Using the Brevo API to Get Users in Javascript
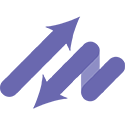
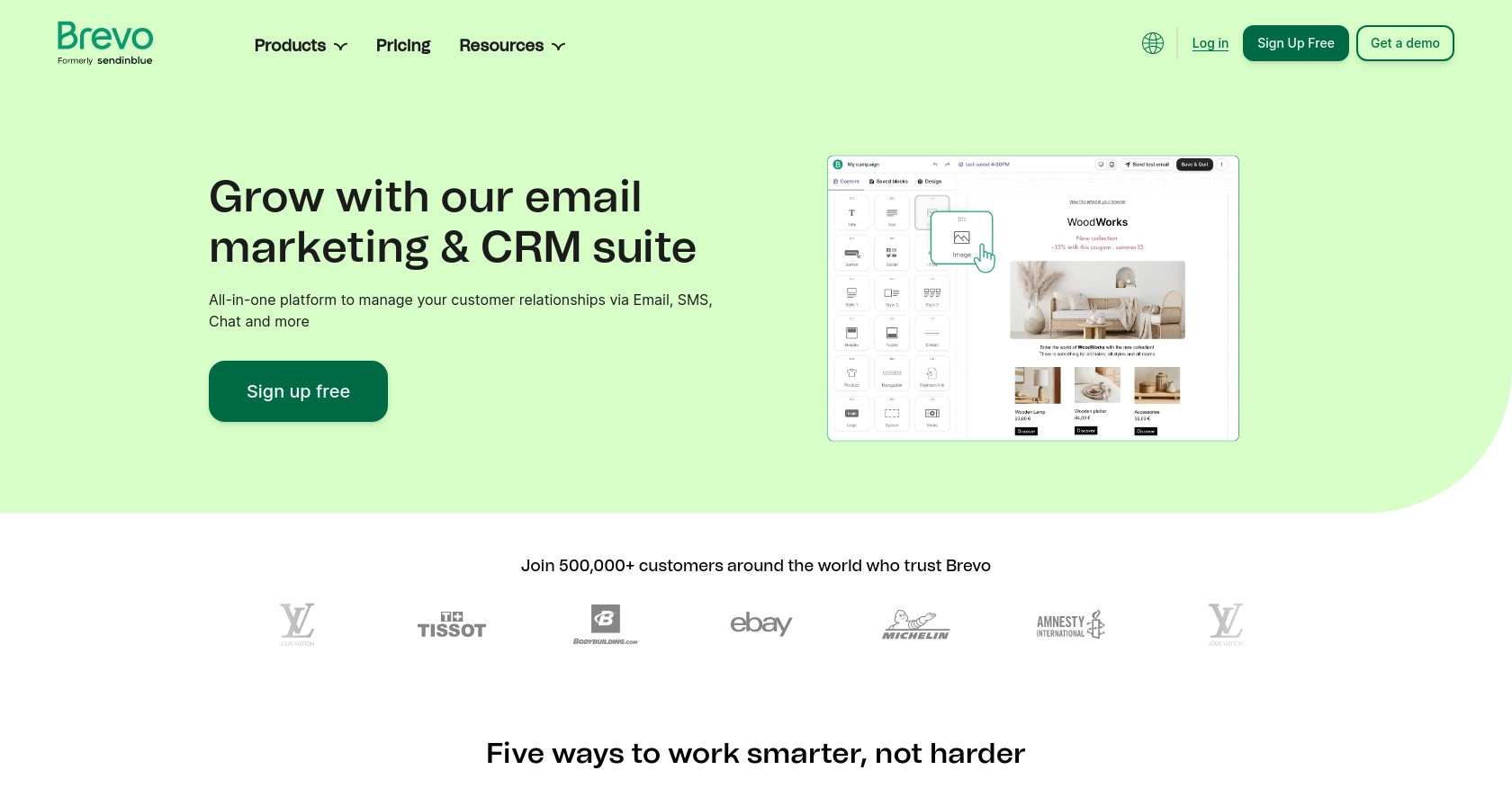
Introduction to Brevo API Integration
Brevo is a versatile platform that offers a range of communication tools, including email, SMS, and WhatsApp campaigns, designed to enhance customer engagement for businesses. Its robust API allows developers to seamlessly integrate these communication features into their applications, providing a unified experience for managing customer interactions.
