Using the Sage Accounting API to Get Sales Invoices (with Javascript examples)
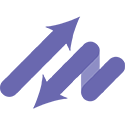
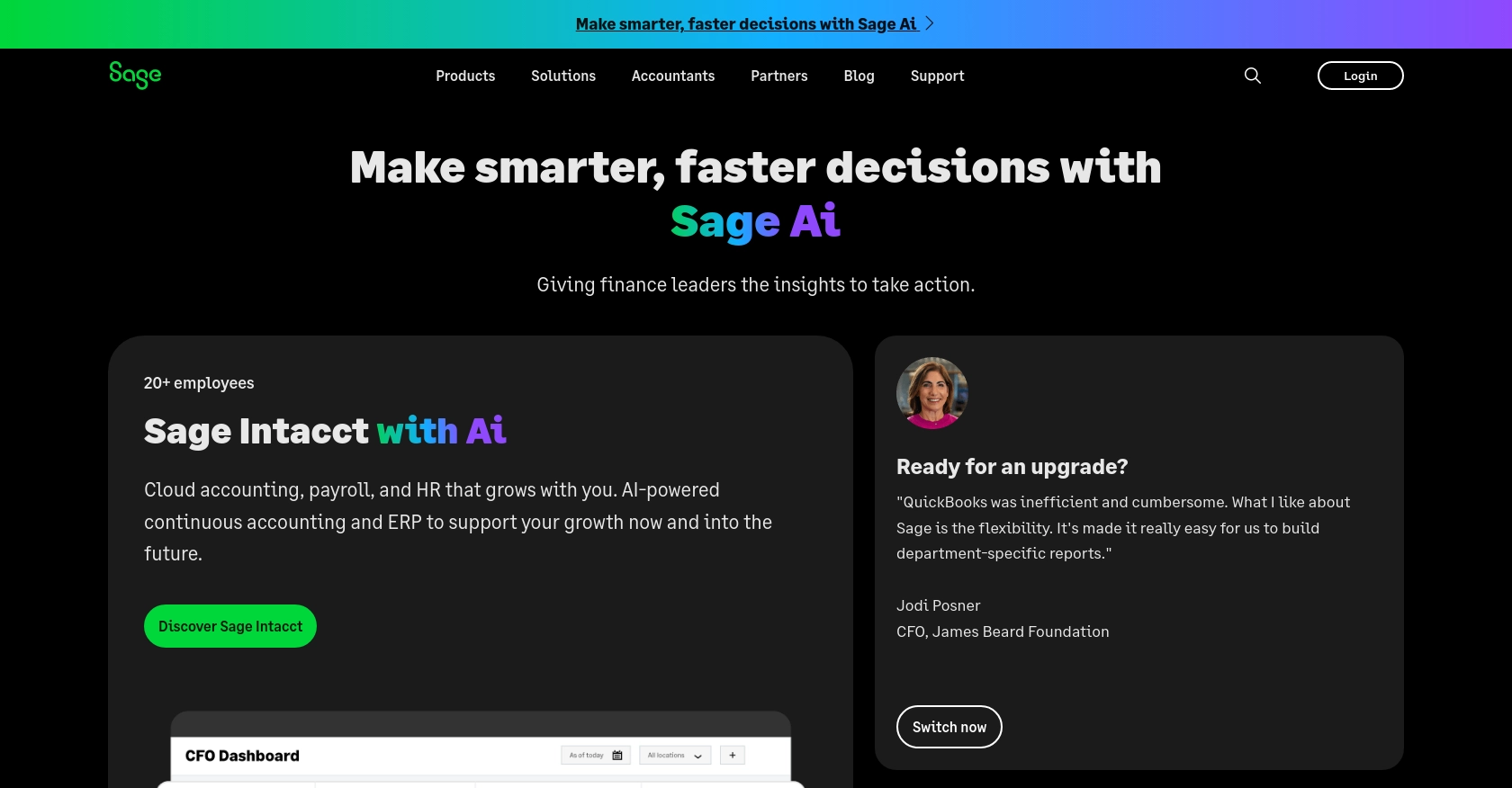
Introduction to Sage Accounting API
Sage Accounting is a robust cloud-based accounting solution tailored for small to medium-sized businesses. It offers a comprehensive suite of tools to manage financial transactions, invoicing, and reporting, helping businesses streamline their accounting processes.
