How to Get Accounts with the Quickbooks API in Javascript
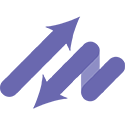
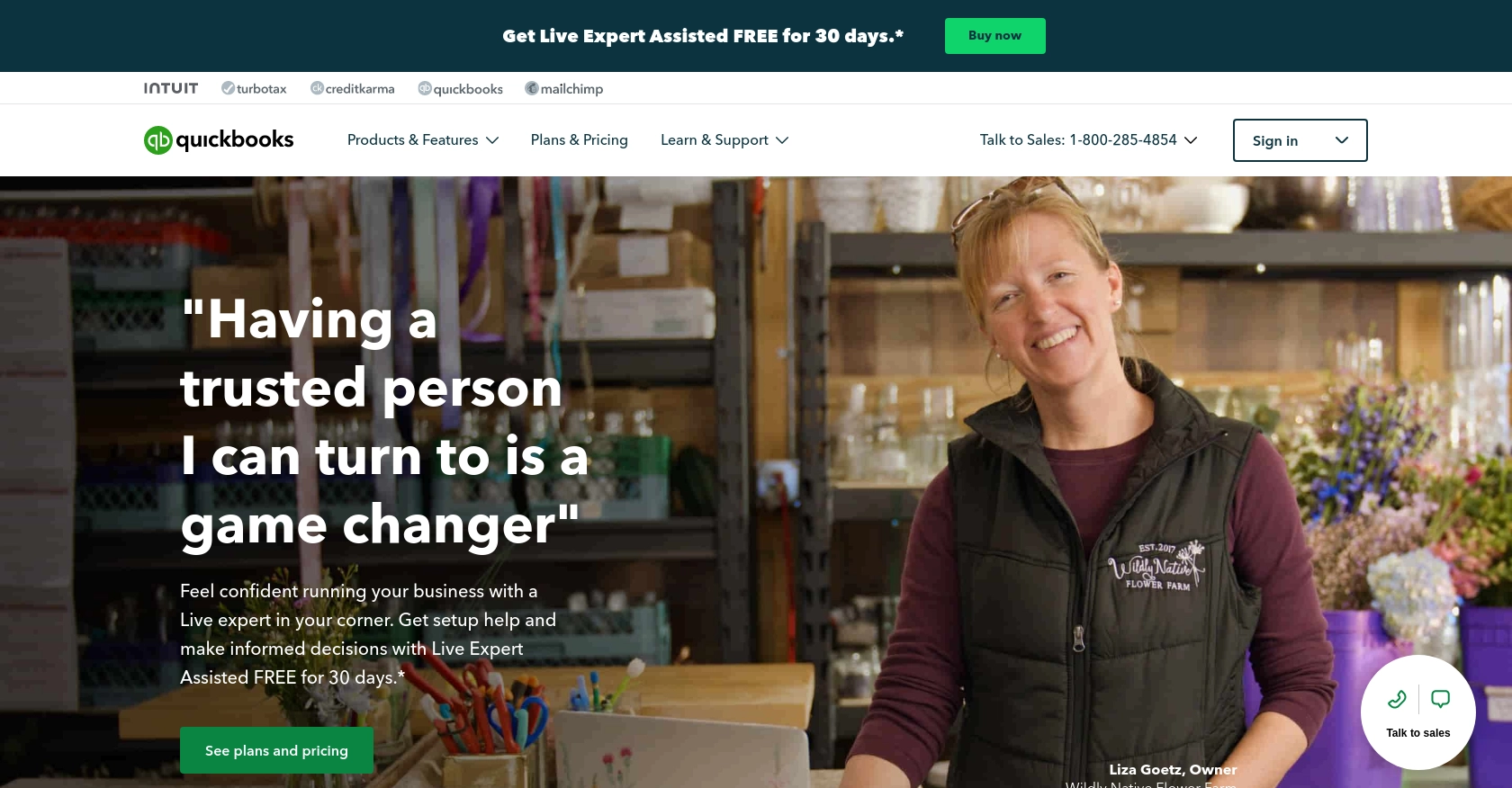
Introduction to QuickBooks API Integration
QuickBooks is a widely-used accounting software that offers a comprehensive suite of tools for managing financial data. It is particularly popular among small to medium-sized businesses for its user-friendly interface and robust features, including invoicing, expense tracking, and financial reporting.
Developers may want to integrate with QuickBooks to streamline financial operations and automate accounting tasks. For example, using the QuickBooks API, a developer can retrieve account information to generate detailed financial reports or synchronize data with other business applications.
