How to Create or Update People with the Capsule API in PHP
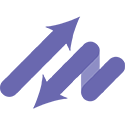
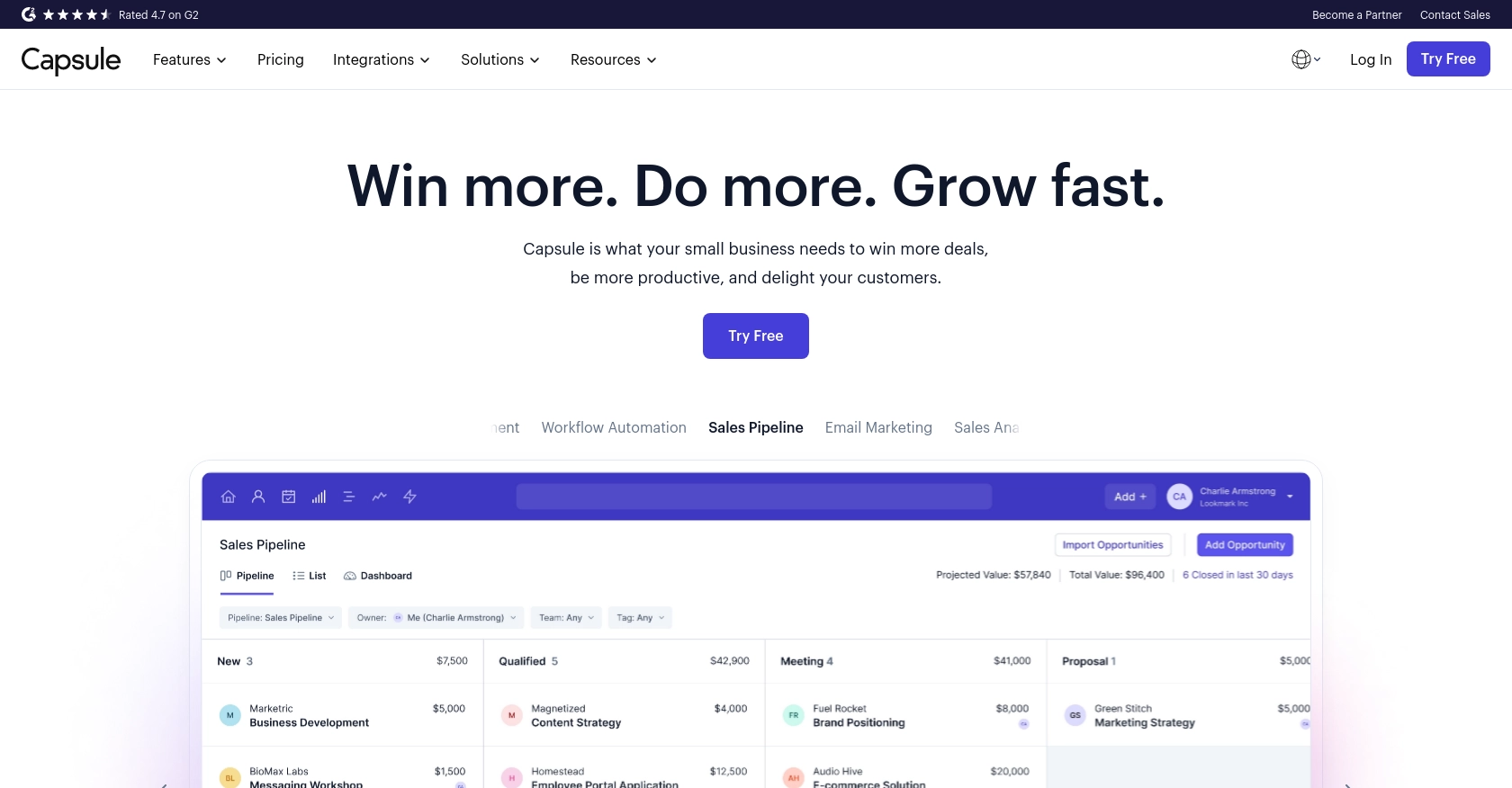
Introduction to Capsule CRM
Capsule CRM is a powerful customer relationship management platform that helps businesses manage their contacts, sales opportunities, and customer interactions efficiently. With its user-friendly interface and robust features, Capsule CRM is a popular choice for businesses looking to streamline their CRM processes.
Developers may want to integrate with Capsule CRM to automate the management of customer data, such as creating or updating contact information. For example, a developer could use the Capsule API to automatically update customer details from an external source, ensuring that the CRM data remains accurate and up-to-date.