Integrating with the Sage Accounting API allows developers to automate and enhance financial operations. For example, by accessing sales invoices through the API, developers can create custom dashboards or automate data entry into other systems, improving efficiency and accuracy.
Setting Up a Sage Accounting Test/Sandbox Account
Before you can start interacting with the Sage Accounting API, it's essential to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting any live data.
Create a Sage Developer Account
To begin, you'll need a Sage Developer account. This account will enable you to register and manage your applications, as well as obtain necessary credentials like the client ID and client secret.
- Visit the Sage Developer Portal.
- Sign up using your GitHub account or an email address.
- Follow the guide on creating an app to obtain your client credentials.
Register a Trial Business Account
Next, you'll need to set up a trial business account for development purposes. Sage offers trial accounts for various regions and subscription tiers.
- Choose the appropriate region and subscription tier from the Sage Developer Portal.
- Sign up for a trial account using your preferred email service.
Create a Sage App for OAuth Authentication
Since the Sage Accounting API uses OAuth for authentication, you'll need to create an app to generate the necessary credentials.
- Log in to your Sage Developer account.
- Navigate to the "Create App" section.
- Enter a name and callback URL for your app. You can find more details on setting up the callback URL in the Sage guide.
- Save your app to receive the client ID and client secret.
Upgrade to a Developer Account
To fully utilize the API, upgrade your trial account to a developer account. This provides 12 months of free access for testing.
- Submit your upgrade request through the Sage Developer Portal.
- Provide your trial account details, including the app name and client ID.
- Wait for confirmation from Sage, which typically takes 3-5 working days.
Once your developer account is set up, you'll be ready to start making API calls to access sales invoices and other financial data through the Sage Accounting API.
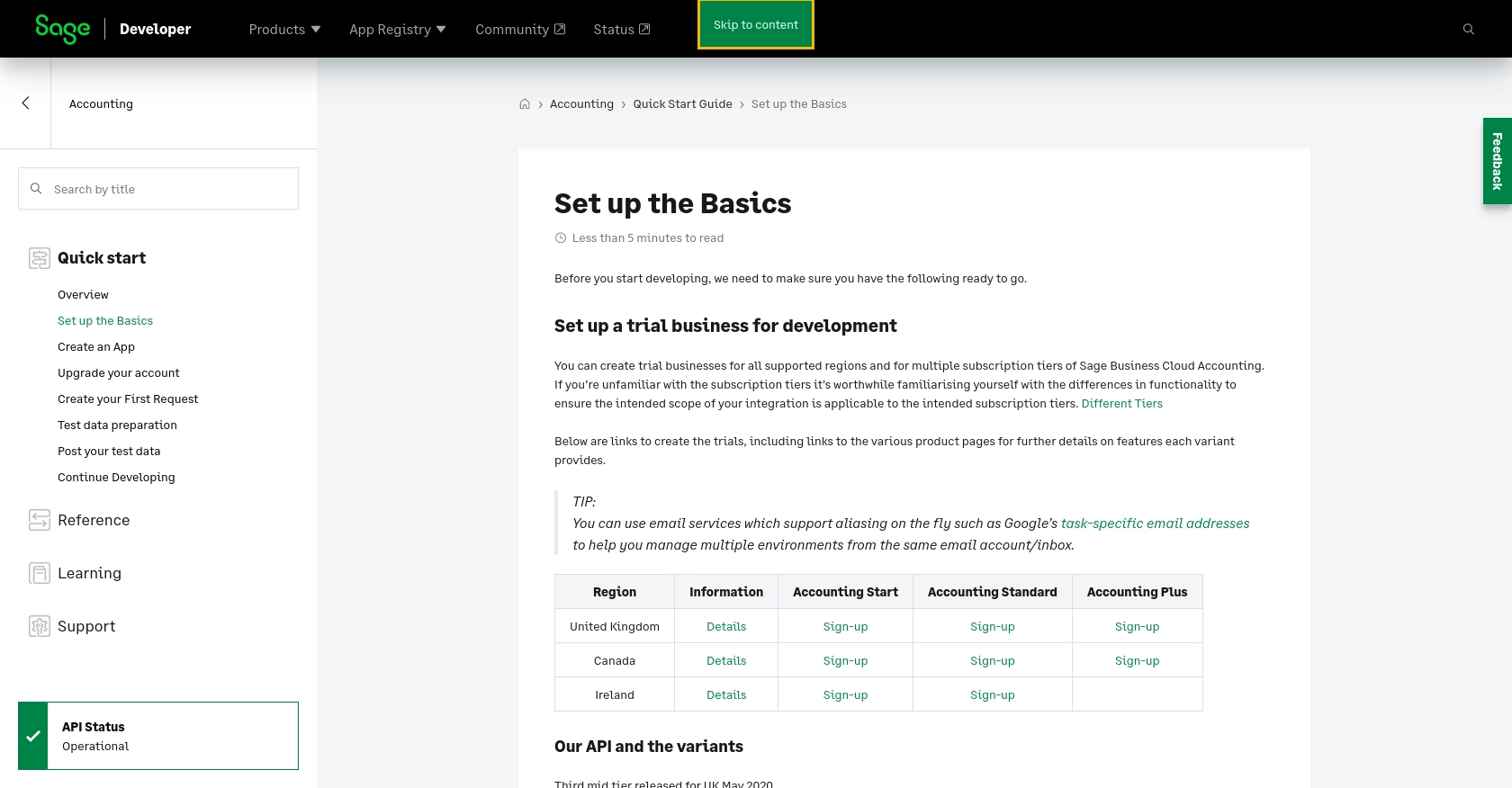
