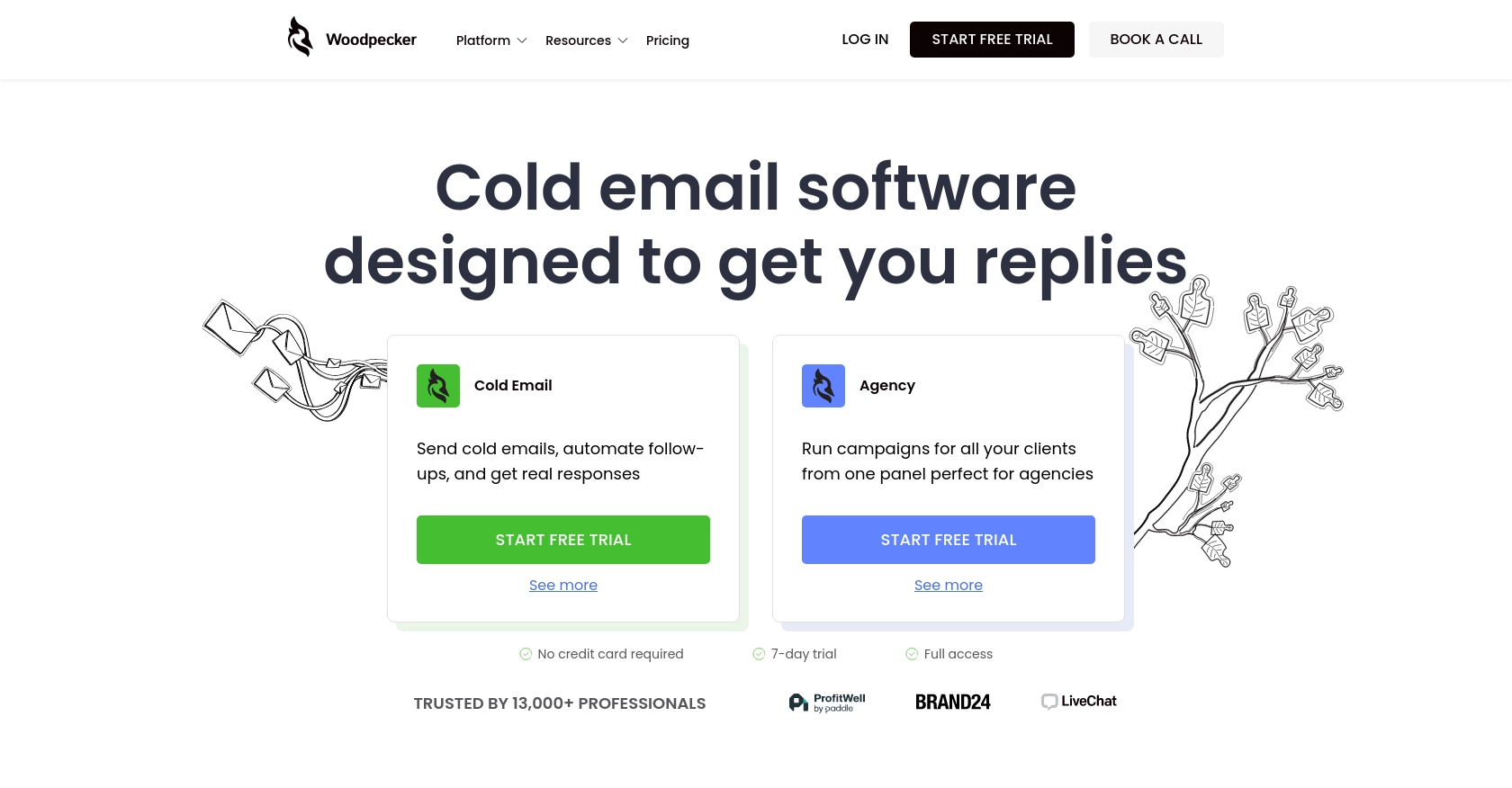
Introduction to Woodpecker API for Prospect Management
Woodpecker is a powerful tool designed to enhance cold email campaigns and automate follow-ups for businesses. It offers a comprehensive platform for managing prospects, tracking engagement, and optimizing outreach efforts. By integrating with the Woodpecker API, developers can streamline the process of managing prospect data, enabling seamless communication and improved campaign efficiency.
