How to Get Product Variants with the BigCommerce API in Javascript
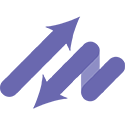
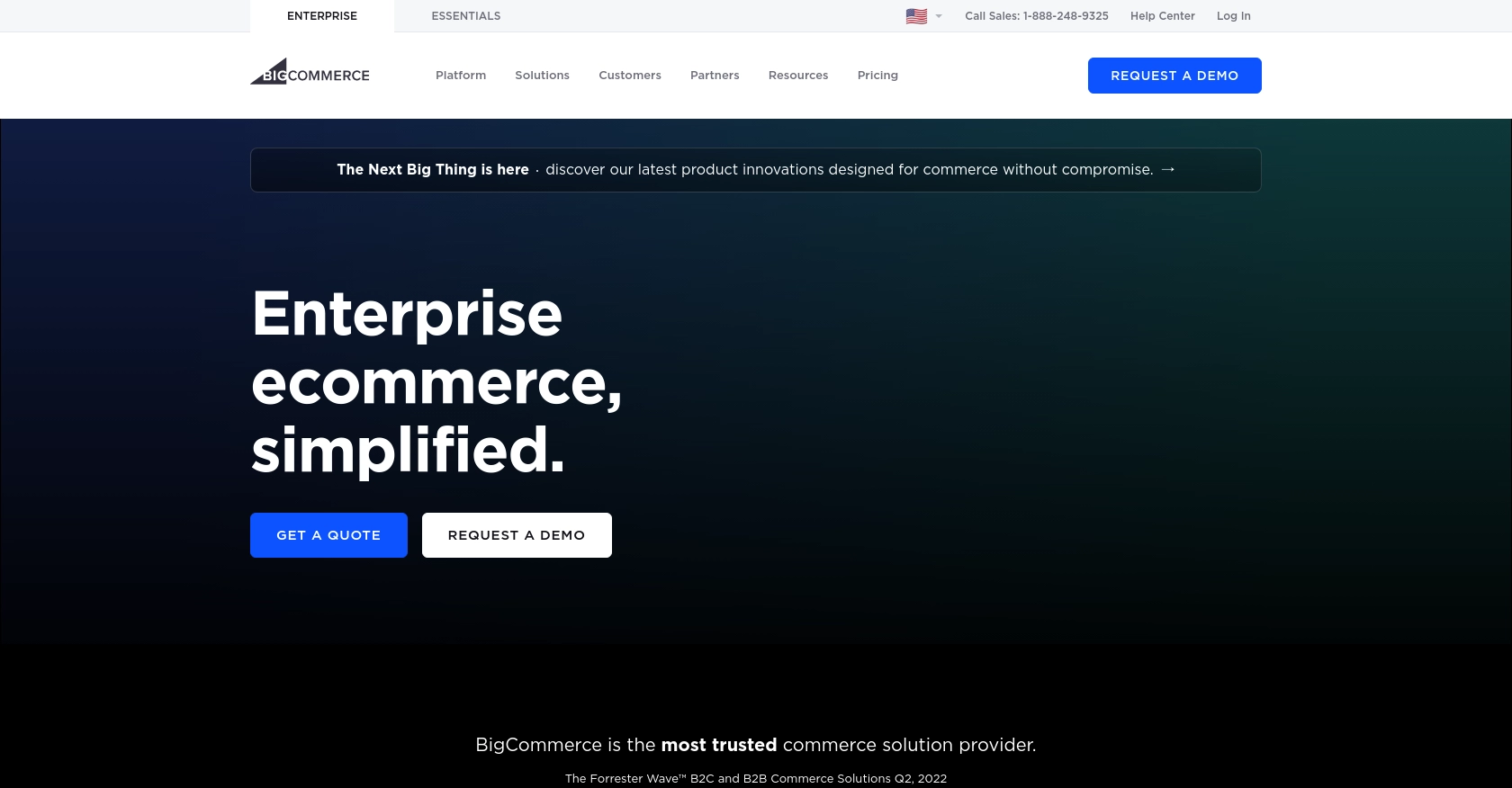
Introduction to BigCommerce API Integration
BigCommerce is a robust e-commerce platform that empowers businesses to create and manage online stores with ease. It offers a wide range of features, including product management, order processing, and customer engagement tools, making it a popular choice for businesses looking to expand their online presence.