sbb-itb-96038d7
Making API Calls to Retrieve Sales Invoices Using Sage Accounting API with JavaScript
Once your Sage Developer account is set up and your app is created, you can begin making API calls to retrieve sales invoices. This section will guide you through the process using JavaScript, ensuring you have the necessary tools and knowledge to interact with the Sage Accounting API effectively.
Prerequisites for Using JavaScript with Sage Accounting API
Before diving into the code, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A code editor such as Visual Studio Code.
- Knowledge of JavaScript and basic understanding of HTTP requests.
Installing Required Dependencies
To make HTTP requests in JavaScript, you can use the Axios library. Install it using npm:
npm install axios
Example Code to Retrieve Sales Invoices
Below is an example of how to retrieve sales invoices using the Sage Accounting API with JavaScript:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.sage.com/accounting/v3.1/sales_invoices';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Function to get sales invoices
async function getSalesInvoices() {
try {
const response = await axios.get(endpoint, { headers });
const invoices = response.data;
console.log('Sales Invoices:', invoices);
} catch (error) {
console.error('Error fetching sales invoices:', error.response ? error.response.data : error.message);
}
}
// Call the function
getSalesInvoices();
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth authentication process.
Verifying API Call Success
After running the code, you should see the sales invoices data printed in the console. To verify the request's success, check the response status code. A status code of 200 indicates a successful request.
Handling Errors and Common Error Codes
When making API calls, it's crucial to handle potential errors. Common error codes include:
- 401 Unauthorized: Check if your access token is valid and not expired.
- 403 Forbidden: Ensure your app has the necessary permissions to access sales invoices.
- 404 Not Found: Verify the endpoint URL is correct.
Refer to the Sage Accounting API documentation for more details on error handling.