Connecting with the Brevo API can empower developers to automate user management tasks, such as retrieving a list of users within an organization. For example, a developer might use the Brevo API to fetch user data and integrate it into a custom dashboard, enabling real-time insights and streamlined user management.
Setting Up Your Brevo Test Account for API Integration
Before you can start using the Brevo API to manage users, you'll need to set up a Brevo account and obtain an API key. This key will allow you to authenticate your requests and interact with Brevo's services programmatically.
Creating a Brevo Account
If you don't already have a Brevo account, you can sign up for free by visiting the Brevo signup page. Follow the instructions to create your account and verify your email address.
Generating Your Brevo API Key
Once your account is set up, you'll need to generate an API key to authenticate your requests:
- Log in to your Brevo account.
- Click on your profile name at the top-right corner of the screen and select SMTP & API from the dropdown menu.
- Navigate to the API keys tab.
- Click Generate a new API key.
- Provide a name for your API key to help you identify it later.
- Click Generate and copy the API key. Store it securely as you'll need it for making API calls.
This API key will be used to authenticate your requests and ensure that they are associated with your Brevo account.
Understanding Brevo API Key Authentication
Brevo uses API key-based authentication to verify the identity of the requester. Each API call you make must include this key in the request headers to ensure successful authentication. This process is crucial for maintaining the security and integrity of your interactions with Brevo's services.
With your Brevo account and API key ready, you can now proceed to make API calls and integrate Brevo's powerful communication tools into your applications.
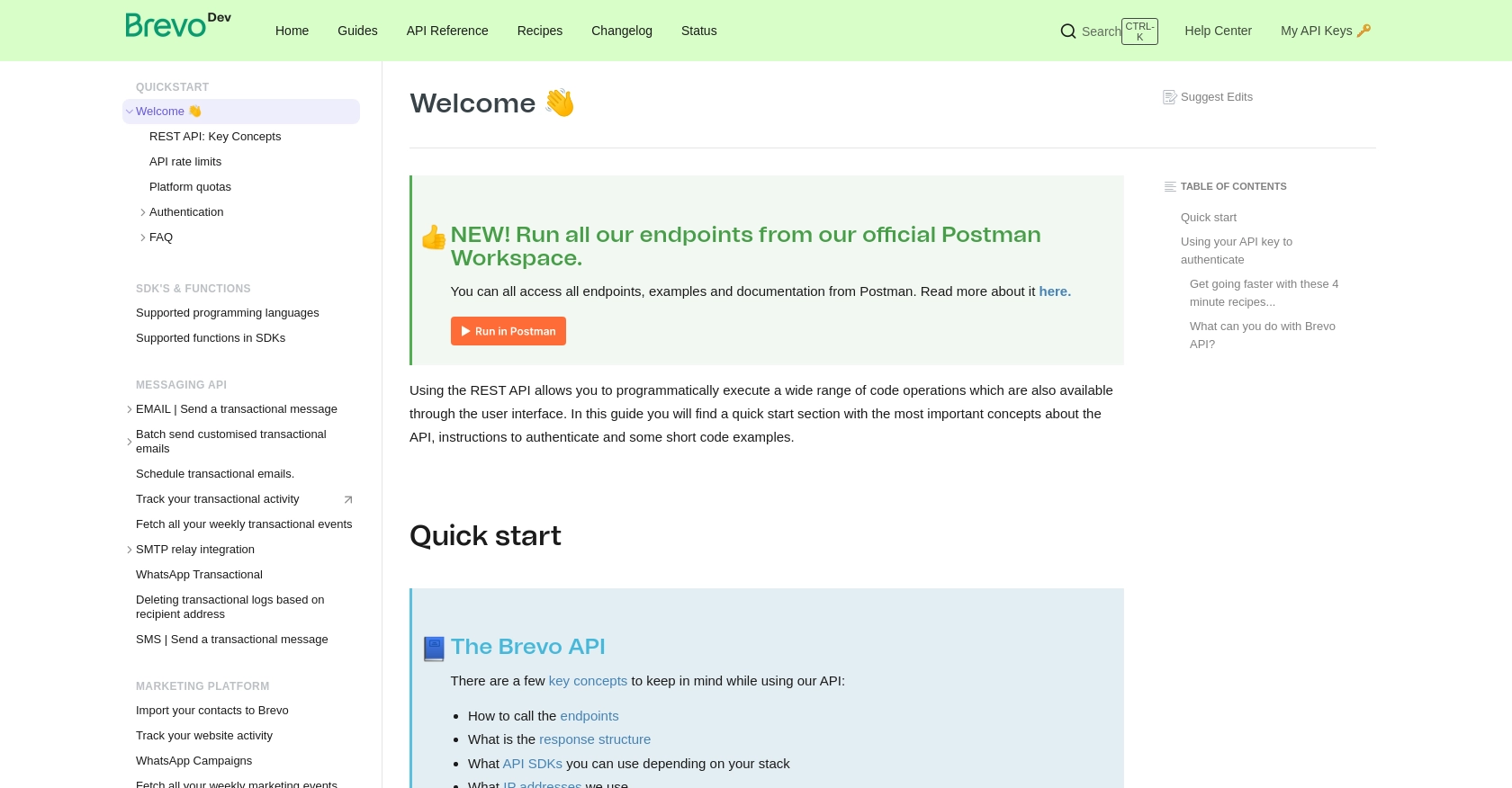
sbb-itb-96038d7
Making API Calls to Retrieve Users with Brevo API in JavaScript
To interact with the Brevo API and retrieve a list of users, you'll need to use JavaScript to make HTTP requests. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for Brevo API
