How to Create Products with the DDI API in Javascript
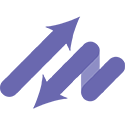
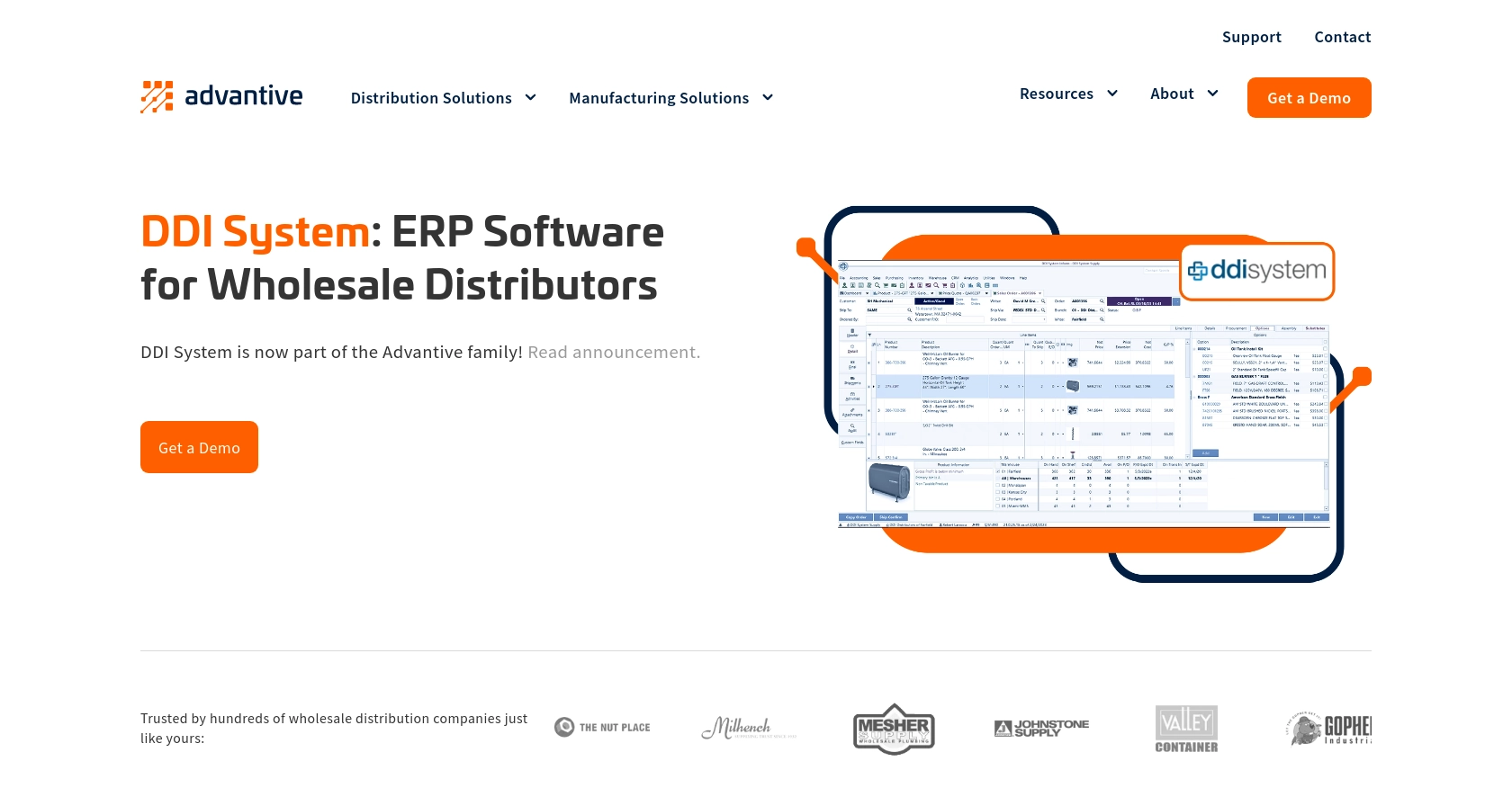
Introduction to DDI Product Integration
Advantive's DDI product offers a unique solution for businesses looking to streamline their product management processes. While it doesn't provide a publicly accessible API, it utilizes a custom FTP integration to facilitate the transfer of product data. This approach allows businesses to efficiently push product information to DDI's FTP server, ensuring seamless integration with their existing systems.
Developers might want to integrate with DDI to automate the management of product data, such as updating inventory quantities or pricing information. For example, a developer could use this integration to automatically update product details from an e-commerce platform, ensuring that the latest information is always available to customers.
Setting Up Your DDI FTP Integration Environment
Before you can start integrating with Advantive's DDI product, you'll need to set up your FTP integration environment. This involves configuring access to the DDI FTP server, where you'll push product data. Follow these steps to ensure a smooth setup process.
