Using the Capsule API to Get Users (with PHP examples)
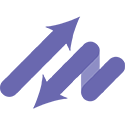
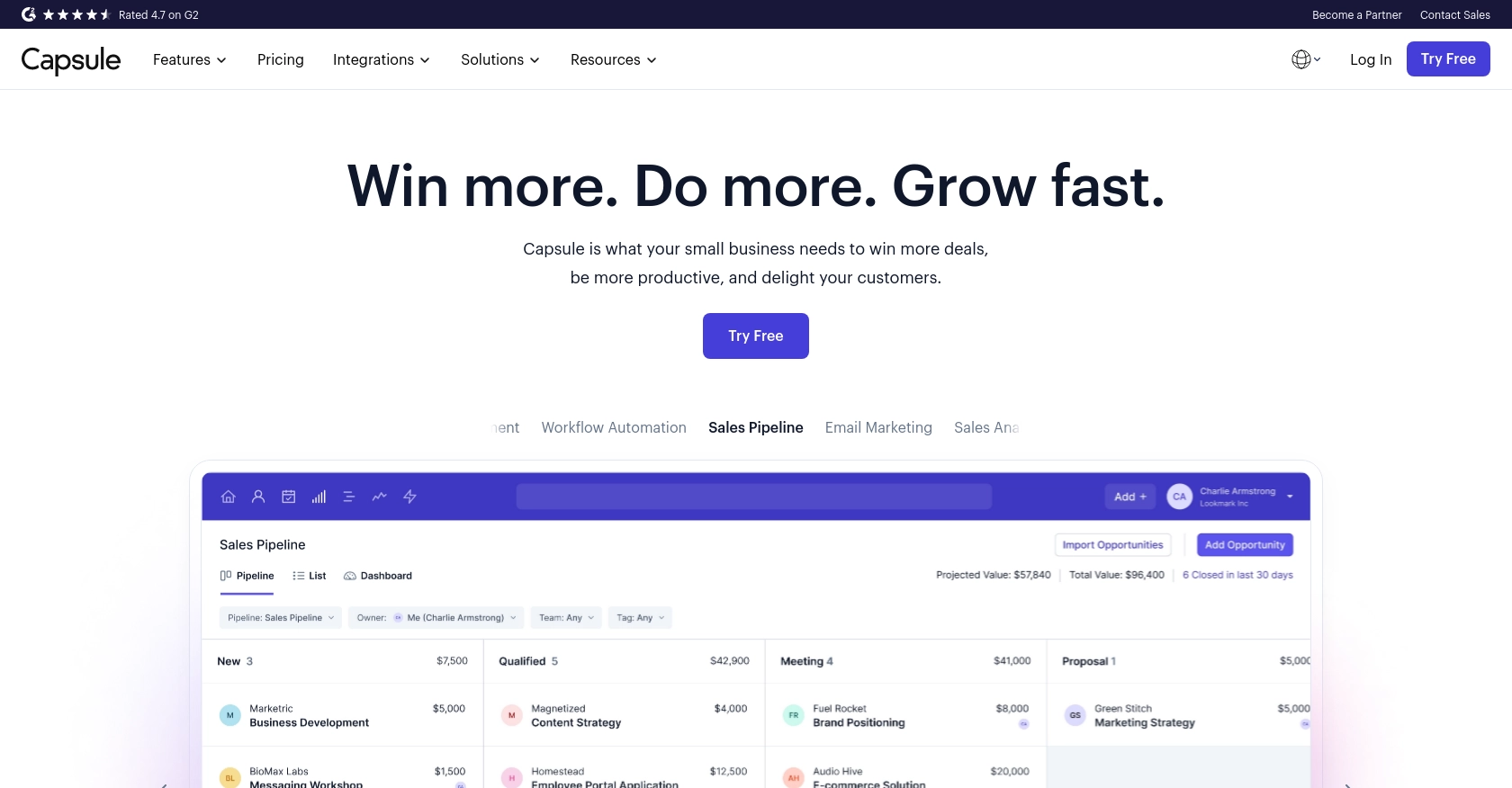
Introduction to Capsule CRM API
Capsule CRM is a robust customer relationship management platform designed to help businesses manage their customer interactions and streamline their sales processes. With features like contact management, sales tracking, and task management, Capsule CRM is a popular choice for businesses looking to enhance their customer engagement strategies.