Setting Up Your Capsule CRM Test or Sandbox Account
Before you can start integrating with Capsule CRM, you'll need to set up a test or sandbox account. This allows you to safely experiment with the Capsule API without affecting live data. Capsule CRM offers a straightforward process to get started with their API using OAuth 2.0 for authentication.
Creating a Capsule CRM Account
If you don't already have a Capsule CRM account, you can sign up for a free trial on their website. This will give you access to all the features you need to test the API integration.
- Visit the Capsule CRM signup page.
- Follow the instructions to create your account.
- Once your account is set up, log in to access the dashboard.
Registering a Capsule CRM Application for OAuth Authentication
To interact with the Capsule API, you'll need to register your application and obtain the necessary credentials for OAuth 2.0 authentication.
- Navigate to the My Preferences section in your Capsule account.
- Select API Authentication Tokens to create a new token for testing purposes.
- For OAuth 2.0, register your application by providing details such as the application name, redirect URI, and scopes required (e.g., read, write).
- Once registered, you'll receive a client ID and client secret. Keep these credentials secure as they are essential for authenticating API requests.
Generating a Bearer Token for Capsule API Access
With your application registered, you can now generate a bearer token to authenticate your API requests.
- Direct users to the Capsule authorization URL to grant access to your application:
- After authorization, Capsule will redirect to your specified URI with an authorization code.
- Exchange this code for an access token by making a POST request to the token exchange URL:
- Store the returned access token securely, as it will be used in the Authorization header of your API requests.
GET https://api.capsulecrm.com/oauth/authorise?response_type=code&client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&scope=read%20write
POST https://api.capsulecrm.com/oauth/token
{
"code": "AUTHORIZATION_CODE",
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET",
"grant_type": "authorization_code"
}
With your Capsule CRM account and OAuth setup complete, you're ready to start making API calls to create or update people in Capsule using PHP.
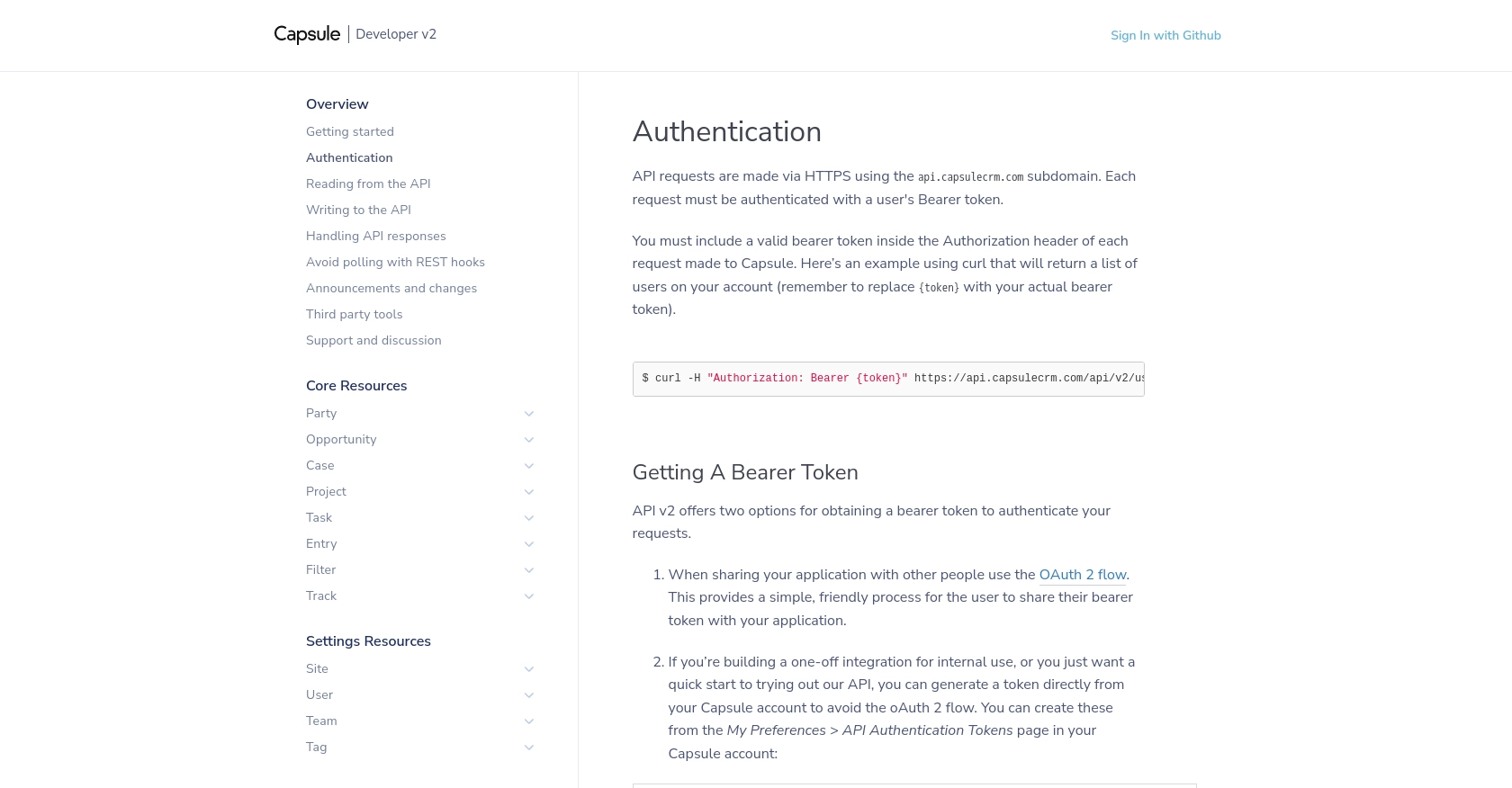