Connecting with the Woodpecker API allows developers to automate the creation and updating of prospect information directly from their applications. For example, a developer might want to automatically update prospect details in Woodpecker whenever there is a change in their CRM system, ensuring that all outreach efforts are based on the most current data.
Setting Up Your Woodpecker API Account for Prospect Management
To begin integrating with the Woodpecker API, you'll need to set up your account and generate an API key. This key will allow you to authenticate your requests and interact with Woodpecker's prospect management features.
Creating a Woodpecker Account
If you don't already have a Woodpecker account, you can sign up for a free trial on the Woodpecker website. This will give you access to the platform's features and allow you to explore its capabilities.
- Visit the Woodpecker website.
- Click on "Start free trial" and follow the instructions to create your account.
- Once your account is set up, log in to access the dashboard.
Generating an API Key for Woodpecker Integration
With your account ready, the next step is to generate an API key. This key is essential for authenticating your API requests.
- Log into your Woodpecker account at app.woodpecker.co.
- Navigate to the Marketplace, then select "INTEGRATIONS" and "API keys."
- Click the green "CREATE A KEY" button to generate a new API key.
- Copy the generated API key and store it securely, as you'll need it for API requests.
For more detailed instructions, refer to the Woodpecker API Key Generation Guide.
Understanding API Key Authentication
Woodpecker uses API key-based authentication. When making API requests, include your API key in the headers to authenticate:
$headers = [
"Authorization: Basic <API_KEY>"
];
Ensure your API key is encoded in Base64 format before using it in requests. For more information, visit the Woodpecker Authentication Documentation.
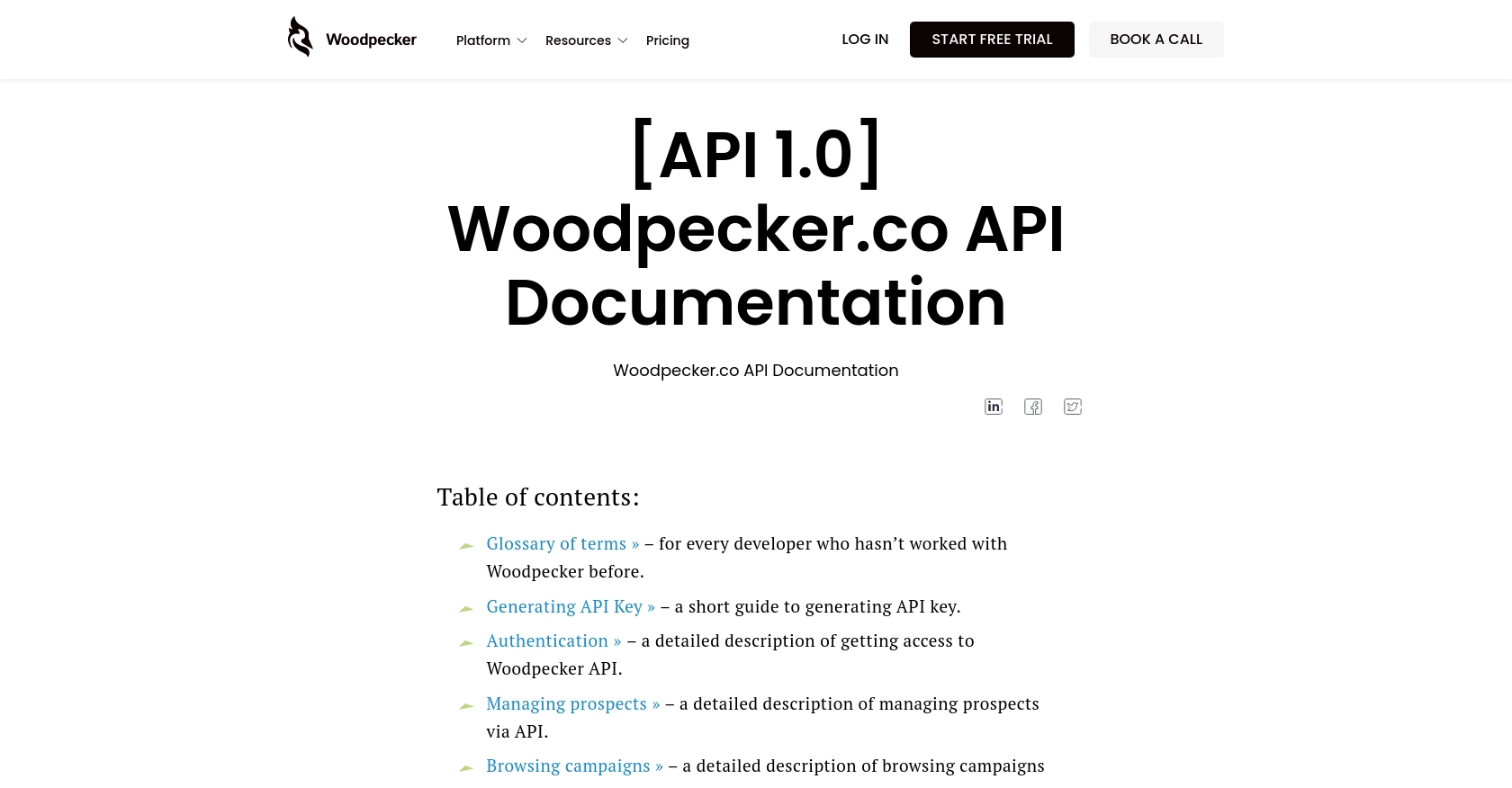
sbb-itb-96038d7
Making API Calls to Create or Update Prospects with Woodpecker API in PHP
To interact with the Woodpecker API for creating or updating prospects, you need to set up your PHP environment and make HTTP requests using the API key for authentication. This section will guide you through the process of making these API calls effectively.
Setting Up Your PHP Environment for Woodpecker API Integration
Before making API calls, ensure you have the following prerequisites:
