Using the Salesflare API to Create or Update Contacts in Javascript
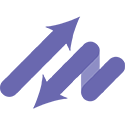
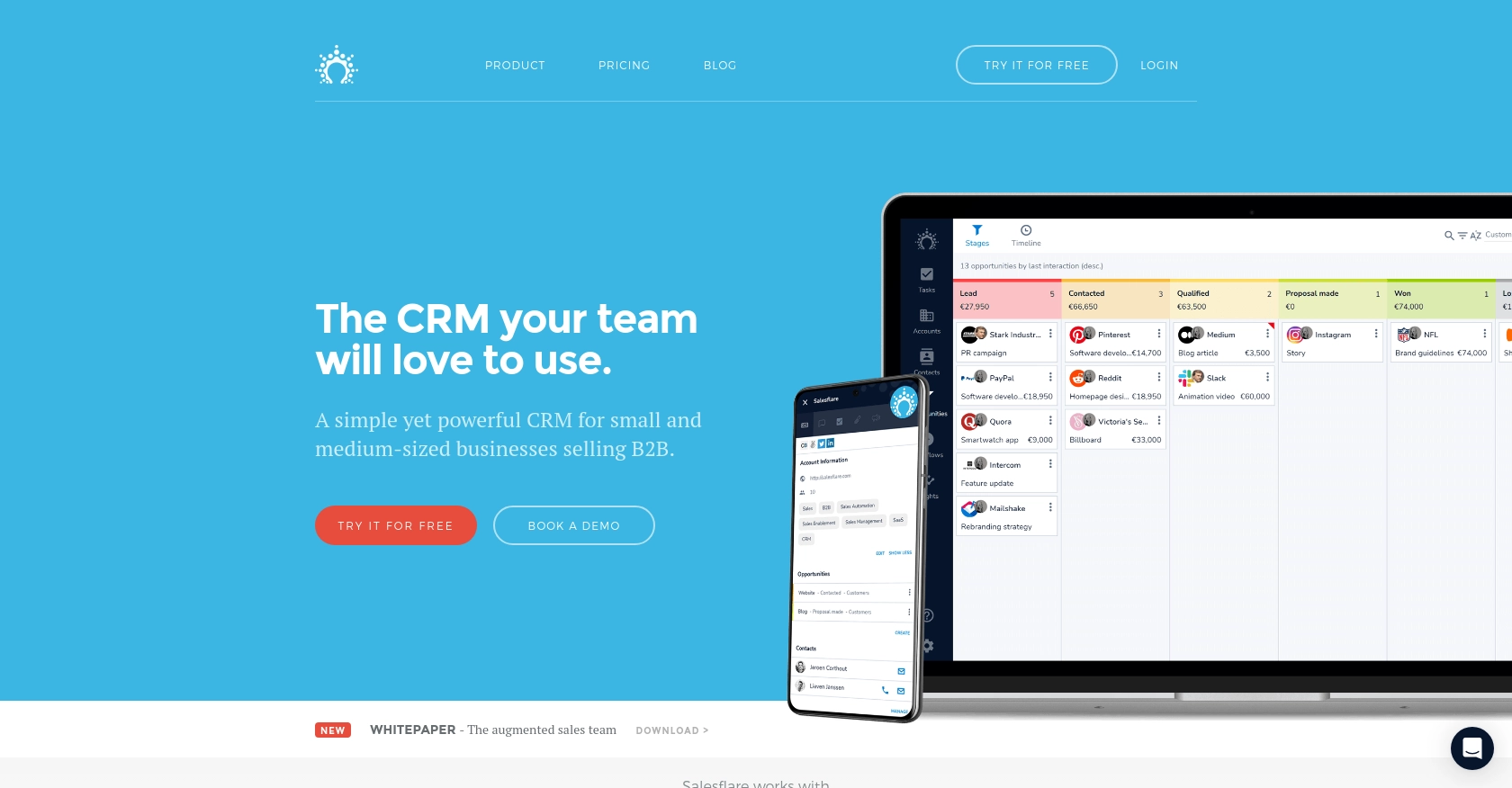
Introduction to Salesflare API Integration
Salesflare is a powerful CRM platform designed to simplify sales processes for small and medium-sized businesses. It offers a user-friendly interface and a range of features that help sales teams manage leads, track customer interactions, and automate follow-ups.