Integrating with the BigCommerce API allows developers to access and manipulate store data programmatically. This can be particularly useful for managing product variants, which are different versions of a product with unique attributes such as size or color. For example, a developer might want to retrieve all product variants to update inventory levels or synchronize product data across multiple platforms.
This article will guide you through the process of using JavaScript to interact with the BigCommerce API, specifically focusing on retrieving product variants. By the end of this tutorial, you'll be equipped with the knowledge to efficiently manage product variants within your BigCommerce store.
Setting Up Your BigCommerce Test Account for API Integration
Before you can start interacting with the BigCommerce API to manage product variants, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your live store data.
Creating a BigCommerce Sandbox Account
To begin, you'll need to create a BigCommerce sandbox account. This account provides a testing environment where you can explore the API's capabilities.
- Visit the BigCommerce Sandbox page and sign up for a free sandbox account.
- Follow the on-screen instructions to complete the registration process. Once registered, you'll have access to a sandbox store where you can test API interactions.
Generating API Credentials for BigCommerce
With your sandbox account ready, the next step is to generate API credentials. These credentials will allow you to authenticate your API requests.
- Log in to your BigCommerce sandbox store's control panel.
- Navigate to Advanced Settings > API Accounts.
- Click on Create API Account and select Create V2/V3 API Token.
- Fill in the required details, such as the name of the API account and the API path.
- Set the necessary OAuth scopes to ensure your API account has permission to access product variants. Refer to the BigCommerce Authentication Documentation for guidance on selecting the appropriate scopes.
- Once configured, click Save to generate your API credentials, including the
X-Auth-Token
.
Configuring API Authentication
BigCommerce uses the X-Auth-Token
header for authenticating API requests. Ensure you have your access token ready, as you'll need it to make authorized requests to the BigCommerce API.
Here's an example of how to structure your API request headers:
// Example of setting up headers for API requests
const headers = {
'X-Auth-Token': 'your_access_token',
'Accept': 'application/json',
'Content-Type': 'application/json'
};
With your sandbox account and API credentials set up, you're now ready to start making API calls to retrieve product variants from your BigCommerce store.
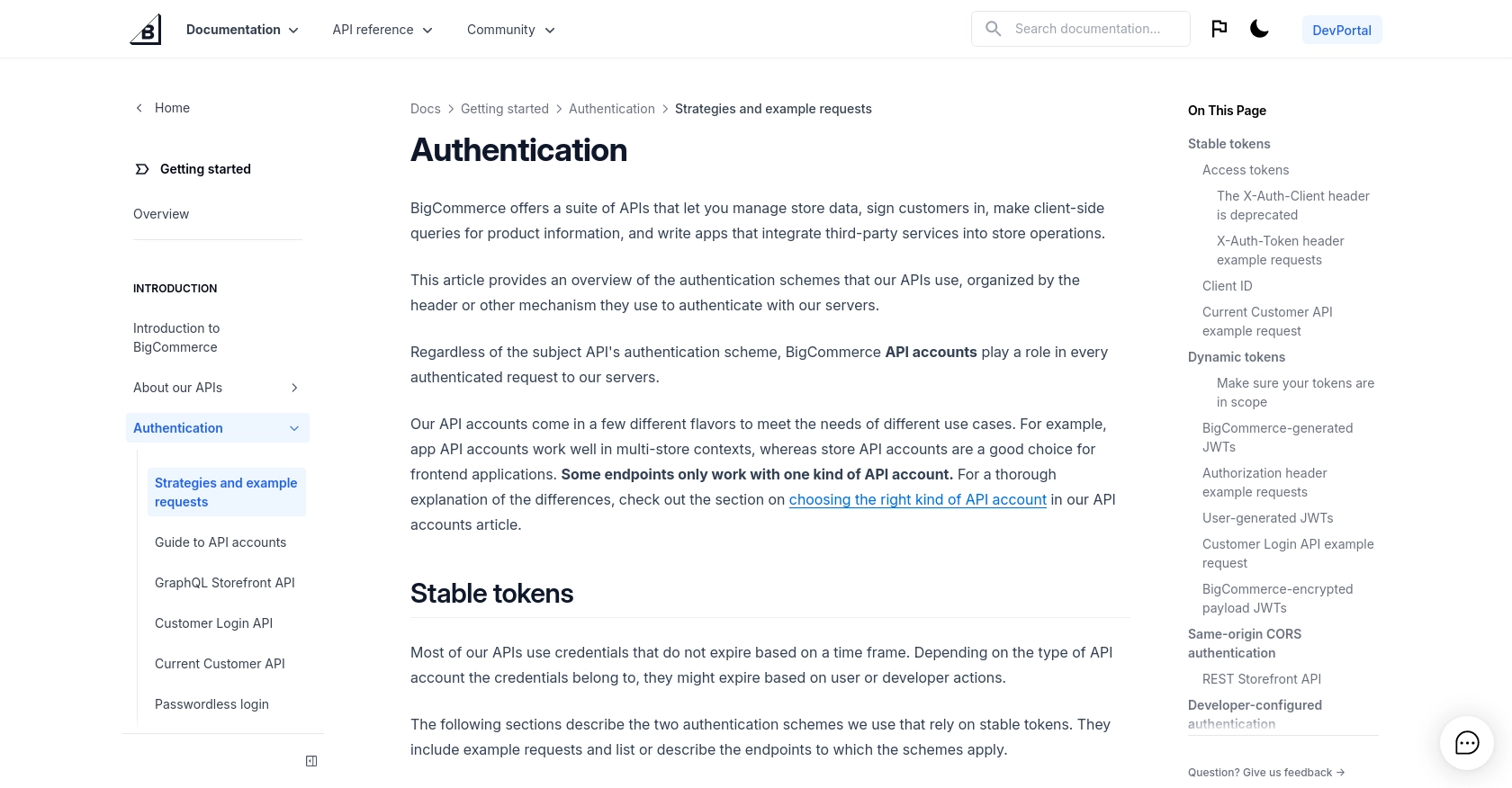
sbb-itb-96038d7