- PHP 7.4 or later installed on your machine.
- Composer for managing dependencies.
Install the necessary dependencies using Composer. The guzzlehttp/guzzle
library is recommended for making HTTP requests:
composer require guzzlehttp/guzzle
Creating a New Prospect with Woodpecker API
To create a new prospect, you will use the POST method to send prospect data to the Woodpecker API. Here's an example of how to do this in PHP:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiKey = 'Your_Base64_Encoded_API_KEY';
$response = $client->post('https://api.woodpecker.co/rest/v1/add_prospects_list', [
'headers' => [
'Authorization' => 'Basic ' . $apiKey,
'Content-Type' => 'application/json'
],
'json' => [
'update' => 'true',
'prospects' => [
[
'email' => 'newprospect@example.com',
'first_name' => 'John',
'last_name' => 'Doe',
'company' => 'Example Corp',
'industry' => 'Software',
'website' => 'http://example.com',
'linkedin_url' => 'https://linkedin.com/in/johndoe',
'tags' => '#NewProspect',
'title' => 'Developer'
]
]
]
]);
echo $response->getBody();
Replace Your_Base64_Encoded_API_KEY
with your actual API key. This code sends a request to add a new prospect to your Woodpecker account. If successful, the response will contain the prospect's details.
Updating an Existing Prospect with Woodpecker API
To update an existing prospect, include the prospect's ID in your request. Here's how you can update a prospect's information:
$response = $client->post('https://api.woodpecker.co/rest/v1/add_prospects_list', [
'headers' => [
'Authorization' => 'Basic ' . $apiKey,
'Content-Type' => 'application/json'
],
'json' => [
'update' => 'true',
'prospects' => [
[
'id' => 12345, // Replace with the actual prospect ID
'email' => 'updatedprospect@example.com',
'first_name' => 'Jane',
'last_name' => 'Doe',
'company' => 'Updated Corp'
]
]
]
]);
echo $response->getBody();
This request updates the specified prospect's details. Ensure you replace the prospect ID with the correct one from your database.
Handling API Responses and Errors
After making an API call, check the response to ensure the request was successful. Woodpecker API returns a status code and message. Handle errors by checking the response code:
if ($response->getStatusCode() === 200) {
echo "Prospect updated successfully.";
} else {
echo "Error: " . $response->getBody();
}
For a complete list of error codes, refer to the Woodpecker Error Code Documentation.