Integrating with Capsule CRM's API allows developers to access and manage user data efficiently. For example, you might want to retrieve user information to personalize customer interactions or automate user-related tasks within your application. This article will guide you through using PHP to interact with the Capsule API, focusing on retrieving user data.
Setting Up Your Capsule CRM Test Account
Before you can start interacting with the Capsule API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting any live data. Capsule CRM offers a straightforward process to get started with their API using OAuth 2.0 authentication.
Creating a Capsule CRM Account
If you don't already have a Capsule CRM account, you can sign up for a free trial on their website. This trial will give you access to all the features you need to test the API integration.
- Visit the Capsule CRM signup page.
- Follow the instructions to create your account.
- Once your account is set up, log in to access the dashboard.
Registering Your Application for OAuth 2.0 Authentication
To interact with the Capsule API, you need to register your application to obtain the necessary client credentials for OAuth 2.0 authentication.
- Navigate to the "My Preferences" section in your Capsule account.
- Go to "API Authentication Tokens" and click on "Register a New Application."
- Fill in the required details, such as the application name and redirect URI.
- After registration, you'll receive a client ID and client secret. Keep these credentials secure as they are essential for API access.
Generating an Access Token
With your application registered, you can now generate an access token to authenticate API requests.
- Redirect users to the Capsule authorization URL:
https://api.capsulecrm.com/oauth/authorise
with the necessary query parameters (response_type, client_id, redirect_uri, scope, state). - Once the user authorizes your application, Capsule will redirect back to your specified redirect URI with an authorization code.
- Exchange this authorization code for an access token by making a POST request to
https://api.capsulecrm.com/oauth/token
with the required parameters (code, client_id, client_secret, grant_type). - Store the access token securely as it will be used in the Authorization header for API requests.
For more detailed information on authentication, refer to the Capsule API Authentication documentation.
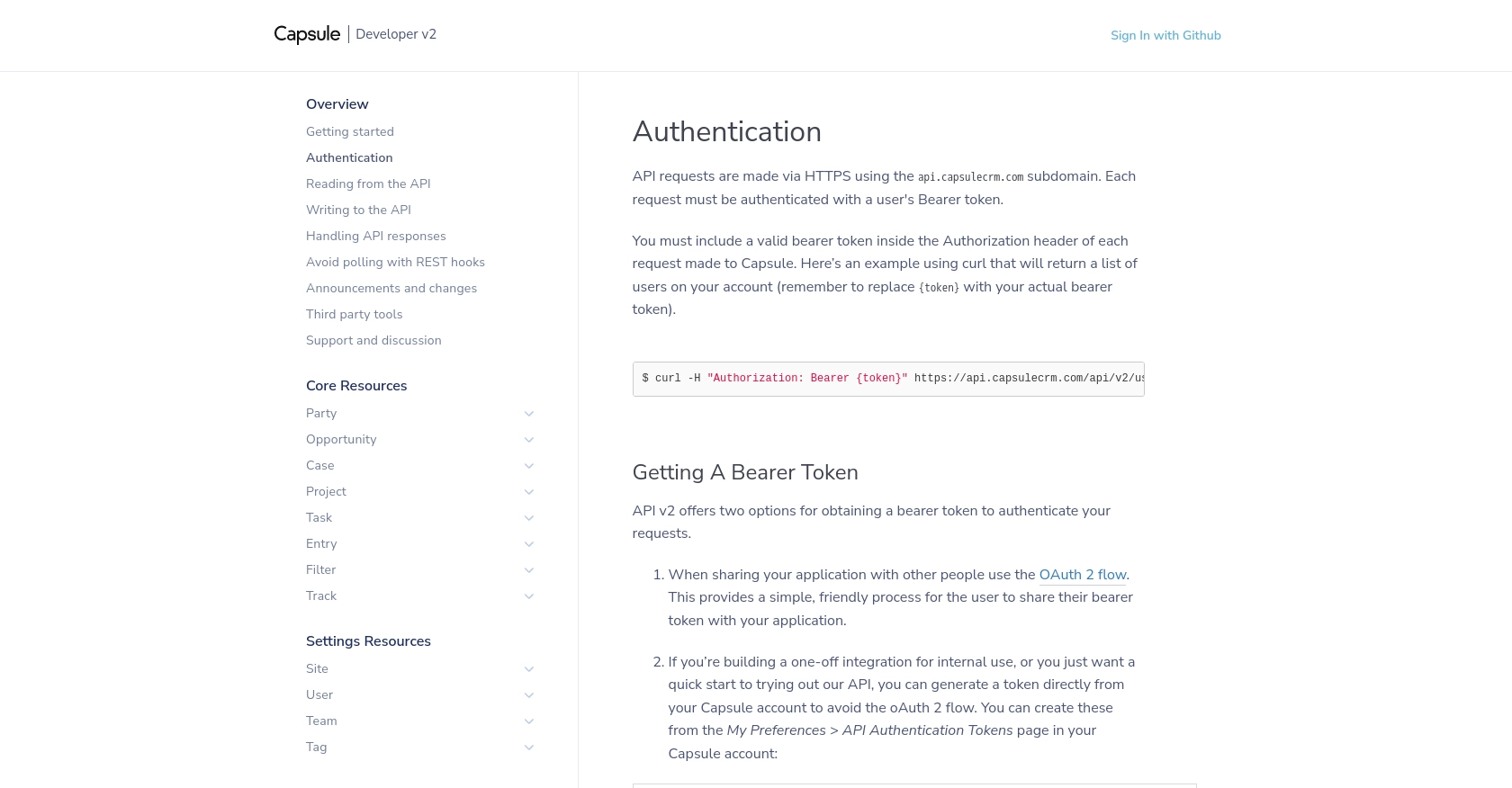
sbb-itb-96038d7
Making API Calls to Retrieve Users from Capsule CRM Using PHP