This article will guide you through the process of using JavaScript to interact with the QuickBooks API, specifically focusing on retrieving account details. By the end of this tutorial, you'll be equipped to efficiently access and manage account data within the QuickBooks platform.
Setting Up Your QuickBooks Sandbox Account for API Integration
Before you can start interacting with the QuickBooks API using JavaScript, you'll need to set up a QuickBooks sandbox account. This sandbox environment allows developers to test API calls without affecting live data, providing a safe space to experiment and develop integrations.
Creating a QuickBooks Developer Account
To begin, you'll need to create a QuickBooks Developer account. Follow these steps:
- Visit the QuickBooks Developer Portal.
- Click on "Sign Up" to create a new account or "Sign In" if you already have one.
- Complete the registration process by providing the necessary information.
Setting Up a QuickBooks Sandbox Company
Once your developer account is ready, you can set up a sandbox company:
- Navigate to the "Dashboard" on the QuickBooks Developer Portal.
- Select "Sandbox" from the menu and click on "Add Sandbox Company."
- Follow the prompts to create a new sandbox company, which will be used for testing API interactions.
Creating a QuickBooks App for OAuth Authentication
QuickBooks API uses OAuth 2.0 for authentication. You'll need to create an app to obtain the necessary credentials:
- In the Developer Portal, go to "My Apps" and click "Create an App."
- Select "QuickBooks Online and Payments" as the platform.
- Fill in the required details, such as app name and description.
- Once the app is created, navigate to the "Keys & OAuth" section.
- Here, you'll find your Client ID and Client Secret. Keep these credentials secure as they are essential for authenticating API requests.
For more detailed instructions, refer to the QuickBooks OAuth Setup Guide.
Configuring OAuth Scopes and Redirect URIs
To ensure your app has the right permissions, configure the OAuth scopes:
- In the app settings, go to the "Scopes" section.
- Select the necessary scopes for accessing account data, such as "Accounting."
- Set up the redirect URIs that will handle the OAuth callback. This is crucial for completing the OAuth flow.
For more information, visit the QuickBooks App Settings Documentation.
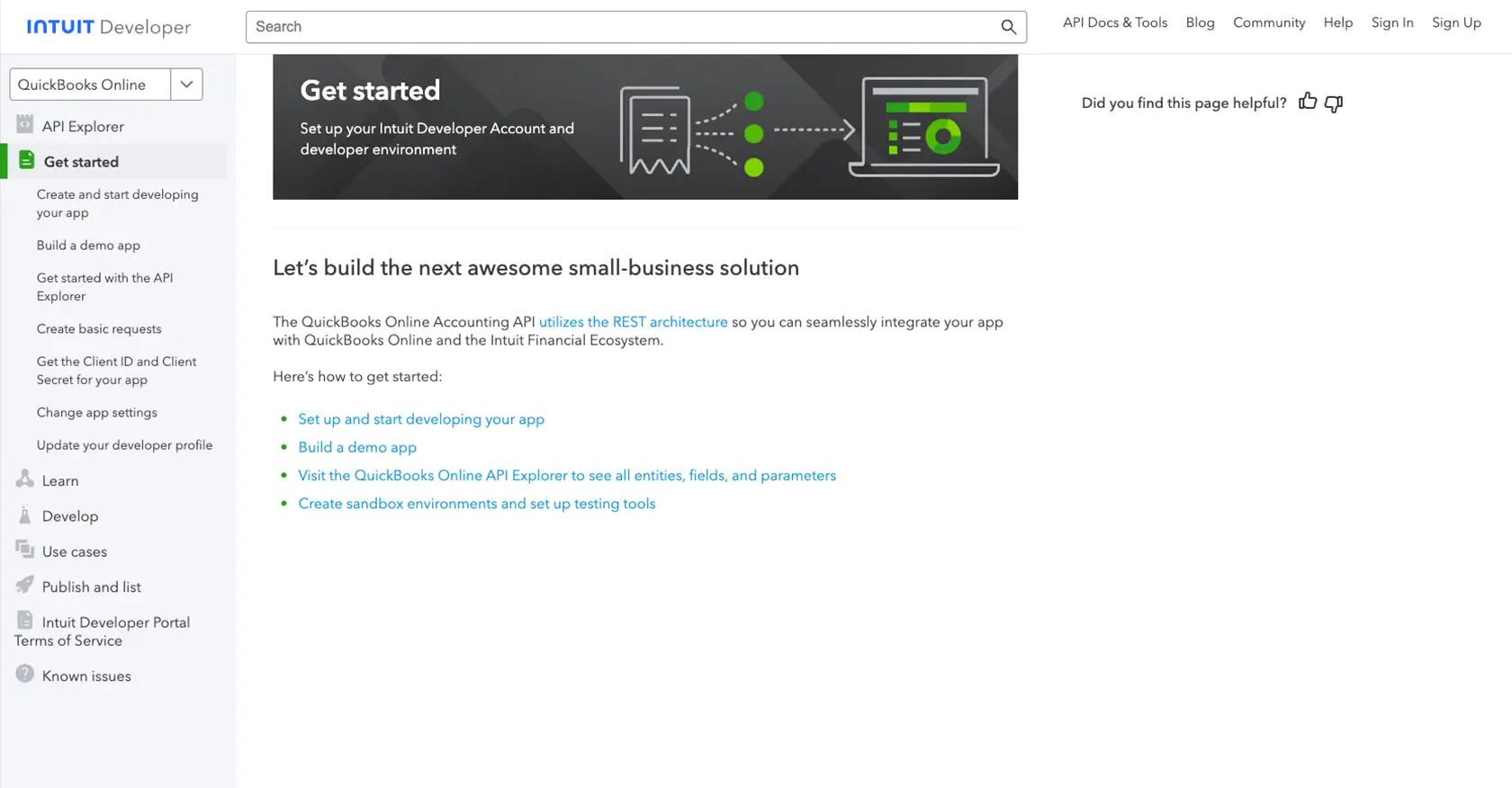