Step 1: Obtain FTP Server Credentials
To interact with the DDI FTP server, you'll need specific credentials. These credentials are typically provided by Advantive or your system administrator. Ensure you have the following information:
- FTP Server Address
- Username
- Password
- Port Number (if different from the default FTP port 21)
Step 2: Configure FTP Client
You'll need an FTP client to connect to the DDI FTP server. You can use any FTP client of your choice, such as FileZilla or WinSCP. Configure the client with the credentials obtained in Step 1:
- Enter the FTP Server Address.
- Provide the Username and Password.
- Specify the Port Number if necessary.
Step 3: Test the FTP Connection
Once your FTP client is configured, test the connection to ensure everything is working correctly. Connect to the FTP server and verify that you can access the directories where product data will be uploaded.
Step 4: Prepare Product Data Files
Product data must be formatted according to the schema provided by DDI. Ensure your data files include the necessary fields such as Variant SKU, Variant Price, and others as specified in the schema. Save these files in a format compatible with the FTP server, typically CSV or XML.
Step 5: Upload Test Data
Before proceeding with live data, it's crucial to test the integration by uploading sample product data to the FTP server. This will help you verify that the data is correctly formatted and that the integration process works as expected.
By following these steps, you'll be ready to start integrating with Advantive's DDI product, automating your product management processes efficiently.
Implementing Product Uploads to DDI FTP Server Using JavaScript
To automate the process of uploading product data to Advantive's DDI FTP server, you can utilize JavaScript to handle file transfers programmatically. This section will guide you through setting up the necessary environment and writing the code to push product data efficiently.
Prerequisites for JavaScript FTP Integration with DDI
Before diving into the code, ensure you have the following prerequisites in place:
- Node.js installed on your machine.
- An FTP library for Node.js, such as
ftp
orbasic-ftp
. - Access to the DDI FTP server with the credentials provided earlier.
Installing Required Node.js Packages
To interact with the FTP server using JavaScript, you need to install an FTP client library. You can use basic-ftp
for this purpose. Run the following command in your terminal to install it:
npm install basic-ftp
Writing JavaScript Code to Upload Product Data to DDI FTP
With the necessary packages installed, you can now write the JavaScript code to upload product data files to the DDI FTP server. Below is an example script:
const ftp = require("basic-ftp");
async function uploadProductData() {
const client = new ftp.Client();
client.ftp.verbose = true;
try {
await client.access({
host: "your-ftp-server-address",
user: "your-username",
password: "your-password",
secure: false
});
console.log("Connected to DDI FTP server");
// Upload the product data file
await client.uploadFrom("path/to/your/product-data.csv", "remote/path/product-data.csv");
console.log("Product data uploaded successfully");
} catch (err) {
console.error("Error uploading product data:", err);
}
client.close();
}
uploadProductData();
Replace your-ftp-server-address
, your-username
, your-password
, and the file paths with your actual FTP server details and file locations.
Verifying Successful Product Data Upload to DDI FTP
After running the script, verify that the product data file has been successfully uploaded to the DDI FTP server. You can do this by checking the remote directory using your FTP client to ensure the file appears as expected.
Handling Errors and Troubleshooting FTP Uploads
While uploading files, you might encounter errors such as connection issues or incorrect file paths. Ensure that:
- Your FTP server credentials are correct.
- The file paths specified in the script are accurate.
- The FTP server is accessible and not experiencing downtime.
By following these steps and using the provided JavaScript code, you can automate the process of uploading product data to Advantive's DDI FTP server, streamlining your product management workflow.
Best Practices for DDI FTP Integration and Product Data Management
Successfully integrating with Advantive's DDI product through FTP requires careful attention to best practices in both data management and integration processes. Here are some key recommendations to ensure a smooth and efficient workflow:
- Securely Store FTP Credentials: Always store your FTP credentials securely, using environment variables or encrypted storage solutions to prevent unauthorized access.
- Validate Product Data: Before uploading, validate your product data against the DDI schema to ensure all required fields are present and correctly formatted.
- Monitor FTP Transfers: Implement logging and monitoring for your FTP transfers to quickly identify and resolve any issues that may arise during data uploads.
- Implement Error Handling: Use robust error handling in your JavaScript code to manage connection failures, incorrect file paths, and other potential issues.
- Automate Regular Updates: Schedule regular updates to your product data to keep information current and reduce manual intervention.
Leveraging Endgrate for Seamless Integration Solutions
While integrating with DDI via FTP can be effective, managing multiple integrations across different platforms can become complex and time-consuming. This is where Endgrate can make a significant difference.
Endgrate offers a unified API endpoint that simplifies the integration process by connecting to multiple platforms and services, including DDI. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product development while Endgrate handles the intricacies of integration.
- Streamline Integration Management: Build once for each use case and avoid redundant efforts across different platforms.
- Enhance Customer Experience: Provide an intuitive and seamless integration experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate and discover the benefits of a simplified, efficient integration process.
Read More
Ready to get started?