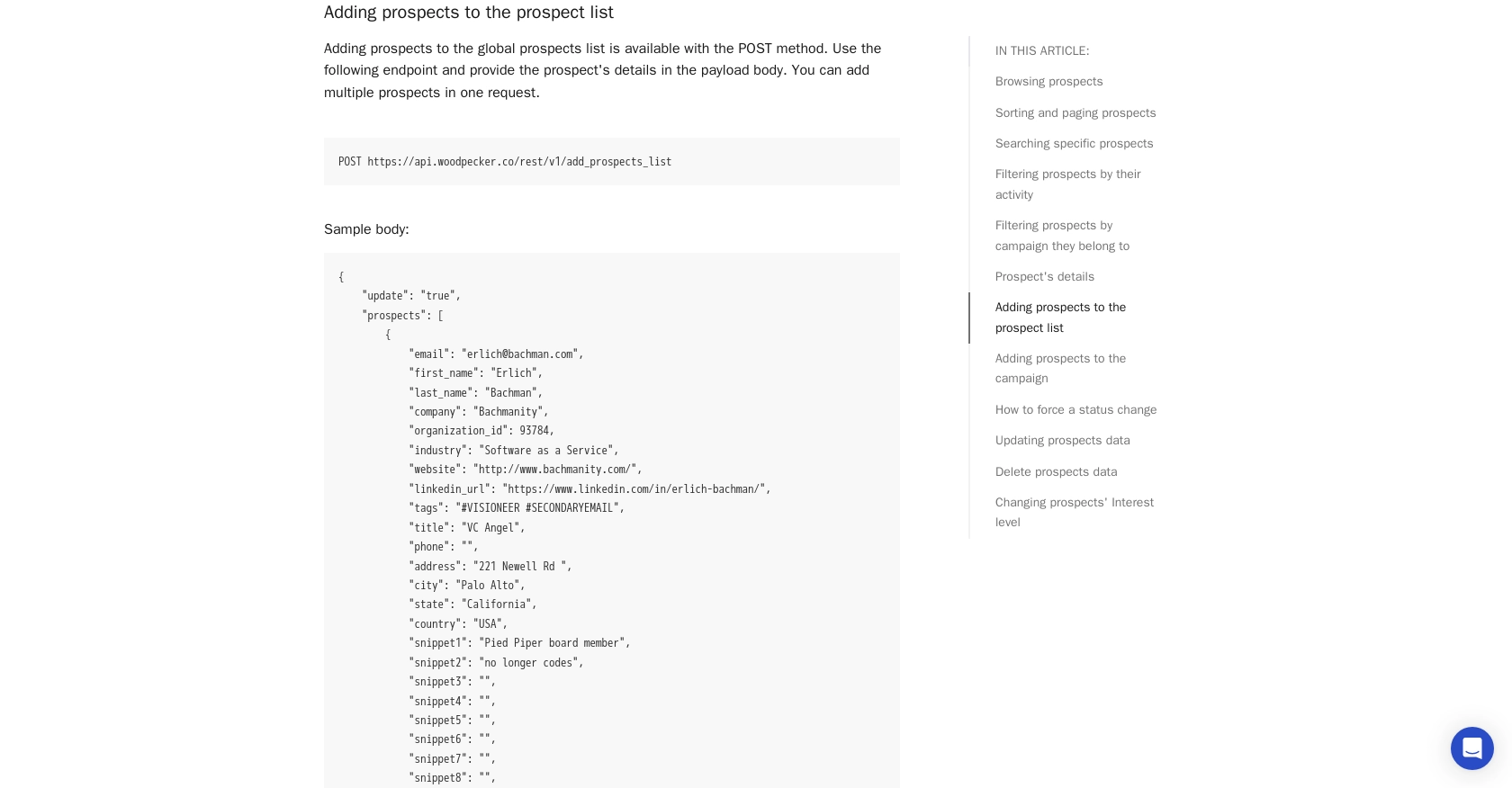
Conclusion and Best Practices for Using Woodpecker API in PHP
Integrating with the Woodpecker API allows developers to efficiently manage prospect data, enhancing the effectiveness of cold email campaigns. By automating the creation and updating of prospects, you can ensure that your outreach efforts are always based on the most current information.
Best Practices for Secure and Efficient Woodpecker API Integration
- Secure API Key Storage: Always store your API keys securely and avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limits: Although Woodpecker allows unlimited API calls per month, remember that only one request can be processed at a time. Plan your requests accordingly to avoid dropped requests.
- Data Standardization: Ensure that the data you send to Woodpecker is standardized and consistent to maintain data integrity across your systems.
- Error Handling: Implement robust error handling to manage API response codes effectively. This will help you quickly identify and resolve issues.
Streamline Your Integrations with Endgrate
While integrating with the Woodpecker API can significantly enhance your email campaigns, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product. By using Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers.
Explore how Endgrate can help you save time and resources by visiting Endgrate today.
Read More
Ready to get started?