sbb-itb-96038d7
Making API Calls to Retrieve Accounts with QuickBooks API Using JavaScript
To interact with the QuickBooks API and retrieve account details, you'll need to use JavaScript to make HTTP requests. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses and errors.
Setting Up Your JavaScript Environment for QuickBooks API
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A package manager like npm (comes with Node.js) to install dependencies.
Once you have Node.js and npm set up, create a new project directory and initialize it:
mkdir quickbooks-api-integration
cd quickbooks-api-integration
npm init -y
Next, install the necessary package for making HTTP requests:
npm install axios
Writing JavaScript Code to Retrieve Accounts from QuickBooks API
Create a new file named getAccounts.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://quickbooks.api.intuit.com/v3/company/YOUR_COMPANY_ID/account';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Accept': 'application/json'
};
// Function to get accounts
async function getAccounts() {
try {
const response = await axios.get(endpoint, { headers });
const accounts = response.data.QueryResponse.Account;
console.log('Accounts:', accounts);
} catch (error) {
console.error('Error fetching accounts:', error.response ? error.response.data : error.message);
}
}
getAccounts();
Replace YOUR_COMPANY_ID
and YOUR_ACCESS_TOKEN
with your actual QuickBooks company ID and access token obtained during the OAuth authentication process.
Running the JavaScript Code and Verifying API Response
To execute the code, run the following command in your terminal:
node getAccounts.js
If successful, you should see a list of accounts printed in the console. This confirms that your API call was successful and the data retrieved matches the accounts in your QuickBooks sandbox environment.
Handling Errors and Understanding QuickBooks API Error Codes
When making API calls, it's essential to handle potential errors gracefully. The QuickBooks API may return various error codes, such as:
- 401 Unauthorized: Indicates issues with authentication, such as an expired or invalid token.
- 403 Forbidden: Indicates insufficient permissions to access the requested resource.
- 404 Not Found: Indicates the requested resource does not exist.
For more detailed information on error codes, refer to the QuickBooks API Documentation.