Before you begin, ensure you have Node.js installed on your machine. Node.js provides the runtime environment for executing JavaScript code outside of a browser. You can download it from the official Node.js website.
Additionally, you'll need to install the axios
library, which simplifies making HTTP requests. Run the following command in your terminal to install it:
npm install axios
Writing JavaScript Code to Fetch Users from Brevo API
With your environment set up, you can now write the JavaScript code to make an API call to Brevo and retrieve the list of users. Create a new file named getBrevoUsers.js
and add the following code:
const axios = require('axios');
// Your Brevo API key
const apiKey = 'Your_API_Key';
// Set the API endpoint and headers
const endpoint = 'https://api.brevo.com/v3/organization/invited/users';
const headers = {
'accept': 'application/json',
'api-key': apiKey
};
// Function to get users
async function getUsers() {
try {
const response = await axios.get(endpoint, { headers });
const users = response.data.users;
console.log('List of Users:', users);
} catch (error) {
console.error('Error fetching users:', error.response ? error.response.data : error.message);
}
}
// Call the function
getUsers();
Replace Your_API_Key
with the API key you generated earlier. This code sets up the API endpoint and headers, makes a GET request to retrieve users, and handles any errors that may occur.
Running the JavaScript Code and Verifying Output
To execute the code, run the following command in your terminal:
node getBrevoUsers.js
If successful, you should see a list of users printed in the console. This output confirms that the API call was successful and that you have retrieved the user data from Brevo.
Handling Errors and Understanding Brevo API Response Codes
When making API calls, it's essential to handle potential errors gracefully. The Brevo API may return various error codes, such as:
- 400 Bad Request: Indicates a malformed request. Check your request parameters.
- 401 Unauthorized: Indicates an authentication error. Verify your API key.
- 404 Not Found: Indicates the requested resource does not exist.
Refer to the Brevo API documentation for more details on error codes and their meanings.