Once you have set up your Capsule CRM account and obtained the necessary access token, you can start making API calls to retrieve user data. This section will guide you through the process of using PHP to interact with the Capsule API, specifically focusing on retrieving a list of users.
Prerequisites for Capsule API Integration with PHP
Before proceeding, ensure you have the following installed on your development environment:
- PHP 7.4 or higher
- cURL extension for PHP
These tools will allow you to make HTTP requests to the Capsule API and handle the responses effectively.
Installing Necessary PHP Dependencies
To make HTTP requests in PHP, you can use the cURL extension. Ensure it's enabled in your php.ini
file:
extension=curl
Example Code to Retrieve Users from Capsule CRM
Below is a PHP example demonstrating how to retrieve a list of users from Capsule CRM using the API:
<?php
// Set the API endpoint
$endpoint = "https://api.capsulecrm.com/api/v2/users";
// Set the access token
$accessToken = "Your_Access_Token";
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Authorization: Bearer $accessToken",
"Content-Type: application/json"
]);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
// Parse the JSON response
$data = json_decode($response, true);
// Display user information
foreach ($data['users'] as $user) {
echo "User ID: " . $user['id'] . "\n";
echo "Name: " . $user['name'] . "\n";
echo "Username: " . $user['username'] . "\n";
echo "Status: " . $user['status'] . "\n\n";
}
}
// Close the cURL session
curl_close($ch);
?>
Replace Your_Access_Token
with the access token you obtained during the authentication process.
Verifying the API Request Success
After running the PHP script, you should see a list of users printed in your terminal. This confirms that the API call was successful. You can verify the retrieved data by cross-referencing it with the user information in your Capsule CRM dashboard.
Handling Errors and API Response Codes
It's crucial to handle potential errors when making API requests. The Capsule API will return specific HTTP status codes for different scenarios:
- 200 OK: The request was successful, and the response contains the requested data.
- 401 Unauthorized: The access token is invalid or expired. Ensure your token is correct and not expired.
- 403 Forbidden: The token does not have the necessary permissions to access the resource.
- 429 Too Many Requests: You have exceeded the rate limit of 4,000 requests per hour. Implement rate limiting strategies to avoid this.
For more details on handling API responses, refer to the Capsule API Handling API Responses documentation.