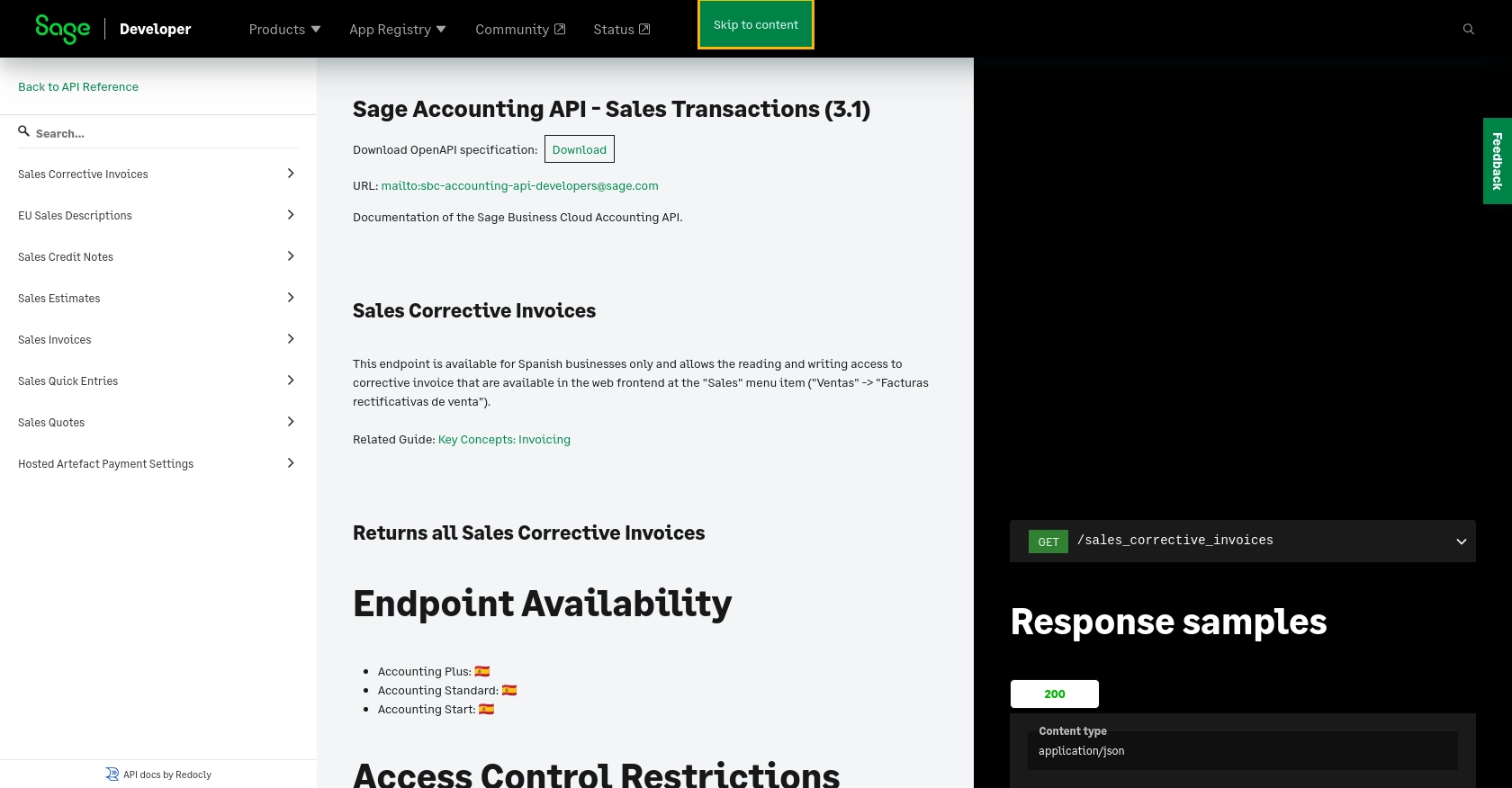
Conclusion and Best Practices for Using Sage Accounting API with JavaScript
Integrating with the Sage Accounting API using JavaScript provides a powerful way to automate and streamline financial operations. By following the steps outlined in this guide, you can efficiently retrieve sales invoices and enhance your business processes.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of API rate limits to avoid service disruptions. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure consistency in data formats when integrating with other systems. This helps in maintaining data integrity and simplifies data processing.
Leverage Endgrate for Simplified Integration Management
While building integrations with Sage Accounting API can be rewarding, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API endpoint that connects to various platforms, including Sage Accounting, allowing you to focus on your core product development.
By using Endgrate, you can:
- Save time and resources by outsourcing integration management.
- Build once for each use case instead of multiple times for different integrations.
- Provide an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration processes by visiting Endgrate today.
Read More
- https://endgrate.com/provider/sage
- https://developer.sage.com/accounting/quick-start/set-up-the-basics/
- https://developer.sage.com/accounting/quick-start/create-an-app/
- https://developer.sage.com/accounting/quick-start/upgrade-your-account/
- https://developer.sage.com/accounting/reference/invoicing-sales/
Ready to get started?