Making API Calls to Retrieve Product Variants from BigCommerce Using JavaScript
With your BigCommerce sandbox account and API credentials in place, you can now proceed to make API calls to retrieve product variants. This section will guide you through the process of using JavaScript to interact with the BigCommerce API effectively.
Setting Up Your JavaScript Environment for BigCommerce API Integration
Before making API calls, ensure your JavaScript environment is properly configured. You'll need Node.js installed on your machine to run JavaScript code outside a browser environment. Additionally, you'll use the node-fetch
library to make HTTP requests.
- Install Node.js from the official website if you haven't already.
- Initialize a new Node.js project and install the
node-fetch
library by running the following commands in your terminal:
npm init -y
npm install node-fetch
Writing JavaScript Code to Fetch Product Variants from BigCommerce
Now, let's write the JavaScript code to make a GET request to the BigCommerce API and retrieve product variants. You'll need to replace your_access_token
, store_hash
, and product_id
with your actual credentials and identifiers.
const fetch = require('node-fetch');
// Define the API endpoint and headers
const storeHash = 'your_store_hash';
const productId = 'your_product_id';
const endpoint = `https://api.bigcommerce.com/stores/${storeHash}/v3/catalog/products/${productId}/variants`;
const headers = {
'X-Auth-Token': 'your_access_token',
'Accept': 'application/json',
'Content-Type': 'application/json'
};
// Function to fetch product variants
async function getProductVariants() {
try {
const response = await fetch(endpoint, { headers });
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
const data = await response.json();
console.log('Product Variants:', data);
} catch (error) {
console.error('Error fetching product variants:', error);
}
}
// Call the function
getProductVariants();
Understanding the API Response and Handling Errors
When you run the above code, it will output the product variants associated with the specified product ID. The response will include details such as SKU, price, and inventory levels.
It's crucial to handle potential errors gracefully. The code checks for HTTP errors and logs them to the console. You can further enhance error handling by implementing retries or logging errors to a monitoring service.
Verifying API Call Success in Your BigCommerce Sandbox
After executing the API call, verify the success by checking the retrieved product variants against your BigCommerce sandbox store. Ensure the data matches the expected product variants for the specified product ID.
By following these steps, you can efficiently retrieve and manage product variants using the BigCommerce API and JavaScript, streamlining your e-commerce operations.