Integrating with Salesflare's API allows developers to enhance their applications by seamlessly managing contact data. For example, a developer might use the Salesflare API to automatically create or update contact information from a web form submission, ensuring that sales teams always have the most current data at their fingertips.
Setting Up Your Salesflare Test Account for API Integration
Before you can start integrating with the Salesflare API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Salesflare offers a straightforward process to get started with their platform.
Creating a Salesflare Account
If you don't already have a Salesflare account, you can sign up for a free trial on their website. This trial will give you access to all the features you need to test the API integration.
- Visit the Salesflare website and click on the "Try for Free" button.
- Follow the on-screen instructions to create your account. You'll need to provide basic information such as your name, email address, and company details.
- Once your account is set up, you'll be logged into the Salesflare dashboard.
Generating Your Salesflare API Key
Salesflare uses API key-based authentication to secure API requests. Follow these steps to generate your API key:
- In the Salesflare dashboard, navigate to the "Settings" section.
- Look for the "API & Integrations" option and click on it.
- Here, you will find the option to generate a new API key. Click on "Generate API Key" and follow the prompts.
- Once generated, copy the API key and store it securely. You will need this key to authenticate your API requests.
For more detailed information, you can refer to the Salesflare API documentation.
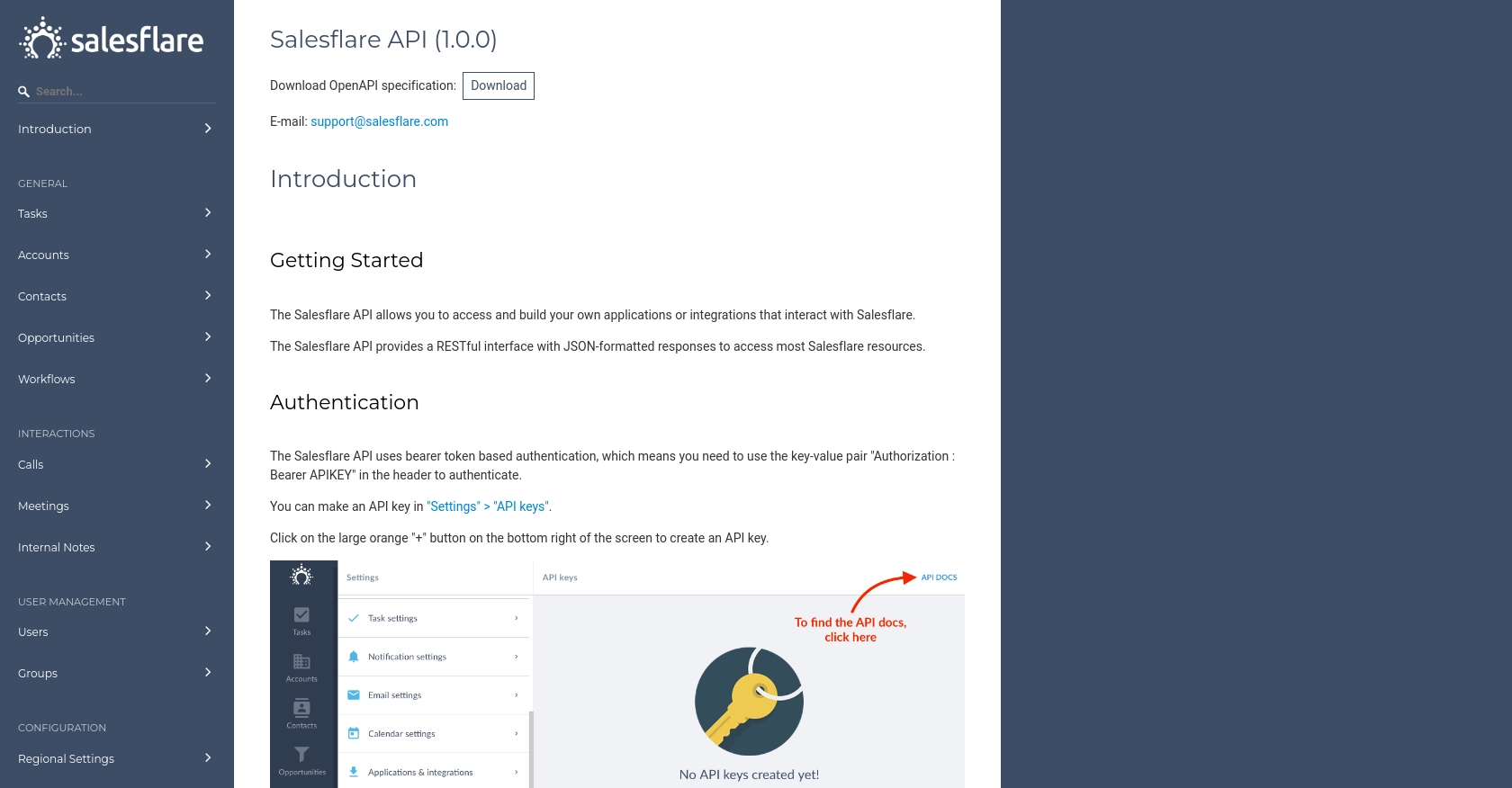
sbb-itb-96038d7
Making API Calls to Salesflare Using JavaScript
To interact with the Salesflare API using JavaScript, you'll need to set up your environment and write code to make HTTP requests. This section will guide you through the process of creating or updating contacts using JavaScript.