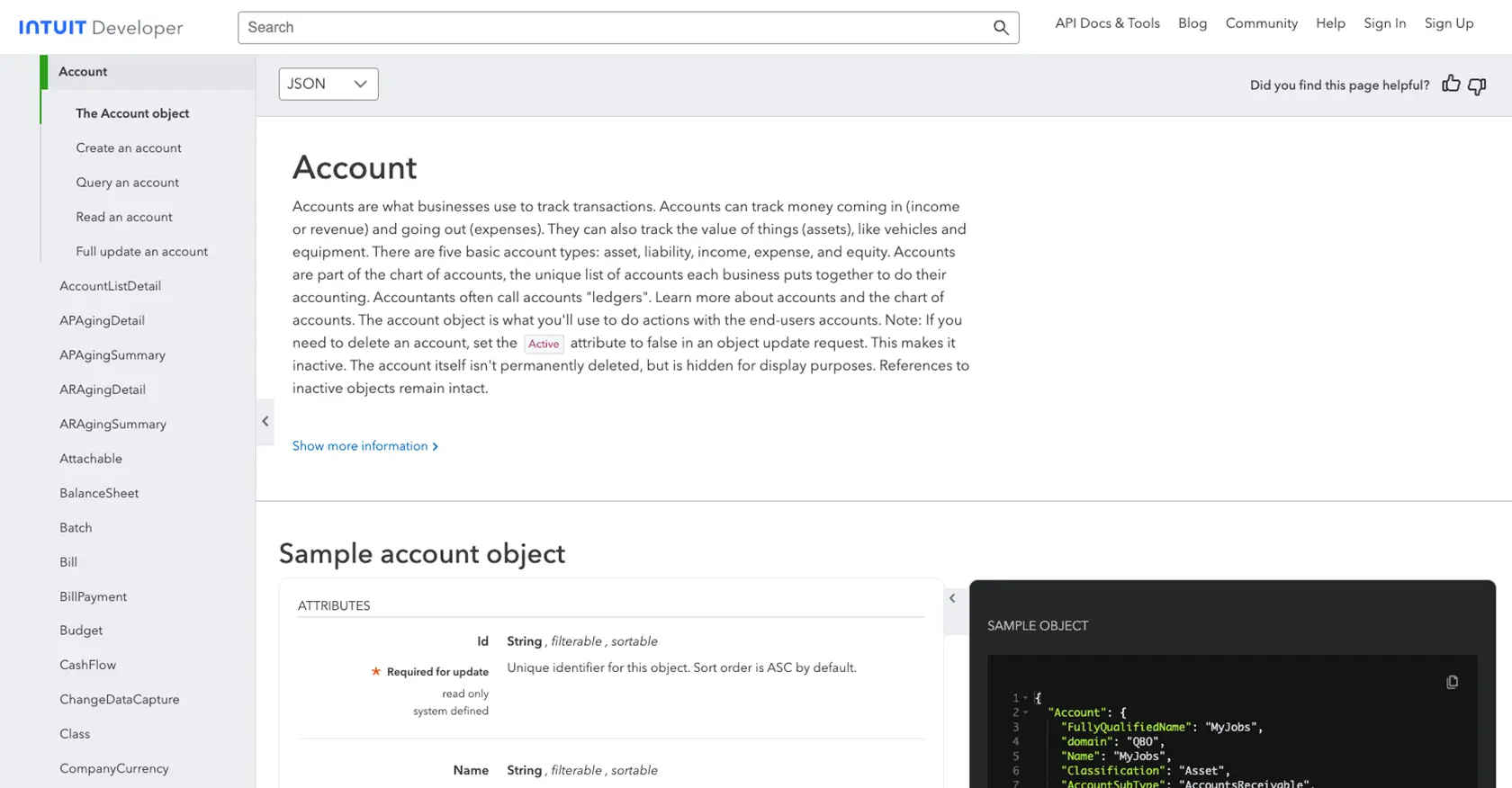
Best Practices for QuickBooks API Integration and Error Handling
When integrating with the QuickBooks API, it's crucial to follow best practices to ensure a smooth and secure experience. Here are some recommendations:
- Securely Store Credentials: Always store your OAuth credentials, such as the Client ID and Client Secret, securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: QuickBooks API has rate limits to prevent abuse. Monitor the response headers for rate limit information and implement exponential backoff strategies to handle retries gracefully.
- Standardize Data Fields: When synchronizing data between QuickBooks and other systems, ensure that data fields are standardized to maintain consistency and accuracy.
- Implement Robust Error Handling: Anticipate potential errors by implementing comprehensive error handling. Log errors for debugging and provide meaningful feedback to users when issues arise.
Enhancing Your Integration Strategy with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including QuickBooks. By leveraging Endgrate, you can:
- Save Time and Resources: Focus on your core product development while Endgrate handles the intricacies of integration.
- Build Once, Use Multiple Times: Develop a single integration for each use case and apply it across different platforms effortlessly.
- Enhance Customer Experience: Provide your customers with a seamless and intuitive integration experience.
Explore how Endgrate can simplify your integration needs by visiting Endgrate's website and discover the benefits of outsourcing your integrations.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/all-entities/account
Ready to get started?