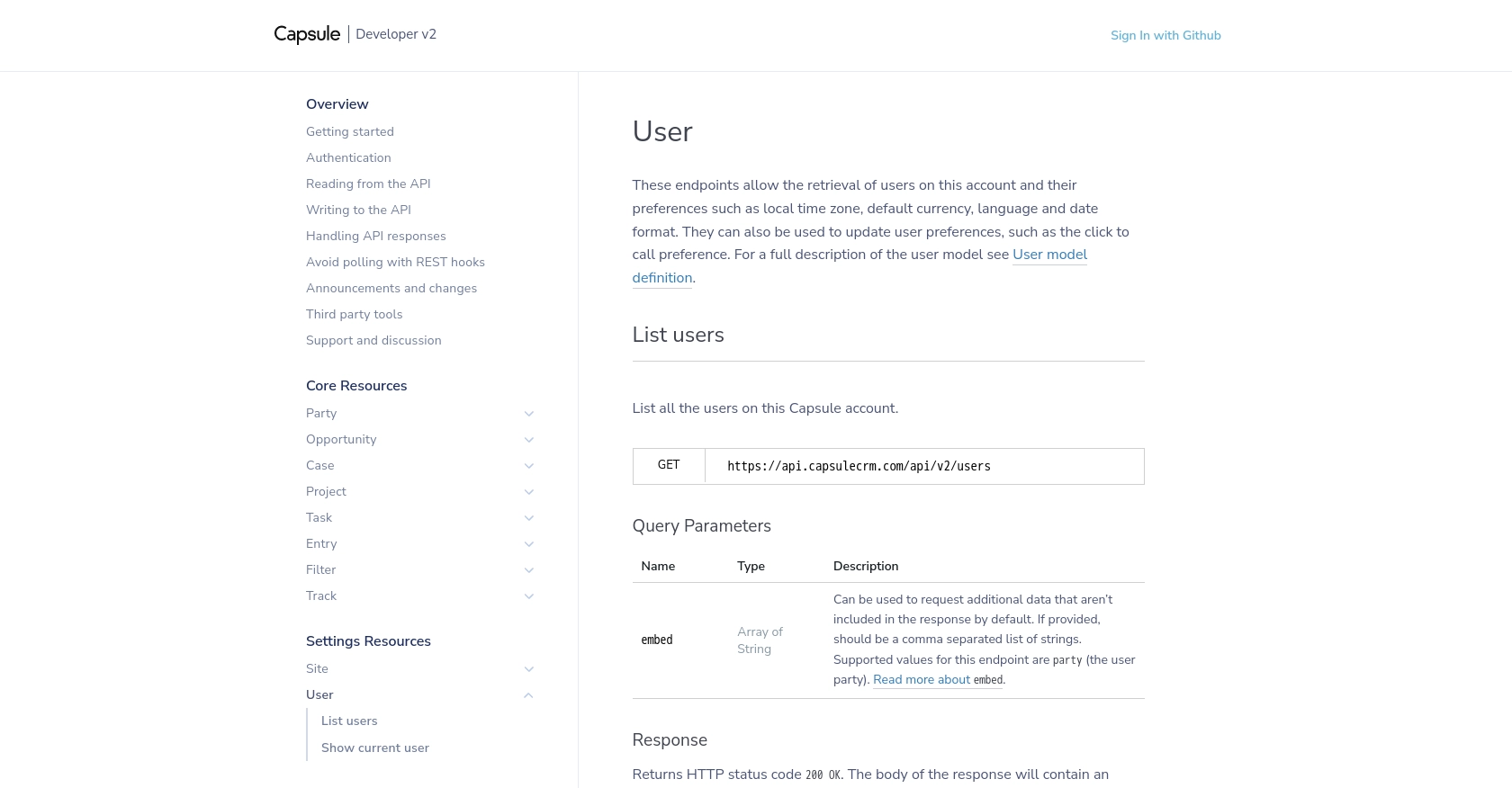
Best Practices for Capsule API Integration and Error Handling
When integrating with the Capsule API, it's essential to follow best practices to ensure a smooth and secure experience. Here are some recommendations:
- Securely Store Access Tokens: Always store access tokens securely, using environment variables or secure vaults, to prevent unauthorized access.
- Implement Rate Limiting: Capsule API allows up to 4,000 requests per hour. Monitor your API usage and implement rate limiting to avoid exceeding this limit. Use the
X-RateLimit-Remaining
header to track your usage. - Handle Errors Gracefully: Implement error handling to manage different HTTP status codes. For example, retry requests after a
429 Too Many Requests
response, and refresh tokens when receiving a401 Unauthorized
error. - Data Standardization: Ensure that data retrieved from Capsule is standardized and transformed as needed to fit your application's requirements.
Leveraging Endgrate for Efficient Capsule API Integrations
While building integrations with Capsule CRM can be rewarding, it can also be time-consuming and complex. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to multiple platforms, including Capsule CRM.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the complexities of API integrations.
- Build Once, Use Everywhere: Develop a single integration for multiple platforms, reducing redundancy and maintenance efforts.
- Enhance Customer Experience: Provide an intuitive and seamless integration experience for your users.
Explore how Endgrate can simplify your integration process by visiting Endgrate.
Read More
Ready to get started?