Setting Up Your JavaScript Environment for Salesflare API
Before making API calls, ensure you have Node.js installed on your machine. Node.js allows you to run JavaScript code outside of a browser, making it ideal for server-side applications.
- Download and install Node.js from the official website.
- Once installed, open your terminal and verify the installation by running
node -v
andnpm -v
. - Create a new directory for your project and navigate into it using the terminal.
- Initialize a new Node.js project by running
npm init -y
. - Install the
axios
library to handle HTTP requests by runningnpm install axios
.
Creating or Updating Contacts with Salesflare API
With your environment set up, you can now write the JavaScript code to create or update contacts in Salesflare. The following example demonstrates how to make a POST request to the Salesflare API.
const axios = require('axios');
// Your Salesflare API key
const apiKey = 'Your_API_Key';
// Function to create or update a contact
async function createOrUpdateContact(contactData) {
try {
const response = await axios.post('https://api.salesflare.com/contacts', contactData, {
headers: {
'Authorization': `Bearer ${apiKey}`,
'Content-Type': 'application/json'
}
});
console.log('Contact created/updated successfully:', response.data);
} catch (error) {
console.error('Error creating/updating contact:', error.response ? error.response.data : error.message);
}
}
// Example contact data
const contactData = {
email: 'example@salesflare.com',
firstName: 'John',
lastName: 'Doe'
};
// Call the function
createOrUpdateContact(contactData);
Replace Your_API_Key
with the API key you generated earlier. The contactData
object contains the contact information you wish to create or update. You can modify this object to include additional fields as needed.
Verifying API Call Success and Handling Errors
After running the script, you should verify that the contact was created or updated in your Salesflare test account. Check the Salesflare dashboard to confirm the changes.
If the API call fails, the error handling in the code will log the error message to the console. Common issues include incorrect API keys or malformed request data. Ensure your API key is correct and that the request data matches the expected format.
For more detailed information on error codes and handling, refer to the Salesflare API documentation.
Conclusion and Best Practices for Using Salesflare API in JavaScript
Integrating with the Salesflare API using JavaScript can significantly enhance your application's ability to manage contact data efficiently. By following the steps outlined in this guide, you can create or update contacts seamlessly, ensuring your sales team always has access to the most current information.
Best Practices for Secure and Efficient API Integration
- Secure Storage of API Keys: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by Salesflare's API to avoid exceeding them. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data you send to Salesflare is standardized and validated to match the expected format, reducing the risk of errors.
- Error Handling: Implement robust error handling to manage API call failures, logging errors for further analysis and troubleshooting.
Streamlining Integrations with Endgrate
While integrating with individual APIs like Salesflare can be beneficial, managing multiple integrations can become complex and time-consuming. This is where Endgrate can help.
Endgrate provides a unified API endpoint that connects to multiple platforms, including Salesflare, allowing you to manage all your integrations through a single interface. This not only saves time and resources but also simplifies the integration experience for your customers.
By leveraging Endgrate, you can focus on your core product development while outsourcing the complexities of integration management. Visit Endgrate to learn more about how it can streamline your integration processes.
Read More
Ready to get started?