sbb-itb-96038d7
Making API Calls to Create or Update People with Capsule API in PHP
In this section, we'll guide you through the process of making API calls to create or update people in Capsule CRM using PHP. This involves setting up your PHP environment, writing the necessary code, and handling responses effectively.
Setting Up Your PHP Environment for Capsule API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need:
- PHP 7.4 or higher
- cURL extension enabled
- Composer for managing dependencies
Install the Guzzle HTTP client, which simplifies making HTTP requests in PHP:
composer require guzzlehttp/guzzle
Writing PHP Code to Create or Update People in Capsule CRM
Now, let's write the PHP code to interact with the Capsule API. We'll demonstrate how to create a new person in Capsule CRM.
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'YOUR_ACCESS_TOKEN';
$response = $client->post('https://api.capsulecrm.com/api/v2/parties', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json'
],
'json' => [
'party' => [
'type' => 'person',
'firstName' => 'John',
'lastName' => 'Doe',
'emailAddresses' => [
['type' => 'Work', 'address' => 'john.doe@example.com']
]
]
]
]);
$data = json_decode($response->getBody(), true);
echo 'Person created with ID: ' . $data['party']['id'];
Replace YOUR_ACCESS_TOKEN
with the token obtained during the OAuth setup. This code sends a POST request to the Capsule API to create a new person with the specified details.
Handling API Responses and Errors
After making the API call, it's crucial to handle responses and potential errors. Capsule API returns HTTP status codes to indicate success or failure:
- 201 Created: The person was successfully created.
- 400 Bad Request: The request was malformed.
- 401 Unauthorized: Invalid or expired access token.
- 403 Forbidden: Insufficient permissions.
Check the response status code and handle errors accordingly:
if ($response->getStatusCode() === 201) {
echo 'Person created successfully.';
} else {
echo 'Error: ' . $response->getStatusCode();
}
Verifying API Requests in Capsule CRM
To verify that your API requests are successful, log in to your Capsule CRM account and check the list of people. The newly created or updated person should appear there.
By following these steps, you can efficiently create or update people in Capsule CRM using PHP, ensuring your CRM data is accurate and up-to-date.