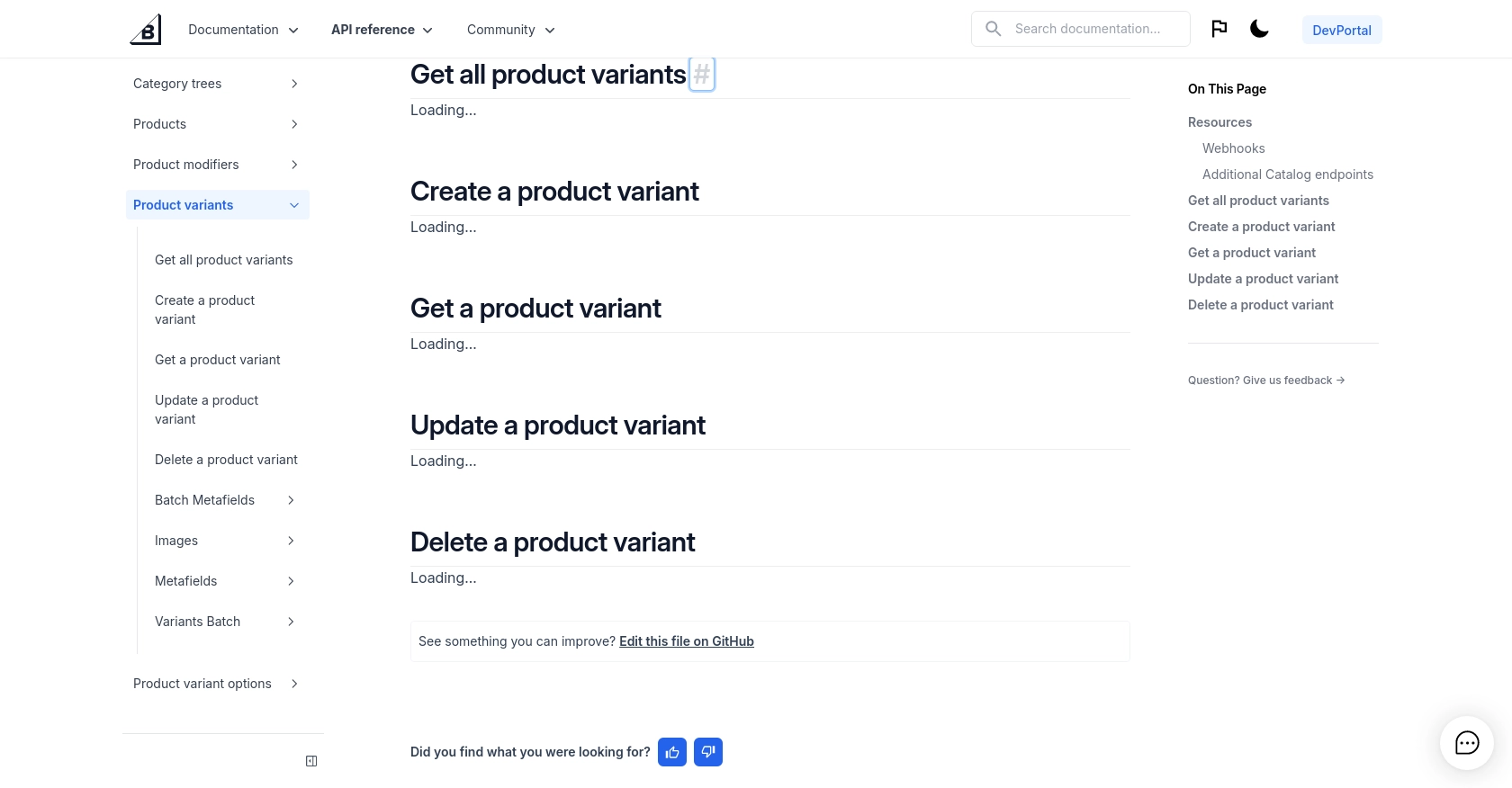
Conclusion and Best Practices for BigCommerce API Integration Using JavaScript
Integrating with the BigCommerce API using JavaScript provides a powerful way to manage product variants and streamline your e-commerce operations. By following the steps outlined in this guide, you can efficiently retrieve and manipulate product data, ensuring your online store remains up-to-date and competitive.
Best Practices for Secure and Efficient BigCommerce API Usage
- Securely Store API Credentials: Always store your API credentials securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of BigCommerce's API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Optimize Data Handling: When dealing with large datasets, consider paginating your API requests to manage data efficiently and reduce load times.
- Log and Monitor API Activity: Implement logging and monitoring to track API usage and errors. This will help you identify and resolve issues quickly, ensuring a smooth integration experience.
Streamline Your Integration Process with Endgrate
While integrating with the BigCommerce API can be highly beneficial, it can also be time-consuming and complex. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including BigCommerce. This allows you to build integrations once and deploy them across various platforms, saving time and resources.
By leveraging Endgrate, you can focus on your core product development while ensuring a seamless integration experience for your customers. Visit Endgrate to learn more about how you can simplify your integration process and enhance your e-commerce capabilities.
Read More
Ready to get started?