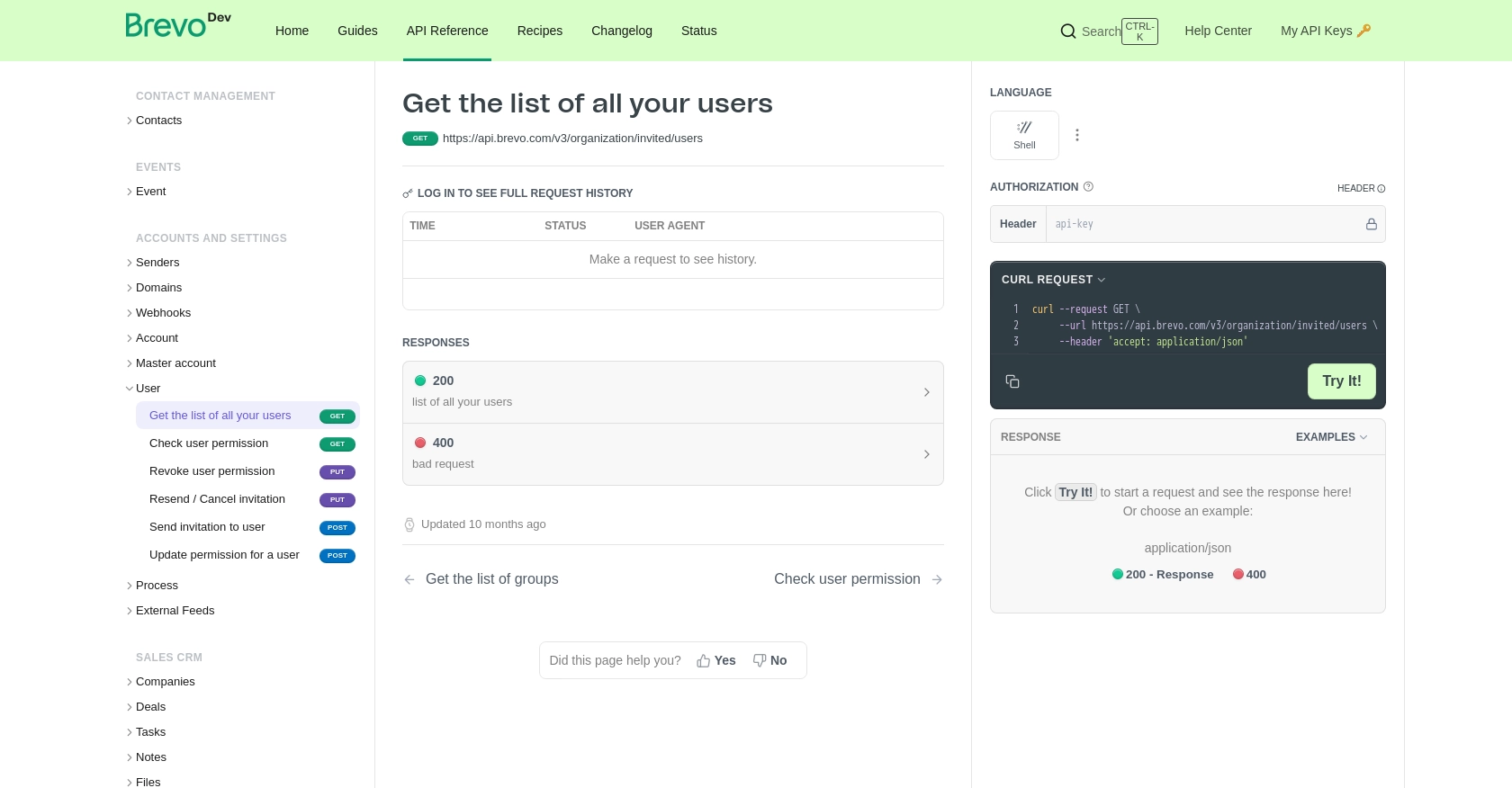
Conclusion and Best Practices for Using Brevo API in JavaScript
Integrating the Brevo API into your JavaScript applications allows you to efficiently manage user data and enhance communication capabilities. By following the steps outlined in this guide, you can seamlessly retrieve user information and incorporate it into your systems.
Best Practices for Secure and Efficient Brevo API Integration
- Secure API Key Storage: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of Brevo's API rate limits to avoid service interruptions. Implement retry logic with exponential backoff to manage rate limit errors gracefully.
- Data Transformation: Consider transforming and standardizing data fields to ensure consistency across different systems and applications.
Enhancing Integration Capabilities with Endgrate
While integrating with Brevo is straightforward, managing multiple integrations can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Brevo. This allows you to focus on your core product while outsourcing integration complexities.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website. Save time and resources by building once for each use case and offering an intuitive integration experience for your customers.
Read More
Ready to get started?