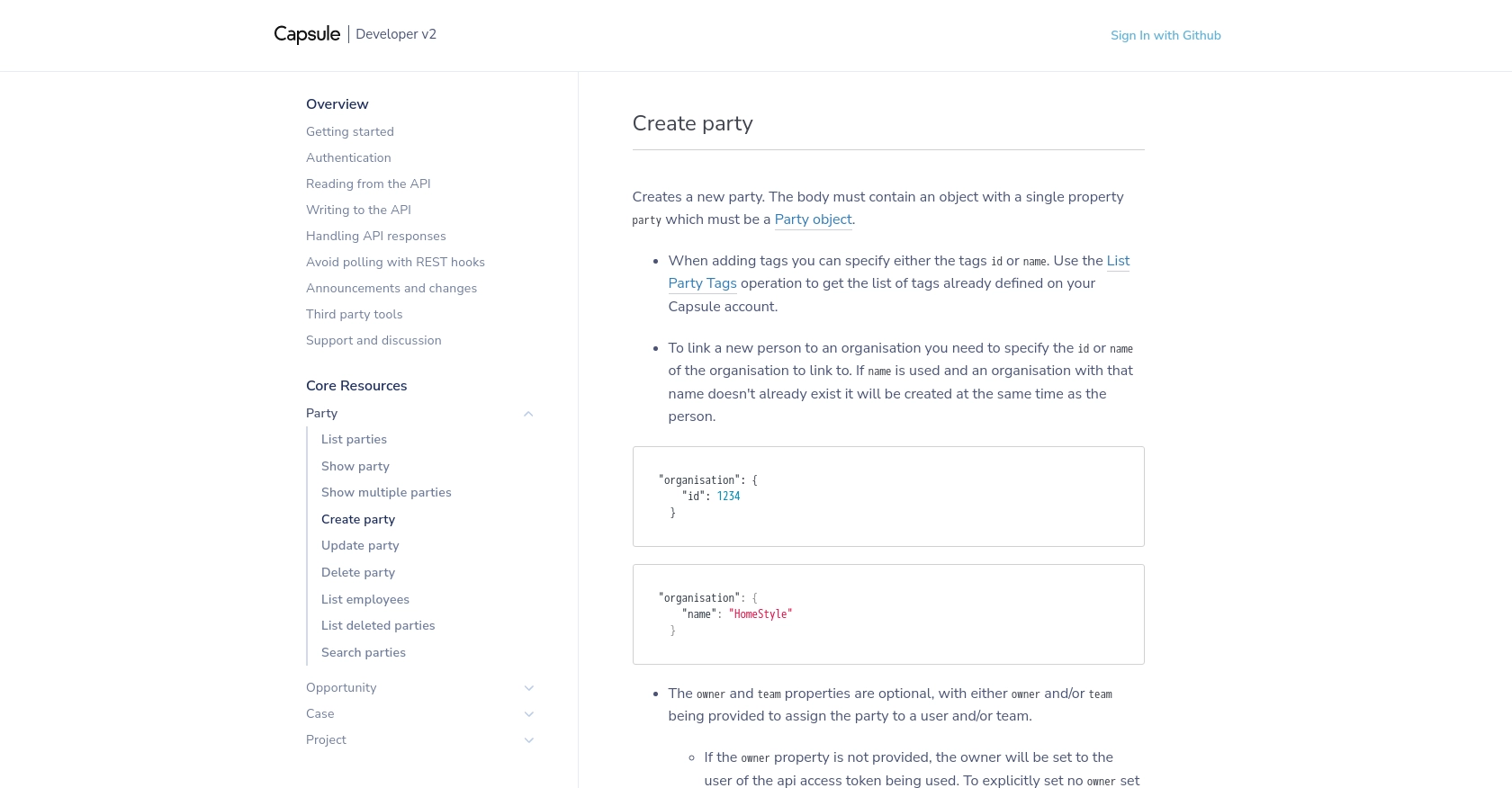
Best Practices for Capsule API Integration in PHP
When integrating with the Capsule API using PHP, it's essential to follow best practices to ensure secure and efficient interactions. Here are some recommendations:
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Capsule API allows up to 4,000 requests per hour. Monitor the
X-RateLimit-Remaining
header in API responses and implement backoff strategies to avoid hitting the limit. Consider caching responses to reduce unnecessary requests. - Implement Error Handling: Check API responses for error codes and handle them appropriately. Use the error messages provided by Capsule to debug and resolve issues efficiently.
- Data Transformation and Standardization: Ensure that data sent to and received from Capsule is correctly formatted and standardized. This helps maintain data integrity and consistency across your systems.
Streamlining Your Integration Process with Endgrate
While integrating with Capsule API directly is a powerful approach, managing multiple integrations can become complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including Capsule CRM.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product development while Endgrate handles the intricacies of multiple integrations.
- Build Once, Use Everywhere: Develop integration logic once and apply it across different platforms, reducing redundancy and effort.
- Enhance Customer Experience: Provide a seamless and intuitive integration experience for your users, improving satisfaction and engagement.
Explore how Endgrate can simplify your integration needs by visiting Endgrate's website and discover the benefits of a streamlined integration process.
Read More
- https://endgrate.com/provider/capsulecrm
- https://developer.capsulecrm.com/v2/overview/authentication
- https://developer.capsulecrm.com/v2/overview/handling-api-responses
- https://developer.capsulecrm.com/v2/overview/avoid-polling-with-rest-hooks
- https://developer.capsulecrm.com/v2/operations/Party#createParty
Ready to get started?